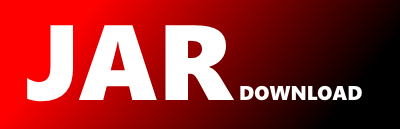
com.vaadin.polymer.marked.widget.MarkedElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from marked-element project by The Polymer Project Authors (https://polymer.github.io/AUTHORS.txt)
* that is licensed with https://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.marked.widget;
import com.vaadin.polymer.marked.*;
import com.vaadin.polymer.marked.widget.event.MarkedLoadendEvent;
import com.vaadin.polymer.marked.widget.event.MarkedLoadendEventHandler;
import com.vaadin.polymer.marked.widget.event.MarkedRenderCompleteEvent;
import com.vaadin.polymer.marked.widget.event.MarkedRenderCompleteEventHandler;
import com.vaadin.polymer.marked.widget.event.MarkedRequestErrorEvent;
import com.vaadin.polymer.marked.widget.event.MarkedRequestErrorEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Element wrapper for the marked library.
* <marked-element>
accepts Markdown source and renders it to a child
element with the class markdown-html
. This child element can be styled
as you would a normal DOM element. If you do not provide a child element
with the markdown-html
class, the Markdown source will still be rendered,
but to a shadow DOM child that cannot be styled.
* Markdown Content
* The Markdown source can be specified several ways:
* Use the markdown
attribute to bind markdown
* <marked-element markdown="`Markdown` is _awesome_!">
* <div class="markdown-html"></div>
* </marked-element>
*
*
*
Use <script type="text/markdown">
element child to inline markdown
* <marked-element>
* <div class="markdown-html"></div>
* <script type="text/markdown">
* Check out my markdown!
*
* We can even embed elements without fear of the HTML parser mucking up their
* textual representation:
*
* ```html
* <awesome-sauce>
* <div>Oops, I'm about to forget to close this div.
* </awesome-sauce>
* ```
* </script>
* </marked-element>
*
*
*
Use <script type="text/markdown" src="URL">
element child to specify remote markdown
* <marked-element>
* <div class="markdown-html"></div>
* <script type="text/markdown" src="../guidelines.md"></script>
* </marked-element>
*
*
*
Note that the <script type="text/markdown">
approach is static. Changes to
the script content will not update the rendered markdown!
* Though, you can data bind to the src
attribute to change the markdown.
* <marked-element>
* <div class="markdown-html"></div>
* <script type="text/markdown" src$="[[source]]"></script>
* </marked-element>
* ...
* <script>
* ...
* this.source = '../guidelines.md';
* </script>
*
* Styling
* If you are using a child with the markdown-html
class, you can style it
as you would a regular DOM element:
* .markdown-html p {
* color: red;
* }
*
* .markdown-html td:first-child {
* padding-left: 24px;
* }
*
*
*
*/
public class MarkedElement extends PolymerWidget {
/**
* Default Constructor.
*/
public MarkedElement() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public MarkedElement(String html) {
super(MarkedElementElement.TAG, MarkedElementElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public MarkedElementElement getPolymerElement() {
return (MarkedElementElement) getElement();
}
/**
* Enable GFM line breaks (regular newlines instead of two spaces for breaks)
*
* JavaScript Info:
* @property breaks
* @type Boolean
*
*/
public boolean getBreaks() {
return getPolymerElement().getBreaks();
}
/**
* Enable GFM line breaks (regular newlines instead of two spaces for breaks)
*
* JavaScript Info:
* @property breaks
* @type Boolean
*
*/
public void setBreaks(boolean value) {
getPolymerElement().setBreaks(value);
}
/**
* A reference to the XMLHttpRequest instance used to generate the
network request.
*
* JavaScript Info:
* @property xhr
* @type XMLHttpRequest
*
*/
public JavaScriptObject getXhr() {
return getPolymerElement().getXhr();
}
/**
* A reference to the XMLHttpRequest instance used to generate the
network request.
*
* JavaScript Info:
* @property xhr
* @type XMLHttpRequest
*
*/
public void setXhr(JavaScriptObject value) {
getPolymerElement().setXhr(value);
}
/**
* Conform to obscure parts of markdown.pl as much as possible. Don’t fix any of the original markdown bugs or poor behavior.
*
* JavaScript Info:
* @property pedantic
* @type Boolean
*
*/
public boolean getPedantic() {
return getPolymerElement().getPedantic();
}
/**
* Conform to obscure parts of markdown.pl as much as possible. Don’t fix any of the original markdown bugs or poor behavior.
*
* JavaScript Info:
* @property pedantic
* @type Boolean
*
*/
public void setPedantic(boolean value) {
getPolymerElement().setPedantic(value);
}
/**
* Sanitize the output. Ignore any HTML that has been input.
*
* JavaScript Info:
* @property sanitize
* @type Boolean
*
*/
public boolean getSanitize() {
return getPolymerElement().getSanitize();
}
/**
* Sanitize the output. Ignore any HTML that has been input.
*
* JavaScript Info:
* @property sanitize
* @type Boolean
*
*/
public void setSanitize(boolean value) {
getPolymerElement().setSanitize(value);
}
/**
* Use “smart” typographic punctuation for things like quotes and dashes.
*
* JavaScript Info:
* @property smartypants
* @type Boolean
*
*/
public boolean getSmartypants() {
return getPolymerElement().getSmartypants();
}
/**
* Use “smart” typographic punctuation for things like quotes and dashes.
*
* JavaScript Info:
* @property smartypants
* @type Boolean
*
*/
public void setSmartypants(boolean value) {
getPolymerElement().setSmartypants(value);
}
/**
* Callback function invoked by Marked after HTML has been rendered.
It must take two arguments: err and text and must return the resulting text.
*
* JavaScript Info:
* @property callback
* @type Function
*
*/
public Function getCallback() {
return getPolymerElement().getCallback();
}
/**
* Callback function invoked by Marked after HTML has been rendered.
It must take two arguments: err and text and must return the resulting text.
*
* JavaScript Info:
* @property callback
* @type Function
*
*/
public void setCallback(Function value) {
getPolymerElement().setCallback(value);
}
/**
* Function used to customize a renderer based on the API specified in the Marked
library.
It takes one argument: a marked renderer object, which is mutated by the function.
*
* JavaScript Info:
* @property renderer
* @type Function
*
*/
public Function getRenderer() {
return getPolymerElement().getRenderer();
}
/**
* Function used to customize a renderer based on the API specified in the Marked
library.
It takes one argument: a marked renderer object, which is mutated by the function.
*
* JavaScript Info:
* @property renderer
* @type Function
*
*/
public void setRenderer(Function value) {
getPolymerElement().setRenderer(value);
}
/**
* The markdown source that should be rendered by this element.
*
* JavaScript Info:
* @property markdown
* @type String
*
*/
public String getMarkdown() {
return getPolymerElement().getMarkdown();
}
/**
* The markdown source that should be rendered by this element.
*
* JavaScript Info:
* @property markdown
* @type String
*
*/
public void setMarkdown(String value) {
getPolymerElement().setMarkdown(value);
}
// Needed in UIBinder
/**
* A reference to the XMLHttpRequest instance used to generate the
network request.
*
* JavaScript Info:
* @attribute xhr
*
*/
public void setXhr(String value) {
Polymer.property(this.getPolymerElement(), "xhr", value);
}
/**
* Unindents the markdown source that will be rendered.
*
* JavaScript Info:
* @method unindent
* @param {} text
*
*
*/
public void unindent(Object text) {
getPolymerElement().unindent(text);
}
/**
* Renders markdown
into this element’s DOM.
* This is automatically called whenever the markdown
property is changed.
* The only case where you should be calling this is if you are providing
markdown via <script type="text/markdown">
after this element has been
constructed (or updating that markdown).
*
* JavaScript Info:
* @method render
*
*
*/
public void render() {
getPolymerElement().render();
}
/**
* Fired when the XHR finishes loading
*
* JavaScript Info:
* @event marked-loadend
*/
public HandlerRegistration addMarkedLoadendHandler(MarkedLoadendEventHandler handler) {
return addDomHandler(handler, MarkedLoadendEvent.TYPE);
}
/**
* The marked-render-complete
event is fired once Markdown to HTML
conversion has finished, and the DOM has been populated via the resulting
HTML.
*
* JavaScript Info:
* @event marked-render-complete
*/
public HandlerRegistration addMarkedRenderCompleteHandler(MarkedRenderCompleteEventHandler handler) {
return addDomHandler(handler, MarkedRenderCompleteEvent.TYPE);
}
/**
* Fired when an error is received while fetching remote markdown content.
*
* JavaScript Info:
* @event marked-request-error
*/
public HandlerRegistration addMarkedRequestErrorHandler(MarkedRequestErrorEventHandler handler) {
return addDomHandler(handler, MarkedRequestErrorEvent.TYPE);
}
}