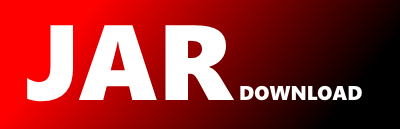
com.vaadin.polymer.iron.widget.IronList Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-list project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* iron-list
displays a virtual, ‘infinite’ list. The template inside
the iron-list element represents the DOM to create for each list item.
The items
property specifies an array of list item data.
* For performance reasons, not every item in the list is rendered at once;
instead a small subset of actual template elements (enough to fill the viewport)
are rendered and reused as the user scrolls. As such, it is important that all
state of the list template be bound to the model driving it, since the view may
be reused with a new model at any time. Particularly, any state that may change
as the result of a user interaction with the list item must be bound to the model
to avoid view state inconsistency.
* Sizing iron-list
* iron-list
must either be explicitly sized, or delegate scrolling to an
explicitly sized parent. By “explicitly sized”, we mean it either has an explicit
CSS height
property set via a class or inline style, or else is sized by other
layout means (e.g. the flex
or fit
classes).
* Flexbox - jsbin
* <template is="x-list">
* <style>
* :host {
* display: block;
* height: 100vh;
* display: flex;
* flex-direction: column;
* }
*
* iron-list {
* flex: 1 1 auto;
* }
* </style>
* <app-toolbar>App name</app-toolbar>
* <iron-list items="[[items]]">
* <template>
* <div>
* ...
* </div>
* </template>
* </iron-list>
* </template>
*
* Explicit size - jsbin
* <template is="x-list">
* <style>
* :host {
* display: block;
* }
*
* iron-list {
* height: 100vh; /* don't use % values unless the parent element is sized. * /
* }
* </style>
* <iron-list items="[[items]]">
* <template>
* <div>
* ...
* </div>
* </template>
* </iron-list>
* </template>
*
* Main document scrolling - jsbin
* <head>
* <style>
* body {
* height: 100vh;
* margin: 0;
* display: flex;
* flex-direction: column;
* }
*
* app-toolbar {
* position: fixed;
* top: 0;
* left: 0;
* right: 0;
* }
*
* iron-list {
* /* add padding since the app-toolbar is fixed at the top * /
* padding-top: 64px;
* }
* </style>
* </head>
* <body>
* <template is="dom-bind">
* <app-toolbar>App name</app-toolbar>
* <iron-list scroll-target="document" items="[[items]]">
* <template>
* <div>
* ...
* </div>
* </template>
* </iron-list>
* </template>
* </body>
*
* iron-list
must be given a <template>
which contains exactly one element. In the examples
above we used a <div>
, but you can provide any element (including custom elements).
* Template model
* List item templates should bind to template models of the following structure:
* {
* index: 0, // index in the item array
* selected: false, // true if the current item is selected
* tabIndex: -1, // a dynamically generated tabIndex for focus management
* item: {} // user data corresponding to items[index]
* }
*
* Alternatively, you can change the property name used as data index by changing the
indexAs
property. The as
property defines the name of the variable to add to the binding
scope for the array.
* For example, given the following data
array:
* data.json
* [
* {"name": "Bob"},
* {"name": "Tim"},
* {"name": "Mike"}
* ]
*
* The following code would render the list (note the name and checked properties are
bound from the model object provided to the template scope):
* <template is="dom-bind">
* <iron-ajax url="data.json" last-response="{{data}}" auto></iron-ajax>
* <iron-list items="[[data]]" as="item">
* <template>
* <div>
* Name: [[item.name]]
* </div>
* </template>
* </iron-list>
* </template>
*
* Grid layout
* iron-list
supports a grid layout in addition to linear layout by setting
the grid
attribute. In this case, the list template item must have both fixed
width and height (e.g. via CSS). Based on this, the number of items
per row are determined automatically based on the size of the list viewport.
* Accessibility
* iron-list
automatically manages the focus state for the items. It also provides
a tabIndex
property within the template scope that can be used for keyboard navigation.
For example, users can press the up and down keys to move to previous and next
items in the list:
* <iron-list items="[[data]]" as="item">
* <template>
* <div tabindex$="[[tabIndex]]">
* Name: [[item.name]]
* </div>
* </template>
* </iron-list>
*
* Styling
* You can use the --iron-list-items-container
mixin to style the container of items:
* iron-list {
* --iron-list-items-container: {
* margin: auto;
* };
* }
*
* Resizing
* iron-list
lays out the items when it receives a notification via the iron-resize
event.
This event is fired by any element that implements IronResizableBehavior
.
* By default, elements such as iron-pages
, paper-tabs
or paper-dialog
will trigger
this event automatically. If you hide the list manually (e.g. you use display: none
)
you might want to implement IronResizableBehavior
or fire this event manually right
after the list became visible again. For example:
* document.querySelector('iron-list').fire('iron-resize');
*
* When should <iron-list>
be used?
* iron-list
should be used when a page has significantly more DOM nodes than the ones
visible on the screen. e.g. the page has 500 nodes, but only 20 are visible at a time.
This is why we refer to it as a virtual
list. In this case, a dom-repeat
will still
create 500 nodes which could slow down the web app, but iron-list
will only create 20.
* However, having an iron-list
does not mean that you can load all the data at once.
Say you have a million records in the database, you want to split the data into pages
so you can bring in a page at the time. The page could contain 500 items, and iron-list
will only render 20.
*/
public class IronList extends PolymerWidget {
/**
* Default Constructor.
*/
public IronList() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronList(String html) {
super(IronListElement.TAG, IronListElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronListElement getPolymerElement() {
return (IronListElement) getElement();
}
/**
* An array containing items determining how many instances of the template
to stamp and that that each template instance should bind to.
*
* JavaScript Info:
* @property items
* @type Array
*
*/
public JsArray getItems() {
return getPolymerElement().getItems();
}
/**
* An array containing items determining how many instances of the template
to stamp and that that each template instance should bind to.
*
* JavaScript Info:
* @property items
* @type Array
*
*/
public void setItems(JsArray value) {
getPolymerElement().setItems(value);
}
/**
* Gets the index of the last visible item in the viewport.
*
* JavaScript Info:
* @property lastVisibleIndex
* @type number
*
*/
public double getLastVisibleIndex() {
return getPolymerElement().getLastVisibleIndex();
}
/**
* Gets the index of the last visible item in the viewport.
*
* JavaScript Info:
* @property lastVisibleIndex
* @type number
*
*/
public void setLastVisibleIndex(double value) {
getPolymerElement().setLastVisibleIndex(value);
}
/**
* The max count of physical items the pool can extend to.
*
* JavaScript Info:
* @property maxPhysicalCount
* @type Number
*
*/
public double getMaxPhysicalCount() {
return getPolymerElement().getMaxPhysicalCount();
}
/**
* The max count of physical items the pool can extend to.
*
* JavaScript Info:
* @property maxPhysicalCount
* @type Number
*
*/
public void setMaxPhysicalCount(double value) {
getPolymerElement().setMaxPhysicalCount(value);
}
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
public Element getScrollTarget() {
return getPolymerElement().getScrollTarget();
}
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
public void setScrollTarget(Element value) {
getPolymerElement().setScrollTarget(value);
}
/**
* The offset top from the scrolling element to the iron-list element.
This value can be computed using the position returned by getBoundingClientRect()
although it’s preferred to use a constant value when possible.
* This property is useful when an external scrolling element is used and there’s
some offset between the scrolling element and the list.
For example: a header is placed above the list.
*
* JavaScript Info:
* @property scrollOffset
* @type Number
*
*/
public double getScrollOffset() {
return getPolymerElement().getScrollOffset();
}
/**
* The offset top from the scrolling element to the iron-list element.
This value can be computed using the position returned by getBoundingClientRect()
although it’s preferred to use a constant value when possible.
* This property is useful when an external scrolling element is used and there’s
some offset between the scrolling element and the list.
For example: a header is placed above the list.
*
* JavaScript Info:
* @property scrollOffset
* @type Number
*
*/
public void setScrollOffset(double value) {
getPolymerElement().setScrollOffset(value);
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public JavaScriptObject getKeyEventTarget() {
return getPolymerElement().getKeyEventTarget();
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(JavaScriptObject value) {
getPolymerElement().setKeyEventTarget(value);
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
public JavaScriptObject getKeyBindings() {
return getPolymerElement().getKeyBindings();
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
public void setKeyBindings(JavaScriptObject value) {
getPolymerElement().setKeyBindings(value);
}
/**
* When multiSelection
is false, this is the currently selected item, or null
if no item is selected.
*
* JavaScript Info:
* @property selectedItem
* @type Object
*
*/
public JavaScriptObject getSelectedItem() {
return getPolymerElement().getSelectedItem();
}
/**
* When multiSelection
is false, this is the currently selected item, or null
if no item is selected.
*
* JavaScript Info:
* @property selectedItem
* @type Object
*
*/
public void setSelectedItem(JavaScriptObject value) {
getPolymerElement().setSelectedItem(value);
}
/**
* When multiSelection
is true, this is an array that contains the selected items.
*
* JavaScript Info:
* @property selectedItems
* @type Object
*
*/
public JavaScriptObject getSelectedItems() {
return getPolymerElement().getSelectedItems();
}
/**
* When multiSelection
is true, this is an array that contains the selected items.
*
* JavaScript Info:
* @property selectedItems
* @type Object
*
*/
public void setSelectedItems(JavaScriptObject value) {
getPolymerElement().setSelectedItems(value);
}
/**
* Gets the index of the first visible item in the viewport.
*
* JavaScript Info:
* @property firstVisibleIndex
* @type number
*
*/
public double getFirstVisibleIndex() {
return getPolymerElement().getFirstVisibleIndex();
}
/**
* Gets the index of the first visible item in the viewport.
*
* JavaScript Info:
* @property firstVisibleIndex
* @type number
*
*/
public void setFirstVisibleIndex(double value) {
getPolymerElement().setFirstVisibleIndex(value);
}
/**
* When true, the list is rendered as a grid. Grid items must have
fixed width and height set via CSS. e.g.
* <iron-list grid>
* <template>
* <div style="width: 100px; height: 100px;"> 100x100 </div>
* </template>
* </iron-list>
*
*
* JavaScript Info:
* @property grid
* @type Boolean
*
*/
public boolean getGrid() {
return getPolymerElement().getGrid();
}
/**
* When true, the list is rendered as a grid. Grid items must have
fixed width and height set via CSS. e.g.
* <iron-list grid>
* <template>
* <div style="width: 100px; height: 100px;"> 100x100 </div>
* </template>
* </iron-list>
*
*
* JavaScript Info:
* @property grid
* @type Boolean
*
*/
public void setGrid(boolean value) {
getPolymerElement().setGrid(value);
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public boolean getStopKeyboardEventPropagation() {
return getPolymerElement().getStopKeyboardEventPropagation();
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public void setStopKeyboardEventPropagation(boolean value) {
getPolymerElement().setStopKeyboardEventPropagation(value);
}
/**
* When true, tapping a row will select the item, placing its data model
in the set of selected items retrievable via the selection property.
* Note that tapping focusable elements within the list item will not
result in selection, since they are presumed to have their * own action.
*
* JavaScript Info:
* @property selectionEnabled
* @type Boolean
*
*/
public boolean getSelectionEnabled() {
return getPolymerElement().getSelectionEnabled();
}
/**
* When true, tapping a row will select the item, placing its data model
in the set of selected items retrievable via the selection property.
* Note that tapping focusable elements within the list item will not
result in selection, since they are presumed to have their * own action.
*
* JavaScript Info:
* @property selectionEnabled
* @type Boolean
*
*/
public void setSelectionEnabled(boolean value) {
getPolymerElement().setSelectionEnabled(value);
}
/**
* When true
, multiple items may be selected at once (in this case,
selected
is an array of currently selected items). When false
,
only one item may be selected at a time.
*
* JavaScript Info:
* @property multiSelection
* @type Boolean
*
*/
public boolean getMultiSelection() {
return getPolymerElement().getMultiSelection();
}
/**
* When true
, multiple items may be selected at once (in this case,
selected
is an array of currently selected items). When false
,
only one item may be selected at a time.
*
* JavaScript Info:
* @property multiSelection
* @type Boolean
*
*/
public void setMultiSelection(boolean value) {
getPolymerElement().setMultiSelection(value);
}
/**
* The name of the variable to add to the binding scope to indicate
if the row is selected.
*
* JavaScript Info:
* @property selectedAs
* @type String
*
*/
public String getSelectedAs() {
return getPolymerElement().getSelectedAs();
}
/**
* The name of the variable to add to the binding scope to indicate
if the row is selected.
*
* JavaScript Info:
* @property selectedAs
* @type String
*
*/
public void setSelectedAs(String value) {
getPolymerElement().setSelectedAs(value);
}
/**
* The name of the variable to add to the binding scope with the index
for the row.
*
* JavaScript Info:
* @property indexAs
* @type String
*
*/
public String getIndexAs() {
return getPolymerElement().getIndexAs();
}
/**
* The name of the variable to add to the binding scope with the index
for the row.
*
* JavaScript Info:
* @property indexAs
* @type String
*
*/
public void setIndexAs(String value) {
getPolymerElement().setIndexAs(value);
}
/**
* The name of the variable to add to the binding scope for the array
element associated with a given template instance.
*
* JavaScript Info:
* @property as
* @type String
*
*/
public String getAs() {
return getPolymerElement().getAs();
}
/**
* The name of the variable to add to the binding scope for the array
element associated with a given template instance.
*
* JavaScript Info:
* @property as
* @type String
*
*/
public void setAs(String value) {
getPolymerElement().setAs(value);
}
// Needed in UIBinder
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @attribute scroll-target
* @behavior IronScrollThreshold
*/
public void setScrollTarget(String value) {
Polymer.property(this.getPolymerElement(), "scrollTarget", value);
}
// Needed in UIBinder
/**
* The offset top from the scrolling element to the iron-list element.
This value can be computed using the position returned by getBoundingClientRect()
although it’s preferred to use a constant value when possible.
* This property is useful when an external scrolling element is used and there’s
some offset between the scrolling element and the list.
For example: a header is placed above the list.
*
* JavaScript Info:
* @attribute scroll-offset
*
*/
public void setScrollOffset(String value) {
Polymer.property(this.getPolymerElement(), "scrollOffset", value);
}
// Needed in UIBinder
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @attribute key-event-target
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(String value) {
Polymer.property(this.getPolymerElement(), "keyEventTarget", value);
}
// Needed in UIBinder
/**
*
*
* JavaScript Info:
* @attribute key-bindings
*
*/
public void setKeyBindings(String value) {
Polymer.property(this.getPolymerElement(), "keyBindings", value);
}
// Needed in UIBinder
/**
* When multiSelection
is false, this is the currently selected item, or null
if no item is selected.
*
* JavaScript Info:
* @attribute selected-item
*
*/
public void setSelectedItem(String value) {
Polymer.property(this.getPolymerElement(), "selectedItem", value);
}
// Needed in UIBinder
/**
* The max count of physical items the pool can extend to.
*
* JavaScript Info:
* @attribute max-physical-count
*
*/
public void setMaxPhysicalCount(String value) {
Polymer.property(this.getPolymerElement(), "maxPhysicalCount", value);
}
// Needed in UIBinder
/**
* Gets the index of the first visible item in the viewport.
*
* JavaScript Info:
* @attribute first-visible-index
*
*/
public void setFirstVisibleIndex(String value) {
Polymer.property(this.getPolymerElement(), "firstVisibleIndex", value);
}
// Needed in UIBinder
/**
* Gets the index of the last visible item in the viewport.
*
* JavaScript Info:
* @attribute last-visible-index
*
*/
public void setLastVisibleIndex(String value) {
Polymer.property(this.getPolymerElement(), "lastVisibleIndex", value);
}
// Needed in UIBinder
/**
* An array containing items determining how many instances of the template
to stamp and that that each template instance should bind to.
*
* JavaScript Info:
* @attribute items
*
*/
public void setItems(String value) {
Polymer.property(this.getPolymerElement(), "items", value);
}
// Needed in UIBinder
/**
* When multiSelection
is true, this is an array that contains the selected items.
*
* JavaScript Info:
* @attribute selected-items
*
*/
public void setSelectedItems(String value) {
Polymer.property(this.getPolymerElement(), "selectedItems", value);
}
/**
* Deselects the given item list if it is already selected.
*
* JavaScript Info:
* @method deselectItem
* @param {(Object|number)} item
*
*
*/
public void deselectItem(Object item) {
getPolymerElement().deselectItem(item);
}
/**
* Updates the size of an item.
*
* JavaScript Info:
* @method updateSizeForItem
* @param {(Object|number)} item
*
*
*/
public void updateSizeForItem(Object item) {
getPolymerElement().updateSizeForItem(item);
}
/**
*
*
* JavaScript Info:
* @method modelForElement
* @param {} el
* @behavior VaadinInfiniteScroller
*
*/
public void modelForElement(Object el) {
getPolymerElement().modelForElement(el);
}
/**
*
*
* JavaScript Info:
* @method stamp
* @param {} model
* @behavior VaadinInfiniteScroller
*
*/
public void stamp(Object model) {
getPolymerElement().stamp(model);
}
/**
* Select or deselect a given item depending on whether the item
has already been selected.
*
* JavaScript Info:
* @method toggleSelectionForItem
* @param {(Object|number)} item
*
*
*/
public void toggleSelectionForItem(Object item) {
getPolymerElement().toggleSelectionForItem(item);
}
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
public void assignParentResizable(Object parentResizable) {
getPolymerElement().assignParentResizable(parentResizable);
}
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
public void stopResizeNotificationsFor(Object target) {
getPolymerElement().stopResizeNotificationsFor(target);
}
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
public void addOwnKeyBinding(Object eventString, Object handlerName) {
getPolymerElement().addOwnKeyBinding(eventString, handlerName);
}
/**
* Select the list item at the given index.
*
* JavaScript Info:
* @method selectItem
* @param {(Object|number)} item
*
*
*/
public void selectItem(Object item) {
getPolymerElement().selectItem(item);
}
/**
*
*
* JavaScript Info:
* @method templatize
* @param {} template
* @behavior VaadinInfiniteScroller
*
*/
public void templatize(Object template) {
getPolymerElement().templatize(template);
}
/**
* Clears the current selection state of the list.
*
* JavaScript Info:
* @method clearSelection
*
*
*/
public void clearSelection() {
getPolymerElement().clearSelection();
}
/**
* Invoke this method if you dynamically update the viewport’s
size or CSS padding.
*
* JavaScript Info:
* @method updateViewportBoundaries
*
*
*/
public void updateViewportBoundaries() {
getPolymerElement().updateViewportBoundaries();
}
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
public void notifyResize() {
getPolymerElement().notifyResize();
}
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
public void removeOwnKeyBindings() {
getPolymerElement().removeOwnKeyBindings();
}
/**
* Enables or disables the scroll event listener.
*
* JavaScript Info:
* @method toggleScrollListener
* @param {boolean} yes
* @behavior IronScrollThreshold
*
*/
public void toggleScrollListener(boolean yes) {
getPolymerElement().toggleScrollListener(yes);
}
/**
* Scroll to a specific index in the virtual list regardless
of the physical items in the DOM tree.
*
* JavaScript Info:
* @method scrollToIndex
* @param {number} idx
*
*
*/
public void scrollToIndex(double idx) {
getPolymerElement().scrollToIndex(idx);
}
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
public boolean resizerShouldNotify(JavaScriptObject element) {
return getPolymerElement().resizerShouldNotify(element);
}
/**
* Scroll to a specific item in the virtual list regardless
of the physical items in the DOM tree.
*
* JavaScript Info:
* @method scrollToItem
* @param {(Object)} item
*
*
*/
public void scrollToItem(JavaScriptObject item) {
getPolymerElement().scrollToItem(item);
}
/**
* Scrolls the content to a particular place.
*
* JavaScript Info:
* @method scroll
* @param {number} left
* @param {number} top
* @behavior IronScrollThreshold
*
*/
public void scroll(double left, double top) {
getPolymerElement().scroll(left, top);
}
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
public boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString) {
return getPolymerElement().keyboardEventMatchesKeys(event, eventString);
}
}