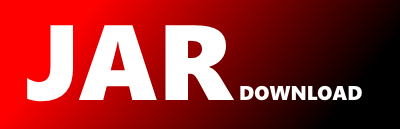
com.vaadin.polymer.iron.widget.IronDocProperty Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-doc-viewer project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Renders documentation describing a specific property of an element.
* Give it a hydrolysis PropertyDescriptor
(via descriptor
), and watch it go!
*/
public class IronDocProperty extends PolymerWidget {
/**
* Default Constructor.
*/
public IronDocProperty() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronDocProperty(String html) {
super(IronDocPropertyElement.TAG, IronDocPropertyElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronDocPropertyElement getPolymerElement() {
return (IronDocPropertyElement) getElement();
}
/**
* Whether the property should show a one-liner, or full summary.
* Note that this property is reflected as an attribute, but we perform
the reflection manually. In order to support the CSS transitions, we
must calculate the element height before setting the attribute.
*
* JavaScript Info:
* @property collapsed
* @type Boolean
*
*/
public boolean getCollapsed() {
return getPolymerElement().getCollapsed();
}
/**
* Whether the property should show a one-liner, or full summary.
* Note that this property is reflected as an attribute, but we perform
the reflection manually. In order to support the CSS transitions, we
must calculate the element height before setting the attribute.
*
* JavaScript Info:
* @property collapsed
* @type Boolean
*
*/
public void setCollapsed(boolean value) {
getPolymerElement().setCollapsed(value);
}
/**
* The Hydrolysis-generated
element descriptor to display details for.
* Alternatively, the element descriptor can be provided as JSON via the text content
of this element.
*
* JavaScript Info:
* @property descriptor
* @type hydrolysis.PropertyDescriptor
*
*/
public JavaScriptObject getDescriptor() {
return getPolymerElement().getDescriptor();
}
/**
* The Hydrolysis-generated
element descriptor to display details for.
* Alternatively, the element descriptor can be provided as JSON via the text content
of this element.
*
* JavaScript Info:
* @property descriptor
* @type hydrolysis.PropertyDescriptor
*
*/
public void setDescriptor(JavaScriptObject value) {
getPolymerElement().setDescriptor(value);
}
/**
* Unique anchor ID for deep-linking.
*
* JavaScript Info:
* @property anchorId
* @type String
*
*/
public String getAnchorId() {
return getPolymerElement().getAnchorId();
}
/**
* Unique anchor ID for deep-linking.
*
* JavaScript Info:
* @property anchorId
* @type String
*
*/
public void setAnchorId(String value) {
getPolymerElement().setAnchorId(value);
}
// Needed in UIBinder
/**
* The Hydrolysis-generated
element descriptor to display details for.
* Alternatively, the element descriptor can be provided as JSON via the text content
of this element.
*
* JavaScript Info:
* @attribute descriptor
*
*/
public void setDescriptor(String value) {
Polymer.property(this.getPolymerElement(), "descriptor", value);
}
}