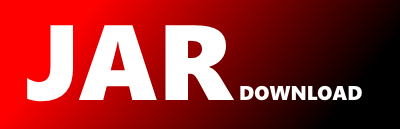
com.vaadin.polymer.paper.PaperDialogElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-dialog project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Material design: Dialogs
* <paper-dialog>
is a dialog with Material Design styling and optional animations when it is
opened or closed. It provides styles for a header, content area, and an action area for buttons.
You can use the <paper-dialog-scrollable>
element (in its own repository) if you need a scrolling
content area. To autofocus a specific child element after opening the dialog, give it the autofocus
attribute. See Polymer.PaperDialogBehavior
and Polymer.IronOverlayBehavior
for specifics.
* For example, the following code implements a dialog with a header, scrolling content area and
buttons. Focus will be given to the dialog-confirm
button when the dialog is opened.
* <paper-dialog>
* <h2>Header</h2>
* <paper-dialog-scrollable>
* Lorem ipsum...
* </paper-dialog-scrollable>
* <div class="buttons">
* <paper-button dialog-dismiss>Cancel</paper-button>
* <paper-button dialog-confirm autofocus>Accept</paper-button>
* </div>
* </paper-dialog>
*
*
*
Styling
* See the docs for Polymer.PaperDialogBehavior
for the custom properties available for styling
this element.
* Animations
* Set the entry-animation
and/or exit-animation
attributes to add an animation when the dialog
is opened or closed. See the documentation in
PolymerElements/neon-animation for more info.
* For example:
* <link rel="import" href="components/neon-animation/animations/scale-up-animation.html">
* <link rel="import" href="components/neon-animation/animations/fade-out-animation.html">
*
* <paper-dialog entry-animation="scale-up-animation"
* exit-animation="fade-out-animation">
* <h2>Header</h2>
* <div>Dialog body</div>
* </paper-dialog>
*
*
*
Accessibility
* See the docs for Polymer.PaperDialogBehavior
for accessibility features implemented by this
element.
*/
@JsType(isNative=true)
public interface PaperDialogElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-dialog";
@JsOverlay public static final String SRC = "paper-dialog/paper-dialog.html";
/**
* The backdrop element.
*
* JavaScript Info:
* @property backdropElement
* @type Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty Element getBackdropElement();
/**
* The backdrop element.
*
* JavaScript Info:
* @property backdropElement
* @type Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setBackdropElement(Element value);
/**
* True if the overlay was canceled when it was last closed.
*
* JavaScript Info:
* @property canceled
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getCanceled();
/**
* True if the overlay was canceled when it was last closed.
*
* JavaScript Info:
* @property canceled
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setCanceled(boolean value);
/**
* Set to true to disable auto-focusing the overlay or child nodes with
the autofocus
attribute` when the overlay is opened.
*
* JavaScript Info:
* @property noAutoFocus
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getNoAutoFocus();
/**
* Set to true to disable auto-focusing the overlay or child nodes with
the autofocus
attribute` when the overlay is opened.
*
* JavaScript Info:
* @property noAutoFocus
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setNoAutoFocus(boolean value);
/**
* Set to true to disable canceling the overlay with the ESC key.
*
* JavaScript Info:
* @property noCancelOnEscKey
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getNoCancelOnEscKey();
/**
* Set to true to disable canceling the overlay with the ESC key.
*
* JavaScript Info:
* @property noCancelOnEscKey
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setNoCancelOnEscKey(boolean value);
/**
* Set to true to disable canceling the overlay by clicking outside it.
*
* JavaScript Info:
* @property noCancelOnOutsideClick
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getNoCancelOnOutsideClick();
/**
* Set to true to disable canceling the overlay by clicking outside it.
*
* JavaScript Info:
* @property noCancelOnOutsideClick
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setNoCancelOnOutsideClick(boolean value);
/**
* True if the overlay is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getOpened();
/**
* True if the overlay is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setOpened(boolean value);
/**
* Set to true to enable restoring of focus when overlay is closed.
*
* JavaScript Info:
* @property restoreFocusOnClose
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getRestoreFocusOnClose();
/**
* Set to true to enable restoring of focus when overlay is closed.
*
* JavaScript Info:
* @property restoreFocusOnClose
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setRestoreFocusOnClose(boolean value);
/**
* Set to true to display a backdrop behind the overlay. It traps the focus
within the light DOM of the overlay.
*
* JavaScript Info:
* @property withBackdrop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getWithBackdrop();
/**
* Set to true to display a backdrop behind the overlay. It traps the focus
within the light DOM of the overlay.
*
* JavaScript Info:
* @property withBackdrop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setWithBackdrop(boolean value);
/**
* Set to true to auto-fit on attach.
*
* JavaScript Info:
* @property autoFitOnAttach
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getAutoFitOnAttach();
/**
* Set to true to auto-fit on attach.
*
* JavaScript Info:
* @property autoFitOnAttach
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setAutoFitOnAttach(boolean value);
/**
* Set to true to keep overlay always on top.
*
* JavaScript Info:
* @property alwaysOnTop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getAlwaysOnTop();
/**
* Set to true to keep overlay always on top.
*
* JavaScript Info:
* @property alwaysOnTop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setAlwaysOnTop(boolean value);
/**
* Will position the element around the positionTarget without overlapping it.
*
* JavaScript Info:
* @property noOverlap
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getNoOverlap();
/**
* Will position the element around the positionTarget without overlapping it.
*
* JavaScript Info:
* @property noOverlap
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setNoOverlap(boolean value);
/**
* If modal
is true, this implies no-cancel-on-outside-click
, no-cancel-on-esc-key
and with-backdrop
.
*
* JavaScript Info:
* @property modal
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getModal();
/**
* If modal
is true, this implies no-cancel-on-outside-click
, no-cancel-on-esc-key
and with-backdrop
.
*
* JavaScript Info:
* @property modal
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setModal(boolean value);
/**
* If true, it will use horizontalAlign
and verticalAlign
values as preferred alignment
and if there’s not enough space, it will pick the values which minimize the cropping.
*
* JavaScript Info:
* @property dynamicAlign
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty boolean getDynamicAlign();
/**
* If true, it will use horizontalAlign
and verticalAlign
values as preferred alignment
and if there’s not enough space, it will pick the values which minimize the cropping.
*
* JavaScript Info:
* @property dynamicAlign
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setDynamicAlign(boolean value);
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
* @behavior PaperTooltip
*/
@JsProperty JavaScriptObject getAnimationConfig();
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
* @behavior PaperTooltip
*/
@JsProperty void setAnimationConfig(JavaScriptObject value);
/**
* The element to fit this
into.
*
* JavaScript Info:
* @property fitInto
* @type Object
* @behavior VaadinContextMenuOverlay
*/
@JsProperty JavaScriptObject getFitInto();
/**
* The element to fit this
into.
*
* JavaScript Info:
* @property fitInto
* @type Object
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setFitInto(JavaScriptObject value);
/**
* Contains the reason(s) this overlay was last closed (see iron-overlay-closed
).
IronOverlayBehavior
provides the canceled
reason; implementers of the
behavior can provide other reasons in addition to canceled
.
*
* JavaScript Info:
* @property closingReason
* @type Object
* @behavior VaadinContextMenuOverlay
*/
@JsProperty JavaScriptObject getClosingReason();
/**
* Contains the reason(s) this overlay was last closed (see iron-overlay-closed
).
IronOverlayBehavior
provides the canceled
reason; implementers of the
behavior can provide other reasons in addition to canceled
.
*
* JavaScript Info:
* @property closingReason
* @type Object
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setClosingReason(JavaScriptObject value);
/**
* The element that should be used to position the element. If not set, it will
default to the parent node.
*
* JavaScript Info:
* @property positionTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty JavaScriptObject getPositionTarget();
/**
* The element that should be used to position the element. If not set, it will
default to the parent node.
*
* JavaScript Info:
* @property positionTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setPositionTarget(JavaScriptObject value);
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @property sizingTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty JavaScriptObject getSizingTarget();
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @property sizingTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setSizingTarget(JavaScriptObject value);
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by horizontalAlign
.
* If horizontalAlign
is “left”, this offset will increase or decrease
the distance to the left side of the screen: a negative offset will
move the dropdown to the left; a positive one, to the right.
* Conversely if horizontalAlign
is “right”, this offset will increase
or decrease the distance to the right side of the screen: a negative
offset will move the dropdown to the right; a positive one, to the left.
*
* JavaScript Info:
* @property horizontalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
@JsProperty double getHorizontalOffset();
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by horizontalAlign
.
* If horizontalAlign
is “left”, this offset will increase or decrease
the distance to the left side of the screen: a negative offset will
move the dropdown to the left; a positive one, to the right.
* Conversely if horizontalAlign
is “right”, this offset will increase
or decrease the distance to the right side of the screen: a negative
offset will move the dropdown to the right; a positive one, to the left.
*
* JavaScript Info:
* @property horizontalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setHorizontalOffset(double value);
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by verticalAlign
.
* If verticalAlign
is “top”, this offset will increase or decrease
the distance to the top side of the screen: a negative offset will
move the dropdown upwards; a positive one, downwards.
* Conversely if verticalAlign
is “bottom”, this offset will increase
or decrease the distance to the bottom side of the screen: a negative
offset will move the dropdown downwards; a positive one, upwards.
*
* JavaScript Info:
* @property verticalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
@JsProperty double getVerticalOffset();
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by verticalAlign
.
* If verticalAlign
is “top”, this offset will increase or decrease
the distance to the top side of the screen: a negative offset will
move the dropdown upwards; a positive one, downwards.
* Conversely if verticalAlign
is “bottom”, this offset will increase
or decrease the distance to the bottom side of the screen: a negative
offset will move the dropdown downwards; a positive one, upwards.
*
* JavaScript Info:
* @property verticalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setVerticalOffset(double value);
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior PaperTooltip
*/
@JsProperty String getExitAnimation();
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior PaperTooltip
*/
@JsProperty void setExitAnimation(String value);
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior PaperTooltip
*/
@JsProperty String getEntryAnimation();
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior PaperTooltip
*/
@JsProperty void setEntryAnimation(String value);
/**
* The orientation against which to align the element vertically
relative to the positionTarget
. Possible values are “top”, “bottom”, “auto”.
*
* JavaScript Info:
* @property verticalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
@JsProperty String getVerticalAlign();
/**
* The orientation against which to align the element vertically
relative to the positionTarget
. Possible values are “top”, “bottom”, “auto”.
*
* JavaScript Info:
* @property verticalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setVerticalAlign(String value);
/**
* The orientation against which to align the element horizontally
relative to the positionTarget
. Possible values are “left”, “right”, “auto”.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
@JsProperty String getHorizontalAlign();
/**
* The orientation against which to align the element horizontally
relative to the positionTarget
. Possible values are “left”, “right”, “auto”.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
@JsProperty void setHorizontalAlign(String value);
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
void assignParentResizable(Object parentResizable);
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
void stopResizeNotificationsFor(Object target);
/**
* Open the overlay.
*
* JavaScript Info:
* @method open
* @behavior VaadinContextMenuOverlay
*
*/
void open();
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
void notifyResize();
/**
* Centers horizontally and vertically if not already positioned. This also sets
position:fixed
.
*
* JavaScript Info:
* @method center
* @behavior VaadinContextMenuOverlay
*
*/
void center();
/**
* Constrains the size of the element to fitInto
by setting max-height
and/or max-width
.
*
* JavaScript Info:
* @method constrain
* @behavior VaadinContextMenuOverlay
*
*/
void constrain();
/**
* Invalidates the cached tabbable nodes. To be called when any of the focusable
content changes (e.g. a button is disabled).
*
* JavaScript Info:
* @method invalidateTabbables
* @behavior VaadinContextMenuOverlay
*
*/
void invalidateTabbables();
/**
* Positions and fits the element into the fitInto
element.
*
* JavaScript Info:
* @method fit
* @behavior VaadinContextMenuOverlay
*
*/
void fit();
/**
* Close the overlay.
*
* JavaScript Info:
* @method close
* @behavior VaadinContextMenuOverlay
*
*/
void close();
/**
* Toggle the opened state of the overlay.
*
* JavaScript Info:
* @method toggle
* @behavior VaadinContextMenuOverlay
*
*/
void toggle();
/**
* Positions the element according to horizontalAlign, verticalAlign
.
*
* JavaScript Info:
* @method position
* @behavior VaadinContextMenuOverlay
*
*/
void position();
/**
* Equivalent to calling resetFit()
and fit()
. Useful to call this after
the element or the fitInto
element has been resized, or if any of the
positioning properties (e.g. horizontalAlign, verticalAlign
) is updated.
It preserves the scroll position of the sizingTarget.
*
* JavaScript Info:
* @method refit
* @behavior VaadinContextMenuOverlay
*
*/
void refit();
/**
* Resets the target element’s position and size constraints, and clear
the memoized data.
*
* JavaScript Info:
* @method resetFit
* @behavior VaadinContextMenuOverlay
*
*/
void resetFit();
/**
* Cancels the currently running animations.
*
* JavaScript Info:
* @method cancelAnimation
* @behavior PaperTooltip
*
*/
void cancelAnimation();
/**
* Plays an animation with an optional type
.
*
* JavaScript Info:
* @method playAnimation
* @param {string=} type
* @param {!Object=} cookie
* @behavior PaperTooltip
*
*/
void playAnimation(String type, JavaScriptObject cookie);
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
boolean resizerShouldNotify(JavaScriptObject element);
/**
* Cancels the overlay.
*
* JavaScript Info:
* @method cancel
* @param {Event=} event
* @behavior VaadinContextMenuOverlay
*
*/
void cancel(JavaScriptObject event);
}