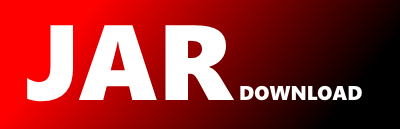
com.vaadin.polymer.app.widget.AppPouchdbSync Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-pouchdb project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app.widget;
import com.vaadin.polymer.app.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* app-pouchdb-sync
arranges for one-directional or bi-directional
synchronization between two PouchDB databases. For one-directional
synchronization, it forwards to PouchDB.replicate
, and for bi-directional
synchronization, it forwards to PouchDB.sync
.
* Here is an example of bi-directional synchronization between a local database
and a remote one:
* <app-pouchdb-sync
* src="cats"
* target="https://example.com:5678/cats"
* bidirectional>
* </app-pouchdb-sync>
*
* For more information on PouchDB synchronization topics, please refer to the
documentation here.
*/
public class AppPouchdbSync extends PolymerWidget {
/**
* Default Constructor.
*/
public AppPouchdbSync() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public AppPouchdbSync(String html) {
super(AppPouchdbSyncElement.TAG, AppPouchdbSyncElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public AppPouchdbSyncElement getPolymerElement() {
return (AppPouchdbSyncElement) getElement();
}
/**
* While false
, synchronization will only happen from the src
to the
target
. One-directional synchronization follows the semantics of
PouchDB.replicate
. Set to true
to make the sync bidirectional,
which uses PouchDB.sync
instead.
*
* JavaScript Info:
* @property bidirectional
* @type Boolean
*
*/
public boolean getBidirectional() {
return getPolymerElement().getBidirectional();
}
/**
* While false
, synchronization will only happen from the src
to the
target
. One-directional synchronization follows the semantics of
PouchDB.replicate
. Set to true
to make the sync bidirectional,
which uses PouchDB.sync
instead.
*
* JavaScript Info:
* @property bidirectional
* @type Boolean
*
*/
public void setBidirectional(boolean value) {
getPolymerElement().setBidirectional(value);
}
/**
* Set to true to log change events that are emitted by the sync
instance.
*
* JavaScript Info:
* @property log
* @type Boolean
*
*/
public boolean getLog() {
return getPolymerElement().getLog();
}
/**
* Set to true to log change events that are emitted by the sync
instance.
*
* JavaScript Info:
* @property log
* @type Boolean
*
*/
public void setLog(boolean value) {
getPolymerElement().setLog(value);
}
/**
* An event emitter that notifies of events in the synchronization
process.
*
* JavaScript Info:
* @property sync
* @type Object
*
*/
public JavaScriptObject getSync() {
return getPolymerElement().getSync();
}
/**
* An event emitter that notifies of events in the synchronization
process.
*
* JavaScript Info:
* @property sync
* @type Object
*
*/
public void setSync(JavaScriptObject value) {
getPolymerElement().setSync(value);
}
/**
* The source to sync from. If this sync is bidirectional
, then the
src
and target
values are interchangeable.
*
* JavaScript Info:
* @property src
* @type String
*
*/
public String getSrc() {
return getPolymerElement().getSrc();
}
/**
* The source to sync from. If this sync is bidirectional
, then the
src
and target
values are interchangeable.
*
* JavaScript Info:
* @property src
* @type String
*
*/
public void setSrc(String value) {
getPolymerElement().setSrc(value);
}
/**
* The target
to sync to. If this sync is bidirectional
, then the
src
and target
values are interchangeable.
*
* JavaScript Info:
* @property target
* @type String
*
*/
public String getTarget() {
return getPolymerElement().getTarget();
}
/**
* The target
to sync to. If this sync is bidirectional
, then the
src
and target
values are interchangeable.
*
* JavaScript Info:
* @property target
* @type String
*
*/
public void setTarget(String value) {
getPolymerElement().setTarget(value);
}
// Needed in UIBinder
/**
* An event emitter that notifies of events in the synchronization
process.
*
* JavaScript Info:
* @attribute sync
*
*/
public void setSync(String value) {
Polymer.property(this.getPolymerElement(), "sync", value);
}
}