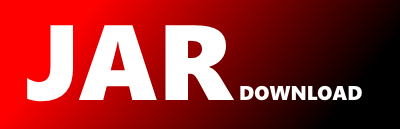
com.vaadin.polymer.iron.IronScrollThresholdElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-scroll-threshold project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* iron-scroll-threshold
is a utility element that listens for scroll
events from a
scrollable region and fires events to indicate when the scroller has reached a pre-defined
limit, specified in pixels from the upper and lower bounds of the scrollable region.
This element may wrap a scrollable region and will listen for scroll
events bubbling
through it from its children. In this case, care should be taken that only one scrollable
region with the same orientation as this element is contained within. Alternatively,
the scrollTarget
property can be set/bound to a non-child scrollable region, from which
it will listen for events.
* Once a threshold has been reached, a lower-threshold
or upper-threshold
event will
be fired, at which point the user may perform actions such as lazily-loading more data
to be displayed. After any work is done, the user must then clear the threshold by
calling the clearTriggers
method on this element, after which it will
begin listening again for the scroll position to reach the threshold again assuming
the content in the scrollable region has grown. If the user no longer wishes to receive
events (e.g. all data has been exhausted), the threshold property in question (e.g.
lowerThreshold
) may be set to a falsy value to disable events and clear the associated
triggered property.
* Example
* <iron-scroll-threshold on-lower-threshold="loadMoreData">
* <div>content</div>
* </iron-scroll-threshold>
*
* loadMoreData: function() {
* // load async stuff. e.g. XHR
* asyncStuff(function done() {
* ironScrollTheshold.clearTriggers();
* });
* }
*
* Using dom-repeat
* <iron-scroll-threshold on-lower-threshold="loadMoreData">
* <template is="dom-repeat" items="[[items]]">
* <div>[[index]]</div>
* </template>
* </iron-scroll-threshold>
*
* Using iron-list
* <iron-scroll-threshold on-lower-threshold="loadMoreData" id="threshold">
* <iron-list scroll-target="threshold" items="[[items]]">
* <template>
* <div>[[index]]</div>
* </template>
* </iron-list>
* </iron-scroll-threshold>
*
*/
@JsType(isNative=true)
public interface IronScrollThresholdElement extends HTMLElement {
@JsOverlay public static final String TAG = "iron-scroll-threshold";
@JsOverlay public static final String SRC = "iron-scroll-threshold/iron-scroll-threshold.html";
/**
* True if the orientation of the scroller is horizontal.
*
* JavaScript Info:
* @property horizontal
* @type Boolean
*
*/
@JsProperty boolean getHorizontal();
/**
* True if the orientation of the scroller is horizontal.
*
* JavaScript Info:
* @property horizontal
* @type Boolean
*
*/
@JsProperty void setHorizontal(boolean value);
/**
* Distance from the bottom (or right, for horizontal) bound of the scroller
where the “lower trigger” will fire.
*
* JavaScript Info:
* @property lowerThreshold
* @type Number
*
*/
@JsProperty double getLowerThreshold();
/**
* Distance from the bottom (or right, for horizontal) bound of the scroller
where the “lower trigger” will fire.
*
* JavaScript Info:
* @property lowerThreshold
* @type Number
*
*/
@JsProperty void setLowerThreshold(double value);
/**
* Distance from the top (or left, for horizontal) bound of the scroller
where the “upper trigger” will fire.
*
* JavaScript Info:
* @property upperThreshold
* @type Number
*
*/
@JsProperty double getUpperThreshold();
/**
* Distance from the top (or left, for horizontal) bound of the scroller
where the “upper trigger” will fire.
*
* JavaScript Info:
* @property upperThreshold
* @type Number
*
*/
@JsProperty void setUpperThreshold(double value);
/**
* Read-only value that tracks the triggered state of the upper threshold.
*
* JavaScript Info:
* @property upperTriggered
* @type Boolean
*
*/
@JsProperty boolean getUpperTriggered();
/**
* Read-only value that tracks the triggered state of the upper threshold.
*
* JavaScript Info:
* @property upperTriggered
* @type Boolean
*
*/
@JsProperty void setUpperTriggered(boolean value);
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
@JsProperty Element getScrollTarget();
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
@JsProperty void setScrollTarget(Element value);
/**
* Read-only value that tracks the triggered state of the lower threshold.
*
* JavaScript Info:
* @property lowerTriggered
* @type Boolean
*
*/
@JsProperty boolean getLowerTriggered();
/**
* Read-only value that tracks the triggered state of the lower threshold.
*
* JavaScript Info:
* @property lowerTriggered
* @type Boolean
*
*/
@JsProperty void setLowerTriggered(boolean value);
/**
*
*
* JavaScript Info:
* @method checkScrollThesholds
*
*
*/
void checkScrollThesholds();
/**
* Checks the scroll thresholds.
This method is automatically called by iron-scroll-threshold.
*
* JavaScript Info:
* @method checkScrollThresholds
*
*
*/
void checkScrollThresholds();
/**
* Clear the upper and lower threshold states.
*
* JavaScript Info:
* @method clearTriggers
*
*
*/
void clearTriggers();
/**
* Enables or disables the scroll event listener.
*
* JavaScript Info:
* @method toggleScrollListener
* @param {boolean} yes
* @behavior IronScrollThreshold
*
*/
void toggleScrollListener(boolean yes);
/**
* Scrolls the content to a particular place.
*
* JavaScript Info:
* @method scroll
* @param {number} left
* @param {number} top
* @behavior IronScrollThreshold
*
*/
void scroll(double left, double top);
}