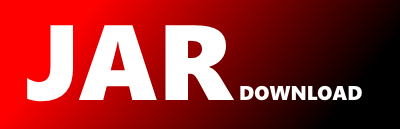
com.vaadin.polymer.paper.PaperBadgeElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-badge project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* <paper-badge>
is a circular text badge that is displayed on the top right
corner of an element, representing a status or a notification. It will badge
the anchor element specified in the for
attribute, or, if that doesn’t exist,
centered to the parent node containing it.
* Badges can also contain an icon by adding the icon
attribute and setting
it to the id of the desired icon. Please note that you should still set the
label
attribute in order to keep the element accessible. Also note that you will need to import
the iron-iconset
that includes the icons you want to use. See iron-icon
for more information on how to import and use icon sets.
* Example:
* <div style="display:inline-block">
* <span>Inbox</span>
* <paper-badge label="3"></paper-badge>
* </div>
*
* <div>
* <paper-button id="btn">Status</paper-button>
* <paper-badge icon="favorite" for="btn" label="favorite icon"></paper-badge>
* </div>
*
* <div>
* <paper-icon-button id="account-box" icon="account-box" alt="account-box"></paper-icon-button>
* <paper-badge icon="social:mood" for="account-box" label="mood icon"></paper-badge>
* </div>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-badge-background
* The background color of the badge
* --accent-color
*
*
* --paper-badge-opacity
* The opacity of the badge
* 1.0
*
*
* --paper-badge-text-color
* The color of the badge text
* white
*
*
* --paper-badge-width
* The width of the badge circle
* 20px
*
*
* --paper-badge-height
* The height of the badge circle
* 20px
*
*
* --paper-badge-margin-left
* Optional spacing added to the left of the badge.
* 0px
*
*
* --paper-badge-margin-bottom
* Optional spacing added to the bottom of the badge.
* 0px
*
*
* --paper-badge
* Mixin applied to the badge
* {}
*
*
*
*/
@JsType(isNative=true)
public interface PaperBadgeElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-badge";
@JsOverlay public static final String SRC = "paper-badge/paper-badge.html";
/**
* An iron-icon ID. When given, the badge content will use an
<iron-icon>
element displaying the given icon ID rather than the
label text. However, the label text will still be used for
accessibility purposes.
*
* JavaScript Info:
* @property icon
* @type String
*
*/
@JsProperty String getIcon();
/**
* An iron-icon ID. When given, the badge content will use an
<iron-icon>
element displaying the given icon ID rather than the
label text. However, the label text will still be used for
accessibility purposes.
*
* JavaScript Info:
* @property icon
* @type String
*
*/
@JsProperty void setIcon(String value);
/**
* The label displayed in the badge. The label is centered, and ideally
should have very few characters.
*
* JavaScript Info:
* @property label
* @type String
*
*/
@JsProperty String getLabel();
/**
* The label displayed in the badge. The label is centered, and ideally
should have very few characters.
*
* JavaScript Info:
* @property label
* @type String
*
*/
@JsProperty void setLabel(String value);
/**
* The id of the element that the badge is anchored to. This element
must be a sibling of the badge.
*
* JavaScript Info:
* @property for
* @type String
*
*/
@JsProperty String getFor();
/**
* The id of the element that the badge is anchored to. This element
must be a sibling of the badge.
*
* JavaScript Info:
* @property for
* @type String
*
*/
@JsProperty void setFor(String value);
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
void stopResizeNotificationsFor(Object target);
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
void assignParentResizable(Object parentResizable);
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
void notifyResize();
/**
* Repositions the badge relative to its anchor element. This is called
automatically when the badge is attached or an iron-resize
event is
fired (for exmaple if the window has resized, or your target is a
custom element that implements IronResizableBehavior).
* You should call this in all other cases when the achor’s position
might have changed (for example, if it’s visibility has changed, or
you’ve manually done a page re-layout).
*
* JavaScript Info:
* @method updatePosition
*
*
*/
void updatePosition();
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
boolean resizerShouldNotify(JavaScriptObject element);
}