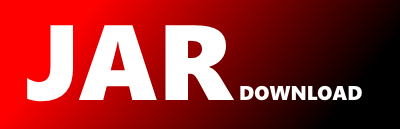
com.vaadin.polymer.neon.NeonAnimatedPagesElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from neon-animation project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.neon;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Material design: Meaningful transitions
* neon-animated-pages
manages a set of pages and runs an animation when switching between them. Its
children pages should implement Polymer.NeonAnimatableBehavior
and define entry
and exit
animations to be run when switching to or switching out of the page.
*/
@JsType(isNative=true)
public interface NeonAnimatedPagesElement extends HTMLElement {
@JsOverlay public static final String TAG = "neon-animated-pages";
@JsOverlay public static final String SRC = "neon-animation/neon-animation.html";
/**
* if true, the initial page selection will also be animated according to its animation config.
*
* JavaScript Info:
* @property animateInitialSelection
* @type Boolean
*
*/
@JsProperty boolean getAnimateInitialSelection();
/**
* if true, the initial page selection will also be animated according to its animation config.
*
* JavaScript Info:
* @property animateInitialSelection
* @type Boolean
*
*/
@JsProperty void setAnimateInitialSelection(boolean value);
/**
* Gets or sets the selected element. The default is to use the index of the item.
*
* JavaScript Info:
* @property selected
* @type (string|number)
* @behavior PaperTabs
*/
@JsProperty Object getSelected();
/**
* Gets or sets the selected element. The default is to use the index of the item.
*
* JavaScript Info:
* @property selected
* @type (string|number)
* @behavior PaperTabs
*/
@JsProperty void setSelected(Object value);
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
*
*/
@JsProperty JavaScriptObject getAnimationConfig();
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
*
*/
@JsProperty void setAnimationConfig(JavaScriptObject value);
/**
* Returns the currently selected item.
*
* JavaScript Info:
* @property selectedItem
* @type ?Object
* @behavior PaperTabs
*/
@JsProperty JavaScriptObject getSelectedItem();
/**
* Returns the currently selected item.
*
* JavaScript Info:
* @property selectedItem
* @type ?Object
* @behavior PaperTabs
*/
@JsProperty void setSelectedItem(JavaScriptObject value);
/**
* The list of items from which a selection can be made.
*
* JavaScript Info:
* @property items
* @type Array
* @behavior PaperTabs
*/
@JsProperty JsArray getItems();
/**
* The list of items from which a selection can be made.
*
* JavaScript Info:
* @property items
* @type Array
* @behavior PaperTabs
*/
@JsProperty void setItems(JsArray value);
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior PaperTooltip
*/
@JsProperty String getExitAnimation();
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior PaperTooltip
*/
@JsProperty void setExitAnimation(String value);
/**
* The class to set on elements when selected.
*
* JavaScript Info:
* @property selectedClass
* @type String
* @behavior PaperTabs
*/
@JsProperty String getSelectedClass();
/**
* The class to set on elements when selected.
*
* JavaScript Info:
* @property selectedClass
* @type String
* @behavior PaperTabs
*/
@JsProperty void setSelectedClass(String value);
/**
* This is a CSS selector string. If this is set, only items that match the CSS selector
are selectable.
*
* JavaScript Info:
* @property selectable
* @type string
* @behavior PaperTabs
*/
@JsProperty String getSelectable();
/**
* This is a CSS selector string. If this is set, only items that match the CSS selector
are selectable.
*
* JavaScript Info:
* @property selectable
* @type string
* @behavior PaperTabs
*/
@JsProperty void setSelectable(String value);
/**
* Default fallback if the selection based on selected with attrForSelected
is not found.
*
* JavaScript Info:
* @property fallbackSelection
* @type String
* @behavior PaperTabs
*/
@JsProperty String getFallbackSelection();
/**
* Default fallback if the selection based on selected with attrForSelected
is not found.
*
* JavaScript Info:
* @property fallbackSelection
* @type String
* @behavior PaperTabs
*/
@JsProperty void setFallbackSelection(String value);
/**
* If you want to use an attribute value or property of an element for
selected
instead of the index, set this to the name of the attribute
or property. Hyphenated values are converted to camel case when used to
look up the property of a selectable element. Camel cased values are
not converted to hyphenated values for attribute lookup. It’s
recommended that you provide the hyphenated form of the name so that
selection works in both cases. (Use attr-or-property-name
instead of
attrOrPropertyName
.)
*
* JavaScript Info:
* @property attrForSelected
* @type String
* @behavior PaperTabs
*/
@JsProperty String getAttrForSelected();
/**
* If you want to use an attribute value or property of an element for
selected
instead of the index, set this to the name of the attribute
or property. Hyphenated values are converted to camel case when used to
look up the property of a selectable element. Camel cased values are
not converted to hyphenated values for attribute lookup. It’s
recommended that you provide the hyphenated form of the name so that
selection works in both cases. (Use attr-or-property-name
instead of
attrOrPropertyName
.)
*
* JavaScript Info:
* @property attrForSelected
* @type String
* @behavior PaperTabs
*/
@JsProperty void setAttrForSelected(String value);
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior PaperTooltip
*/
@JsProperty String getEntryAnimation();
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior PaperTooltip
*/
@JsProperty void setEntryAnimation(String value);
/**
* The attribute to set on elements when selected.
*
* JavaScript Info:
* @property selectedAttribute
* @type String
* @behavior PaperTabs
*/
@JsProperty String getSelectedAttribute();
/**
* The attribute to set on elements when selected.
*
* JavaScript Info:
* @property selectedAttribute
* @type String
* @behavior PaperTabs
*/
@JsProperty void setSelectedAttribute(String value);
/**
* The event that fires from items when they are selected. Selectable
will listen for this event from items and update the selection state.
Set to empty string to listen to no events.
*
* JavaScript Info:
* @property activateEvent
* @type String
* @behavior PaperTabs
*/
@JsProperty String getActivateEvent();
/**
* The event that fires from items when they are selected. Selectable
will listen for this event from items and update the selection state.
Set to empty string to listen to no events.
*
* JavaScript Info:
* @property activateEvent
* @type String
* @behavior PaperTabs
*/
@JsProperty void setActivateEvent(String value);
/**
* Selects the item at the given index.
*
* JavaScript Info:
* @method selectIndex
* @param {} index
* @behavior PaperTabs
*
*/
void selectIndex(Object index);
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
void assignParentResizable(Object parentResizable);
/**
* Selects the given value.
*
* JavaScript Info:
* @method select
* @param {(string|number)} value
* @behavior PaperTabs
*
*/
void select(Object value);
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
void stopResizeNotificationsFor(Object target);
/**
* Plays an animation with an optional type
.
*
* JavaScript Info:
* @method playAnimation
* @param {string=} type
* @param {!Object=} cookie
* @behavior PaperTooltip
*
*/
void playAnimation(String type, JavaScriptObject cookie);
/**
* Cancels the currently running animations.
*
* JavaScript Info:
* @method cancelAnimation
*
*
*/
void cancelAnimation();
/**
* Force a synchronous update of the items
property.
* NOTE: Consider listening for the iron-items-changed
event to respond to
updates to the set of selectable items after updates to the DOM list and
selection state have been made.
* WARNING: If you are using this method, you should probably consider an
alternate approach. Synchronously querying for items is potentially
slow for many use cases. The items
property will update asynchronously
on its own to reflect selectable items in the DOM.
*
* JavaScript Info:
* @method forceSynchronousItemUpdate
* @behavior PaperTabs
*
*/
void forceSynchronousItemUpdate();
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
void notifyResize();
/**
* Selects the next item.
*
* JavaScript Info:
* @method selectNext
* @behavior PaperTabs
*
*/
void selectNext();
/**
* Selects the previous item.
*
* JavaScript Info:
* @method selectPrevious
* @behavior PaperTabs
*
*/
void selectPrevious();
/**
* Returns the index of the given item.
*
* JavaScript Info:
* @method indexOf
* @param {Object} item
* @behavior PaperTabs
*
*/
void indexOf(JavaScriptObject item);
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
boolean resizerShouldNotify(JavaScriptObject element);
}