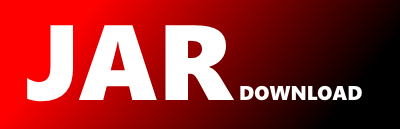
com.vaadin.polymer.paper.PaperDrawerPanelElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-drawer-panel project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Material design: Navigation drawer
* paper-drawer-panel
contains a drawer panel and a main panel. The drawer
and the main panel are side-by-side with drawer on the left. When the browser
window size is smaller than the responsiveWidth
, paper-drawer-panel
changes to narrow layout. In narrow layout, the drawer will be stacked on top
of the main panel. The drawer will slide in/out to hide/reveal the main
panel.
* Use the attribute drawer
to indicate that the element is the drawer panel and
main
to indicate that the element is the main panel.
* Example:
* <paper-drawer-panel>
* <div drawer> Drawer panel... </div>
* <div main> Main panel... </div>
* </paper-drawer-panel>
*
*
*
The drawer and the main panels are not scrollable. You can set CSS overflow
property on the elements to make them scrollable or use paper-header-panel
.
* Example:
* <paper-drawer-panel>
* <paper-header-panel drawer>
* <paper-toolbar></paper-toolbar>
* <div> Drawer content... </div>
* </paper-header-panel>
* <paper-header-panel main>
* <paper-toolbar></paper-toolbar>
* <div> Main content... </div>
* </paper-header-panel>
* </paper-drawer-panel>
*
*
*
An element that should toggle the drawer will automatically do so if it’s
given the paper-drawer-toggle
attribute. Also this element will automatically
be hidden in wide layout.
* Example:
* <paper-drawer-panel>
* <paper-header-panel drawer>
* <paper-toolbar>
* <div>Application</div>
* </paper-toolbar>
* <div> Drawer content... </div>
* </paper-header-panel>
* <paper-header-panel main>
* <paper-toolbar>
* <paper-icon-button icon="menu" paper-drawer-toggle></paper-icon-button>
* <div>Title</div>
* </paper-toolbar>
* <div> Main content... </div>
* </paper-header-panel>
* </paper-drawer-panel>
*
*
*
To position the drawer to the right, add right-drawer
attribute.
* <paper-drawer-panel right-drawer>
* <div drawer> Drawer panel... </div>
* <div main> Main panel... </div>
* </paper-drawer-panel>
*
*
*
Styling
* To change the main container:
* paper-drawer-panel {
* --paper-drawer-panel-main-container: {
* background-color: gray;
* };
* }
*
*
*
To change the drawer container when it’s in the left side:
* paper-drawer-panel {
* --paper-drawer-panel-left-drawer-container: {
* background-color: white;
* };
* }
*
*
*
To change the drawer container when it’s in the right side:
* paper-drawer-panel {
* --paper-drawer-panel-right-drawer-container: {
* background-color: white;
* };
* }
*
*
*
To customize the scrim:
* paper-drawer-panel {
* --paper-drawer-panel-scrim: {
* background-color: red;
* };
* }
*
*
*
The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-drawer-panel-scrim-opacity
* Scrim opacity
* 1
*
*
* --paper-drawer-panel-drawer-container
* Mixin applied to drawer container
* {}
*
*
* --paper-drawer-panel-left-drawer-container
* Mixin applied to container when it’s in the left side
* {}
*
*
* --paper-drawer-panel-main-container
* Mixin applied to main container
* {}
*
*
* --paper-drawer-panel-right-drawer-container
* Mixin applied to container when it’s in the right side
* {}
*
*
* --paper-drawer-panel-scrim
* Mixin applied to scrim
* {}
*
*
*
*/
@JsType(isNative=true)
public interface PaperDrawerPanelElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-drawer-panel";
@JsOverlay public static final String SRC = "paper-drawer-panel/paper-drawer-panel.html";
/**
* Whether the user is dragging the drawer interactively.
*
* JavaScript Info:
* @property dragging
* @type Boolean
*
*/
@JsProperty boolean getDragging();
/**
* Whether the user is dragging the drawer interactively.
*
* JavaScript Info:
* @property dragging
* @type Boolean
*
*/
@JsProperty void setDragging(boolean value);
/**
* How many pixels on the side of the screen are sensitive to edge
swipes and peek.
*
* JavaScript Info:
* @property edgeSwipeSensitivity
* @type Number
*
*/
@JsProperty double getEdgeSwipeSensitivity();
/**
* How many pixels on the side of the screen are sensitive to edge
swipes and peek.
*
* JavaScript Info:
* @property edgeSwipeSensitivity
* @type Number
*
*/
@JsProperty void setEdgeSwipeSensitivity(double value);
/**
* If true, ignore responsiveWidth
setting and force the narrow layout.
*
* JavaScript Info:
* @property forceNarrow
* @type Boolean
*
*/
@JsProperty boolean getForceNarrow();
/**
* If true, ignore responsiveWidth
setting and force the narrow layout.
*
* JavaScript Info:
* @property forceNarrow
* @type Boolean
*
*/
@JsProperty void setForceNarrow(boolean value);
/**
* If true, swipe to open/close the drawer is disabled.
*
* JavaScript Info:
* @property disableSwipe
* @type Boolean
*
*/
@JsProperty boolean getDisableSwipe();
/**
* If true, swipe to open/close the drawer is disabled.
*
* JavaScript Info:
* @property disableSwipe
* @type Boolean
*
*/
@JsProperty void setDisableSwipe(boolean value);
/**
* Whether the browser has support for the will-change CSS property.
*
* JavaScript Info:
* @property hasWillChange
* @type Boolean
*
*/
@JsProperty boolean getHasWillChange();
/**
* Whether the browser has support for the will-change CSS property.
*
* JavaScript Info:
* @property hasWillChange
* @type Boolean
*
*/
@JsProperty void setHasWillChange(boolean value);
/**
* Returns true if the panel is in narrow layout. This is useful if you
need to show/hide elements based on the layout.
*
* JavaScript Info:
* @property narrow
* @type Boolean
*
*/
@JsProperty boolean getNarrow();
/**
* Returns true if the panel is in narrow layout. This is useful if you
need to show/hide elements based on the layout.
*
* JavaScript Info:
* @property narrow
* @type Boolean
*
*/
@JsProperty void setNarrow(boolean value);
/**
* Whether the drawer is peeking out from the edge.
*
* JavaScript Info:
* @property peeking
* @type Boolean
*
*/
@JsProperty boolean getPeeking();
/**
* Whether the drawer is peeking out from the edge.
*
* JavaScript Info:
* @property peeking
* @type Boolean
*
*/
@JsProperty void setPeeking(boolean value);
/**
* If true, position the drawer to the right.
*
* JavaScript Info:
* @property rightDrawer
* @type Boolean
*
*/
@JsProperty boolean getRightDrawer();
/**
* If true, position the drawer to the right.
*
* JavaScript Info:
* @property rightDrawer
* @type Boolean
*
*/
@JsProperty void setRightDrawer(boolean value);
/**
* The panel that is being selected. drawer
for the drawer panel and
main
for the main panel.
*
* JavaScript Info:
* @property selected
* @type (string|null)
*
*/
@JsProperty Object getSelected();
/**
* The panel that is being selected. drawer
for the drawer panel and
main
for the main panel.
*
* JavaScript Info:
* @property selected
* @type (string|null)
*
*/
@JsProperty void setSelected(Object value);
/**
* If true, swipe from the edge is disabled.
*
* JavaScript Info:
* @property disableEdgeSwipe
* @type Boolean
*
*/
@JsProperty boolean getDisableEdgeSwipe();
/**
* If true, swipe from the edge is disabled.
*
* JavaScript Info:
* @property disableEdgeSwipe
* @type Boolean
*
*/
@JsProperty void setDisableEdgeSwipe(boolean value);
/**
* Whether the browser has support for the transform CSS property.
*
* JavaScript Info:
* @property hasTransform
* @type Boolean
*
*/
@JsProperty boolean getHasTransform();
/**
* Whether the browser has support for the transform CSS property.
*
* JavaScript Info:
* @property hasTransform
* @type Boolean
*
*/
@JsProperty void setHasTransform(boolean value);
/**
* Max-width when the panel changes to narrow layout.
*
* JavaScript Info:
* @property responsiveWidth
* @type String
*
*/
@JsProperty String getResponsiveWidth();
/**
* Max-width when the panel changes to narrow layout.
*
* JavaScript Info:
* @property responsiveWidth
* @type String
*
*/
@JsProperty void setResponsiveWidth(String value);
/**
* The panel to be selected when paper-drawer-panel
changes to narrow
layout.
*
* JavaScript Info:
* @property defaultSelected
* @type String
*
*/
@JsProperty String getDefaultSelected();
/**
* The panel to be selected when paper-drawer-panel
changes to narrow
layout.
*
* JavaScript Info:
* @property defaultSelected
* @type String
*
*/
@JsProperty void setDefaultSelected(String value);
/**
* Width of the drawer panel.
*
* JavaScript Info:
* @property drawerWidth
* @type String
*
*/
@JsProperty String getDrawerWidth();
/**
* Width of the drawer panel.
*
* JavaScript Info:
* @property drawerWidth
* @type String
*
*/
@JsProperty void setDrawerWidth(String value);
/**
* The attribute on elements that should toggle the drawer on tap, also elements will
automatically be hidden in wide layout.
*
* JavaScript Info:
* @property drawerToggleAttribute
* @type String
*
*/
@JsProperty String getDrawerToggleAttribute();
/**
* The attribute on elements that should toggle the drawer on tap, also elements will
automatically be hidden in wide layout.
*
* JavaScript Info:
* @property drawerToggleAttribute
* @type String
*
*/
@JsProperty void setDrawerToggleAttribute(String value);
/**
* The CSS selector for the element that should receive focus when the drawer is open.
By default, when the drawer opens, it focuses the first tabbable element. That is,
the first element that can receive focus.
* To disable this behavior, you can set drawerFocusSelector
to null
or an empty string.
*
* JavaScript Info:
* @property drawerFocusSelector
* @type String
*
*/
@JsProperty String getDrawerFocusSelector();
/**
* The CSS selector for the element that should receive focus when the drawer is open.
By default, when the drawer opens, it focuses the first tabbable element. That is,
the first element that can receive focus.
* To disable this behavior, you can set drawerFocusSelector
to null
or an empty string.
*
* JavaScript Info:
* @property drawerFocusSelector
* @type String
*
*/
@JsProperty void setDrawerFocusSelector(String value);
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
void stopResizeNotificationsFor(Object target);
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
void assignParentResizable(Object parentResizable);
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
boolean resizerShouldNotify(JavaScriptObject element);
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
void notifyResize();
/**
* Opens the drawer.
*
* JavaScript Info:
* @method openDrawer
*
*
*/
void openDrawer();
/**
* Toggles the panel open and closed.
*
* JavaScript Info:
* @method togglePanel
*
*
*/
void togglePanel();
/**
* Closes the drawer.
*
* JavaScript Info:
* @method closeDrawer
*
*
*/
void closeDrawer();
}