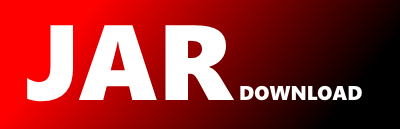
com.vaadin.polymer.neon.ReverseRippleAnimationElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from neon-animation project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.neon;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* <reverse-ripple-animation>
scales and transform an element such that it appears to ripple down from this element, to either
a shared element, or a screen position.
* If using as a shared element animation in <neon-animated-pages>
, use this animation in an exit
animation in the source page and in an entry
animation in the destination page. Also, define the
reverse-ripple elements in the sharedElements
property (not a configuration property, see
Polymer.NeonSharedElementAnimatableBehavior
).
If using a screen position, define the gesture
property.
Configuration:
* {
* name: 'reverse-ripple-animation`.
* id: <shared-element-id>, /* set this or gesture * /
* gesture: {x: <page-x>, y: <page-y>}, /* set this or id * /
* timing: <animation-timing>,
* toPage: <node>, /* define for the destination page * /
* fromPage: <node>, /* define for the source page * /
* }
*
*/
@JsType(isNative=true)
public interface ReverseRippleAnimationElement extends HTMLElement {
@JsOverlay public static final String TAG = "reverse-ripple-animation";
@JsOverlay public static final String SRC = "neon-animation/neon-animation.html";
/**
* Defines the animation timing.
*
* JavaScript Info:
* @property animationTiming
* @type Object
*
*/
@JsProperty JavaScriptObject getAnimationTiming();
/**
* Defines the animation timing.
*
* JavaScript Info:
* @property animationTiming
* @type Object
*
*/
@JsProperty void setAnimationTiming(JavaScriptObject value);
/**
* Can be used to determine that elements implement this behavior.
*
* JavaScript Info:
* @property isNeonAnimation
* @type boolean
*
*/
@JsProperty boolean getIsNeonAnimation();
/**
* Can be used to determine that elements implement this behavior.
*
* JavaScript Info:
* @property isNeonAnimation
* @type boolean
*
*/
@JsProperty void setIsNeonAnimation(boolean value);
/**
* Cached copy of shared elements.
*
* JavaScript Info:
* @property sharedElements
* @type Object
*
*/
@JsProperty JavaScriptObject getSharedElements();
/**
* Cached copy of shared elements.
*
* JavaScript Info:
* @property sharedElements
* @type Object
*
*/
@JsProperty void setSharedElements(JavaScriptObject value);
/**
* Finds shared elements based on config
.
*
* JavaScript Info:
* @method findSharedElements
* @param {} config
* @behavior ReverseRippleAnimation
*
*/
void findSharedElements(Object config);
/**
* Returns the animation timing by mixing in properties from config
to the defaults defined
by the animation.
*
* JavaScript Info:
* @method timingFromConfig
* @param {} config
* @behavior PaperMenuShrinkHeightAnimation
*
*/
void timingFromConfig(Object config);
/**
* Sets transform
and transformOrigin
properties along with the prefixed versions.
*
* JavaScript Info:
* @method setPrefixedProperty
* @param {} node
* @param {} property
* @param {} value
*
*
*/
void setPrefixedProperty(Object node, Object property, Object value);
/**
* Called when the animation finishes.
*
* JavaScript Info:
* @method complete
* @behavior ReverseRippleAnimation
*
*/
void complete();
}