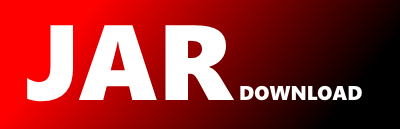
com.vaadin.polymer.iron.IronFitBehavior Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-fit-behavior project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Polymer.IronFitBehavior
fits an element in another element using max-height
and max-width
, and
optionally centers it in the window or another element.
* The element will only be sized and/or positioned if it has not already been sized and/or positioned
by CSS.
*
*
*
* CSS properties
* Action
*
*
*
*
* position
set
* Element is not centered horizontally or vertically
*
*
* top
or bottom
set
* Element is not vertically centered
*
*
* left
or right
set
* Element is not horizontally centered
*
*
* max-height
set
* Element respects max-height
*
*
* max-width
set
* Element respects max-width
*
*
*
* Polymer.IronFitBehavior
can position an element into another element using
verticalAlign
and horizontalAlign
. This will override the element’s css position.
* <div class="container">
* <iron-fit-impl vertical-align="top" horizontal-align="auto">
* Positioned into the container
* </iron-fit-impl>
* </div>
*
*
*
Use noOverlap
to position the element around another element without overlapping it.
* <div class="container">
* <iron-fit-impl no-overlap vertical-align="auto" horizontal-align="auto">
* Positioned around the container
* </iron-fit-impl>
* </div>
*
*
*
Use horizontalOffset, verticalOffset
to offset the element from its positionTarget
;
Polymer.IronFitBehavior
will collapse these in order to keep the element
within fitInto
boundaries, while preserving the element’s CSS margin values.
* <div class="container">
* <iron-fit-impl vertical-align="top" vertical-offset="20">
* With vertical offset
* </iron-fit-impl>
* </div>
*
*
*
*
*/
@JsType(isNative=true)
public interface IronFitBehavior {
@JsOverlay public static final String NAME = "Polymer.IronFitBehavior";
@JsOverlay public static final String SRC = "iron-fit-behavior/iron-fit-behavior.html";
/**
* Will position the element around the positionTarget without overlapping it.
*
* JavaScript Info:
* @property noOverlap
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getNoOverlap();
/**
* Will position the element around the positionTarget without overlapping it.
*
* JavaScript Info:
* @property noOverlap
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setNoOverlap(boolean value);
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by verticalAlign
.
* If verticalAlign
is “top”, this offset will increase or decrease
the distance to the top side of the screen: a negative offset will
move the dropdown upwards; a positive one, downwards.
* Conversely if verticalAlign
is “bottom”, this offset will increase
or decrease the distance to the bottom side of the screen: a negative
offset will move the dropdown downwards; a positive one, upwards.
*
* JavaScript Info:
* @property verticalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
@JsProperty double getVerticalOffset();
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by verticalAlign
.
* If verticalAlign
is “top”, this offset will increase or decrease
the distance to the top side of the screen: a negative offset will
move the dropdown upwards; a positive one, downwards.
* Conversely if verticalAlign
is “bottom”, this offset will increase
or decrease the distance to the bottom side of the screen: a negative
offset will move the dropdown downwards; a positive one, upwards.
*
* JavaScript Info:
* @property verticalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setVerticalOffset(double value);
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by horizontalAlign
.
* If horizontalAlign
is “left”, this offset will increase or decrease
the distance to the left side of the screen: a negative offset will
move the dropdown to the left; a positive one, to the right.
* Conversely if horizontalAlign
is “right”, this offset will increase
or decrease the distance to the right side of the screen: a negative
offset will move the dropdown to the right; a positive one, to the left.
*
* JavaScript Info:
* @property horizontalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
@JsProperty double getHorizontalOffset();
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by horizontalAlign
.
* If horizontalAlign
is “left”, this offset will increase or decrease
the distance to the left side of the screen: a negative offset will
move the dropdown to the left; a positive one, to the right.
* Conversely if horizontalAlign
is “right”, this offset will increase
or decrease the distance to the right side of the screen: a negative
offset will move the dropdown to the right; a positive one, to the left.
*
* JavaScript Info:
* @property horizontalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setHorizontalOffset(double value);
/**
* The element that should be used to position the element. If not set, it will
default to the parent node.
*
* JavaScript Info:
* @property positionTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty JavaScriptObject getPositionTarget();
/**
* The element that should be used to position the element. If not set, it will
default to the parent node.
*
* JavaScript Info:
* @property positionTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setPositionTarget(JavaScriptObject value);
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @property sizingTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty JavaScriptObject getSizingTarget();
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @property sizingTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setSizingTarget(JavaScriptObject value);
/**
* The element to fit this
into.
*
* JavaScript Info:
* @property fitInto
* @type Object
* @behavior VaadinContextMenuOverlay
*/
@JsProperty JavaScriptObject getFitInto();
/**
* The element to fit this
into.
*
* JavaScript Info:
* @property fitInto
* @type Object
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setFitInto(JavaScriptObject value);
/**
* Set to true to auto-fit on attach.
*
* JavaScript Info:
* @property autoFitOnAttach
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getAutoFitOnAttach();
/**
* Set to true to auto-fit on attach.
*
* JavaScript Info:
* @property autoFitOnAttach
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setAutoFitOnAttach(boolean value);
/**
* If true, it will use horizontalAlign
and verticalAlign
values as preferred alignment
and if there’s not enough space, it will pick the values which minimize the cropping.
*
* JavaScript Info:
* @property dynamicAlign
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getDynamicAlign();
/**
* If true, it will use horizontalAlign
and verticalAlign
values as preferred alignment
and if there’s not enough space, it will pick the values which minimize the cropping.
*
* JavaScript Info:
* @property dynamicAlign
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setDynamicAlign(boolean value);
/**
* The orientation against which to align the element vertically
relative to the positionTarget
. Possible values are “top”, “bottom”, “auto”.
*
* JavaScript Info:
* @property verticalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
@JsProperty String getVerticalAlign();
/**
* The orientation against which to align the element vertically
relative to the positionTarget
. Possible values are “top”, “bottom”, “auto”.
*
* JavaScript Info:
* @property verticalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setVerticalAlign(String value);
/**
* The orientation against which to align the element horizontally
relative to the positionTarget
. Possible values are “left”, “right”, “auto”.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
@JsProperty String getHorizontalAlign();
/**
* The orientation against which to align the element horizontally
relative to the positionTarget
. Possible values are “left”, “right”, “auto”.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setHorizontalAlign(String value);
/**
* Constrains the size of the element to fitInto
by setting max-height
and/or max-width
.
*
* JavaScript Info:
* @method constrain
* @behavior VaadinContextMenuOverlay
*
*/
void constrain();
/**
* Positions the element according to horizontalAlign, verticalAlign
.
*
* JavaScript Info:
* @method position
* @behavior VaadinContextMenuOverlay
*
*/
void position();
/**
* Equivalent to calling resetFit()
and fit()
. Useful to call this after
the element or the fitInto
element has been resized, or if any of the
positioning properties (e.g. horizontalAlign, verticalAlign
) is updated.
It preserves the scroll position of the sizingTarget.
*
* JavaScript Info:
* @method refit
* @behavior VaadinContextMenuOverlay
*
*/
void refit();
/**
* Resets the target element’s position and size constraints, and clear
the memoized data.
*
* JavaScript Info:
* @method resetFit
* @behavior VaadinContextMenuOverlay
*
*/
void resetFit();
/**
* Positions and fits the element into the fitInto
element.
*
* JavaScript Info:
* @method fit
* @behavior VaadinContextMenuOverlay
*
*/
void fit();
/**
* Centers horizontally and vertically if not already positioned. This also sets
position:fixed
.
*
* JavaScript Info:
* @method center
* @behavior VaadinContextMenuOverlay
*
*/
void center();
}