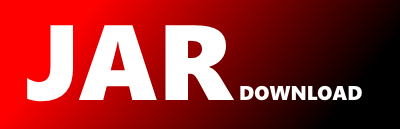
com.vaadin.polymer.iron.IronAjaxElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-ajax project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* The iron-ajax
element exposes network request functionality.
* <iron-ajax
* auto
* url="https://www.googleapis.com/youtube/v3/search"
* params='{"part":"snippet", "q":"polymer", "key": "YOUTUBE_API_KEY", "type": "video"}'
* handle-as="json"
* on-response="handleResponse"
* debounce-duration="300"></iron-ajax>
*
*
*
With auto
set to true
, the element performs a request whenever
its url
, params
or body
properties are changed. Automatically generated
requests will be debounced in the case that multiple attributes are changed
sequentially.
* Note: The params
attribute must be double quoted JSON.
* You can trigger a request explicitly by calling generateRequest
on the
element.
*/
@JsType(isNative=true)
public interface IronAjaxElement extends HTMLElement {
@JsOverlay public static final String TAG = "iron-ajax";
@JsOverlay public static final String SRC = "iron-ajax/iron-ajax.html";
/**
* An Array of all in-flight requests originating from this iron-ajax
element.
*
* JavaScript Info:
* @property activeRequests
* @type Array
*
*/
@JsProperty JsArray getActiveRequests();
/**
* An Array of all in-flight requests originating from this iron-ajax
element.
*
* JavaScript Info:
* @property activeRequests
* @type Array
*
*/
@JsProperty void setActiveRequests(JsArray value);
/**
* By default, iron-ajax’s events do not bubble. Setting this attribute will cause its
request and response events as well as its iron-ajax-request, -response, and -error
events to bubble to the window object. The vanilla error event never bubbles when
using shadow dom even if this.bubbles is true because a scoped flag is not passed with
it (first link) and because the shadow dom spec did not used to allow certain events,
including events named error, to leak outside of shadow trees (second link).
https://www.w3.org/TR/shadow-dom/#scoped-flag
https://www.w3.org/TR/2015/WD-shadow-dom-20151215/#events-that-are-not-leaked-into-ancestor-trees
*
* JavaScript Info:
* @property bubbles
* @type Boolean
*
*/
@JsProperty boolean getBubbles();
/**
* By default, iron-ajax’s events do not bubble. Setting this attribute will cause its
request and response events as well as its iron-ajax-request, -response, and -error
events to bubble to the window object. The vanilla error event never bubbles when
using shadow dom even if this.bubbles is true because a scoped flag is not passed with
it (first link) and because the shadow dom spec did not used to allow certain events,
including events named error, to leak outside of shadow trees (second link).
https://www.w3.org/TR/shadow-dom/#scoped-flag
https://www.w3.org/TR/2015/WD-shadow-dom-20151215/#events-that-are-not-leaked-into-ancestor-trees
*
* JavaScript Info:
* @property bubbles
* @type Boolean
*
*/
@JsProperty void setBubbles(boolean value);
/**
* Set the withCredentials flag on the request.
*
* JavaScript Info:
* @property withCredentials
* @type Boolean
*
*/
@JsProperty boolean getWithCredentials();
/**
* Set the withCredentials flag on the request.
*
* JavaScript Info:
* @property withCredentials
* @type Boolean
*
*/
@JsProperty void setWithCredentials(boolean value);
/**
* Length of time in milliseconds to debounce multiple automatically generated requests.
*
* JavaScript Info:
* @property debounceDuration
* @type Number
*
*/
@JsProperty double getDebounceDuration();
/**
* Length of time in milliseconds to debounce multiple automatically generated requests.
*
* JavaScript Info:
* @property debounceDuration
* @type Number
*
*/
@JsProperty void setDebounceDuration(double value);
/**
* If true, error messages will automatically be logged to the console.
*
* JavaScript Info:
* @property verbose
* @type Boolean
*
*/
@JsProperty boolean getVerbose();
/**
* If true, error messages will automatically be logged to the console.
*
* JavaScript Info:
* @property verbose
* @type Boolean
*
*/
@JsProperty void setVerbose(boolean value);
/**
* If true, automatically performs an Ajax request when either url
or
params
changes.
*
* JavaScript Info:
* @property auto
* @type Boolean
*
*/
@JsProperty boolean getAuto();
/**
* If true, automatically performs an Ajax request when either url
or
params
changes.
*
* JavaScript Info:
* @property auto
* @type Boolean
*
*/
@JsProperty void setAuto(boolean value);
/**
* Set the timeout flag on the request.
*
* JavaScript Info:
* @property timeout
* @type Number
*
*/
@JsProperty double getTimeout();
/**
* Set the timeout flag on the request.
*
* JavaScript Info:
* @property timeout
* @type Number
*
*/
@JsProperty void setTimeout(double value);
/**
* Toggle whether XHR is synchronous or asynchronous. Don’t change this
to true unless You Know What You Are Doing™.
*
* JavaScript Info:
* @property sync
* @type Boolean
*
*/
@JsProperty boolean getSync();
/**
* Toggle whether XHR is synchronous or asynchronous. Don’t change this
to true unless You Know What You Are Doing™.
*
* JavaScript Info:
* @property sync
* @type Boolean
*
*/
@JsProperty void setSync(boolean value);
/**
* lastRequest’s error, if any.
*
* JavaScript Info:
* @property lastError
* @type Object
*
*/
@JsProperty JavaScriptObject getLastError();
/**
* lastRequest’s error, if any.
*
* JavaScript Info:
* @property lastError
* @type Object
*
*/
@JsProperty void setLastError(JavaScriptObject value);
/**
* The most recent request made by this iron-ajax element.
*
* JavaScript Info:
* @property lastRequest
* @type Object
*
*/
@JsProperty JavaScriptObject getLastRequest();
/**
* The most recent request made by this iron-ajax element.
*
* JavaScript Info:
* @property lastRequest
* @type Object
*
*/
@JsProperty void setLastRequest(JavaScriptObject value);
/**
* lastRequest’s response.
* Note that lastResponse and lastError are set when lastRequest finishes,
so if loading is true, then lastResponse and lastError will correspond
to the result of the previous request.
* The type of the response is determined by the value of handleAs
at
the time that the request was generated.
*
* JavaScript Info:
* @property lastResponse
* @type Object
*
*/
@JsProperty JavaScriptObject getLastResponse();
/**
* lastRequest’s response.
* Note that lastResponse and lastError are set when lastRequest finishes,
so if loading is true, then lastResponse and lastError will correspond
to the result of the previous request.
* The type of the response is determined by the value of handleAs
at
the time that the request was generated.
*
* JavaScript Info:
* @property lastResponse
* @type Object
*
*/
@JsProperty void setLastResponse(JavaScriptObject value);
/**
* HTTP request headers to send.
* Example:
* <iron-ajax
* auto
* url="http://somesite.com"
* headers='{"X-Requested-With": "XMLHttpRequest"}'
* handle-as="json"></iron-ajax>
*
*
*
Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
@JsProperty JavaScriptObject getHeaders();
/**
* HTTP request headers to send.
* Example:
* <iron-ajax
* auto
* url="http://somesite.com"
* headers='{"X-Requested-With": "XMLHttpRequest"}'
* handle-as="json"></iron-ajax>
*
*
*
Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
@JsProperty void setHeaders(JavaScriptObject value);
/**
* An object that contains query parameters to be appended to the
specified url
when generating a request. If you wish to set the body
content when making a POST request, you should use the body
property
instead.
*
* JavaScript Info:
* @property params
* @type Object
*
*/
@JsProperty JavaScriptObject getParams();
/**
* An object that contains query parameters to be appended to the
specified url
when generating a request. If you wish to set the body
content when making a POST request, you should use the body
property
instead.
*
* JavaScript Info:
* @property params
* @type Object
*
*/
@JsProperty void setParams(JavaScriptObject value);
/**
* Body content to send with the request, typically used with “POST”
requests.
* If body is a string it will be sent unmodified.
* If Content-Type is set to a value listed below, then
the body will be encoded accordingly.
*
* content-type="application/json"
* - body is encoded like
{"foo":"bar baz","x":1}
*
*
* content-type="application/x-www-form-urlencoded"
* - body is encoded like
foo=bar+baz&x=1
*
*
*
* Otherwise the body will be passed to the browser unmodified, and it
will handle any encoding (e.g. for FormData, Blob, ArrayBuffer).
*
* JavaScript Info:
* @property body
* @type Object
*
*/
@JsProperty JavaScriptObject getBody();
/**
* Body content to send with the request, typically used with “POST”
requests.
* If body is a string it will be sent unmodified.
* If Content-Type is set to a value listed below, then
the body will be encoded accordingly.
*
* content-type="application/json"
* - body is encoded like
{"foo":"bar baz","x":1}
*
*
* content-type="application/x-www-form-urlencoded"
* - body is encoded like
foo=bar+baz&x=1
*
*
*
* Otherwise the body will be passed to the browser unmodified, and it
will handle any encoding (e.g. for FormData, Blob, ArrayBuffer).
*
* JavaScript Info:
* @property body
* @type Object
*
*/
@JsProperty void setBody(JavaScriptObject value);
/**
* True while lastRequest is in flight.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
@JsProperty boolean getLoading();
/**
* True while lastRequest is in flight.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
@JsProperty void setLoading(boolean value);
/**
* The HTTP method to use such as ‘GET’, ‘POST’, ‘PUT’, or ‘DELETE’.
Default is ‘GET’.
*
* JavaScript Info:
* @property method
* @type String
*
*/
@JsProperty String getMethod();
/**
* The HTTP method to use such as ‘GET’, ‘POST’, ‘PUT’, or ‘DELETE’.
Default is ‘GET’.
*
* JavaScript Info:
* @property method
* @type String
*
*/
@JsProperty void setMethod(String value);
/**
* Prefix to be stripped from a JSON response before parsing it.
* In order to prevent an attack using CSRF with Array responses
(http://haacked.com/archive/2008/11/20/anatomy-of-a-subtle-json-vulnerability.aspx/)
many backends will mitigate this by prefixing all JSON response bodies
with a string that would be nonsensical to a JavaScript parser.
*
* JavaScript Info:
* @property jsonPrefix
* @type String
*
*/
@JsProperty String getJsonPrefix();
/**
* Prefix to be stripped from a JSON response before parsing it.
* In order to prevent an attack using CSRF with Array responses
(http://haacked.com/archive/2008/11/20/anatomy-of-a-subtle-json-vulnerability.aspx/)
many backends will mitigate this by prefixing all JSON response bodies
with a string that would be nonsensical to a JavaScript parser.
*
* JavaScript Info:
* @property jsonPrefix
* @type String
*
*/
@JsProperty void setJsonPrefix(String value);
/**
* The URL target of the request.
*
* JavaScript Info:
* @property url
* @type String
*
*/
@JsProperty String getUrl();
/**
* The URL target of the request.
*
* JavaScript Info:
* @property url
* @type String
*
*/
@JsProperty void setUrl(String value);
/**
* Specifies what data to store in the response
property, and
to deliver as event.detail.response
in response
events.
* One of:
* text
: uses XHR.responseText
.
* xml
: uses XHR.responseXML
.
* json
: uses XHR.responseText
parsed as JSON.
* arraybuffer
: uses XHR.response
.
* blob
: uses XHR.response
.
* document
: uses XHR.response
.
*
* JavaScript Info:
* @property handleAs
* @type String
*
*/
@JsProperty String getHandleAs();
/**
* Specifies what data to store in the response
property, and
to deliver as event.detail.response
in response
events.
* One of:
* text
: uses XHR.responseText
.
* xml
: uses XHR.responseXML
.
* json
: uses XHR.responseText
parsed as JSON.
* arraybuffer
: uses XHR.response
.
* blob
: uses XHR.response
.
* document
: uses XHR.response
.
*
* JavaScript Info:
* @property handleAs
* @type String
*
*/
@JsProperty void setHandleAs(String value);
/**
* Content type to use when sending data. If the contentType
property
is set and a Content-Type
header is specified in the headers
property, the headers
property value will take precedence.
* Varies the handling of the body
param.
*
* JavaScript Info:
* @property contentType
* @type String
*
*/
@JsProperty String getContentType();
/**
* Content type to use when sending data. If the contentType
property
is set and a Content-Type
header is specified in the headers
property, the headers
property value will take precedence.
* Varies the handling of the body
param.
*
* JavaScript Info:
* @property contentType
* @type String
*
*/
@JsProperty void setContentType(String value);
/**
* Performs an AJAX request to the specified URL.
*
* JavaScript Info:
* @method generateRequest
*
* @return {JavaScriptObject}
*/
JavaScriptObject generateRequest();
/**
* Request options suitable for generating an iron-request
instance based
on the current state of the iron-ajax
instance’s properties.
*
* JavaScript Info:
* @method toRequestOptions
*
* @return {Object}
*/
Object toRequestOptions();
}