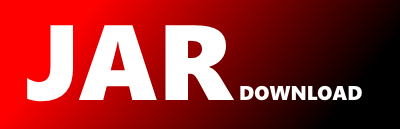
com.vaadin.polymer.paper.PaperInputElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-input project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Material design: Text fields
* <paper-input>
is a single-line text field with Material Design styling.
* <paper-input label="Input label"></paper-input>
*
*
*
It may include an optional error message or character counter.
* <paper-input error-message="Invalid input!" label="Input label"></paper-input>
* <paper-input char-counter label="Input label"></paper-input>
*
*
*
It can also include custom prefix or suffix elements, which are displayed
before or after the text input itself. In order for an element to be
considered as a prefix, it must have the prefix
attribute (and similarly
for suffix
).
* <paper-input label="total">
* <div prefix>$</div>
* <paper-icon-button suffix icon="clear"></paper-icon-button>
* </paper-input>
*
*
*
A paper-input
can use the native type=search
or type=file
features.
However, since we can’t control the native styling of the input (search icon,
file button, date placeholder, etc.), in these cases the label will be
automatically floated. The placeholder
attribute can still be used for
additional informational text.
* <paper-input label="search!" type="search"
* placeholder="search for cats" autosave="test" results="5">
* </paper-input>
*
*
*
See Polymer.PaperInputBehavior
for more API docs.
* Focus
* To focus a paper-input, you can call the native focus()
method as long as the
paper input has a tab index.
* Styling
* See Polymer.PaperInputContainer
for a list of custom properties used to
style this element.
*/
@JsType(isNative=true)
public interface PaperInputElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-input";
@JsOverlay public static final String SRC = "paper-input/paper-input.html";
/**
* The maximum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s maxlength
property.
*
* JavaScript Info:
* @property maxlength
* @type Number
*
*/
@JsProperty double getMaxlength();
/**
* The maximum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s maxlength
property.
*
* JavaScript Info:
* @property maxlength
* @type Number
*
*/
@JsProperty void setMaxlength(double value);
/**
* The minimum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @property minlength
* @type Number
*
*/
@JsProperty double getMinlength();
/**
* The minimum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @property minlength
* @type Number
*
*/
@JsProperty void setMinlength(double value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s results
property,
used with type=search.
*
* JavaScript Info:
* @property results
* @type Number
*
*/
@JsProperty double getResults();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s results
property,
used with type=search.
*
* JavaScript Info:
* @property results
* @type Number
*
*/
@JsProperty void setResults(double value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @property size
* @type Number
* @behavior PaperTextarea
*/
@JsProperty double getSize();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @property size
* @type Number
* @behavior PaperTextarea
*/
@JsProperty void setSize(double value);
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getFocused();
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setFocused(boolean value);
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getDisabled();
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setDisabled(boolean value);
/**
* Set to true to show a character counter.
*
* JavaScript Info:
* @property charCounter
* @type Boolean
*
*/
@JsProperty boolean getCharCounter();
/**
* Set to true to show a character counter.
*
* JavaScript Info:
* @property charCounter
* @type Boolean
*
*/
@JsProperty void setCharCounter(boolean value);
/**
* Set to true to auto-validate the input value. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s autoValidate
property.
*
* JavaScript Info:
* @property autoValidate
* @type Boolean
* @behavior PaperTextarea
*/
@JsProperty boolean getAutoValidate();
/**
* Set to true to auto-validate the input value. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s autoValidate
property.
*
* JavaScript Info:
* @property autoValidate
* @type Boolean
* @behavior PaperTextarea
*/
@JsProperty void setAutoValidate(boolean value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the<input is="iron-input">
‘s multiple
property,
used with type=file.
*
* JavaScript Info:
* @property multiple
* @type Boolean
*
*/
@JsProperty boolean getMultiple();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the<input is="iron-input">
‘s multiple
property,
used with type=file.
*
* JavaScript Info:
* @property multiple
* @type Boolean
*
*/
@JsProperty void setMultiple(boolean value);
/**
* Set to true to always float the label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
*
*/
@JsProperty boolean getAlwaysFloatLabel();
/**
* Set to true to always float the label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
*
*/
@JsProperty void setAlwaysFloatLabel(boolean value);
/**
* Set to true to disable the floating label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
*
*/
@JsProperty boolean getNoLabelFloat();
/**
* Set to true to disable the floating label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
*
*/
@JsProperty void setNoLabelFloat(boolean value);
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getStopKeyboardEventPropagation();
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setStopKeyboardEventPropagation(boolean value);
/**
* Returns true if the value is invalid. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to both the
<paper-input-container>
‘s and the input’s invalid
property.
* If autoValidate
is true, the invalid
attribute is managed automatically,
which can clobber attempts to manage it manually.
*
* JavaScript Info:
* @property invalid
* @type Boolean
*
*/
@JsProperty boolean getInvalid();
/**
* Returns true if the value is invalid. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to both the
<paper-input-container>
‘s and the input’s invalid
property.
* If autoValidate
is true, the invalid
attribute is managed automatically,
which can clobber attempts to manage it manually.
*
* JavaScript Info:
* @property invalid
* @type Boolean
*
*/
@JsProperty void setInvalid(boolean value);
/**
* Set to true to mark the input as required. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s required
property.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior PaperTextarea
*/
@JsProperty boolean getRequired();
/**
* Set to true to mark the input as required. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s required
property.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior PaperTextarea
*/
@JsProperty void setRequired(boolean value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s readonly
property.
*
* JavaScript Info:
* @property readonly
* @type Boolean
* @behavior PaperTextarea
*/
@JsProperty boolean getReadonly();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s readonly
property.
*
* JavaScript Info:
* @property readonly
* @type Boolean
* @behavior PaperTextarea
*/
@JsProperty void setReadonly(boolean value);
/**
* Set to true to prevent the user from entering invalid input. If you’re
using PaperInputBehavior to implement your own paper-input-like element,
bind this to <input is="iron-input">
‘s preventInvalidInput
property.
*
* JavaScript Info:
* @property preventInvalidInput
* @type Boolean
*
*/
@JsProperty boolean getPreventInvalidInput();
/**
* Set to true to prevent the user from entering invalid input. If you’re
using PaperInputBehavior to implement your own paper-input-like element,
bind this to <input is="iron-input">
‘s preventInvalidInput
property.
*
* JavaScript Info:
* @property preventInvalidInput
* @type Boolean
*
*/
@JsProperty void setPreventInvalidInput(boolean value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autofocus
property.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
*
*/
@JsProperty boolean getAutofocus();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autofocus
property.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
*
*/
@JsProperty void setAutofocus(boolean value);
/**
* Limits the numeric or date-time increments.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s step
property.
*
* JavaScript Info:
* @property step
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getStep();
/**
* Limits the numeric or date-time increments.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s step
property.
*
* JavaScript Info:
* @property step
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setStep(String value);
/**
* The error message to display when the input is invalid. If you’re using
PaperInputBehavior to implement your own paper-input-like element,
bind this to the <paper-input-error>
‘s content, if using.
*
* JavaScript Info:
* @property errorMessage
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getErrorMessage();
/**
* The error message to display when the input is invalid. If you’re using
PaperInputBehavior to implement your own paper-input-like element,
bind this to the <paper-input-error>
‘s content, if using.
*
* JavaScript Info:
* @property errorMessage
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setErrorMessage(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s inputmode
property.
*
* JavaScript Info:
* @property inputmode
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getInputmode();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s inputmode
property.
*
* JavaScript Info:
* @property inputmode
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setInputmode(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autosave
property,
used with type=search.
*
* JavaScript Info:
* @property autosave
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getAutosave();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autosave
property,
used with type=search.
*
* JavaScript Info:
* @property autosave
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setAutosave(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocorrect
property.
*
* JavaScript Info:
* @property autocorrect
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getAutocorrect();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocorrect
property.
*
* JavaScript Info:
* @property autocorrect
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setAutocorrect(String value);
/**
* The label for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
<label>
‘s content and hidden
property, e.g.
<label hidden$="[[!label]]">[[label]]</label>
in your template
*
* JavaScript Info:
* @property label
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getLabel();
/**
* The label for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
<label>
‘s content and hidden
property, e.g.
<label hidden$="[[!label]]">[[label]]</label>
in your template
*
* JavaScript Info:
* @property label
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setLabel(String value);
/**
* The datalist of the input (if any). This should match the id of an existing <datalist>
.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s list
property.
*
* JavaScript Info:
* @property list
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getList();
/**
* The datalist of the input (if any). This should match the id of an existing <datalist>
.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s list
property.
*
* JavaScript Info:
* @property list
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setList(String value);
/**
* The maximum (numeric or date-time) input value.
Can be a String (e.g. "2000-01-01"
) or a Number (e.g. 2
).
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s max
property.
*
* JavaScript Info:
* @property max
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getMax();
/**
* The maximum (numeric or date-time) input value.
Can be a String (e.g. "2000-01-01"
) or a Number (e.g. 2
).
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s max
property.
*
* JavaScript Info:
* @property max
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setMax(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocomplete
property.
*
* JavaScript Info:
* @property autocomplete
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getAutocomplete();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocomplete
property.
*
* JavaScript Info:
* @property autocomplete
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setAutocomplete(String value);
/**
* The minimum (numeric or date-time) input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s min
property.
*
* JavaScript Info:
* @property min
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getMin();
/**
* The minimum (numeric or date-time) input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s min
property.
*
* JavaScript Info:
* @property min
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setMin(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocapitalize
property.
*
* JavaScript Info:
* @property autocapitalize
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getAutocapitalize();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocapitalize
property.
*
* JavaScript Info:
* @property autocapitalize
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setAutocapitalize(String value);
/**
* Set this to specify the pattern allowed by preventInvalidInput
. If
you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s allowedPattern
property.
*
* JavaScript Info:
* @property allowedPattern
* @type String
*
*/
@JsProperty String getAllowedPattern();
/**
* Set this to specify the pattern allowed by preventInvalidInput
. If
you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s allowedPattern
property.
*
* JavaScript Info:
* @property allowedPattern
* @type String
*
*/
@JsProperty void setAllowedPattern(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s name
property.
*
* JavaScript Info:
* @property name
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getName();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s name
property.
*
* JavaScript Info:
* @property name
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setName(String value);
/**
* A pattern to validate the input
with. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s pattern
property.
*
* JavaScript Info:
* @property pattern
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getPattern();
/**
* A pattern to validate the input
with. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s pattern
property.
*
* JavaScript Info:
* @property pattern
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setPattern(String value);
/**
* A placeholder string in addition to the label. If this is set, the label will always float.
*
* JavaScript Info:
* @property placeholder
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getPlaceholder();
/**
* A placeholder string in addition to the label. If this is set, the label will always float.
*
* JavaScript Info:
* @property placeholder
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setPlaceholder(String value);
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s accept
property,
used with type=file.
*
* JavaScript Info:
* @property accept
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getAccept();
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s accept
property,
used with type=file.
*
* JavaScript Info:
* @property accept
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setAccept(String value);
/**
* The value for this element.
*
* JavaScript Info:
* @property value
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty String getValue();
/**
* The value for this element.
*
* JavaScript Info:
* @property value
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty void setValue(String value);
/**
* Name of the validator to use. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s validator
property.
*
* JavaScript Info:
* @property validator
* @type String
*
*/
@JsProperty String getValidator();
/**
* Name of the validator to use. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s validator
property.
*
* JavaScript Info:
* @property validator
* @type String
*
*/
@JsProperty void setValidator(String value);
/**
* The type of the input. The supported types are text
, number
and password
.
If you’re using PaperInputBehavior to implement your own paper-input-like element,
bind this to the <input is="iron-input">
‘s type
property.
*
* JavaScript Info:
* @property type
* @type String
* @behavior PaperTextarea
*/
@JsProperty String getType();
/**
* The type of the input. The supported types are text
, number
and password
.
If you’re using PaperInputBehavior to implement your own paper-input-like element,
bind this to the <input is="iron-input">
‘s type
property.
*
* JavaScript Info:
* @property type
* @type String
* @behavior PaperTextarea
*/
@JsProperty void setType(String value);
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
void addOwnKeyBinding(Object eventString, Object handlerName);
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
void removeOwnKeyBindings();
/**
* Validates the input element and sets an error style if needed.
*
* JavaScript Info:
* @method validate
*
* @return {boolean}
*/
boolean validate();
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString);
/**
* Restores the cursor to its original position after updating the value.
*
* JavaScript Info:
* @method updateValueAndPreserveCaret
* @param {string} newValue
*
*
*/
void updateValueAndPreserveCaret(String newValue);
}