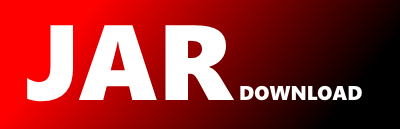
com.vaadin.polymer.paper.widget.PaperProgress Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-progress project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Material design: Progress & activity
* The progress bars are for situations where the percentage completed can be
determined. They give users a quick sense of how much longer an operation
will take.
* Example:
* <paper-progress value="10"></paper-progress>
*
*
*
There is also a secondary progress which is useful for displaying intermediate
progress, such as the buffer level during a streaming playback progress bar.
* Example:
* <paper-progress value="10" secondary-progress="30"></paper-progress>
*
*
*
Styling progress bar:
* To change the active progress bar color:
* paper-progress {
* --paper-progress-active-color: #e91e63;
* }
*
*
*
To change the secondary progress bar color:
* paper-progress {
* --paper-progress-secondary-color: #f8bbd0;
* }
*
*
*
To change the progress bar background color:
* paper-progress {
* --paper-progress-container-color: #64ffda;
* }
*
*
*
Add the class transiting
to a paper-progress to animate the progress bar when
the value changed. You can also customize the transition:
* paper-progress {
* --paper-progress-transition-duration: 0.08s;
* --paper-progress-transition-timing-function: ease;
* --paper-progress-transition-transition-delay: 0s;
* }
*
*
*
To change the duration of the indeterminate cycle:
* paper-progress {
* --paper-progress-indeterminate-cycle-duration: 2s;
* }
*
*
*
The following mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-progress-container
* Mixin applied to container
* {}
*
*
* --paper-progress-transition-duration
* Duration of the transition
* 0.008s
*
*
* --paper-progress-transition-timing-function
* The timing function for the transition
* ease
*
*
* --paper-progress-transition-delay
* delay for the transition
* 0s
*
*
* --paper-progress-container-color
* Color of the container
* --google-grey-300
*
*
* --paper-progress-active-color
* The color of the active bar
* --google-green-500
*
*
* --paper-progress-secondary-color
* The color of the secondary bar
* --google-green-100
*
*
* --paper-progress-disabled-active-color
* The color of the active bar if disabled
* --google-grey-500
*
*
* --paper-progress-disabled-secondary-color
* The color of the secondary bar if disabled
* --google-grey-300
*
*
* --paper-progress-height
* The height of the progress bar
* 4px
*
*
* --paper-progress-indeterminate-cycle-duration
* Duration of an indeterminate cycle
* 2s
*
*
*
*/
public class PaperProgress extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperProgress() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperProgress(String html) {
super(PaperProgressElement.TAG, PaperProgressElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperProgressElement getPolymerElement() {
return (PaperProgressElement) getElement();
}
/**
* True if the progress is disabled.
*
* JavaScript Info:
* @property disabled
* @type Boolean
*
*/
public boolean getDisabled() {
return getPolymerElement().getDisabled();
}
/**
* True if the progress is disabled.
*
* JavaScript Info:
* @property disabled
* @type Boolean
*
*/
public void setDisabled(boolean value) {
getPolymerElement().setDisabled(value);
}
/**
* Use an indeterminate progress indicator.
*
* JavaScript Info:
* @property indeterminate
* @type Boolean
*
*/
public boolean getIndeterminate() {
return getPolymerElement().getIndeterminate();
}
/**
* Use an indeterminate progress indicator.
*
* JavaScript Info:
* @property indeterminate
* @type Boolean
*
*/
public void setIndeterminate(boolean value) {
getPolymerElement().setIndeterminate(value);
}
/**
* The number that represents the current secondary progress.
*
* JavaScript Info:
* @property secondaryProgress
* @type Number
*
*/
public double getSecondaryProgress() {
return getPolymerElement().getSecondaryProgress();
}
/**
* The number that represents the current secondary progress.
*
* JavaScript Info:
* @property secondaryProgress
* @type Number
*
*/
public void setSecondaryProgress(double value) {
getPolymerElement().setSecondaryProgress(value);
}
/**
* The secondary ratio
*
* JavaScript Info:
* @property secondaryRatio
* @type Number
*
*/
public double getSecondaryRatio() {
return getPolymerElement().getSecondaryRatio();
}
/**
* The secondary ratio
*
* JavaScript Info:
* @property secondaryRatio
* @type Number
*
*/
public void setSecondaryRatio(double value) {
getPolymerElement().setSecondaryRatio(value);
}
/**
* The number that indicates the maximum value of the range.
*
* JavaScript Info:
* @property max
* @type Number
* @behavior PaperSlider
*/
public double getMax() {
return getPolymerElement().getMax();
}
/**
* The number that indicates the maximum value of the range.
*
* JavaScript Info:
* @property max
* @type Number
* @behavior PaperSlider
*/
public void setMax(double value) {
getPolymerElement().setMax(value);
}
/**
* The number that indicates the minimum value of the range.
*
* JavaScript Info:
* @property min
* @type Number
* @behavior PaperSlider
*/
public double getMin() {
return getPolymerElement().getMin();
}
/**
* The number that indicates the minimum value of the range.
*
* JavaScript Info:
* @property min
* @type Number
* @behavior PaperSlider
*/
public void setMin(double value) {
getPolymerElement().setMin(value);
}
/**
* Returns the ratio of the value.
*
* JavaScript Info:
* @property ratio
* @type Number
* @behavior PaperSlider
*/
public double getRatio() {
return getPolymerElement().getRatio();
}
/**
* Returns the ratio of the value.
*
* JavaScript Info:
* @property ratio
* @type Number
* @behavior PaperSlider
*/
public void setRatio(double value) {
getPolymerElement().setRatio(value);
}
/**
* Specifies the value granularity of the range’s value.
*
* JavaScript Info:
* @property step
* @type Number
* @behavior PaperSlider
*/
public double getStep() {
return getPolymerElement().getStep();
}
/**
* Specifies the value granularity of the range’s value.
*
* JavaScript Info:
* @property step
* @type Number
* @behavior PaperSlider
*/
public void setStep(double value) {
getPolymerElement().setStep(value);
}
/**
* The number that represents the current value.
*
* JavaScript Info:
* @property value
* @type Number
* @behavior PaperSlider
*/
public double getValue() {
return getPolymerElement().getValue();
}
/**
* The number that represents the current value.
*
* JavaScript Info:
* @property value
* @type Number
* @behavior PaperSlider
*/
public void setValue(double value) {
getPolymerElement().setValue(value);
}
// Needed in UIBinder
/**
* The number that represents the current secondary progress.
*
* JavaScript Info:
* @attribute secondary-progress
*
*/
public void setSecondaryProgress(String value) {
Polymer.property(this.getPolymerElement(), "secondaryProgress", value);
}
// Needed in UIBinder
/**
* The secondary ratio
*
* JavaScript Info:
* @attribute secondary-ratio
*
*/
public void setSecondaryRatio(String value) {
Polymer.property(this.getPolymerElement(), "secondaryRatio", value);
}
// Needed in UIBinder
/**
* The number that indicates the maximum value of the range.
*
* JavaScript Info:
* @attribute max
* @behavior PaperSlider
*/
public void setMax(String value) {
Polymer.property(this.getPolymerElement(), "max", value);
}
// Needed in UIBinder
/**
* The number that indicates the minimum value of the range.
*
* JavaScript Info:
* @attribute min
* @behavior PaperSlider
*/
public void setMin(String value) {
Polymer.property(this.getPolymerElement(), "min", value);
}
// Needed in UIBinder
/**
* Returns the ratio of the value.
*
* JavaScript Info:
* @attribute ratio
* @behavior PaperSlider
*/
public void setRatio(String value) {
Polymer.property(this.getPolymerElement(), "ratio", value);
}
// Needed in UIBinder
/**
* Specifies the value granularity of the range’s value.
*
* JavaScript Info:
* @attribute step
* @behavior PaperSlider
*/
public void setStep(String value) {
Polymer.property(this.getPolymerElement(), "step", value);
}
// Needed in UIBinder
/**
* The number that represents the current value.
*
* JavaScript Info:
* @attribute value
* @behavior PaperSlider
*/
public void setValue(String value) {
Polymer.property(this.getPolymerElement(), "value", value);
}
}