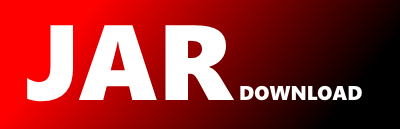
com.vaadin.polymer.paper.PaperSliderElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-slider project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Material design: Sliders
* paper-slider
allows user to select a value from a range of values by
moving the slider thumb. The interactive nature of the slider makes it a
great choice for settings that reflect intensity levels, such as volume,
brightness, or color saturation.
* Example:
* <paper-slider></paper-slider>
*
*
*
Use min
and max
to specify the slider range. Default is 0 to 100.
* Example:
* <paper-slider min="10" max="200" value="110"></paper-slider>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-slider-container-color
* The background color of the bar
* --paper-grey-400
*
*
* --paper-slider-bar-color
* The background color of the slider
* transparent
*
*
* --paper-slider-active-color
* The progress bar color
* --google-blue-700
*
*
* --paper-slider-secondary-color
* The secondary progress bar color
* --google-blue-300
*
*
* --paper-slider-knob-color
* The knob color
* --google-blue-700
*
*
* --paper-slider-disabled-knob-color
* The disabled knob color
* --paper-grey-400
*
*
* --paper-slider-pin-color
* The pin color
* --google-blue-700
*
*
* --paper-slider-font-color
* The pin’s text color
* #fff
*
*
* --paper-slider-markers-color
* The snaps markers color
* #000
*
*
* --paper-slider-disabled-active-color
* The disabled progress bar color
* --paper-grey-400
*
*
* --paper-slider-disabled-secondary-color
* The disabled secondary progress bar color
* --paper-grey-400
*
*
* --paper-slider-knob-start-color
* The fill color of the knob at the far left
* transparent
*
*
* --paper-slider-knob-start-border-color
* The border color of the knob at the far left
* --paper-grey-400
*
*
* --paper-slider-pin-start-color
* The color of the pin at the far left
* --paper-grey-400
*
*
* --paper-slider-height
* Height of the progress bar
* 2px
*
*
* --paper-slider-input
* Mixin applied to the input in editable mode
* {}
*
*
*
*/
@JsType(isNative=true)
public interface PaperSliderElement extends HTMLElement {
@JsOverlay public static final String TAG = "paper-slider";
@JsOverlay public static final String SRC = "paper-slider/paper-slider.html";
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @property markers
* @type Array
*
*/
@JsProperty JsArray getMarkers();
/**
*
*
* JavaScript Info:
* @property markers
* @type Array
*
*/
@JsProperty void setMarkers(JsArray value);
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getStopKeyboardEventPropagation();
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setStopKeyboardEventPropagation(boolean value);
/**
* If true, the element will not produce a ripple effect when interacted
with via the pointer.
*
* JavaScript Info:
* @property noink
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getNoink();
/**
* If true, the element will not produce a ripple effect when interacted
with via the pointer.
*
* JavaScript Info:
* @property noink
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setNoink(boolean value);
/**
* If true, a pin with numeric value label is shown when the slider thumb
is pressed. Use for settings for which users need to know the exact
value of the setting.
*
* JavaScript Info:
* @property pin
* @type Boolean
*
*/
@JsProperty boolean getPin();
/**
* If true, a pin with numeric value label is shown when the slider thumb
is pressed. Use for settings for which users need to know the exact
value of the setting.
*
* JavaScript Info:
* @property pin
* @type Boolean
*
*/
@JsProperty void setPin(boolean value);
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getActive();
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setActive(boolean value);
/**
* If true, the slider thumb snaps to tick marks evenly spaced based
on the step
property value.
*
* JavaScript Info:
* @property snaps
* @type Boolean
*
*/
@JsProperty boolean getSnaps();
/**
* If true, the slider thumb snaps to tick marks evenly spaced based
on the step
property value.
*
* JavaScript Info:
* @property snaps
* @type Boolean
*
*/
@JsProperty void setSnaps(boolean value);
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getPointerDown();
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setPointerDown(boolean value);
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getPressed();
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setPressed(boolean value);
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getReceivedFocusFromKeyboard();
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setReceivedFocusFromKeyboard(boolean value);
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getToggles();
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setToggles(boolean value);
/**
* Set to true to mark the input as required. If used in a form, a
custom element that uses this behavior should also use
Polymer.IronValidatableBehavior and define a custom validation method.
Otherwise, a required
element will always be considered valid.
It’s also strongly recommended to provide a visual style for the element
when its value is invalid.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty boolean getRequired();
/**
* Set to true to mark the input as required. If used in a form, a
custom element that uses this behavior should also use
Polymer.IronValidatableBehavior and define a custom validation method.
Otherwise, a required
element will always be considered valid.
It’s also strongly recommended to provide a visual style for the element
when its value is invalid.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior VaadinDatePicker
*/
@JsProperty void setRequired(boolean value);
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getDisabled();
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setDisabled(boolean value);
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getFocused();
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setFocused(boolean value);
/**
* If true, an input is shown and user can use it to set the slider value.
*
* JavaScript Info:
* @property editable
* @type Boolean
*
*/
@JsProperty boolean getEditable();
/**
* If true, an input is shown and user can use it to set the slider value.
*
* JavaScript Info:
* @property editable
* @type Boolean
*
*/
@JsProperty void setEditable(boolean value);
/**
*
*
* JavaScript Info:
* @property transiting
* @type Boolean
*
*/
@JsProperty boolean getTransiting();
/**
*
*
* JavaScript Info:
* @property transiting
* @type Boolean
*
*/
@JsProperty void setTransiting(boolean value);
/**
* If true, the knob is expanded
*
* JavaScript Info:
* @property expand
* @type Boolean
*
*/
@JsProperty boolean getExpand();
/**
* If true, the knob is expanded
*
* JavaScript Info:
* @property expand
* @type Boolean
*
*/
@JsProperty void setExpand(boolean value);
/**
* True when the user is dragging the slider.
*
* JavaScript Info:
* @property dragging
* @type Boolean
*
*/
@JsProperty boolean getDragging();
/**
* True when the user is dragging the slider.
*
* JavaScript Info:
* @property dragging
* @type Boolean
*
*/
@JsProperty void setDragging(boolean value);
/**
* Specifies the value granularity of the range’s value.
*
* JavaScript Info:
* @property step
* @type Number
* @behavior PaperSlider
*/
@JsProperty double getStep();
/**
* Specifies the value granularity of the range’s value.
*
* JavaScript Info:
* @property step
* @type Number
* @behavior PaperSlider
*/
@JsProperty void setStep(double value);
/**
* The number that represents the current value.
*
* JavaScript Info:
* @property value
* @type Number
* @behavior PaperSlider
*/
@JsProperty double getValue();
/**
* The number that represents the current value.
*
* JavaScript Info:
* @property value
* @type Number
* @behavior PaperSlider
*/
@JsProperty void setValue(double value);
/**
* The maximum number of markers
*
* JavaScript Info:
* @property maxMarkers
* @type Number
*
*/
@JsProperty double getMaxMarkers();
/**
* The maximum number of markers
*
* JavaScript Info:
* @property maxMarkers
* @type Number
*
*/
@JsProperty void setMaxMarkers(double value);
/**
* The immediate value of the slider. This value is updated while the user
is dragging the slider.
*
* JavaScript Info:
* @property immediateValue
* @type Number
*
*/
@JsProperty double getImmediateValue();
/**
* The immediate value of the slider. This value is updated while the user
is dragging the slider.
*
* JavaScript Info:
* @property immediateValue
* @type Number
*
*/
@JsProperty void setImmediateValue(double value);
/**
* The number that represents the current secondary progress.
*
* JavaScript Info:
* @property secondaryProgress
* @type Number
*
*/
@JsProperty double getSecondaryProgress();
/**
* The number that represents the current secondary progress.
*
* JavaScript Info:
* @property secondaryProgress
* @type Number
*
*/
@JsProperty void setSecondaryProgress(double value);
/**
* Returns the ratio of the value.
*
* JavaScript Info:
* @property ratio
* @type Number
* @behavior PaperSlider
*/
@JsProperty double getRatio();
/**
* Returns the ratio of the value.
*
* JavaScript Info:
* @property ratio
* @type Number
* @behavior PaperSlider
*/
@JsProperty void setRatio(double value);
/**
* The number that indicates the maximum value of the range.
*
* JavaScript Info:
* @property max
* @type Number
* @behavior PaperSlider
*/
@JsProperty double getMax();
/**
* The number that indicates the maximum value of the range.
*
* JavaScript Info:
* @property max
* @type Number
* @behavior PaperSlider
*/
@JsProperty void setMax(double value);
/**
* The number that indicates the minimum value of the range.
*
* JavaScript Info:
* @property min
* @type Number
* @behavior PaperSlider
*/
@JsProperty double getMin();
/**
* The number that indicates the minimum value of the range.
*
* JavaScript Info:
* @property min
* @type Number
* @behavior PaperSlider
*/
@JsProperty void setMin(double value);
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
@JsProperty String getAriaActiveAttribute();
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
@JsProperty void setAriaActiveAttribute(String value);
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty String getName();
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
@JsProperty void setName(String value);
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
void addOwnKeyBinding(Object eventString, Object handlerName);
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
void removeOwnKeyBindings();
/**
* Increases value by step
but not above max
.
*
* JavaScript Info:
* @method increment
*
*
*/
void increment();
/**
* Returns the <paper-ripple>
element used by this element to create
ripple effects. The element’s ripple is created on demand, when
necessary, and calling this method will force the
ripple to be created.
*
* JavaScript Info:
* @method getRipple
* @behavior PaperToggleButton
*
*/
void getRipple();
/**
* Returns true if this element currently contains a ripple effect.
*
* JavaScript Info:
* @method hasRipple
* @behavior PaperToggleButton
* @return {boolean}
*/
boolean hasRipple();
/**
* Decreases value by step
but not below min
.
*
* JavaScript Info:
* @method decrement
*
*
*/
void decrement();
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString);
/**
* Ensures this element contains a ripple effect. For startup efficiency
the ripple effect is dynamically on demand when needed.
*
* JavaScript Info:
* @method ensureRipple
* @param {!Event=} optTriggeringEvent
* @behavior PaperTab
*
*/
void ensureRipple(JavaScriptObject optTriggeringEvent);
}