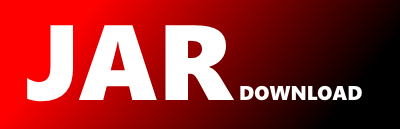
com.vaadin.polymer.paper.PaperRippleElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-ripple project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Material design: Surface reaction
* paper-ripple
provides a visual effect that other paper elements can
use to simulate a rippling effect emanating from the point of contact. The
effect can be visualized as a concentric circle with motion.
* Example:
* <div style="position:relative">
* <paper-ripple></paper-ripple>
* </div>
*
*
*
Note, it’s important that the parent container of the ripple be relative position, otherwise
the ripple will emanate outside of the desired container.
* paper-ripple
listens to “mousedown” and “mouseup” events so it would display ripple
effect when touches on it. You can also defeat the default behavior and
manually route the down and up actions to the ripple element. Note that it is
important if you call downAction()
you will have to make sure to call
upAction()
so that paper-ripple
would end the animation loop.
* Example:
* <paper-ripple id="ripple" style="pointer-events: none;"></paper-ripple>
* ...
* downAction: function(e) {
* this.$.ripple.downAction({detail: {x: e.x, y: e.y}});
* },
* upAction: function(e) {
* this.$.ripple.upAction();
* }
*
*
*
Styling ripple effect:
* Use CSS color property to style the ripple:
* paper-ripple {
* color: #4285f4;
* }
*
*
*
Note that CSS color property is inherited so it is not required to set it on
the paper-ripple
element directly.
* By default, the ripple is centered on the point of contact. Apply the recenters
attribute to have the ripple grow toward the center of its container.
* <paper-ripple recenters></paper-ripple>
*
*
*
You can also center the ripple inside its container from the start.
* <paper-ripple center></paper-ripple>
*
*
*
Apply circle
class to make the rippling effect within a circle.
* <paper-ripple class="circle"></paper-ripple>
*
*
*
*/
@JsType(isNative=true)
public interface PaperRippleElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-ripple";
@JsOverlay public static final String SRC = "paper-ripple/paper-ripple.html";
/**
* The initial opacity set on the wave.
*
* JavaScript Info:
* @property initialOpacity
* @type Number
*
*/
@JsProperty double getInitialOpacity();
/**
* The initial opacity set on the wave.
*
* JavaScript Info:
* @property initialOpacity
* @type Number
*
*/
@JsProperty void setInitialOpacity(double value);
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
* How fast (opacity per second) the wave fades out.
*
* JavaScript Info:
* @property opacityDecayVelocity
* @type Number
*
*/
@JsProperty double getOpacityDecayVelocity();
/**
* How fast (opacity per second) the wave fades out.
*
* JavaScript Info:
* @property opacityDecayVelocity
* @type Number
*
*/
@JsProperty void setOpacityDecayVelocity(double value);
/**
* A list of the visual ripples.
*
* JavaScript Info:
* @property ripples
* @type Array
*
*/
@JsProperty JsArray getRipples();
/**
* A list of the visual ripples.
*
* JavaScript Info:
* @property ripples
* @type Array
*
*/
@JsProperty void setRipples(JsArray value);
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
* If true, the ripple will remain in the “down” state until holdDown
is set to false again.
*
* JavaScript Info:
* @property holdDown
* @type Boolean
*
*/
@JsProperty boolean getHoldDown();
/**
* If true, the ripple will remain in the “down” state until holdDown
is set to false again.
*
* JavaScript Info:
* @property holdDown
* @type Boolean
*
*/
@JsProperty void setHoldDown(boolean value);
/**
* If true, ripples will exhibit a gravitational pull towards
the center of their container as they fade away.
*
* JavaScript Info:
* @property recenters
* @type Boolean
*
*/
@JsProperty boolean getRecenters();
/**
* If true, ripples will exhibit a gravitational pull towards
the center of their container as they fade away.
*
* JavaScript Info:
* @property recenters
* @type Boolean
*
*/
@JsProperty void setRecenters(boolean value);
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getStopKeyboardEventPropagation();
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setStopKeyboardEventPropagation(boolean value);
/**
* If true, ripples will center inside its container
*
* JavaScript Info:
* @property center
* @type Boolean
*
*/
@JsProperty boolean getCenter();
/**
* If true, ripples will center inside its container
*
* JavaScript Info:
* @property center
* @type Boolean
*
*/
@JsProperty void setCenter(boolean value);
/**
* True when there are visible ripples animating within the
element.
*
* JavaScript Info:
* @property animating
* @type Boolean
*
*/
@JsProperty boolean getAnimating();
/**
* True when there are visible ripples animating within the
element.
*
* JavaScript Info:
* @property animating
* @type Boolean
*
*/
@JsProperty void setAnimating(boolean value);
/**
* If true, the ripple will not generate a ripple effect
via pointer interaction.
Calling ripple’s imperative api like simulatedRipple
will
still generate the ripple effect.
*
* JavaScript Info:
* @property noink
* @type Boolean
*
*/
@JsProperty boolean getNoink();
/**
* If true, the ripple will not generate a ripple effect
via pointer interaction.
Calling ripple’s imperative api like simulatedRipple
will
still generate the ripple effect.
*
* JavaScript Info:
* @property noink
* @type Boolean
*
*/
@JsProperty void setNoink(boolean value);
/**
*
*
* JavaScript Info:
* @method removeRipple
* @param {} ripple
*
*
*/
void removeRipple(Object ripple);
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
void addOwnKeyBinding(Object eventString, Object handlerName);
/**
* This conflicts with Element#antimate().
https://developer.mozilla.org/en-US/docs/Web/API/Element/animate
*
* JavaScript Info:
* @method animate
*
*
*/
void animate();
/**
*
*
* JavaScript Info:
* @method onAnimationComplete
*
*
*/
void onAnimationComplete();
/**
*
*
* JavaScript Info:
* @method simulatedRipple
*
*
*/
void simulatedRipple();
/**
*
*
* JavaScript Info:
* @method addRipple
*
*
*/
void addRipple();
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
void removeOwnKeyBindings();
/**
* Provokes a ripple down effect via a UI event,
not respecting the noink
property.
*
* JavaScript Info:
* @method downAction
* @param {Event=} event
*
*
*/
void downAction(JavaScriptObject event);
/**
* Provokes a ripple up effect via a UI event,
not respecting the noink
property.
*
* JavaScript Info:
* @method upAction
* @param {Event=} event
*
*
*/
void upAction(JavaScriptObject event);
/**
* Provokes a ripple up effect via a UI event,
respecting the noink
property.
*
* JavaScript Info:
* @method uiUpAction
* @param {Event=} event
*
*
*/
void uiUpAction(JavaScriptObject event);
/**
* Provokes a ripple down effect via a UI event,
respecting the noink
property.
*
* JavaScript Info:
* @method uiDownAction
* @param {Event=} event
*
*
*/
void uiDownAction(JavaScriptObject event);
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString);
}