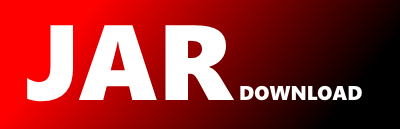
com.vaadin.polymer.iron.widget.IronRequest Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-ajax project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* iron-request can be used to perform XMLHttpRequests.
* <iron-request id="xhr"></iron-request>
* ...
* this.$.xhr.send({url: url, body: params});
*
*
*/
public class IronRequest extends PolymerWidget {
/**
* Default Constructor.
*/
public IronRequest() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronRequest(String html) {
super(IronRequestElement.TAG, IronRequestElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronRequestElement getPolymerElement() {
return (IronRequestElement) getElement();
}
/**
* A promise that resolves when the xhr
response comes back, or rejects
if there is an error before the xhr
completes.
*
* JavaScript Info:
* @property completes
* @type Promise
*
*/
public JavaScriptObject getCompletes() {
return getPolymerElement().getCompletes();
}
/**
* A promise that resolves when the xhr
response comes back, or rejects
if there is an error before the xhr
completes.
*
* JavaScript Info:
* @property completes
* @type Promise
*
*/
public void setCompletes(JavaScriptObject value) {
getPolymerElement().setCompletes(value);
}
/**
* Errored will be true if the browser fired an error event from the
XHR object (mainly network errors).
*
* JavaScript Info:
* @property errored
* @type Boolean
*
*/
public boolean getErrored() {
return getPolymerElement().getErrored();
}
/**
* Errored will be true if the browser fired an error event from the
XHR object (mainly network errors).
*
* JavaScript Info:
* @property errored
* @type Boolean
*
*/
public void setErrored(boolean value) {
getPolymerElement().setErrored(value);
}
/**
* An object that contains progress information emitted by the XHR if
available.
*
* JavaScript Info:
* @property progress
* @type Object
*
*/
public JavaScriptObject getProgress() {
return getPolymerElement().getProgress();
}
/**
* An object that contains progress information emitted by the XHR if
available.
*
* JavaScript Info:
* @property progress
* @type Object
*
*/
public void setProgress(JavaScriptObject value) {
getPolymerElement().setProgress(value);
}
/**
* A reference to the parsed response body, if the xhr
has completely
resolved.
*
* JavaScript Info:
* @property response
* @type *
*
*/
public JavaScriptObject getResponse() {
return getPolymerElement().getResponse();
}
/**
* A reference to the parsed response body, if the xhr
has completely
resolved.
*
* JavaScript Info:
* @property response
* @type *
*
*/
public void setResponse(JavaScriptObject value) {
getPolymerElement().setResponse(value);
}
/**
* Aborted will be true if an abort of the request is attempted.
*
* JavaScript Info:
* @property aborted
* @type Boolean
*
*/
public boolean getAborted() {
return getPolymerElement().getAborted();
}
/**
* Aborted will be true if an abort of the request is attempted.
*
* JavaScript Info:
* @property aborted
* @type Boolean
*
*/
public void setAborted(boolean value) {
getPolymerElement().setAborted(value);
}
/**
* A reference to the status code, if the xhr
has completely resolved.
*
* JavaScript Info:
* @property status
* @type Number
*
*/
public double getStatus() {
return getPolymerElement().getStatus();
}
/**
* A reference to the status code, if the xhr
has completely resolved.
*
* JavaScript Info:
* @property status
* @type Number
*
*/
public void setStatus(double value) {
getPolymerElement().setStatus(value);
}
/**
* A reference to the XMLHttpRequest instance used to generate the
network request.
*
* JavaScript Info:
* @property xhr
* @type XMLHttpRequest
*
*/
public JavaScriptObject getXhr() {
return getPolymerElement().getXhr();
}
/**
* A reference to the XMLHttpRequest instance used to generate the
network request.
*
* JavaScript Info:
* @property xhr
* @type XMLHttpRequest
*
*/
public void setXhr(JavaScriptObject value) {
getPolymerElement().setXhr(value);
}
/**
* TimedOut will be true if the XHR threw a timeout event.
*
* JavaScript Info:
* @property timedOut
* @type Boolean
*
*/
public boolean getTimedOut() {
return getPolymerElement().getTimedOut();
}
/**
* TimedOut will be true if the XHR threw a timeout event.
*
* JavaScript Info:
* @property timedOut
* @type Boolean
*
*/
public void setTimedOut(boolean value) {
getPolymerElement().setTimedOut(value);
}
/**
* A reference to the status text, if the xhr
has completely resolved.
*
* JavaScript Info:
* @property statusText
* @type String
*
*/
public String getStatusText() {
return getPolymerElement().getStatusText();
}
/**
* A reference to the status text, if the xhr
has completely resolved.
*
* JavaScript Info:
* @property statusText
* @type String
*
*/
public void setStatusText(String value) {
getPolymerElement().setStatusText(value);
}
// Needed in UIBinder
/**
* A reference to the status code, if the xhr
has completely resolved.
*
* JavaScript Info:
* @attribute status
*
*/
public void setStatus(String value) {
Polymer.property(this.getPolymerElement(), "status", value);
}
// Needed in UIBinder
/**
* A promise that resolves when the xhr
response comes back, or rejects
if there is an error before the xhr
completes.
*
* JavaScript Info:
* @attribute completes
*
*/
public void setCompletes(String value) {
Polymer.property(this.getPolymerElement(), "completes", value);
}
// Needed in UIBinder
/**
* A reference to the parsed response body, if the xhr
has completely
resolved.
*
* JavaScript Info:
* @attribute response
*
*/
public void setResponse(String value) {
Polymer.property(this.getPolymerElement(), "response", value);
}
// Needed in UIBinder
/**
* A reference to the XMLHttpRequest instance used to generate the
network request.
*
* JavaScript Info:
* @attribute xhr
*
*/
public void setXhr(String value) {
Polymer.property(this.getPolymerElement(), "xhr", value);
}
// Needed in UIBinder
/**
* An object that contains progress information emitted by the XHR if
available.
*
* JavaScript Info:
* @attribute progress
*
*/
public void setProgress(String value) {
Polymer.property(this.getPolymerElement(), "progress", value);
}
/**
* Sends an HTTP request to the server and returns the XHR object.
* The handling of the body
parameter will vary based on the Content-Type
header. See the docs for iron-ajax’s body
param for details.
*
* JavaScript Info:
* @method send
* @param {{url: string, method: (string|undefined), async: (boolean|undefined), body: (ArrayBuffer|ArrayBufferView|Blob|Document|FormData|null|string|undefined|Object), headers: (Object|undefined), handleAs: (string|undefined), jsonPrefix: (string|undefined), withCredentials: (boolean|undefined)}} options
*
* @return {JavaScriptObject}
*/
public JavaScriptObject send(Object options) {
return getPolymerElement().send(options);
}
/**
* Aborts the request.
*
* JavaScript Info:
* @method abort
*
*
*/
public void abort() {
getPolymerElement().abort();
}
/**
* Attempts to parse the response body of the XHR. If parsing succeeds,
the value returned will be deserialized based on the responseType
set on the XHR.
*
* JavaScript Info:
* @method parseResponse
*
* @return {JavaScriptObject}
*/
public JavaScriptObject parseResponse() {
return getPolymerElement().parseResponse();
}
}