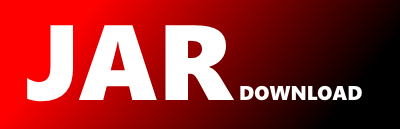
com.vaadin.polymer.iron.widget.IronAutogrowTextarea Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-autogrow-textarea project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.*;
import com.vaadin.polymer.iron.widget.event.IronFormElementRegisterEvent;
import com.vaadin.polymer.iron.widget.event.IronFormElementRegisterEventHandler;
import com.vaadin.polymer.iron.widget.event.IronFormElementUnregisterEvent;
import com.vaadin.polymer.iron.widget.event.IronFormElementUnregisterEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* iron-autogrow-textarea
is an element containing a textarea that grows in height as more
lines of input are entered. Unless an explicit height or the maxRows
property is set, it will
never scroll.
* Example:
* <iron-autogrow-textarea></iron-autogrow-textarea>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --iron-autogrow-textarea
* Mixin applied to the textarea
* {}
*
*
* --iron-autogrow-textarea-placeholder
* Mixin applied to the textarea placeholder
* {}
*
*
*
*/
public class IronAutogrowTextarea extends PolymerWidget {
/**
* Default Constructor.
*/
public IronAutogrowTextarea() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronAutogrowTextarea(String html) {
super(IronAutogrowTextareaElement.TAG, IronAutogrowTextareaElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronAutogrowTextareaElement getPolymerElement() {
return (IronAutogrowTextareaElement) getElement();
}
/**
* Set to true to mark the textarea as required.
*
* JavaScript Info:
* @property required
* @type Boolean
*
*/
public boolean getRequired() {
return getPolymerElement().getRequired();
}
/**
* Set to true to mark the textarea as required.
*
* JavaScript Info:
* @property required
* @type Boolean
*
*/
public void setRequired(boolean value) {
getPolymerElement().setRequired(value);
}
/**
* The maximum number of rows this element can grow to until it
scrolls. 0 means no maximum.
*
* JavaScript Info:
* @property maxRows
* @type Number
*
*/
public double getMaxRows() {
return getPolymerElement().getMaxRows();
}
/**
* The maximum number of rows this element can grow to until it
scrolls. 0 means no maximum.
*
* JavaScript Info:
* @property maxRows
* @type Number
*
*/
public void setMaxRows(double value) {
getPolymerElement().setMaxRows(value);
}
/**
* The maximum length of the input value.
*
* JavaScript Info:
* @property maxlength
* @type Number
*
*/
public double getMaxlength() {
return getPolymerElement().getMaxlength();
}
/**
* The maximum length of the input value.
*
* JavaScript Info:
* @property maxlength
* @type Number
*
*/
public void setMaxlength(double value) {
getPolymerElement().setMaxlength(value);
}
/**
* The minimum length of the input value.
*
* JavaScript Info:
* @property minlength
* @type Number
*
*/
public double getMinlength() {
return getPolymerElement().getMinlength();
}
/**
* The minimum length of the input value.
*
* JavaScript Info:
* @property minlength
* @type Number
*
*/
public void setMinlength(double value) {
getPolymerElement().setMinlength(value);
}
/**
* Use this property instead of value
for two-way data binding.
This property will be deprecated in the future. Use value
instead.
*
* JavaScript Info:
* @property bindValue
* @type (string|number)
*
*/
public Object getBindValue() {
return getPolymerElement().getBindValue();
}
/**
* Use this property instead of value
for two-way data binding.
This property will be deprecated in the future. Use value
instead.
*
* JavaScript Info:
* @property bindValue
* @type (string|number)
*
*/
public void setBindValue(Object value) {
getPolymerElement().setBindValue(value);
}
/**
* The initial number of rows.
*
* JavaScript Info:
* @property rows
* @type Number
*
*/
public double getRows() {
return getPolymerElement().getRows();
}
/**
* The initial number of rows.
*
* JavaScript Info:
* @property rows
* @type Number
*
*/
public void setRows(double value) {
getPolymerElement().setRows(value);
}
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
public boolean getDisabled() {
return getPolymerElement().getDisabled();
}
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
public void setDisabled(boolean value) {
getPolymerElement().setDisabled(value);
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
public boolean getFocused() {
return getPolymerElement().getFocused();
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
public void setFocused(boolean value) {
getPolymerElement().setFocused(value);
}
/**
* True if the last call to validate
is invalid.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior VaadinDatePicker
*/
public boolean getInvalid() {
return getPolymerElement().getInvalid();
}
/**
* True if the last call to validate
is invalid.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior VaadinDatePicker
*/
public void setInvalid(boolean value) {
getPolymerElement().setInvalid(value);
}
/**
* Bound to the textarea’s autofocus
attribute.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
*
*/
public boolean getAutofocus() {
return getPolymerElement().getAutofocus();
}
/**
* Bound to the textarea’s autofocus
attribute.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
*
*/
public void setAutofocus(boolean value) {
getPolymerElement().setAutofocus(value);
}
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
public String getName() {
return getPolymerElement().getName();
}
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
public void setName(String value) {
getPolymerElement().setName(value);
}
/**
* Bound to the textarea’s autocomplete
attribute.
*
* JavaScript Info:
* @property autocomplete
* @type String
*
*/
public String getAutocomplete() {
return getPolymerElement().getAutocomplete();
}
/**
* Bound to the textarea’s autocomplete
attribute.
*
* JavaScript Info:
* @property autocomplete
* @type String
*
*/
public void setAutocomplete(String value) {
getPolymerElement().setAutocomplete(value);
}
/**
* The value for this element.
*
* JavaScript Info:
* @property value
* @type String
* @behavior VaadinDatePicker
*/
public String getValue() {
return getPolymerElement().getValue();
}
/**
* The value for this element.
*
* JavaScript Info:
* @property value
* @type String
* @behavior VaadinDatePicker
*/
public void setValue(String value) {
getPolymerElement().setValue(value);
}
/**
* Bound to the textarea’s inputmode
attribute.
*
* JavaScript Info:
* @property inputmode
* @type String
*
*/
public String getInputmode() {
return getPolymerElement().getInputmode();
}
/**
* Bound to the textarea’s inputmode
attribute.
*
* JavaScript Info:
* @property inputmode
* @type String
*
*/
public void setInputmode(String value) {
getPolymerElement().setInputmode(value);
}
/**
* Bound to the textarea’s placeholder
attribute.
*
* JavaScript Info:
* @property placeholder
* @type String
*
*/
public String getPlaceholder() {
return getPolymerElement().getPlaceholder();
}
/**
* Bound to the textarea’s placeholder
attribute.
*
* JavaScript Info:
* @property placeholder
* @type String
*
*/
public void setPlaceholder(String value) {
getPolymerElement().setPlaceholder(value);
}
/**
* Namespace for this validator. This property is deprecated and should
not be used. For all intents and purposes, please consider it a
read-only, config-time property.
*
* JavaScript Info:
* @property validatorType
* @type String
* @behavior VaadinDatePicker
*/
public String getValidatorType() {
return getPolymerElement().getValidatorType();
}
/**
* Namespace for this validator. This property is deprecated and should
not be used. For all intents and purposes, please consider it a
read-only, config-time property.
*
* JavaScript Info:
* @property validatorType
* @type String
* @behavior VaadinDatePicker
*/
public void setValidatorType(String value) {
getPolymerElement().setValidatorType(value);
}
/**
* Name of the validator to use.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior VaadinDatePicker
*/
public String getValidator() {
return getPolymerElement().getValidator();
}
/**
* Name of the validator to use.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior VaadinDatePicker
*/
public void setValidator(String value) {
getPolymerElement().setValidator(value);
}
/**
* Bound to the textarea’s readonly
attribute.
*
* JavaScript Info:
* @property readonly
* @type String
*
*/
public String getReadonly() {
return getPolymerElement().getReadonly();
}
/**
* Bound to the textarea’s readonly
attribute.
*
* JavaScript Info:
* @property readonly
* @type String
*
*/
public void setReadonly(String value) {
getPolymerElement().setReadonly(value);
}
// Needed in UIBinder
/**
* The minimum length of the input value.
*
* JavaScript Info:
* @attribute minlength
*
*/
public void setMinlength(String value) {
Polymer.property(this.getPolymerElement(), "minlength", value);
}
// Needed in UIBinder
/**
* Use this property instead of value
for two-way data binding.
This property will be deprecated in the future. Use value
instead.
*
* JavaScript Info:
* @attribute bind-value
*
*/
public void setBindValue(String value) {
Polymer.property(this.getPolymerElement(), "bindValue", value);
}
// Needed in UIBinder
/**
* The initial number of rows.
*
* JavaScript Info:
* @attribute rows
*
*/
public void setRows(String value) {
Polymer.property(this.getPolymerElement(), "rows", value);
}
// Needed in UIBinder
/**
* The maximum length of the input value.
*
* JavaScript Info:
* @attribute maxlength
*
*/
public void setMaxlength(String value) {
Polymer.property(this.getPolymerElement(), "maxlength", value);
}
// Needed in UIBinder
/**
* The maximum number of rows this element can grow to until it
scrolls. 0 means no maximum.
*
* JavaScript Info:
* @attribute max-rows
*
*/
public void setMaxRows(String value) {
Polymer.property(this.getPolymerElement(), "maxRows", value);
}
/**
*
*
* JavaScript Info:
* @method hasValidator
* @behavior VaadinDatePicker
* @return {boolean}
*/
public boolean hasValidator() {
return getPolymerElement().hasValidator();
}
/**
* Returns true if value
is valid. The validator provided in validator
will be used first, if it exists; otherwise, the textarea
‘s validity
is used.
*
* JavaScript Info:
* @method validate
*
* @return {boolean}
*/
public boolean validate() {
return getPolymerElement().validate();
}
/**
* Returns true if the value
is valid, and updates invalid
. If you want
your element to have custom validation logic, do not override this method;
override _getValidity(value)
instead.
*
* JavaScript Info:
* @method validate
* @param {Object} value
* @behavior VaadinDatePicker
* @return {boolean}
*/
public boolean validate(JavaScriptObject value) {
return getPolymerElement().validate(value);
}
/**
* Fired when the element is added to an iron-form
.
*
* JavaScript Info:
* @event iron-form-element-register
*/
public HandlerRegistration addIronFormElementRegisterHandler(IronFormElementRegisterEventHandler handler) {
return addDomHandler(handler, IronFormElementRegisterEvent.TYPE);
}
/**
* Fired when the element is removed from an iron-form
.
*
* JavaScript Info:
* @event iron-form-element-unregister
*/
public HandlerRegistration addIronFormElementUnregisterHandler(IronFormElementUnregisterEventHandler handler) {
return addDomHandler(handler, IronFormElementUnregisterEvent.TYPE);
}
}