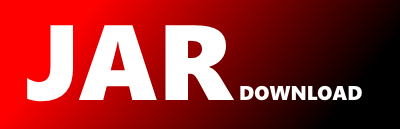
com.vaadin.polymer.paper.PaperIconItemElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-item project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* <paper-icon-item>
is a convenience element to make an item with icon. It is an interactive list
item with a fixed-width icon area, according to Material Design. This is useful if the icons are of
varying widths, but you want the item bodies to line up. Use this like a <paper-item>
. The child
node with the attribute item-icon
is placed in the icon area.
* <paper-icon-item>
* <iron-icon icon="favorite" item-icon></iron-icon>
* Favorite
* </paper-icon-item>
* <paper-icon-item>
* <div class="avatar" item-icon></div>
* Avatar
* </paper-icon-item>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-item-icon-width
* Width of the icon area
* 56px
*
*
* --paper-item-icon
* Mixin applied to the icon area
* {}
*
*
* --paper-icon-item
* Mixin applied to the item
* {}
*
*
* --paper-item-selected-weight
* The font weight of a selected item
* bold
*
*
* --paper-item-selected
* Mixin applied to selected paper-items
* {}
*
*
* --paper-item-disabled-color
* The color for disabled paper-items
* --disabled-text-color
*
*
* --paper-item-disabled
* Mixin applied to disabled paper-items
* {}
*
*
* --paper-item-focused
* Mixin applied to focused paper-items
* {}
*
*
* --paper-item-focused-before
* Mixin applied to :before focused paper-items
* {}
*
*
*
*/
@JsType(isNative=true)
public interface PaperIconItemElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-icon-item";
@JsOverlay public static final String SRC = "paper-item/all-imports.html";
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTab
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTab
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getFocused();
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setFocused(boolean value);
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getPointerDown();
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setPointerDown(boolean value);
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getPressed();
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setPressed(boolean value);
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getReceivedFocusFromKeyboard();
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setReceivedFocusFromKeyboard(boolean value);
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getStopKeyboardEventPropagation();
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setStopKeyboardEventPropagation(boolean value);
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getToggles();
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setToggles(boolean value);
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getActive();
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setActive(boolean value);
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getDisabled();
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setDisabled(boolean value);
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
@JsProperty String getAriaActiveAttribute();
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
@JsProperty void setAriaActiveAttribute(String value);
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
void addOwnKeyBinding(Object eventString, Object handlerName);
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
void removeOwnKeyBindings();
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString);
}