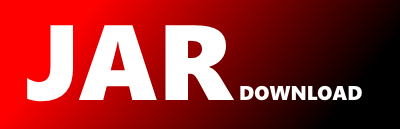
com.vaadin.polymer.iron.IronComponentPageElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-component-page project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Loads Polymer element and behavior documentation using
Hydrolysis and renders a complete
documentation page including demos (if available).
* To display a warning inside an iron-component-page
element,
add the .warning
class to a child.
*/
@JsType(isNative=true)
public interface IronComponentPageElement extends HTMLElement {
@JsOverlay public static final String TAG = "iron-component-page";
@JsOverlay public static final String SRC = "iron-component-page/iron-component-page.html";
/**
* Toggle flag to be used when this element is being displayed in the
Polymer Elements catalog.
*
* JavaScript Info:
* @property catalog
* @type Boolean
*
*/
@JsProperty boolean getCatalog();
/**
* Toggle flag to be used when this element is being displayed in the
Polymer Elements catalog.
*
* JavaScript Info:
* @property catalog
* @type Boolean
*
*/
@JsProperty void setCatalog(boolean value);
/**
* The Hydrolysis behavior descriptors that have been loaded.
*
* JavaScript Info:
* @property docBehaviors
* @type Array
*
*/
@JsProperty JsArray getDocBehaviors();
/**
* The Hydrolysis behavior descriptors that have been loaded.
*
* JavaScript Info:
* @property docBehaviors
* @type Array
*
*/
@JsProperty void setDocBehaviors(JsArray value);
/**
* Demos for the currently selected element.
*
* JavaScript Info:
* @property docDemos
* @type Array
*
*/
@JsProperty JsArray getDocDemos();
/**
* Demos for the currently selected element.
*
* JavaScript Info:
* @property docDemos
* @type Array
*
*/
@JsProperty void setDocDemos(JsArray value);
/**
* The Hydrolysis element descriptors that have been loaded.
*
* JavaScript Info:
* @property docElements
* @type Array
*
*/
@JsProperty JsArray getDocElements();
/**
* The Hydrolysis element descriptors that have been loaded.
*
* JavaScript Info:
* @property docElements
* @type Array
*
*/
@JsProperty void setDocElements(JsArray value);
/**
* Whether all dependencies should be loaded and documented.
* Turning this on will probably slow down the load process dramatically.
*
* JavaScript Info:
* @property transitive
* @type Boolean
*
*/
@JsProperty boolean getTransitive();
/**
* Whether all dependencies should be loaded and documented.
* Turning this on will probably slow down the load process dramatically.
*
* JavaScript Info:
* @property transitive
* @type Boolean
*
*/
@JsProperty void setTransitive(boolean value);
/**
* The relative root for determining paths to demos and default source
detection.
*
* JavaScript Info:
* @property base
* @type String
*
*/
@JsProperty String getBase();
/**
* The relative root for determining paths to demos and default source
detection.
*
* JavaScript Info:
* @property base
* @type String
*
*/
@JsProperty void setBase(String value);
/**
* The scroll mode for the page. For details about the modes,
see the mode property in paper-header-panel.
*
* JavaScript Info:
* @property scrollMode
* @type String
*
*/
@JsProperty String getScrollMode();
/**
* The scroll mode for the page. For details about the modes,
see the mode property in paper-header-panel.
*
* JavaScript Info:
* @property scrollMode
* @type String
*
*/
@JsProperty void setScrollMode(String value);
/**
* The URL to an import that declares (or transitively imports) the
elements that you wish to see documented.
* If the URL is relative, it will be resolved relative to the master
document.
* If a src
URL is not specified, it will resolve the name of the
directory containing this element, followed by dirname.html
. For
example:
* awesome-sauce/index.html
:
* <iron-doc-viewer></iron-doc-viewer>
*
*
*
Would implicitly have src="awesome-sauce.html"
.
*
* JavaScript Info:
* @property src
* @type String
*
*/
@JsProperty String getSrc();
/**
* The URL to an import that declares (or transitively imports) the
elements that you wish to see documented.
* If the URL is relative, it will be resolved relative to the master
document.
* If a src
URL is not specified, it will resolve the name of the
directory containing this element, followed by dirname.html
. For
example:
* awesome-sauce/index.html
:
* <iron-doc-viewer></iron-doc-viewer>
*
*
*
Would implicitly have src="awesome-sauce.html"
.
*
* JavaScript Info:
* @property src
* @type String
*
*/
@JsProperty void setSrc(String value);
/**
* The URL to a precompiled JSON descriptor. If you have precompiled
and stored a documentation set using Hydrolysis, you can load the
analyzer directly via AJAX by specifying this attribute.
* If a doc-src
is not specified, it is ignored and the default
rules according to the src
attribute are used.
*
* JavaScript Info:
* @property docSrc
* @type String
*
*/
@JsProperty String getDocSrc();
/**
* The URL to a precompiled JSON descriptor. If you have precompiled
and stored a documentation set using Hydrolysis, you can load the
analyzer directly via AJAX by specifying this attribute.
* If a doc-src
is not specified, it is ignored and the default
rules according to the src
attribute are used.
*
* JavaScript Info:
* @property docSrc
* @type String
*
*/
@JsProperty void setDocSrc(String value);
/**
* An optional version string.
*
* JavaScript Info:
* @property version
* @type string
*
*/
@JsProperty String getVersion();
/**
* An optional version string.
*
* JavaScript Info:
* @property version
* @type string
*
*/
@JsProperty void setVersion(String value);
/**
* The current view. Can be docs
or demo
.
*
* JavaScript Info:
* @property view
* @type String
*
*/
@JsProperty String getView();
/**
* The current view. Can be docs
or demo
.
*
* JavaScript Info:
* @property view
* @type String
*
*/
@JsProperty void setView(String value);
/**
* The name of a branch used to fetch source for gh-pages.
If not specified master
is used.
*
* JavaScript Info:
* @property branchName
* @type String
*
*/
@JsProperty String getBranchName();
/**
* The name of a branch used to fetch source for gh-pages.
If not specified master
is used.
*
* JavaScript Info:
* @property branchName
* @type String
*
*/
@JsProperty void setBranchName(String value);
/**
* The element or behavior that will be displayed on the page. Defaults
to the element matching the name of the source file.
*
* JavaScript Info:
* @property active
* @type String
*
*/
@JsProperty String getActive();
/**
* The element or behavior that will be displayed on the page. Defaults
to the element matching the name of the source file.
*
* JavaScript Info:
* @property active
* @type String
*
*/
@JsProperty void setActive(String value);
/**
* Renders this element into static HTML for offline use.
* This is mostly useful for debugging and one-off documentation generation.
If you want to integrate doc generation into your build process, you
probably want to be calling hydrolysis.Analyzer.analyze()
directly.
*
* JavaScript Info:
* @method marshal
*
* @return {String}
*/
String marshal();
}