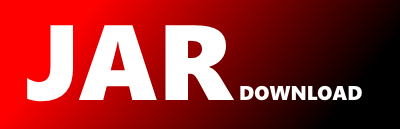
com.vaadin.polymer.iron.IronFormElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-form project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* <iron-form>
is an HTML <form>
element that can validate and submit any custom
elements that implement Polymer.IronFormElementBehavior
, as well as any
native HTML elements. For more information on which attributes are
available on the native form element, see https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form
* It supports both get
and post
methods, and uses an iron-ajax
element to
submit the form data to the action URL.
* Example:
* <form is="iron-form" id="form" method="post" action="/form/handler">
* <paper-input name="name" label="name"></paper-input>
* <input name="address">
* ...
* </form>
*
*
*
By default, a native <button>
element will submit this form. However, if you
want to submit it from a custom element’s click handler, you need to explicitly
call the form’s submit
method.
* Example:
* <paper-button raised onclick="submitForm()">Submit</paper-button>
*
* function submitForm() {
* document.getElementById('form').submit();
* }
*
*
*
To customize the request sent to the server, you can listen to the iron-form-presubmit
event, and modify the form’siron-ajax
object. However, If you want to not use iron-ajax
at all, you can cancel the
event and do your own custom submission:
* Example of modifying the request, but still using the build-in form submission:
* form.addEventListener('iron-form-presubmit', function() {
* this.request.method = 'put';
* this.request.params = someCustomParams;
* });
*
*
*
Example of bypassing the build-in form submission:
* form.addEventListener('iron-form-presubmit', function(event) {
* event.preventDefault();
* var firebase = new Firebase(form.getAttribute('action'));
* firebase.set(form.serialize());
* });
*
*
*
*/
@JsType(isNative=true)
public interface IronFormElement extends HTMLElement {
@JsOverlay public static final String TAG = "iron-form";
@JsOverlay public static final String SRC = "iron-form/iron-form.html";
/**
* HTTP request headers to send.
* Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
@JsProperty JavaScriptObject getHeaders();
/**
* HTTP request headers to send.
* Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
@JsProperty void setHeaders(JavaScriptObject value);
/**
* iron-ajax request object used to submit the form.
*
* JavaScript Info:
* @property request
* @type Object
*
*/
@JsProperty JavaScriptObject getRequest();
/**
* iron-ajax request object used to submit the form.
*
* JavaScript Info:
* @property request
* @type Object
*
*/
@JsProperty void setRequest(JavaScriptObject value);
/**
* By default, the form will display the browser’s native validation
UI (i.e. popup bubbles and invalid styles on invalid fields). You can
manually disable this; however, if you do, note that you will have to
manually style invalid native HTML fields yourself, as you are
explicitly preventing the native form from doing so.
*
* JavaScript Info:
* @property disableNativeValidationUi
* @type Boolean
*
*/
@JsProperty boolean getDisableNativeValidationUi();
/**
* By default, the form will display the browser’s native validation
UI (i.e. popup bubbles and invalid styles on invalid fields). You can
manually disable this; however, if you do, note that you will have to
manually style invalid native HTML fields yourself, as you are
explicitly preventing the native form from doing so.
*
* JavaScript Info:
* @property disableNativeValidationUi
* @type Boolean
*
*/
@JsProperty void setDisableNativeValidationUi(boolean value);
/**
* Set the withCredentials flag when sending data.
*
* JavaScript Info:
* @property withCredentials
* @type Boolean
*
*/
@JsProperty boolean getWithCredentials();
/**
* Set the withCredentials flag when sending data.
*
* JavaScript Info:
* @property withCredentials
* @type Boolean
*
*/
@JsProperty void setWithCredentials(boolean value);
/**
* Content type to use when sending data. If the contentType
property
is set and a Content-Type
header is specified in the headers
property, the headers
property value will take precedence.
If Content-Type is set to a value listed below, then
the body
(typically used with POST requests) will be encoded accordingly.
*
* content-type="application/json"
* - body is encoded like
{"foo":"bar baz","x":1}
*
*
* content-type="application/x-www-form-urlencoded"
* - body is encoded like
foo=bar+baz&x=1
*
*
*
*
* JavaScript Info:
* @property contentType
* @type String
*
*/
@JsProperty String getContentType();
/**
* Content type to use when sending data. If the contentType
property
is set and a Content-Type
header is specified in the headers
property, the headers
property value will take precedence.
If Content-Type is set to a value listed below, then
the body
(typically used with POST requests) will be encoded accordingly.
*
* content-type="application/json"
* - body is encoded like
{"foo":"bar baz","x":1}
*
*
* content-type="application/x-www-form-urlencoded"
* - body is encoded like
foo=bar+baz&x=1
*
*
*
*
* JavaScript Info:
* @property contentType
* @type String
*
*/
@JsProperty void setContentType(String value);
/**
* Submits the form.
*
* JavaScript Info:
* @method submit
*
*
*/
void submit();
/**
* Validates all the required elements (custom and native) in the form.
*
* JavaScript Info:
* @method validate
*
* @return {boolean}
*/
boolean validate();
/**
* Returns a json object containing name/value pairs for all the registered
custom components and native elements of the form. If there are elements
with duplicate names, then their values will get aggregated into an
array of values.
*
* JavaScript Info:
* @method serialize
*
* @return {JavaScriptObject}
*/
JavaScriptObject serialize();
}