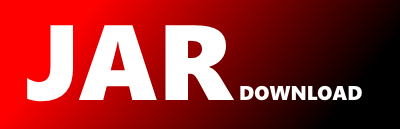
com.vaadin.polymer.app.widget.AppRoute Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-route project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app.widget;
import com.vaadin.polymer.app.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* app-route
is an element that enables declarative, self-describing routing
for a web app.
*
* n.b. app-route is still in beta. We expect it will need some changes. We’re counting on your feedback!
*
* In its typical usage, a app-route
element consumes an object that describes
some state about the current route, via the route
property. It then parses
that state using the pattern
property, and produces two artifacts: some data
related to the route
, and a tail
that contains the rest of the route
that
did not match.
* Here is a basic example, when used with app-location
:
* <app-location route="{{route}}"></app-location>
* <app-route
* route="{{route}}"
* pattern="/:page"
* data="{{data}}"
* tail="{{tail}}">
* </app-route>
*
*
*
In the above example, the app-location
produces a route
value. Then, the
route.path
property is matched by comparing it to the pattern
property. If
the pattern
property matches route.path
, the app-route
will set or update
its data
property with an object whose properties correspond to the parameters
in pattern
. So, in the above example, if route.path
was '/about'
, the value
of data
would be {"page": "about"}
.
* The tail
property represents the remaining part of the route state after the
pattern
has been applied to a matching route
.
* Here is another example, where tail
is used:
* <app-location route="{{route}}"></app-location>
* <app-route
* route="{{route}}"
* pattern="/:page"
* data="{{routeData}}"
* tail="{{subroute}}">
* </app-route>
* <app-route
* route="{{subroute}}"
* pattern="/:id"
* data="{{subrouteData}}">
* </app-route>
*
*
*
In the above example, there are two app-route
elements. The first
app-route
consumes a route
. When the route
is matched, the first
app-route
also produces routeData
from its data
, and subroute
from
its tail
. The second app-route
consumes the subroute
, and when it
matches, it produces an object called subrouteData
from its data
.
* So, when route.path
is '/about'
, the routeData
object will look like
this: { page: 'about' }
* And subrouteData
will be null. However, if route.path
changes to
'/article/123'
, the routeData
object will look like this:
{ page: 'article' }
* And the subrouteData
will look like this: { id: '123' }
* app-route
is responsive to bi-directional changes to the data
objects
they produce. So, if routeData.page
changed from 'article'
to 'about'
,
the app-route
will update route.path
. This in-turn will update the
app-location
, and cause the global location bar to change its value.
*/
public class AppRoute extends PolymerWidget {
/**
* Default Constructor.
*/
public AppRoute() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public AppRoute(String html) {
super(AppRouteElement.TAG, AppRouteElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public AppRouteElement getPolymerElement() {
return (AppRouteElement) getElement();
}
/**
* Whether the current route is active. True if route.path
matches the
pattern
, false otherwise.
*
* JavaScript Info:
* @property active
* @type Boolean
*
*/
public boolean getActive() {
return getPolymerElement().getActive();
}
/**
* Whether the current route is active. True if route.path
matches the
pattern
, false otherwise.
*
* JavaScript Info:
* @property active
* @type Boolean
*
*/
public void setActive(boolean value) {
getPolymerElement().setActive(value);
}
/**
* The parameterized values that are extracted from the route as
described by pattern
.
*
* JavaScript Info:
* @property data
* @type Object
*
*/
public JavaScriptObject getData() {
return getPolymerElement().getData();
}
/**
* The parameterized values that are extracted from the route as
described by pattern
.
*
* JavaScript Info:
* @property data
* @type Object
*
*/
public void setData(JavaScriptObject value) {
getPolymerElement().setData(value);
}
/**
*
*
* JavaScript Info:
* @property queryParams
* @type ?Object
*
*/
public JavaScriptObject getQueryParams() {
return getPolymerElement().getQueryParams();
}
/**
*
*
* JavaScript Info:
* @property queryParams
* @type ?Object
*
*/
public void setQueryParams(JavaScriptObject value) {
getPolymerElement().setQueryParams(value);
}
/**
* The URL component managed by this element.
*
* JavaScript Info:
* @property route
* @type Object
*
*/
public JavaScriptObject getRoute() {
return getPolymerElement().getRoute();
}
/**
* The URL component managed by this element.
*
* JavaScript Info:
* @property route
* @type Object
*
*/
public void setRoute(JavaScriptObject value) {
getPolymerElement().setRoute(value);
}
/**
* The part of route.path
NOT consumed by pattern
.
*
* JavaScript Info:
* @property tail
* @type Object
*
*/
public JavaScriptObject getTail() {
return getPolymerElement().getTail();
}
/**
* The part of route.path
NOT consumed by pattern
.
*
* JavaScript Info:
* @property tail
* @type Object
*
*/
public void setTail(JavaScriptObject value) {
getPolymerElement().setTail(value);
}
/**
* The pattern of slash-separated segments to match route.path
against.
* For example the pattern “/foo” will match “/foo” or “/foo/bar”
but not “/foobar”.
* Path segments like /:named
are mapped to properties on the data
object.
*
* JavaScript Info:
* @property pattern
* @type String
*
*/
public String getPattern() {
return getPolymerElement().getPattern();
}
/**
* The pattern of slash-separated segments to match route.path
against.
* For example the pattern “/foo” will match “/foo” or “/foo/bar”
but not “/foobar”.
* Path segments like /:named
are mapped to properties on the data
object.
*
* JavaScript Info:
* @property pattern
* @type String
*
*/
public void setPattern(String value) {
getPolymerElement().setPattern(value);
}
// Needed in UIBinder
/**
* The parameterized values that are extracted from the route as
described by pattern
.
*
* JavaScript Info:
* @attribute data
*
*/
public void setData(String value) {
Polymer.property(this.getPolymerElement(), "data", value);
}
// Needed in UIBinder
/**
*
*
* JavaScript Info:
* @attribute query-params
*
*/
public void setQueryParams(String value) {
Polymer.property(this.getPolymerElement(), "queryParams", value);
}
// Needed in UIBinder
/**
* The URL component managed by this element.
*
* JavaScript Info:
* @attribute route
*
*/
public void setRoute(String value) {
Polymer.property(this.getPolymerElement(), "route", value);
}
// Needed in UIBinder
/**
* The part of route.path
NOT consumed by pattern
.
*
* JavaScript Info:
* @attribute tail
*
*/
public void setTail(String value) {
Polymer.property(this.getPolymerElement(), "tail", value);
}
}