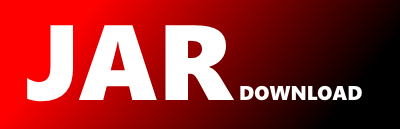
com.vaadin.polymer.paper.PaperInputBehavior Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-input project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Use Polymer.PaperInputBehavior
to implement inputs with <paper-input-container>
. This
behavior is implemented by <paper-input>
. It exposes a number of properties from
<paper-input-container>
and <input is="iron-input">
and they should be bound in your
template.
* The input element can be accessed by the inputElement
property if you need to access
properties or methods that are not exposed.
*/
@JsType(isNative=true)
public interface PaperInputBehavior {
@JsOverlay public static final String NAME = "Polymer.PaperInputBehavior";
@JsOverlay public static final String SRC = "paper-input/paper-textarea.html";
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
* Set to true to auto-validate the input value. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s autoValidate
property.
*
* JavaScript Info:
* @property autoValidate
* @type Boolean
*
*/
@JsProperty boolean getAutoValidate();
/**
* Set to true to auto-validate the input value. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s autoValidate
property.
*
* JavaScript Info:
* @property autoValidate
* @type Boolean
*
*/
@JsProperty void setAutoValidate(boolean value);
/**
* Set to true to show a character counter.
*
* JavaScript Info:
* @property charCounter
* @type Boolean
*
*/
@JsProperty boolean getCharCounter();
/**
* Set to true to show a character counter.
*
* JavaScript Info:
* @property charCounter
* @type Boolean
*
*/
@JsProperty void setCharCounter(boolean value);
/**
* Set to true to always float the label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
*
*/
@JsProperty boolean getAlwaysFloatLabel();
/**
* Set to true to always float the label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
*
*/
@JsProperty void setAlwaysFloatLabel(boolean value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the<input is="iron-input">
‘s multiple
property,
used with type=file.
*
* JavaScript Info:
* @property multiple
* @type Boolean
*
*/
@JsProperty boolean getMultiple();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the<input is="iron-input">
‘s multiple
property,
used with type=file.
*
* JavaScript Info:
* @property multiple
* @type Boolean
*
*/
@JsProperty void setMultiple(boolean value);
/**
* Returns true if the value is invalid. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to both the
<paper-input-container>
‘s and the input’s invalid
property.
* If autoValidate
is true, the invalid
attribute is managed automatically,
which can clobber attempts to manage it manually.
*
* JavaScript Info:
* @property invalid
* @type Boolean
*
*/
@JsProperty boolean getInvalid();
/**
* Returns true if the value is invalid. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to both the
<paper-input-container>
‘s and the input’s invalid
property.
* If autoValidate
is true, the invalid
attribute is managed automatically,
which can clobber attempts to manage it manually.
*
* JavaScript Info:
* @property invalid
* @type Boolean
*
*/
@JsProperty void setInvalid(boolean value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autofocus
property.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
*
*/
@JsProperty boolean getAutofocus();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autofocus
property.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
*
*/
@JsProperty void setAutofocus(boolean value);
/**
* Set to true to mark the input as required. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s required
property.
*
* JavaScript Info:
* @property required
* @type Boolean
*
*/
@JsProperty boolean getRequired();
/**
* Set to true to mark the input as required. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s required
property.
*
* JavaScript Info:
* @property required
* @type Boolean
*
*/
@JsProperty void setRequired(boolean value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s readonly
property.
*
* JavaScript Info:
* @property readonly
* @type Boolean
*
*/
@JsProperty boolean getReadonly();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s readonly
property.
*
* JavaScript Info:
* @property readonly
* @type Boolean
*
*/
@JsProperty void setReadonly(boolean value);
/**
* Set to true to prevent the user from entering invalid input. If you’re
using PaperInputBehavior to implement your own paper-input-like element,
bind this to <input is="iron-input">
‘s preventInvalidInput
property.
*
* JavaScript Info:
* @property preventInvalidInput
* @type Boolean
*
*/
@JsProperty boolean getPreventInvalidInput();
/**
* Set to true to prevent the user from entering invalid input. If you’re
using PaperInputBehavior to implement your own paper-input-like element,
bind this to <input is="iron-input">
‘s preventInvalidInput
property.
*
* JavaScript Info:
* @property preventInvalidInput
* @type Boolean
*
*/
@JsProperty void setPreventInvalidInput(boolean value);
/**
* Set to true to disable the floating label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
*
*/
@JsProperty boolean getNoLabelFloat();
/**
* Set to true to disable the floating label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
*
*/
@JsProperty void setNoLabelFloat(boolean value);
/**
* Set to true to disable this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
both the <paper-input-container>
‘s and the input’s disabled
property.
*
* JavaScript Info:
* @property disabled
* @type Boolean
*
*/
@JsProperty boolean getDisabled();
/**
* Set to true to disable this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
both the <paper-input-container>
‘s and the input’s disabled
property.
*
* JavaScript Info:
* @property disabled
* @type Boolean
*
*/
@JsProperty void setDisabled(boolean value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @property size
* @type Number
*
*/
@JsProperty double getSize();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @property size
* @type Number
*
*/
@JsProperty void setSize(double value);
/**
* The maximum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s maxlength
property.
*
* JavaScript Info:
* @property maxlength
* @type Number
*
*/
@JsProperty double getMaxlength();
/**
* The maximum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s maxlength
property.
*
* JavaScript Info:
* @property maxlength
* @type Number
*
*/
@JsProperty void setMaxlength(double value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s results
property,
used with type=search.
*
* JavaScript Info:
* @property results
* @type Number
*
*/
@JsProperty double getResults();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s results
property,
used with type=search.
*
* JavaScript Info:
* @property results
* @type Number
*
*/
@JsProperty void setResults(double value);
/**
* The minimum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @property minlength
* @type Number
*
*/
@JsProperty double getMinlength();
/**
* The minimum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @property minlength
* @type Number
*
*/
@JsProperty void setMinlength(double value);
/**
* Limits the numeric or date-time increments.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s step
property.
*
* JavaScript Info:
* @property step
* @type String
*
*/
@JsProperty String getStep();
/**
* Limits the numeric or date-time increments.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s step
property.
*
* JavaScript Info:
* @property step
* @type String
*
*/
@JsProperty void setStep(String value);
/**
* Set this to specify the pattern allowed by preventInvalidInput
. If
you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s allowedPattern
property.
*
* JavaScript Info:
* @property allowedPattern
* @type String
*
*/
@JsProperty String getAllowedPattern();
/**
* Set this to specify the pattern allowed by preventInvalidInput
. If
you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s allowedPattern
property.
*
* JavaScript Info:
* @property allowedPattern
* @type String
*
*/
@JsProperty void setAllowedPattern(String value);
/**
* The minimum (numeric or date-time) input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s min
property.
*
* JavaScript Info:
* @property min
* @type String
*
*/
@JsProperty String getMin();
/**
* The minimum (numeric or date-time) input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s min
property.
*
* JavaScript Info:
* @property min
* @type String
*
*/
@JsProperty void setMin(String value);
/**
* A pattern to validate the input
with. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s pattern
property.
*
* JavaScript Info:
* @property pattern
* @type String
*
*/
@JsProperty String getPattern();
/**
* A pattern to validate the input
with. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s pattern
property.
*
* JavaScript Info:
* @property pattern
* @type String
*
*/
@JsProperty void setPattern(String value);
/**
* A placeholder string in addition to the label. If this is set, the label will always float.
*
* JavaScript Info:
* @property placeholder
* @type String
*
*/
@JsProperty String getPlaceholder();
/**
* A placeholder string in addition to the label. If this is set, the label will always float.
*
* JavaScript Info:
* @property placeholder
* @type String
*
*/
@JsProperty void setPlaceholder(String value);
/**
* The maximum (numeric or date-time) input value.
Can be a String (e.g. "2000-01-01"
) or a Number (e.g. 2
).
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s max
property.
*
* JavaScript Info:
* @property max
* @type String
*
*/
@JsProperty String getMax();
/**
* The maximum (numeric or date-time) input value.
Can be a String (e.g. "2000-01-01"
) or a Number (e.g. 2
).
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s max
property.
*
* JavaScript Info:
* @property max
* @type String
*
*/
@JsProperty void setMax(String value);
/**
* The datalist of the input (if any). This should match the id of an existing <datalist>
.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s list
property.
*
* JavaScript Info:
* @property list
* @type String
*
*/
@JsProperty String getList();
/**
* The datalist of the input (if any). This should match the id of an existing <datalist>
.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s list
property.
*
* JavaScript Info:
* @property list
* @type String
*
*/
@JsProperty void setList(String value);
/**
* The label for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
<label>
‘s content and hidden
property, e.g.
<label hidden$="[[!label]]">[[label]]</label>
in your template
*
* JavaScript Info:
* @property label
* @type String
*
*/
@JsProperty String getLabel();
/**
* The label for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
<label>
‘s content and hidden
property, e.g.
<label hidden$="[[!label]]">[[label]]</label>
in your template
*
* JavaScript Info:
* @property label
* @type String
*
*/
@JsProperty void setLabel(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s inputmode
property.
*
* JavaScript Info:
* @property inputmode
* @type String
*
*/
@JsProperty String getInputmode();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s inputmode
property.
*
* JavaScript Info:
* @property inputmode
* @type String
*
*/
@JsProperty void setInputmode(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocapitalize
property.
*
* JavaScript Info:
* @property autocapitalize
* @type String
*
*/
@JsProperty String getAutocapitalize();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocapitalize
property.
*
* JavaScript Info:
* @property autocapitalize
* @type String
*
*/
@JsProperty void setAutocapitalize(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s name
property.
*
* JavaScript Info:
* @property name
* @type String
*
*/
@JsProperty String getName();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s name
property.
*
* JavaScript Info:
* @property name
* @type String
*
*/
@JsProperty void setName(String value);
/**
* The type of the input. The supported types are text
, number
and password
.
If you’re using PaperInputBehavior to implement your own paper-input-like element,
bind this to the <input is="iron-input">
‘s type
property.
*
* JavaScript Info:
* @property type
* @type String
*
*/
@JsProperty String getType();
/**
* The type of the input. The supported types are text
, number
and password
.
If you’re using PaperInputBehavior to implement your own paper-input-like element,
bind this to the <input is="iron-input">
‘s type
property.
*
* JavaScript Info:
* @property type
* @type String
*
*/
@JsProperty void setType(String value);
/**
* The error message to display when the input is invalid. If you’re using
PaperInputBehavior to implement your own paper-input-like element,
bind this to the <paper-input-error>
‘s content, if using.
*
* JavaScript Info:
* @property errorMessage
* @type String
*
*/
@JsProperty String getErrorMessage();
/**
* The error message to display when the input is invalid. If you’re using
PaperInputBehavior to implement your own paper-input-like element,
bind this to the <paper-input-error>
‘s content, if using.
*
* JavaScript Info:
* @property errorMessage
* @type String
*
*/
@JsProperty void setErrorMessage(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autosave
property,
used with type=search.
*
* JavaScript Info:
* @property autosave
* @type String
*
*/
@JsProperty String getAutosave();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autosave
property,
used with type=search.
*
* JavaScript Info:
* @property autosave
* @type String
*
*/
@JsProperty void setAutosave(String value);
/**
* Name of the validator to use. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s validator
property.
*
* JavaScript Info:
* @property validator
* @type String
*
*/
@JsProperty String getValidator();
/**
* Name of the validator to use. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s validator
property.
*
* JavaScript Info:
* @property validator
* @type String
*
*/
@JsProperty void setValidator(String value);
/**
* The value for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s bindValue
property, or the value property of your input that is notify:true
.
*
* JavaScript Info:
* @property value
* @type String
*
*/
@JsProperty String getValue();
/**
* The value for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s bindValue
property, or the value property of your input that is notify:true
.
*
* JavaScript Info:
* @property value
* @type String
*
*/
@JsProperty void setValue(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocorrect
property.
*
* JavaScript Info:
* @property autocorrect
* @type String
*
*/
@JsProperty String getAutocorrect();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocorrect
property.
*
* JavaScript Info:
* @property autocorrect
* @type String
*
*/
@JsProperty void setAutocorrect(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocomplete
property.
*
* JavaScript Info:
* @property autocomplete
* @type String
*
*/
@JsProperty String getAutocomplete();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocomplete
property.
*
* JavaScript Info:
* @property autocomplete
* @type String
*
*/
@JsProperty void setAutocomplete(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s accept
property,
used with type=file.
*
* JavaScript Info:
* @property accept
* @type String
*
*/
@JsProperty String getAccept();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s accept
property,
used with type=file.
*
* JavaScript Info:
* @property accept
* @type String
*
*/
@JsProperty void setAccept(String value);
/**
* Validates the input element and sets an error style if needed.
*
* JavaScript Info:
* @method validate
*
* @return {boolean}
*/
boolean validate();
/**
* Restores the cursor to its original position after updating the value.
*
* JavaScript Info:
* @method updateValueAndPreserveCaret
* @param {string} newValue
*
*
*/
void updateValueAndPreserveCaret(String newValue);
}