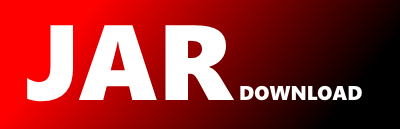
com.vaadin.polymer.neon.widget.NeonAnimatable Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from neon-animation project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.neon.widget;
import com.vaadin.polymer.neon.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* <neon-animatable>
is a simple container element implementing Polymer.NeonAnimatableBehavior
. This is a convenience element for use with <neon-animated-pages>
.
* <neon-animated-pages selected="0"
* entry-animation="slide-from-right-animation"
* exit-animation="slide-left-animation">
* <neon-animatable>1</neon-animatable>
* <neon-animatable>2</neon-animatable>
* </neon-animated-pages>
*
*/
public class NeonAnimatable extends PolymerWidget {
/**
* Default Constructor.
*/
public NeonAnimatable() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public NeonAnimatable(String html) {
super(NeonAnimatableElement.TAG, NeonAnimatableElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public NeonAnimatableElement getPolymerElement() {
return (NeonAnimatableElement) getElement();
}
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
* @behavior PaperTooltip
*/
public JavaScriptObject getAnimationConfig() {
return getPolymerElement().getAnimationConfig();
}
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
* @behavior PaperTooltip
*/
public void setAnimationConfig(JavaScriptObject value) {
getPolymerElement().setAnimationConfig(value);
}
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior PaperTooltip
*/
public String getEntryAnimation() {
return getPolymerElement().getEntryAnimation();
}
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior PaperTooltip
*/
public void setEntryAnimation(String value) {
getPolymerElement().setEntryAnimation(value);
}
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior PaperTooltip
*/
public String getExitAnimation() {
return getPolymerElement().getExitAnimation();
}
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior PaperTooltip
*/
public void setExitAnimation(String value) {
getPolymerElement().setExitAnimation(value);
}
// Needed in UIBinder
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @attribute animation-config
* @behavior PaperTooltip
*/
public void setAnimationConfig(String value) {
Polymer.property(this.getPolymerElement(), "animationConfig", value);
}
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
public void assignParentResizable(Object parentResizable) {
getPolymerElement().assignParentResizable(parentResizable);
}
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
public void stopResizeNotificationsFor(Object target) {
getPolymerElement().stopResizeNotificationsFor(target);
}
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
public void notifyResize() {
getPolymerElement().notifyResize();
}
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
public boolean resizerShouldNotify(JavaScriptObject element) {
return getPolymerElement().resizerShouldNotify(element);
}
}