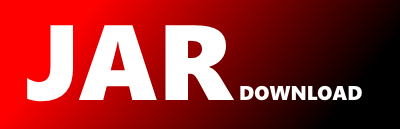
com.vaadin.polymer.paper.widget.PaperRipple Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-ripple project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.*;
import com.vaadin.polymer.paper.widget.event.TransitionendEvent;
import com.vaadin.polymer.paper.widget.event.TransitionendEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Material design: Surface reaction
* paper-ripple
provides a visual effect that other paper elements can
use to simulate a rippling effect emanating from the point of contact. The
effect can be visualized as a concentric circle with motion.
* Example:
* <div style="position:relative">
* <paper-ripple></paper-ripple>
* </div>
*
*
*
Note, it’s important that the parent container of the ripple be relative position, otherwise
the ripple will emanate outside of the desired container.
* paper-ripple
listens to “mousedown” and “mouseup” events so it would display ripple
effect when touches on it. You can also defeat the default behavior and
manually route the down and up actions to the ripple element. Note that it is
important if you call downAction()
you will have to make sure to call
upAction()
so that paper-ripple
would end the animation loop.
* Example:
* <paper-ripple id="ripple" style="pointer-events: none;"></paper-ripple>
* ...
* downAction: function(e) {
* this.$.ripple.downAction({detail: {x: e.x, y: e.y}});
* },
* upAction: function(e) {
* this.$.ripple.upAction();
* }
*
*
*
Styling ripple effect:
* Use CSS color property to style the ripple:
* paper-ripple {
* color: #4285f4;
* }
*
*
*
Note that CSS color property is inherited so it is not required to set it on
the paper-ripple
element directly.
* By default, the ripple is centered on the point of contact. Apply the recenters
attribute to have the ripple grow toward the center of its container.
* <paper-ripple recenters></paper-ripple>
*
*
*
You can also center the ripple inside its container from the start.
* <paper-ripple center></paper-ripple>
*
*
*
Apply circle
class to make the rippling effect within a circle.
* <paper-ripple class="circle"></paper-ripple>
*
*
*
*/
public class PaperRipple extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperRipple() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperRipple(String html) {
super(PaperRippleElement.TAG, PaperRippleElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperRippleElement getPolymerElement() {
return (PaperRippleElement) getElement();
}
/**
* The initial opacity set on the wave.
*
* JavaScript Info:
* @property initialOpacity
* @type Number
*
*/
public double getInitialOpacity() {
return getPolymerElement().getInitialOpacity();
}
/**
* The initial opacity set on the wave.
*
* JavaScript Info:
* @property initialOpacity
* @type Number
*
*/
public void setInitialOpacity(double value) {
getPolymerElement().setInitialOpacity(value);
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
public JavaScriptObject getKeyBindings() {
return getPolymerElement().getKeyBindings();
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
public void setKeyBindings(JavaScriptObject value) {
getPolymerElement().setKeyBindings(value);
}
/**
* How fast (opacity per second) the wave fades out.
*
* JavaScript Info:
* @property opacityDecayVelocity
* @type Number
*
*/
public double getOpacityDecayVelocity() {
return getPolymerElement().getOpacityDecayVelocity();
}
/**
* How fast (opacity per second) the wave fades out.
*
* JavaScript Info:
* @property opacityDecayVelocity
* @type Number
*
*/
public void setOpacityDecayVelocity(double value) {
getPolymerElement().setOpacityDecayVelocity(value);
}
/**
* A list of the visual ripples.
*
* JavaScript Info:
* @property ripples
* @type Array
*
*/
public JsArray getRipples() {
return getPolymerElement().getRipples();
}
/**
* A list of the visual ripples.
*
* JavaScript Info:
* @property ripples
* @type Array
*
*/
public void setRipples(JsArray value) {
getPolymerElement().setRipples(value);
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public JavaScriptObject getKeyEventTarget() {
return getPolymerElement().getKeyEventTarget();
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(JavaScriptObject value) {
getPolymerElement().setKeyEventTarget(value);
}
/**
* If true, the ripple will remain in the “down” state until holdDown
is set to false again.
*
* JavaScript Info:
* @property holdDown
* @type Boolean
*
*/
public boolean getHoldDown() {
return getPolymerElement().getHoldDown();
}
/**
* If true, the ripple will remain in the “down” state until holdDown
is set to false again.
*
* JavaScript Info:
* @property holdDown
* @type Boolean
*
*/
public void setHoldDown(boolean value) {
getPolymerElement().setHoldDown(value);
}
/**
* If true, ripples will exhibit a gravitational pull towards
the center of their container as they fade away.
*
* JavaScript Info:
* @property recenters
* @type Boolean
*
*/
public boolean getRecenters() {
return getPolymerElement().getRecenters();
}
/**
* If true, ripples will exhibit a gravitational pull towards
the center of their container as they fade away.
*
* JavaScript Info:
* @property recenters
* @type Boolean
*
*/
public void setRecenters(boolean value) {
getPolymerElement().setRecenters(value);
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public boolean getStopKeyboardEventPropagation() {
return getPolymerElement().getStopKeyboardEventPropagation();
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public void setStopKeyboardEventPropagation(boolean value) {
getPolymerElement().setStopKeyboardEventPropagation(value);
}
/**
* If true, ripples will center inside its container
*
* JavaScript Info:
* @property center
* @type Boolean
*
*/
public boolean getCenter() {
return getPolymerElement().getCenter();
}
/**
* If true, ripples will center inside its container
*
* JavaScript Info:
* @property center
* @type Boolean
*
*/
public void setCenter(boolean value) {
getPolymerElement().setCenter(value);
}
/**
* True when there are visible ripples animating within the
element.
*
* JavaScript Info:
* @property animating
* @type Boolean
*
*/
public boolean getAnimating() {
return getPolymerElement().getAnimating();
}
/**
* True when there are visible ripples animating within the
element.
*
* JavaScript Info:
* @property animating
* @type Boolean
*
*/
public void setAnimating(boolean value) {
getPolymerElement().setAnimating(value);
}
/**
* If true, the ripple will not generate a ripple effect
via pointer interaction.
Calling ripple’s imperative api like simulatedRipple
will
still generate the ripple effect.
*
* JavaScript Info:
* @property noink
* @type Boolean
*
*/
public boolean getNoink() {
return getPolymerElement().getNoink();
}
/**
* If true, the ripple will not generate a ripple effect
via pointer interaction.
Calling ripple’s imperative api like simulatedRipple
will
still generate the ripple effect.
*
* JavaScript Info:
* @property noink
* @type Boolean
*
*/
public void setNoink(boolean value) {
getPolymerElement().setNoink(value);
}
// Needed in UIBinder
/**
* A list of the visual ripples.
*
* JavaScript Info:
* @attribute ripples
*
*/
public void setRipples(String value) {
Polymer.property(this.getPolymerElement(), "ripples", value);
}
// Needed in UIBinder
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @attribute key-bindings
* @behavior VaadinDatePicker
*/
public void setKeyBindings(String value) {
Polymer.property(this.getPolymerElement(), "keyBindings", value);
}
// Needed in UIBinder
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @attribute key-event-target
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(String value) {
Polymer.property(this.getPolymerElement(), "keyEventTarget", value);
}
// Needed in UIBinder
/**
* How fast (opacity per second) the wave fades out.
*
* JavaScript Info:
* @attribute opacity-decay-velocity
*
*/
public void setOpacityDecayVelocity(String value) {
Polymer.property(this.getPolymerElement(), "opacityDecayVelocity", value);
}
// Needed in UIBinder
/**
* The initial opacity set on the wave.
*
* JavaScript Info:
* @attribute initial-opacity
*
*/
public void setInitialOpacity(String value) {
Polymer.property(this.getPolymerElement(), "initialOpacity", value);
}
/**
*
*
* JavaScript Info:
* @method removeRipple
* @param {} ripple
*
*
*/
public void removeRipple(Object ripple) {
getPolymerElement().removeRipple(ripple);
}
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
public void addOwnKeyBinding(Object eventString, Object handlerName) {
getPolymerElement().addOwnKeyBinding(eventString, handlerName);
}
/**
*
*
* JavaScript Info:
* @method simulatedRipple
*
*
*/
public void simulatedRipple() {
getPolymerElement().simulatedRipple();
}
/**
*
*
* JavaScript Info:
* @method onAnimationComplete
*
*
*/
public void onAnimationComplete() {
getPolymerElement().onAnimationComplete();
}
/**
* This conflicts with Element#antimate().
https://developer.mozilla.org/en-US/docs/Web/API/Element/animate
*
* JavaScript Info:
* @method animate
*
*
*/
public void animate() {
getPolymerElement().animate();
}
/**
*
*
* JavaScript Info:
* @method addRipple
*
*
*/
public void addRipple() {
getPolymerElement().addRipple();
}
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
public void removeOwnKeyBindings() {
getPolymerElement().removeOwnKeyBindings();
}
/**
* Provokes a ripple down effect via a UI event,
not respecting the noink
property.
*
* JavaScript Info:
* @method downAction
* @param {Event=} event
*
*
*/
public void downAction(JavaScriptObject event) {
getPolymerElement().downAction(event);
}
/**
* Provokes a ripple up effect via a UI event,
not respecting the noink
property.
*
* JavaScript Info:
* @method upAction
* @param {Event=} event
*
*
*/
public void upAction(JavaScriptObject event) {
getPolymerElement().upAction(event);
}
/**
* Provokes a ripple up effect via a UI event,
respecting the noink
property.
*
* JavaScript Info:
* @method uiUpAction
* @param {Event=} event
*
*
*/
public void uiUpAction(JavaScriptObject event) {
getPolymerElement().uiUpAction(event);
}
/**
* Provokes a ripple down effect via a UI event,
respecting the noink
property.
*
* JavaScript Info:
* @method uiDownAction
* @param {Event=} event
*
*
*/
public void uiDownAction(JavaScriptObject event) {
getPolymerElement().uiDownAction(event);
}
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
public boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString) {
return getPolymerElement().keyboardEventMatchesKeys(event, eventString);
}
/**
* Fired when the animation finishes.
* This is useful if you want to wait until
* the ripple animation finishes to perform some action.
*
*
*
*
* JavaScript Info:
* @event transitionend
*/
public HandlerRegistration addTransitionendHandler(TransitionendEventHandler handler) {
return addDomHandler(handler, TransitionendEvent.TYPE);
}
}