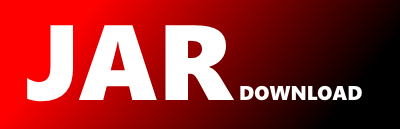
com.vaadin.polymer.paper.PaperDropdownMenuElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-dropdown-menu project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Material design: Dropdown menus
* paper-dropdown-menu
is similar to a native browser select element.
paper-dropdown-menu
works with selectable content. The currently selected
item is displayed in the control. If no item is selected, the label
is
displayed instead.
* Example:
* <paper-dropdown-menu label="Your favourite pastry">
* <paper-listbox class="dropdown-content">
* <paper-item>Croissant</paper-item>
* <paper-item>Donut</paper-item>
* <paper-item>Financier</paper-item>
* <paper-item>Madeleine</paper-item>
* </paper-listbox>
* </paper-dropdown-menu>
*
*
*
This example renders a dropdown menu with 4 options.
* The child element with the class dropdown-content
is used as the dropdown
menu. This can be a paper-listbox
, or any other or
element that acts like an iron-selector
.
* Specifically, the menu child must fire an
iron-select
event when one of its
children is selected, and an iron-deselect
event when a child is deselected. The selected or deselected item must
be passed as the event’s detail.item
property.
* Applications can listen for the iron-select
and iron-deselect
events
to react when options are selected and deselected.
* Styling
* The following custom properties and mixins are also available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-dropdown-menu
* A mixin that is applied to the element host
* {}
*
*
* --paper-dropdown-menu-disabled
* A mixin that is applied to the element host when disabled
* {}
*
*
* --paper-dropdown-menu-ripple
* A mixin that is applied to the internal ripple
* {}
*
*
* --paper-dropdown-menu-button
* A mixin that is applied to the internal menu button
* {}
*
*
* --paper-dropdown-menu-input
* A mixin that is applied to the internal paper input
* {}
*
*
* --paper-dropdown-menu-icon
* A mixin that is applied to the internal icon
* {}
*
*
*
* You can also use any of the paper-input-container
and paper-menu-button
style mixins and custom properties to style the internal input and menu button
respectively.
*/
@JsType(isNative=true)
public interface PaperDropdownMenuElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-dropdown-menu";
@JsOverlay public static final String SRC = "paper-dropdown-menu/paper-dropdown-menu.html";
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getPressed();
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setPressed(boolean value);
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
* The last selected item. An item is selected if the dropdown menu has
a child with class dropdown-content
, and that child triggers an
iron-select
event with the selected item
in the detail
.
*
* JavaScript Info:
* @property selectedItem
* @type ?Object
*
*/
@JsProperty JavaScriptObject getSelectedItem();
/**
* The last selected item. An item is selected if the dropdown menu has
a child with class dropdown-content
, and that child triggers an
iron-select
event with the selected item
in the detail
.
*
* JavaScript Info:
* @property selectedItem
* @type ?Object
*
*/
@JsProperty void setSelectedItem(JavaScriptObject value);
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getActive();
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setActive(boolean value);
/**
* True if the dropdown is open. Otherwise, false.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty boolean getOpened();
/**
* True if the dropdown is open. Otherwise, false.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty void setOpened(boolean value);
/**
* If true, the horizontalAlign
and verticalAlign
properties will
be considered preferences instead of strict requirements when
positioning the dropdown and may be changed if doing so reduces
the area of the dropdown falling outside of fitInto
.
*
* JavaScript Info:
* @property dynamicAlign
* @type Boolean
*
*/
@JsProperty boolean getDynamicAlign();
/**
* If true, the horizontalAlign
and verticalAlign
properties will
be considered preferences instead of strict requirements when
positioning the dropdown and may be changed if doing so reduces
the area of the dropdown falling outside of fitInto
.
*
* JavaScript Info:
* @property dynamicAlign
* @type Boolean
*
*/
@JsProperty void setDynamicAlign(boolean value);
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getPointerDown();
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setPointerDown(boolean value);
/**
* Set to true to always float the label. Bind this to the
<paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
*
*/
@JsProperty boolean getAlwaysFloatLabel();
/**
* Set to true to always float the label. Bind this to the
<paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
*
*/
@JsProperty void setAlwaysFloatLabel(boolean value);
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getReceivedFocusFromKeyboard();
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setReceivedFocusFromKeyboard(boolean value);
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getToggles();
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setToggles(boolean value);
/**
* Whether focus should be restored to the dropdown when the menu closes.
*
* JavaScript Info:
* @property restoreFocusOnClose
* @type Boolean
*
*/
@JsProperty boolean getRestoreFocusOnClose();
/**
* Whether focus should be restored to the dropdown when the menu closes.
*
* JavaScript Info:
* @property restoreFocusOnClose
* @type Boolean
*
*/
@JsProperty void setRestoreFocusOnClose(boolean value);
/**
* True if the last call to validate
is invalid.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getInvalid();
/**
* True if the last call to validate
is invalid.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setInvalid(boolean value);
/**
* Set to true to mark the input as required. If used in a form, a
custom element that uses this behavior should also use
Polymer.IronValidatableBehavior and define a custom validation method.
Otherwise, a required
element will always be considered valid.
It’s also strongly recommended to provide a visual style for the element
when its value is invalid.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getRequired();
/**
* Set to true to mark the input as required. If used in a form, a
custom element that uses this behavior should also use
Polymer.IronValidatableBehavior and define a custom validation method.
Otherwise, a required
element will always be considered valid.
It’s also strongly recommended to provide a visual style for the element
when its value is invalid.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setRequired(boolean value);
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getFocused();
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setFocused(boolean value);
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getDisabled();
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setDisabled(boolean value);
/**
* Set to true to disable animations when opening and closing the
dropdown.
*
* JavaScript Info:
* @property noAnimations
* @type Boolean
*
*/
@JsProperty boolean getNoAnimations();
/**
* Set to true to disable animations when opening and closing the
dropdown.
*
* JavaScript Info:
* @property noAnimations
* @type Boolean
*
*/
@JsProperty void setNoAnimations(boolean value);
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getStopKeyboardEventPropagation();
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setStopKeyboardEventPropagation(boolean value);
/**
* Set to true to disable the floating label. Bind this to the
<paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
*
*/
@JsProperty boolean getNoLabelFloat();
/**
* Set to true to disable the floating label. Bind this to the
<paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
*
*/
@JsProperty void setNoLabelFloat(boolean value);
/**
* By default, the dropdown will constrain scrolling on the page
to itself when opened.
Set to true in order to prevent scroll from being constrained
to the dropdown when it opens.
*
* JavaScript Info:
* @property allowOutsideScroll
* @type Boolean
*
*/
@JsProperty boolean getAllowOutsideScroll();
/**
* By default, the dropdown will constrain scrolling on the page
to itself when opened.
Set to true in order to prevent scroll from being constrained
to the dropdown when it opens.
*
* JavaScript Info:
* @property allowOutsideScroll
* @type Boolean
*
*/
@JsProperty void setAllowOutsideScroll(boolean value);
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
@JsProperty String getAriaActiveAttribute();
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
@JsProperty void setAriaActiveAttribute(String value);
/**
* The value for this element that will be used when submitting in
a form. It is read only, and will always have the same value
as selectedItemLabel
.
*
* JavaScript Info:
* @property value
* @type String
*
*/
@JsProperty String getValue();
/**
* The value for this element that will be used when submitting in
a form. It is read only, and will always have the same value
as selectedItemLabel
.
*
* JavaScript Info:
* @property value
* @type String
*
*/
@JsProperty void setValue(String value);
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty String getName();
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty void setName(String value);
/**
* The derived “label” of the currently selected item. This value
is the label
property on the selected item if set, or else the
trimmed text content of the selected item.
*
* JavaScript Info:
* @property selectedItemLabel
* @type String
*
*/
@JsProperty String getSelectedItemLabel();
/**
* The derived “label” of the currently selected item. This value
is the label
property on the selected item if set, or else the
trimmed text content of the selected item.
*
* JavaScript Info:
* @property selectedItemLabel
* @type String
*
*/
@JsProperty void setSelectedItemLabel(String value);
/**
* The placeholder for the dropdown.
*
* JavaScript Info:
* @property placeholder
* @type String
*
*/
@JsProperty String getPlaceholder();
/**
* The placeholder for the dropdown.
*
* JavaScript Info:
* @property placeholder
* @type String
*
*/
@JsProperty void setPlaceholder(String value);
/**
* The orientation against which to align the menu dropdown
vertically relative to the dropdown trigger.
*
* JavaScript Info:
* @property verticalAlign
* @type String
*
*/
@JsProperty String getVerticalAlign();
/**
* The orientation against which to align the menu dropdown
vertically relative to the dropdown trigger.
*
* JavaScript Info:
* @property verticalAlign
* @type String
*
*/
@JsProperty void setVerticalAlign(String value);
/**
* The orientation against which to align the menu dropdown
horizontally relative to the dropdown trigger.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
*
*/
@JsProperty String getHorizontalAlign();
/**
* The orientation against which to align the menu dropdown
horizontally relative to the dropdown trigger.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
*
*/
@JsProperty void setHorizontalAlign(String value);
/**
* The error message to display when invalid.
*
* JavaScript Info:
* @property errorMessage
* @type String
*
*/
@JsProperty String getErrorMessage();
/**
* The error message to display when invalid.
*
* JavaScript Info:
* @property errorMessage
* @type String
*
*/
@JsProperty void setErrorMessage(String value);
/**
* Namespace for this validator. This property is deprecated and should
not be used. For all intents and purposes, please consider it a
read-only, config-time property.
*
* JavaScript Info:
* @property validatorType
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty String getValidatorType();
/**
* Namespace for this validator. This property is deprecated and should
not be used. For all intents and purposes, please consider it a
read-only, config-time property.
*
* JavaScript Info:
* @property validatorType
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty void setValidatorType(String value);
/**
* Name of the validator to use.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty String getValidator();
/**
* Name of the validator to use.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty void setValidator(String value);
/**
* The label for the dropdown.
*
* JavaScript Info:
* @property label
* @type String
*
*/
@JsProperty String getLabel();
/**
* The label for the dropdown.
*
* JavaScript Info:
* @property label
* @type String
*
*/
@JsProperty void setLabel(String value);
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
void addOwnKeyBinding(Object eventString, Object handlerName);
/**
*
*
* JavaScript Info:
* @method hasValidator
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean hasValidator();
/**
* Hide the dropdown content.
*
* JavaScript Info:
* @method close
*
*
*/
void close();
/**
* Show the dropdown content.
*
* JavaScript Info:
* @method open
*
*
*/
void open();
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
void removeOwnKeyBindings();
/**
* Returns true if the value
is valid, and updates invalid
. If you want
your element to have custom validation logic, do not override this method;
override _getValidity(value)
instead.
*
* JavaScript Info:
* @method validate
* @param {Object} value
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean validate(JavaScriptObject value);
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString);
}