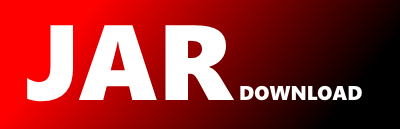
com.vaadin.polymer.paper.PaperDropdownMenuLightElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-dropdown-menu project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Material design: Dropdown menus
* This is a faster, lighter version of paper-dropdown-menu
, that does not
use a <paper-input>
internally. Use this element if you’re concerned about
the performance of this element, i.e., if you plan on using many dropdowns on
the same page. Note that this element has a slightly different styling API
than paper-dropdown-menu
.
* paper-dropdown-menu-light
is similar to a native browser select element.
paper-dropdown-menu-light
works with selectable content. The currently selected
item is displayed in the control. If no item is selected, the label
is
displayed instead.
* Example:
* <paper-dropdown-menu-light label="Your favourite pastry">
* <paper-listbox class="dropdown-content">
* <paper-item>Croissant</paper-item>
* <paper-item>Donut</paper-item>
* <paper-item>Financier</paper-item>
* <paper-item>Madeleine</paper-item>
* </paper-listbox>
* </paper-dropdown-menu-light>
*
*
*
This example renders a dropdown menu with 4 options.
* The child element with the class dropdown-content
is used as the dropdown
menu. This can be a paper-listbox
, or any other or
element that acts like an iron-selector
.
* Specifically, the menu child must fire an
iron-select
event when one of its
children is selected, and an iron-deselect
event when a child is deselected. The selected or deselected item must
be passed as the event’s detail.item
property.
* Applications can listen for the iron-select
and iron-deselect
events
to react when options are selected and deselected.
* Styling
* The following custom properties and mixins are also available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-dropdown-menu
* A mixin that is applied to the element host
* {}
*
*
* --paper-dropdown-menu-disabled
* A mixin that is applied to the element host when disabled
* {}
*
*
* --paper-dropdown-menu-ripple
* A mixin that is applied to the internal ripple
* {}
*
*
* --paper-dropdown-menu-button
* A mixin that is applied to the internal menu button
* {}
*
*
* --paper-dropdown-menu-icon
* A mixin that is applied to the internal icon
* {}
*
*
* --paper-dropdown-menu-disabled-opacity
* The opacity of the dropdown when disabled
* 0.33
*
*
* --paper-dropdown-menu-color
* The color of the input/label/underline when the dropdown is unfocused
* --primary-text-color
*
*
* --paper-dropdown-menu-focus-color
* The color of the label/underline when the dropdown is focused
* --primary-color
*
*
* --paper-dropdown-error-color
* The color of the label/underline when the dropdown is invalid
* --error-color
*
*
* --paper-dropdown-menu-label
* Mixin applied to the label
* {}
*
*
* --paper-dropdown-menu-input
* Mixin appled to the input
* {}
*
*
*
* Note that in this element, the underline is just the bottom border of the “input”.
To style it:
* <style is=custom-style>
* paper-dropdown-menu-light.custom {
* --paper-dropdown-menu-input: {
* border-bottom: 2px dashed lavender;
* };
* </style>
*
*
*
*/
@JsType(isNative=true)
public interface PaperDropdownMenuLightElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-dropdown-menu-light";
@JsOverlay public static final String SRC = "paper-dropdown-menu/paper-dropdown-menu-light.html";
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getActive();
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setActive(boolean value);
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
* The last selected item. An item is selected if the dropdown menu has
a child with class dropdown-content
, and that child triggers an
iron-select
event with the selected item
in the detail
.
*
* JavaScript Info:
* @property selectedItem
* @type ?Object
*
*/
@JsProperty JavaScriptObject getSelectedItem();
/**
* The last selected item. An item is selected if the dropdown menu has
a child with class dropdown-content
, and that child triggers an
iron-select
event with the selected item
in the detail
.
*
* JavaScript Info:
* @property selectedItem
* @type ?Object
*
*/
@JsProperty void setSelectedItem(JavaScriptObject value);
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
* Set to true to mark the input as required. If used in a form, a
custom element that uses this behavior should also use
Polymer.IronValidatableBehavior and define a custom validation method.
Otherwise, a required
element will always be considered valid.
It’s also strongly recommended to provide a visual style for the element
when its value is invalid.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getRequired();
/**
* Set to true to mark the input as required. If used in a form, a
custom element that uses this behavior should also use
Polymer.IronValidatableBehavior and define a custom validation method.
Otherwise, a required
element will always be considered valid.
It’s also strongly recommended to provide a visual style for the element
when its value is invalid.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setRequired(boolean value);
/**
* If true, the element will not produce a ripple effect when interacted
with via the pointer.
*
* JavaScript Info:
* @property noink
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getNoink();
/**
* If true, the element will not produce a ripple effect when interacted
with via the pointer.
*
* JavaScript Info:
* @property noink
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setNoink(boolean value);
/**
* By default, the dropdown will constrain scrolling on the page
to itself when opened.
Set to true in order to prevent scroll from being constrained
to the dropdown when it opens.
*
* JavaScript Info:
* @property allowOutsideScroll
* @type Boolean
*
*/
@JsProperty boolean getAllowOutsideScroll();
/**
* By default, the dropdown will constrain scrolling on the page
to itself when opened.
Set to true in order to prevent scroll from being constrained
to the dropdown when it opens.
*
* JavaScript Info:
* @property allowOutsideScroll
* @type Boolean
*
*/
@JsProperty void setAllowOutsideScroll(boolean value);
/**
* Set to true to disable animations when opening and closing the
dropdown.
*
* JavaScript Info:
* @property noAnimations
* @type Boolean
*
*/
@JsProperty boolean getNoAnimations();
/**
* Set to true to disable animations when opening and closing the
dropdown.
*
* JavaScript Info:
* @property noAnimations
* @type Boolean
*
*/
@JsProperty void setNoAnimations(boolean value);
/**
*
*
* JavaScript Info:
* @property hasContent
* @type Boolean
*
*/
@JsProperty boolean getHasContent();
/**
*
*
* JavaScript Info:
* @property hasContent
* @type Boolean
*
*/
@JsProperty void setHasContent(boolean value);
/**
* Set to true to disable the floating label. Bind this to the
<paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
*
*/
@JsProperty boolean getNoLabelFloat();
/**
* Set to true to disable the floating label. Bind this to the
<paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
*
*/
@JsProperty void setNoLabelFloat(boolean value);
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getPointerDown();
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setPointerDown(boolean value);
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getPressed();
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setPressed(boolean value);
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getReceivedFocusFromKeyboard();
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setReceivedFocusFromKeyboard(boolean value);
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getToggles();
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setToggles(boolean value);
/**
* Set to true to always float the label. Bind this to the
<paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
*
*/
@JsProperty boolean getAlwaysFloatLabel();
/**
* Set to true to always float the label. Bind this to the
<paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
*
*/
@JsProperty void setAlwaysFloatLabel(boolean value);
/**
* True if the dropdown is open. Otherwise, false.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty boolean getOpened();
/**
* True if the dropdown is open. Otherwise, false.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty void setOpened(boolean value);
/**
* True if the last call to validate
is invalid.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getInvalid();
/**
* True if the last call to validate
is invalid.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setInvalid(boolean value);
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getStopKeyboardEventPropagation();
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setStopKeyboardEventPropagation(boolean value);
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getDisabled();
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setDisabled(boolean value);
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getFocused();
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setFocused(boolean value);
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
@JsProperty String getAriaActiveAttribute();
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
@JsProperty void setAriaActiveAttribute(String value);
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty String getName();
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty void setName(String value);
/**
* The value for this element that will be used when submitting in
a form. It is read only, and will always have the same value
as selectedItemLabel
.
*
* JavaScript Info:
* @property value
* @type String
*
*/
@JsProperty String getValue();
/**
* The value for this element that will be used when submitting in
a form. It is read only, and will always have the same value
as selectedItemLabel
.
*
* JavaScript Info:
* @property value
* @type String
*
*/
@JsProperty void setValue(String value);
/**
* The derived “label” of the currently selected item. This value
is the label
property on the selected item if set, or else the
trimmed text content of the selected item.
*
* JavaScript Info:
* @property selectedItemLabel
* @type String
*
*/
@JsProperty String getSelectedItemLabel();
/**
* The derived “label” of the currently selected item. This value
is the label
property on the selected item if set, or else the
trimmed text content of the selected item.
*
* JavaScript Info:
* @property selectedItemLabel
* @type String
*
*/
@JsProperty void setSelectedItemLabel(String value);
/**
* The orientation against which to align the menu dropdown
vertically relative to the dropdown trigger.
*
* JavaScript Info:
* @property verticalAlign
* @type String
*
*/
@JsProperty String getVerticalAlign();
/**
* The orientation against which to align the menu dropdown
vertically relative to the dropdown trigger.
*
* JavaScript Info:
* @property verticalAlign
* @type String
*
*/
@JsProperty void setVerticalAlign(String value);
/**
* The label for the dropdown.
*
* JavaScript Info:
* @property label
* @type String
*
*/
@JsProperty String getLabel();
/**
* The label for the dropdown.
*
* JavaScript Info:
* @property label
* @type String
*
*/
@JsProperty void setLabel(String value);
/**
* The orientation against which to align the menu dropdown
horizontally relative to the dropdown trigger.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
*
*/
@JsProperty String getHorizontalAlign();
/**
* The orientation against which to align the menu dropdown
horizontally relative to the dropdown trigger.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
*
*/
@JsProperty void setHorizontalAlign(String value);
/**
* Namespace for this validator. This property is deprecated and should
not be used. For all intents and purposes, please consider it a
read-only, config-time property.
*
* JavaScript Info:
* @property validatorType
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty String getValidatorType();
/**
* Namespace for this validator. This property is deprecated and should
not be used. For all intents and purposes, please consider it a
read-only, config-time property.
*
* JavaScript Info:
* @property validatorType
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty void setValidatorType(String value);
/**
* Name of the validator to use.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty String getValidator();
/**
* Name of the validator to use.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty void setValidator(String value);
/**
* The placeholder for the dropdown.
*
* JavaScript Info:
* @property placeholder
* @type String
*
*/
@JsProperty String getPlaceholder();
/**
* The placeholder for the dropdown.
*
* JavaScript Info:
* @property placeholder
* @type String
*
*/
@JsProperty void setPlaceholder(String value);
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
void addOwnKeyBinding(Object eventString, Object handlerName);
/**
* Show the dropdown content.
*
* JavaScript Info:
* @method open
*
*
*/
void open();
/**
* Hide the dropdown content.
*
* JavaScript Info:
* @method close
*
*
*/
void close();
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
void removeOwnKeyBindings();
/**
* Returns true if this element currently contains a ripple effect.
*
* JavaScript Info:
* @method hasRipple
* @behavior PaperTab
* @return {boolean}
*/
boolean hasRipple();
/**
*
*
* JavaScript Info:
* @method hasValidator
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean hasValidator();
/**
* Returns the <paper-ripple>
element used by this element to create
ripple effects. The element’s ripple is created on demand, when
necessary, and calling this method will force the
ripple to be created.
*
* JavaScript Info:
* @method getRipple
* @behavior PaperTab
*
*/
void getRipple();
/**
* Returns true if the value
is valid, and updates invalid
. If you want
your element to have custom validation logic, do not override this method;
override _getValidity(value)
instead.
*
* JavaScript Info:
* @method validate
* @param {Object} value
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean validate(JavaScriptObject value);
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString);
/**
* Ensures this element contains a ripple effect. For startup efficiency
the ripple effect is dynamically on demand when needed.
*
* JavaScript Info:
* @method ensureRipple
* @param {!Event=} optTriggeringEvent
* @behavior PaperTab
*
*/
void ensureRipple(JavaScriptObject optTriggeringEvent);
}