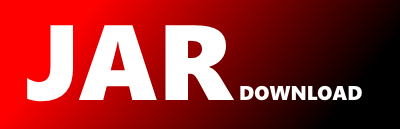
com.vaadin.polymer.iron.widget.IronIconset Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-iconset project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* The iron-iconset
element allows users to define their own icon set using
an image file. (To create an iconset using SVG icons, see iron-iconset-svg
.)
* The src
property specifies the url of the icon image. Multiple icons may
be included in this image and they may be organized into rows.
The icons
property is a space separated list of names corresponding to the
icons. The names must be ordered as the icons are ordered in the icon image.
Icons are expected to be square and are the size specified by the size
property. The width
property corresponds to the width of the icon image
and must be specified if icons are arranged into multiple rows in the image.
* All iron-iconset
elements are available for use by other iron-iconset
elements via a database keyed by id. Typically, an element author that wants
to support a set of custom icons uses a iron-iconset
to retrieve
and use another, user-defined icon set, or simply uses the iron-icon
element to display an icon by specifying its icon set name and id.
* Example:
* <iron-iconset id="my-icons" src="my-icons.png" width="96" size="24"
* icons="location place starta stopb bus car train walk">
* </iron-iconset>
*
*
*
This will automatically register the icon set “my-icons” to the iconset
database. To use these icons from within another element, make a
iron-iconset
element and call the byId
method to retrieve a
given iconset. To apply a particular icon to an element, use the
applyIcon
method. For example:
* iconset.applyIcon(iconNode, 'car');
*
*
*
Themed icon sets are also supported. The iron-iconset
can contain child
property
elements that specify a theme with an offsetX and offsetY of the
theme within the icon resource. For example.
* <iron-iconset id="my-icons" src="my-icons.png" width="96" size="24"
* icons="location place starta stopb bus car train walk">
* <property theme="special" offsetX="256" offsetY="24"></property>
* </iron-iconset>
*
*
*
Then a themed icon can be applied like this:
* iconset.applyIcon(iconNode, 'car', 'special');
*
*
*
See also:
*
* iron-iconset-svg
. Build icon sets with SVG elements.
* iron-icons
. Predefined icon sets.
* iron-icon
. Simple element to display an icon.
*
*/
public class IronIconset extends PolymerWidget {
/**
* Default Constructor.
*/
public IronIconset() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronIconset(String html) {
super(IronIconsetElement.TAG, IronIconsetElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronIconsetElement getPolymerElement() {
return (IronIconsetElement) getElement();
}
/**
* Array of fully-qualified names of icons in this set.
*
* JavaScript Info:
* @property iconNames
* @type Array
*
*/
public JsArray getIconNames() {
return getPolymerElement().getIconNames();
}
/**
* Array of fully-qualified names of icons in this set.
*
* JavaScript Info:
* @property iconNames
* @type Array
*
*/
public void setIconNames(JsArray value) {
getPolymerElement().setIconNames(value);
}
/**
* The size of an individual icon. Note that icons must be square.
*
* JavaScript Info:
* @property size
* @type Number
*
*/
public double getSize() {
return getPolymerElement().getSize();
}
/**
* The size of an individual icon. Note that icons must be square.
*
* JavaScript Info:
* @property size
* @type Number
*
*/
public void setSize(double value) {
getPolymerElement().setSize(value);
}
/**
* The width of the iconset image. This must only be specified if the
icons are arranged into separate rows inside the image.
*
* JavaScript Info:
* @property width
* @type Number
*
*/
public double getWidth() {
return getPolymerElement().getWidth();
}
/**
* The width of the iconset image. This must only be specified if the
icons are arranged into separate rows inside the image.
*
* JavaScript Info:
* @property width
* @type Number
*
*/
public void setWidth(double value) {
getPolymerElement().setWidth(value);
}
/**
* A space separated list of names corresponding to icons in the iconset
image file. This list must be ordered the same as the icon images
in the image file.
*
* JavaScript Info:
* @property icons
* @type String
*
*/
public String getIcons() {
return getPolymerElement().getIcons();
}
/**
* A space separated list of names corresponding to icons in the iconset
image file. This list must be ordered the same as the icon images
in the image file.
*
* JavaScript Info:
* @property icons
* @type String
*
*/
public void setIcons(String value) {
getPolymerElement().setIcons(value);
}
/**
* The name of the iconset.
*
* JavaScript Info:
* @property name
* @type String
*
*/
public String getName() {
return getPolymerElement().getName();
}
/**
* The name of the iconset.
*
* JavaScript Info:
* @property name
* @type String
*
*/
public void setName(String value) {
getPolymerElement().setName(value);
}
/**
* The URL of the iconset image.
*
* JavaScript Info:
* @property src
* @type String
*
*/
public String getSrc() {
return getPolymerElement().getSrc();
}
/**
* The URL of the iconset image.
*
* JavaScript Info:
* @property src
* @type String
*
*/
public void setSrc(String value) {
getPolymerElement().setSrc(value);
}
// Needed in UIBinder
/**
* Array of fully-qualified names of icons in this set.
*
* JavaScript Info:
* @attribute icon-names
*
*/
public void setIconNames(String value) {
Polymer.property(this.getPolymerElement(), "iconNames", value);
}
// Needed in UIBinder
/**
* The size of an individual icon. Note that icons must be square.
*
* JavaScript Info:
* @attribute size
*
*/
public void setSize(String value) {
Polymer.property(this.getPolymerElement(), "size", value);
}
// Needed in UIBinder
/**
* The width of the iconset image. This must only be specified if the
icons are arranged into separate rows inside the image.
*
* JavaScript Info:
* @attribute width
*
*/
public void setWidth(String value) {
Polymer.property(this.getPolymerElement(), "width", value);
}
/**
* Remove an icon from the given element by undoing the changes effected
by applyIcon
.
*
* JavaScript Info:
* @method removeIcon
* @param {Element} element
*
*
*/
public void removeIcon(Element element) {
getPolymerElement().removeIcon(element);
}
/**
* Applies an icon to the given element as a css background image. This
method does not size the element, and it’s usually necessary to set
the element’s height and width so that the background image is visible.
*
* JavaScript Info:
* @method applyIcon
* @param {Element} element
* @param {(string|number)} icon
* @param {string=} theme
* @param {number=} scale
*
*
*/
public void applyIcon(Element element, Object icon, String theme, double scale) {
getPolymerElement().applyIcon(element, icon, theme, scale);
}
}