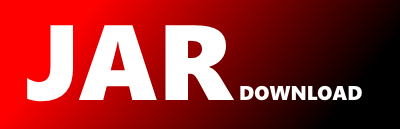
com.vaadin.polymer.hydrolysis.widget.HydrolysisAnalyzer Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from hydrolysis project by unknown author
* that is licensed with unknown license.
*/
package com.vaadin.polymer.hydrolysis.widget;
import com.vaadin.polymer.hydrolysis.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
*
*/
public class HydrolysisAnalyzer extends PolymerWidget {
/**
* Default Constructor.
*/
public HydrolysisAnalyzer() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public HydrolysisAnalyzer(String html) {
super(HydrolysisAnalyzerElement.TAG, HydrolysisAnalyzerElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public HydrolysisAnalyzerElement getPolymerElement() {
return (HydrolysisAnalyzerElement) getElement();
}
/**
* The resultant Analyzer
object from Hydrolysis.
*
* JavaScript Info:
* @property analyzer
* @type Object
*
*/
public JavaScriptObject getAnalyzer() {
return getPolymerElement().getAnalyzer();
}
/**
* The resultant Analyzer
object from Hydrolysis.
*
* JavaScript Info:
* @property analyzer
* @type Object
*
*/
public void setAnalyzer(JavaScriptObject value) {
getPolymerElement().setAnalyzer(value);
}
/**
* Whether the hydrolysis descriptors should be cleaned of redundant
properties.
*
* JavaScript Info:
* @property clean
* @type Boolean
*
*/
public boolean getClean() {
return getPolymerElement().getClean();
}
/**
* Whether the hydrolysis descriptors should be cleaned of redundant
properties.
*
* JavaScript Info:
* @property clean
* @type Boolean
*
*/
public void setClean(boolean value) {
getPolymerElement().setClean(value);
}
/**
* Whether the analyzer is loading/analyzing resources.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
public boolean getLoading() {
return getPolymerElement().getLoading();
}
/**
* Whether the analyzer is loading/analyzing resources.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
public void setLoading(boolean value) {
getPolymerElement().setLoading(value);
}
/**
* Whether all dependencies should be loaded and analyzed.
* Turning this on will probably slow down the load process dramatically.
*
* JavaScript Info:
* @property transitive
* @type Boolean
*
*/
public boolean getTransitive() {
return getPolymerElement().getTransitive();
}
/**
* Whether all dependencies should be loaded and analyzed.
* Turning this on will probably slow down the load process dramatically.
*
* JavaScript Info:
* @property transitive
* @type Boolean
*
*/
public void setTransitive(boolean value) {
getPolymerElement().setTransitive(value);
}
/**
*
*
* JavaScript Info:
* @property resolver
* @type String
*
*/
public String getResolver() {
return getPolymerElement().getResolver();
}
/**
*
*
* JavaScript Info:
* @property resolver
* @type String
*
*/
public void setResolver(String value) {
getPolymerElement().setResolver(value);
}
/**
* The URL to an import that declares (or transitively imports) the
elements that you wish to see analyzed.
* If the URL is relative, it will be resolved relative to the master
document.
* If you change this value after the <hydrolysis-analyzer>
has been
instantiated, you must call analyze()
.
*
* JavaScript Info:
* @property src
* @type String
*
*/
public String getSrc() {
return getPolymerElement().getSrc();
}
/**
* The URL to an import that declares (or transitively imports) the
elements that you wish to see analyzed.
* If the URL is relative, it will be resolved relative to the master
document.
* If you change this value after the <hydrolysis-analyzer>
has been
instantiated, you must call analyze()
.
*
* JavaScript Info:
* @property src
* @type String
*
*/
public void setSrc(String value) {
getPolymerElement().setSrc(value);
}
// Needed in UIBinder
/**
* The resultant Analyzer
object from Hydrolysis.
*
* JavaScript Info:
* @attribute analyzer
*
*/
public void setAnalyzer(String value) {
Polymer.property(this.getPolymerElement(), "analyzer", value);
}
/**
* Begins loading the imports referenced by src
.
* If you make changes to this element’s configuration, you must call this
function to kick off another analysis pass.
*
* JavaScript Info:
* @method analyze
*
*
*/
public void analyze() {
getPolymerElement().analyze();
}
}