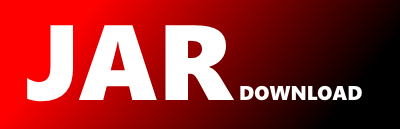
com.vaadin.polymer.iron.widget.IronA11yKeys Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-a11y-keys project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.*;
import com.vaadin.polymer.iron.widget.event.KeysPressedEvent;
import com.vaadin.polymer.iron.widget.event.KeysPressedEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* iron-a11y-keys
provides a cross-browser interface for processing
keyboard commands. The interface adheres to WAI-ARIA best
practices.
It uses an expressive syntax to filter key presses.
* Basic usage
* The sample code below is a portion of a custom element. The goal is to call
the onEnter
method whenever the paper-input
element is in focus and
the Enter
key is pressed.
* <iron-a11y-keys id="a11y" target="[[target]]" keys="enter"
* on-keys-pressed="onEnter"></iron-a11y-keys>
* <paper-input id="input"
* placeholder="Type something. Press enter. Check console."
* value="{{userInput::input}}"></paper-input>
*
*
*
The custom element declares an iron-a11y-keys
element that is bound to a
property called target
. The target
property
needs to evaluate to the paper-input
node. iron-a11y-keys
registers
an event handler for the target node using Polymer’s annotated event handler
syntax. {{userInput::input}}
sets the userInput
property to the
user’s input on each keystroke.
* The last step is to link the two elements within the custom element’s
registration.
* ...
* properties: {
* userInput: {
* type: String,
* notify: true,
* },
* target: {
* type: Object,
* value: function() {
* return this.$.input;
* }
* },
* },
* onEnter: function() {
* console.log(this.userInput);
* }
* ...
*
*
*
The keys
attribute
* The keys
attribute expresses what combination of keys triggers the event.
* The attribute accepts a space-separated, plus-sign-concatenated
set of modifier keys and some common keyboard keys.
* The common keys are: a-z
, 0-9
(top row and number pad), *
(shift 8 and
number pad), F1-F12
, Page Up
, Page Down
, Left Arrow
, Right Arrow
,
Down Arrow
, Up Arrow
, Home
, End
, Escape
, Space
, Tab
, Enter
.
* The modifier keys are: Shift
, Control
, Alt
, Meta
.
* All keys are expected to be lowercase and shortened. E.g.
Left Arrow
is left
, Page Down
is pagedown
, Control
is ctrl
,
F1
is f1
, Escape
is esc
, etc.
* Grammar
* Below is the EBNF
Grammar of the keys
attribute.
* modifier = "shift" | "ctrl" | "alt" | "meta";
* ascii = ? /[a-z0-9]/ ? ;
* fnkey = ? f1 through f12 ? ;
* arrow = "up" | "down" | "left" | "right" ;
* key = "tab" | "esc" | "space" | "*" | "pageup" | "pagedown" |
* "home" | "end" | arrow | ascii | fnkey;
* event = "keypress" | "keydown" | "keyup";
* keycombo = { modifier, "+" }, key, [ ":", event ] ;
* keys = keycombo, { " ", keycombo } ;
*
*
*
Example
* Given the following value for keys
:
* ctrl+shift+f7 up pagedown esc space alt+m
* The event is fired if any of the following key combinations are fired:
Control
and Shift
and F7
keys, Up Arrow
key, Page Down
key,
Escape
key, Space
key, Alt
and M
keys.
* WAI-ARIA Slider Example
* The following is an example of the set of keys that fulfills WAI-ARIA’s
“slider” role best
practices:
* <iron-a11y-keys target="[[target]]" keys="left pagedown down"
* on-keys-pressed="decrement"></iron-a11y-keys>
* <iron-a11y-keys target="[[target]]" keys="right pageup up"
* on-keys-pressed="increment"></iron-a11y-keys>
* <iron-a11y-keys target="[[target]]" keys="home"
* on-keys-pressed="setMin"></iron-a11y-keys>
* <iron-a11y-keys target="[[target]]" keys="end"
* on-keys-pressed="setMax"></iron-a11y-keys>
*
*
*
The target
properties must evaluate to a node. See the basic usage
example above.
* Each of the values for the on-keys-pressed
attributes must evalute
to methods. The increment
method should move the slider a set amount
toward the maximum value. decrement
should move the slider a set amount
toward the minimum value. setMin
should move the slider to the minimum
value. setMax
should move the slider to the maximum value.
*/
public class IronA11yKeys extends PolymerWidget {
/**
* Default Constructor.
*/
public IronA11yKeys() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronA11yKeys(String html) {
super(IronA11yKeysElement.TAG, IronA11yKeysElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronA11yKeysElement getPolymerElement() {
return (IronA11yKeysElement) getElement();
}
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @property keyBindings
* @type !Object
* @behavior VaadinDatePicker
*/
public JavaScriptObject getKeyBindings() {
return getPolymerElement().getKeyBindings();
}
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @property keyBindings
* @type !Object
* @behavior VaadinDatePicker
*/
public void setKeyBindings(JavaScriptObject value) {
getPolymerElement().setKeyBindings(value);
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public JavaScriptObject getKeyEventTarget() {
return getPolymerElement().getKeyEventTarget();
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(JavaScriptObject value) {
getPolymerElement().setKeyEventTarget(value);
}
/**
*
*
* JavaScript Info:
* @property target
* @type ?Node
*
*/
public JavaScriptObject getTarget() {
return getPolymerElement().getTarget();
}
/**
*
*
* JavaScript Info:
* @property target
* @type ?Node
*
*/
public void setTarget(JavaScriptObject value) {
getPolymerElement().setTarget(value);
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public boolean getStopKeyboardEventPropagation() {
return getPolymerElement().getStopKeyboardEventPropagation();
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public void setStopKeyboardEventPropagation(boolean value) {
getPolymerElement().setStopKeyboardEventPropagation(value);
}
/**
* Space delimited list of keys where each key follows the format:
[MODIFIER+]*KEY[:EVENT]
.
e.g. keys="space ctrl+shift+tab enter:keyup"
.
More detail can be found in the “Grammar” section of the documentation
*
* JavaScript Info:
* @property keys
* @type String
*
*/
public String getKeys() {
return getPolymerElement().getKeys();
}
/**
* Space delimited list of keys where each key follows the format:
[MODIFIER+]*KEY[:EVENT]
.
e.g. keys="space ctrl+shift+tab enter:keyup"
.
More detail can be found in the “Grammar” section of the documentation
*
* JavaScript Info:
* @property keys
* @type String
*
*/
public void setKeys(String value) {
getPolymerElement().setKeys(value);
}
// Needed in UIBinder
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @attribute key-event-target
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(String value) {
Polymer.property(this.getPolymerElement(), "keyEventTarget", value);
}
// Needed in UIBinder
/**
*
*
* JavaScript Info:
* @attribute target
*
*/
public void setTarget(String value) {
Polymer.property(this.getPolymerElement(), "target", value);
}
// Needed in UIBinder
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @attribute key-bindings
* @behavior VaadinDatePicker
*/
public void setKeyBindings(String value) {
Polymer.property(this.getPolymerElement(), "keyBindings", value);
}
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
public void addOwnKeyBinding(Object eventString, Object handlerName) {
getPolymerElement().addOwnKeyBinding(eventString, handlerName);
}
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
public boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString) {
return getPolymerElement().keyboardEventMatchesKeys(event, eventString);
}
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
public void removeOwnKeyBindings() {
getPolymerElement().removeOwnKeyBindings();
}
/**
*
*
* JavaScript Info:
* @event keys-pressed
*/
public HandlerRegistration addKeysPressedHandler(KeysPressedEventHandler handler) {
return addDomHandler(handler, KeysPressedEvent.TYPE);
}
}