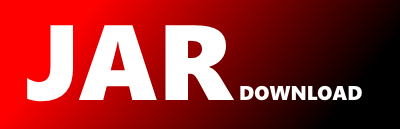
com.vaadin.polymer.iron.IronOverlayBehavior Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-overlay-behavior project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Use Polymer.IronOverlayBehavior
to implement an element that can be hidden or shown, and displays
on top of other content. It includes an optional backdrop, and can be used to implement a variety
of UI controls including dialogs and drop downs. Multiple overlays may be displayed at once.
* See the demo source code
for an example.
* Closing and canceling
* An overlay may be hidden by closing or canceling. The difference between close and cancel is user
intent. Closing generally implies that the user acknowledged the content on the overlay. By default,
it will cancel whenever the user taps outside it or presses the escape key. This behavior is
configurable with the no-cancel-on-esc-key
and the no-cancel-on-outside-click
properties.
close()
should be called explicitly by the implementer when the user interacts with a control
in the overlay element. When the dialog is canceled, the overlay fires an ‘iron-overlay-canceled’
event. Call preventDefault
on this event to prevent the overlay from closing.
* Positioning
* By default the element is sized and positioned to fit and centered inside the window. You can
position and size it manually using CSS. See Polymer.IronFitBehavior
.
* Backdrop
* Set the with-backdrop
attribute to display a backdrop behind the overlay. The backdrop is
appended to <body>
and is of type <iron-overlay-backdrop>
. See its doc page for styling
options.
* In addition, with-backdrop
will wrap the focus within the content in the light DOM.
Override the _focusableNodes
getter
to achieve a different behavior.
* Limitations
* The element is styled to appear on top of other content by setting its z-index
property. You
must ensure no element has a stacking context with a higher z-index
than its parent stacking
context. You should place this element as a child of <body>
whenever possible.
*/
@JsType(isNative=true)
public interface IronOverlayBehavior {
@JsOverlay public static final String NAME = "Polymer.IronOverlayBehavior";
@JsOverlay public static final String SRC = "iron-overlay-behavior/iron-overlay-behavior.html";
/**
* Set to true to disable canceling the overlay by clicking outside it.
*
* JavaScript Info:
* @property noCancelOnOutsideClick
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getNoCancelOnOutsideClick();
/**
* Set to true to disable canceling the overlay by clicking outside it.
*
* JavaScript Info:
* @property noCancelOnOutsideClick
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setNoCancelOnOutsideClick(boolean value);
/**
* Contains the reason(s) this overlay was last closed (see iron-overlay-closed
).
IronOverlayBehavior
provides the canceled
reason; implementers of the
behavior can provide other reasons in addition to canceled
.
*
* JavaScript Info:
* @property closingReason
* @type Object
* @behavior VaadinContextMenuOverlay
*/
@JsProperty JavaScriptObject getClosingReason();
/**
* Contains the reason(s) this overlay was last closed (see iron-overlay-closed
).
IronOverlayBehavior
provides the canceled
reason; implementers of the
behavior can provide other reasons in addition to canceled
.
*
* JavaScript Info:
* @property closingReason
* @type Object
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setClosingReason(JavaScriptObject value);
/**
* The backdrop element.
*
* JavaScript Info:
* @property backdropElement
* @type Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty Element getBackdropElement();
/**
* The backdrop element.
*
* JavaScript Info:
* @property backdropElement
* @type Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setBackdropElement(Element value);
/**
* Set to true to keep overlay always on top.
*
* JavaScript Info:
* @property alwaysOnTop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getAlwaysOnTop();
/**
* Set to true to keep overlay always on top.
*
* JavaScript Info:
* @property alwaysOnTop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setAlwaysOnTop(boolean value);
/**
* True if the overlay is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getOpened();
/**
* True if the overlay is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setOpened(boolean value);
/**
* Set to true to enable restoring of focus when overlay is closed.
*
* JavaScript Info:
* @property restoreFocusOnClose
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getRestoreFocusOnClose();
/**
* Set to true to enable restoring of focus when overlay is closed.
*
* JavaScript Info:
* @property restoreFocusOnClose
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setRestoreFocusOnClose(boolean value);
/**
* Set to true to disable auto-focusing the overlay or child nodes with
the autofocus
attribute` when the overlay is opened.
*
* JavaScript Info:
* @property noAutoFocus
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getNoAutoFocus();
/**
* Set to true to disable auto-focusing the overlay or child nodes with
the autofocus
attribute` when the overlay is opened.
*
* JavaScript Info:
* @property noAutoFocus
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setNoAutoFocus(boolean value);
/**
* Set to true to display a backdrop behind the overlay. It traps the focus
within the light DOM of the overlay.
*
* JavaScript Info:
* @property withBackdrop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getWithBackdrop();
/**
* Set to true to display a backdrop behind the overlay. It traps the focus
within the light DOM of the overlay.
*
* JavaScript Info:
* @property withBackdrop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setWithBackdrop(boolean value);
/**
* Set to true to disable canceling the overlay with the ESC key.
*
* JavaScript Info:
* @property noCancelOnEscKey
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getNoCancelOnEscKey();
/**
* Set to true to disable canceling the overlay with the ESC key.
*
* JavaScript Info:
* @property noCancelOnEscKey
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setNoCancelOnEscKey(boolean value);
/**
* True if the overlay was canceled when it was last closed.
*
* JavaScript Info:
* @property canceled
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getCanceled();
/**
* True if the overlay was canceled when it was last closed.
*
* JavaScript Info:
* @property canceled
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setCanceled(boolean value);
/**
* Close the overlay.
*
* JavaScript Info:
* @method close
* @behavior VaadinContextMenuOverlay
*
*/
void close();
/**
* Invalidates the cached tabbable nodes. To be called when any of the focusable
content changes (e.g. a button is disabled).
*
* JavaScript Info:
* @method invalidateTabbables
* @behavior VaadinContextMenuOverlay
*
*/
void invalidateTabbables();
/**
* Open the overlay.
*
* JavaScript Info:
* @method open
* @behavior VaadinContextMenuOverlay
*
*/
void open();
/**
* Toggle the opened state of the overlay.
*
* JavaScript Info:
* @method toggle
* @behavior VaadinContextMenuOverlay
*
*/
void toggle();
/**
* Cancels the overlay.
*
* JavaScript Info:
* @method cancel
* @param {Event=} event
* @behavior VaadinContextMenuOverlay
*
*/
void cancel(JavaScriptObject event);
}