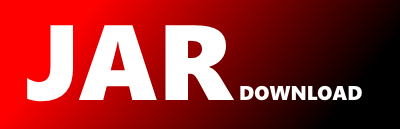
com.vaadin.polymer.app.widget.AppPouchdbQuery Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-pouchdb project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app.widget;
import com.vaadin.polymer.app.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* app-pouchdb-query
allows for declarative, read-only querying into a PouchDB
database. The semantics for querying match those of the
pouchdb-find plugin.
* In order to create an app-pouchdb-query
against any field other than _id
, at
least one index needs to have been created in your PouchDB database. You can use
app-pouchdb-index
to do this declaratively. For example:
* <app-pouchdb-index
* db-name="cats"
* fields='["name"]'>
* </app-pouchdb-index>
* <app-pouchdb-query
* db-name="cats"
* selector="name $exists true"
* fields='["_id","name"]'
* sort='["name"]'
* data="{{cats}}">
* </app-pouchdb-query>
*
* In the above example, an index is created on the “name” field of the “cats”
database. This allows the query to select by the “name” field, and sort by the
“name” field.
* By default, PouchDB creates an index on the “_id” field, so if you don’t
particularly care about sorting or selecting on other fields, you don’t need to
create any extra indexes.
* Describing selectors
* This element requires a specialized selector syntax that maps to the semantics
of the pouchdb-find plugin. For example, if you wish to create the following
selector:
* {
* series: 'Mario',
* debut: { $gt: 1990 }
* }
*
* You should format the selector
property this way:
* "series $eq 'Mario', debut $gt 1990"
*
* This makes selectors more convenient to write declaratively, while still
maintaining the ability to express selectors with full fidelity. For more
documentation on pouchdb-find selectors, please check out the docs
here.
*/
public class AppPouchdbQuery extends PolymerWidget {
/**
* Default Constructor.
*/
public AppPouchdbQuery() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public AppPouchdbQuery(String html) {
super(AppPouchdbQueryElement.TAG, AppPouchdbQueryElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public AppPouchdbQueryElement getPolymerElement() {
return (AppPouchdbQueryElement) getElement();
}
/**
* If true, all attempts to “put” or “post” values into the database
will be automatically structured as an “upsert”, where documents are
updated if already available, or created if not.
*
* JavaScript Info:
* @property upsert
* @type Boolean
* @behavior AppPouchdbQuery
*/
public boolean getUpsert() {
return getPolymerElement().getUpsert();
}
/**
* If true, all attempts to “put” or “post” values into the database
will be automatically structured as an “upsert”, where documents are
updated if already available, or created if not.
*
* JavaScript Info:
* @property upsert
* @type Boolean
* @behavior AppPouchdbQuery
*/
public void setUpsert(boolean value) {
getPolymerElement().setUpsert(value);
}
/**
* The fields to include in the returned documents.
*
* JavaScript Info:
* @property fields
* @type Array
*
*/
public JsArray getFields() {
return getPolymerElement().getFields();
}
/**
* The fields to include in the returned documents.
*
* JavaScript Info:
* @property fields
* @type Array
*
*/
public void setFields(JsArray value) {
getPolymerElement().setFields(value);
}
/**
* The results of the query, if any.
*
* JavaScript Info:
* @property data
* @type Array
*
*/
public JsArray getData() {
return getPolymerElement().getData();
}
/**
* The results of the query, if any.
*
* JavaScript Info:
* @property data
* @type Array
*
*/
public void setData(JsArray value) {
getPolymerElement().setData(value);
}
/**
* If desired, assign a function that implements a conflict resolution
strategy. This conflict resolution strategy will take precedence when
a conflict occurs, and will prevent conflict notification events from
being fired.
* Consider using an app-pouchdb-conflict-resolver
element instead for
a more declarative experience!
*
* JavaScript Info:
* @property resolveConflict
* @type Function
* @behavior AppPouchdbQuery
*/
public Function getResolveConflict() {
return getPolymerElement().getResolveConflict();
}
/**
* If desired, assign a function that implements a conflict resolution
strategy. This conflict resolution strategy will take precedence when
a conflict occurs, and will prevent conflict notification events from
being fired.
* Consider using an app-pouchdb-conflict-resolver
element instead for
a more declarative experience!
*
* JavaScript Info:
* @property resolveConflict
* @type Function
* @behavior AppPouchdbQuery
*/
public void setResolveConflict(Function value) {
getPolymerElement().setResolveConflict(value);
}
/**
* A list of field names to sort by. Fields in this list must have
indexes created for them.
*
* JavaScript Info:
* @property sort
* @type Array
*
*/
public JsArray getSort() {
return getPolymerElement().getSort();
}
/**
* A list of field names to sort by. Fields in this list must have
indexes created for them.
*
* JavaScript Info:
* @property sort
* @type Array
*
*/
public void setSort(JsArray value) {
getPolymerElement().setSort(value);
}
/**
* An object representing the parsed form of the selector, mapping to
a valid selector value as described in
the pouchdb-find docs.
*
* JavaScript Info:
* @property parsedSelector
* @type Object
*
*/
public JavaScriptObject getParsedSelector() {
return getPolymerElement().getParsedSelector();
}
/**
* An object representing the parsed form of the selector, mapping to
a valid selector value as described in
the pouchdb-find docs.
*
* JavaScript Info:
* @property parsedSelector
* @type Object
*
*/
public void setParsedSelector(JavaScriptObject value) {
getPolymerElement().setParsedSelector(value);
}
/**
* A configuration object suitable to be passed to the find
method of
the PouchDB database. For more information, refer to the docs
here
*
* JavaScript Info:
* @property query
* @type Object
*
*/
public JavaScriptObject getQuery() {
return getPolymerElement().getQuery();
}
/**
* A configuration object suitable to be passed to the find
method of
the PouchDB database. For more information, refer to the docs
here
*
* JavaScript Info:
* @property query
* @type Object
*
*/
public void setQuery(JavaScriptObject value) {
getPolymerElement().setQuery(value);
}
/**
* A reference to the PouchDB database instance that has been created.
*
* JavaScript Info:
* @property db
* @type Object
* @behavior AppPouchdbQuery
*/
public JavaScriptObject getDb() {
return getPolymerElement().getDb();
}
/**
* A reference to the PouchDB database instance that has been created.
*
* JavaScript Info:
* @property db
* @type Object
* @behavior AppPouchdbQuery
*/
public void setDb(JavaScriptObject value) {
getPolymerElement().setDb(value);
}
/**
* The number of documents to skip before returning results that match
the query. In other words, the offset from the beginning of the
of the result set to start at.
*
* JavaScript Info:
* @property skip
* @type Number
*
*/
public double getSkip() {
return getPolymerElement().getSkip();
}
/**
* The number of documents to skip before returning results that match
the query. In other words, the offset from the beginning of the
of the result set to start at.
*
* JavaScript Info:
* @property skip
* @type Number
*
*/
public void setSkip(double value) {
getPolymerElement().setSkip(value);
}
/**
* The number of document revisions to keep track of. The default value
(0) indicates no limit.
*
* JavaScript Info:
* @property revsLimit
* @type Number
* @behavior AppPouchdbQuery
*/
public double getRevsLimit() {
return getPolymerElement().getRevsLimit();
}
/**
* The number of document revisions to keep track of. The default value
(0) indicates no limit.
*
* JavaScript Info:
* @property revsLimit
* @type Number
* @behavior AppPouchdbQuery
*/
public void setRevsLimit(double value) {
getPolymerElement().setRevsLimit(value);
}
/**
* The maximum number of documents that can be returned. The default (0)
specifies no limit.
*
* JavaScript Info:
* @property limit
* @type Number
*
*/
public double getLimit() {
return getPolymerElement().getLimit();
}
/**
* The maximum number of documents that can be returned. The default (0)
specifies no limit.
*
* JavaScript Info:
* @property limit
* @type Number
*
*/
public void setLimit(double value) {
getPolymerElement().setLimit(value);
}
/**
* The PouchDB adapter to use. For more information on PouchDB adapters,
please refer to the PouchDB documentation
here.
*
* JavaScript Info:
* @property adapter
* @type String
* @behavior AppPouchdbQuery
*/
public String getAdapter() {
return getPolymerElement().getAdapter();
}
/**
* The PouchDB adapter to use. For more information on PouchDB adapters,
please refer to the PouchDB documentation
here.
*
* JavaScript Info:
* @property adapter
* @type String
* @behavior AppPouchdbQuery
*/
public void setAdapter(String value) {
getPolymerElement().setAdapter(value);
}
/**
* The name of the database. This can be either a local database (such
as “cats”), or a remote one (e.g., “https://example.com:5678/cats“).
*
* JavaScript Info:
* @property dbName
* @type String
* @behavior AppPouchdbQuery
*/
public String getDbName() {
return getPolymerElement().getDbName();
}
/**
* The name of the database. This can be either a local database (such
as “cats”), or a remote one (e.g., “https://example.com:5678/cats“).
*
* JavaScript Info:
* @property dbName
* @type String
* @behavior AppPouchdbQuery
*/
public void setDbName(String value) {
getPolymerElement().setDbName(value);
}
/**
* The selector to use when querying for documents. Fields referenced
in the selector must have indexes created for them.
*
* JavaScript Info:
* @property selector
* @type String
*
*/
public String getSelector() {
return getPolymerElement().getSelector();
}
/**
* The selector to use when querying for documents. Fields referenced
in the selector must have indexes created for them.
*
* JavaScript Info:
* @property selector
* @type String
*
*/
public void setSelector(String value) {
getPolymerElement().setSelector(value);
}
// Needed in UIBinder
/**
* The results of the query, if any.
*
* JavaScript Info:
* @attribute data
*
*/
public void setData(String value) {
Polymer.property(this.getPolymerElement(), "data", value);
}
// Needed in UIBinder
/**
* A list of field names to sort by. Fields in this list must have
indexes created for them.
*
* JavaScript Info:
* @attribute sort
*
*/
public void setSort(String value) {
Polymer.property(this.getPolymerElement(), "sort", value);
}
// Needed in UIBinder
/**
* The maximum number of documents that can be returned. The default (0)
specifies no limit.
*
* JavaScript Info:
* @attribute limit
*
*/
public void setLimit(String value) {
Polymer.property(this.getPolymerElement(), "limit", value);
}
// Needed in UIBinder
/**
* The number of documents to skip before returning results that match
the query. In other words, the offset from the beginning of the
of the result set to start at.
*
* JavaScript Info:
* @attribute skip
*
*/
public void setSkip(String value) {
Polymer.property(this.getPolymerElement(), "skip", value);
}
// Needed in UIBinder
/**
* The number of document revisions to keep track of. The default value
(0) indicates no limit.
*
* JavaScript Info:
* @attribute revs-limit
* @behavior AppPouchdbQuery
*/
public void setRevsLimit(String value) {
Polymer.property(this.getPolymerElement(), "revsLimit", value);
}
// Needed in UIBinder
/**
* The fields to include in the returned documents.
*
* JavaScript Info:
* @attribute fields
*
*/
public void setFields(String value) {
Polymer.property(this.getPolymerElement(), "fields", value);
}
// Needed in UIBinder
/**
* A configuration object suitable to be passed to the find
method of
the PouchDB database. For more information, refer to the docs
here
*
* JavaScript Info:
* @attribute query
*
*/
public void setQuery(String value) {
Polymer.property(this.getPolymerElement(), "query", value);
}
// Needed in UIBinder
/**
* A reference to the PouchDB database instance that has been created.
*
* JavaScript Info:
* @attribute db
* @behavior AppPouchdbQuery
*/
public void setDb(String value) {
Polymer.property(this.getPolymerElement(), "db", value);
}
// Needed in UIBinder
/**
* An object representing the parsed form of the selector, mapping to
a valid selector value as described in
the pouchdb-find docs.
*
* JavaScript Info:
* @attribute parsed-selector
*
*/
public void setParsedSelector(String value) {
Polymer.property(this.getPolymerElement(), "parsedSelector", value);
}
/**
* PouchDB only notifies of additive changes to the result set of a query.
In order to keep the query results up to date with other types of
changes, this method can be called to perform the query again without
changing any of this element’s other properties.
*
* JavaScript Info:
* @method refresh
*
*
*/
public void refresh() {
getPolymerElement().refresh();
}
}