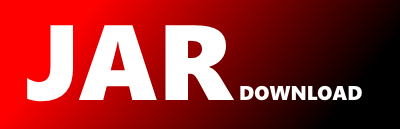
com.vaadin.polymer.iron.widget.IronComponentPage Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-component-page project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Loads Polymer element and behavior documentation using
Hydrolysis and renders a complete
documentation page including demos (if available).
* To display a warning inside an iron-component-page
element,
add the .warning
class to a child.
*/
public class IronComponentPage extends PolymerWidget {
/**
* Default Constructor.
*/
public IronComponentPage() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronComponentPage(String html) {
super(IronComponentPageElement.TAG, IronComponentPageElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronComponentPageElement getPolymerElement() {
return (IronComponentPageElement) getElement();
}
/**
* Toggle flag to be used when this element is being displayed in the
Polymer Elements catalog.
*
* JavaScript Info:
* @property catalog
* @type Boolean
*
*/
public boolean getCatalog() {
return getPolymerElement().getCatalog();
}
/**
* Toggle flag to be used when this element is being displayed in the
Polymer Elements catalog.
*
* JavaScript Info:
* @property catalog
* @type Boolean
*
*/
public void setCatalog(boolean value) {
getPolymerElement().setCatalog(value);
}
/**
* The Hydrolysis behavior descriptors that have been loaded.
*
* JavaScript Info:
* @property docBehaviors
* @type Array
*
*/
public JsArray getDocBehaviors() {
return getPolymerElement().getDocBehaviors();
}
/**
* The Hydrolysis behavior descriptors that have been loaded.
*
* JavaScript Info:
* @property docBehaviors
* @type Array
*
*/
public void setDocBehaviors(JsArray value) {
getPolymerElement().setDocBehaviors(value);
}
/**
* Demos for the currently selected element.
*
* JavaScript Info:
* @property docDemos
* @type Array
*
*/
public JsArray getDocDemos() {
return getPolymerElement().getDocDemos();
}
/**
* Demos for the currently selected element.
*
* JavaScript Info:
* @property docDemos
* @type Array
*
*/
public void setDocDemos(JsArray value) {
getPolymerElement().setDocDemos(value);
}
/**
* The Hydrolysis element descriptors that have been loaded.
*
* JavaScript Info:
* @property docElements
* @type Array
*
*/
public JsArray getDocElements() {
return getPolymerElement().getDocElements();
}
/**
* The Hydrolysis element descriptors that have been loaded.
*
* JavaScript Info:
* @property docElements
* @type Array
*
*/
public void setDocElements(JsArray value) {
getPolymerElement().setDocElements(value);
}
/**
* Whether all dependencies should be loaded and documented.
* Turning this on will probably slow down the load process dramatically.
*
* JavaScript Info:
* @property transitive
* @type Boolean
*
*/
public boolean getTransitive() {
return getPolymerElement().getTransitive();
}
/**
* Whether all dependencies should be loaded and documented.
* Turning this on will probably slow down the load process dramatically.
*
* JavaScript Info:
* @property transitive
* @type Boolean
*
*/
public void setTransitive(boolean value) {
getPolymerElement().setTransitive(value);
}
/**
* The relative root for determining paths to demos and default source
detection.
*
* JavaScript Info:
* @property base
* @type String
*
*/
public String getBase() {
return getPolymerElement().getBase();
}
/**
* The relative root for determining paths to demos and default source
detection.
*
* JavaScript Info:
* @property base
* @type String
*
*/
public void setBase(String value) {
getPolymerElement().setBase(value);
}
/**
* The scroll mode for the page. For details about the modes,
see the mode property in paper-header-panel.
*
* JavaScript Info:
* @property scrollMode
* @type String
*
*/
public String getScrollMode() {
return getPolymerElement().getScrollMode();
}
/**
* The scroll mode for the page. For details about the modes,
see the mode property in paper-header-panel.
*
* JavaScript Info:
* @property scrollMode
* @type String
*
*/
public void setScrollMode(String value) {
getPolymerElement().setScrollMode(value);
}
/**
* The URL to an import that declares (or transitively imports) the
elements that you wish to see documented.
* If the URL is relative, it will be resolved relative to the master
document.
* If a src
URL is not specified, it will resolve the name of the
directory containing this element, followed by dirname.html
. For
example:
* awesome-sauce/index.html
:
* <iron-doc-viewer></iron-doc-viewer>
*
*
*
Would implicitly have src="awesome-sauce.html"
.
*
* JavaScript Info:
* @property src
* @type String
*
*/
public String getSrc() {
return getPolymerElement().getSrc();
}
/**
* The URL to an import that declares (or transitively imports) the
elements that you wish to see documented.
* If the URL is relative, it will be resolved relative to the master
document.
* If a src
URL is not specified, it will resolve the name of the
directory containing this element, followed by dirname.html
. For
example:
* awesome-sauce/index.html
:
* <iron-doc-viewer></iron-doc-viewer>
*
*
*
Would implicitly have src="awesome-sauce.html"
.
*
* JavaScript Info:
* @property src
* @type String
*
*/
public void setSrc(String value) {
getPolymerElement().setSrc(value);
}
/**
* The URL to a precompiled JSON descriptor. If you have precompiled
and stored a documentation set using Hydrolysis, you can load the
analyzer directly via AJAX by specifying this attribute.
* If a doc-src
is not specified, it is ignored and the default
rules according to the src
attribute are used.
*
* JavaScript Info:
* @property docSrc
* @type String
*
*/
public String getDocSrc() {
return getPolymerElement().getDocSrc();
}
/**
* The URL to a precompiled JSON descriptor. If you have precompiled
and stored a documentation set using Hydrolysis, you can load the
analyzer directly via AJAX by specifying this attribute.
* If a doc-src
is not specified, it is ignored and the default
rules according to the src
attribute are used.
*
* JavaScript Info:
* @property docSrc
* @type String
*
*/
public void setDocSrc(String value) {
getPolymerElement().setDocSrc(value);
}
/**
* An optional version string.
*
* JavaScript Info:
* @property version
* @type string
*
*/
public String getVersion() {
return getPolymerElement().getVersion();
}
/**
* An optional version string.
*
* JavaScript Info:
* @property version
* @type string
*
*/
public void setVersion(String value) {
getPolymerElement().setVersion(value);
}
/**
* The current view. Can be docs
or demo
.
*
* JavaScript Info:
* @property view
* @type String
*
*/
public String getView() {
return getPolymerElement().getView();
}
/**
* The current view. Can be docs
or demo
.
*
* JavaScript Info:
* @property view
* @type String
*
*/
public void setView(String value) {
getPolymerElement().setView(value);
}
/**
* The name of a branch used to fetch source for gh-pages.
If not specified master
is used.
*
* JavaScript Info:
* @property branchName
* @type String
*
*/
public String getBranchName() {
return getPolymerElement().getBranchName();
}
/**
* The name of a branch used to fetch source for gh-pages.
If not specified master
is used.
*
* JavaScript Info:
* @property branchName
* @type String
*
*/
public void setBranchName(String value) {
getPolymerElement().setBranchName(value);
}
/**
* The element or behavior that will be displayed on the page. Defaults
to the element matching the name of the source file.
*
* JavaScript Info:
* @property active
* @type String
*
*/
public String getActive() {
return getPolymerElement().getActive();
}
/**
* The element or behavior that will be displayed on the page. Defaults
to the element matching the name of the source file.
*
* JavaScript Info:
* @property active
* @type String
*
*/
public void setActive(String value) {
getPolymerElement().setActive(value);
}
// Needed in UIBinder
/**
* The Hydrolysis behavior descriptors that have been loaded.
*
* JavaScript Info:
* @attribute doc-behaviors
*
*/
public void setDocBehaviors(String value) {
Polymer.property(this.getPolymerElement(), "docBehaviors", value);
}
// Needed in UIBinder
/**
* Demos for the currently selected element.
*
* JavaScript Info:
* @attribute doc-demos
*
*/
public void setDocDemos(String value) {
Polymer.property(this.getPolymerElement(), "docDemos", value);
}
// Needed in UIBinder
/**
* The Hydrolysis element descriptors that have been loaded.
*
* JavaScript Info:
* @attribute doc-elements
*
*/
public void setDocElements(String value) {
Polymer.property(this.getPolymerElement(), "docElements", value);
}
/**
* Renders this element into static HTML for offline use.
* This is mostly useful for debugging and one-off documentation generation.
If you want to integrate doc generation into your build process, you
probably want to be calling hydrolysis.Analyzer.analyze()
directly.
*
* JavaScript Info:
* @method marshal
*
* @return {String}
*/
public String marshal() {
return getPolymerElement().marshal();
}
}