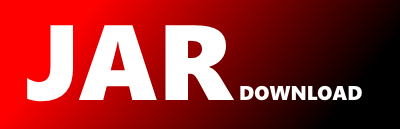
com.vaadin.polymer.iron.IronA11yKeysBehavior Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-a11y-keys-behavior project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Polymer.IronA11yKeysBehavior
provides a normalized interface for processing
keyboard commands that pertain to WAI-ARIA best practices.
The element takes care of browser differences with respect to Keyboard events
and uses an expressive syntax to filter key presses.
* Use the keyBindings
prototype property to express what combination of keys
will trigger the callback. A key binding has the format
"KEY+MODIFIER:EVENT": "callback"
("KEY": "callback"
or
"KEY:EVENT": "callback"
are valid as well). Some examples:
* keyBindings: {
* 'space': '_onKeydown', // same as 'space:keydown'
* 'shift+tab': '_onKeydown',
* 'enter:keypress': '_onKeypress',
* 'esc:keyup': '_onKeyup'
* }
*
*
*
The callback will receive with an event containing the following information in event.detail
:
* _onKeydown: function(event) {
* console.log(event.detail.combo); // KEY+MODIFIER, e.g. "shift+tab"
* console.log(event.detail.key); // KEY only, e.g. "tab"
* console.log(event.detail.event); // EVENT, e.g. "keydown"
* console.log(event.detail.keyboardEvent); // the original KeyboardEvent
* }
*
*
*
Use the keyEventTarget
attribute to set up event handlers on a specific
node.
* See the demo source code
for an example.
*/
@JsType(isNative=true)
public interface IronA11yKeysBehavior {
@JsOverlay public static final String NAME = "Polymer.IronA11yKeysBehavior";
@JsOverlay public static final String SRC = "iron-a11y-keys-behavior/iron-a11y-keys-behavior.html";
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @property keyBindings
* @type !Object
* @behavior VaadinDatePicker
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @property keyBindings
* @type !Object
* @behavior VaadinDatePicker
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getStopKeyboardEventPropagation();
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setStopKeyboardEventPropagation(boolean value);
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
void addOwnKeyBinding(Object eventString, Object handlerName);
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString);
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
void removeOwnKeyBindings();
}