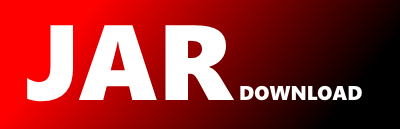
com.vaadin.polymer.iron.IronResizableBehavior Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-resizable-behavior project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* IronResizableBehavior
is a behavior that can be used in Polymer elements to
coordinate the flow of resize events between “resizers” (elements that control the
size or hidden state of their children) and “resizables” (elements that need to be
notified when they are resized or un-hidden by their parents in order to take
action on their new measurements).
* Elements that perform measurement should add the IronResizableBehavior
behavior to
their element definition and listen for the iron-resize
event on themselves.
This event will be fired when they become showing after having been hidden,
when they are resized explicitly by another resizable, or when the window has been
resized.
* Note, the iron-resize
event is non-bubbling.
*/
@JsType(isNative=true)
public interface IronResizableBehavior {
@JsOverlay public static final String NAME = "Polymer.IronResizableBehavior";
@JsOverlay public static final String SRC = "iron-resizable-behavior/iron-resizable-behavior.html";
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
void assignParentResizable(Object parentResizable);
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
void stopResizeNotificationsFor(Object target);
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
boolean resizerShouldNotify(JavaScriptObject element);
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
void notifyResize();
}