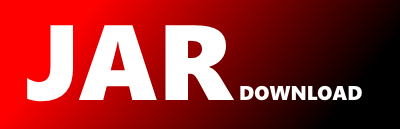
com.vaadin.polymer.paper.widget.PaperScrollHeaderPanel Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-scroll-header-panel project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.*;
import com.vaadin.polymer.paper.widget.event.ContentScrollEvent;
import com.vaadin.polymer.paper.widget.event.ContentScrollEventHandler;
import com.vaadin.polymer.paper.widget.event.PaperHeaderTransformEvent;
import com.vaadin.polymer.paper.widget.event.PaperHeaderTransformEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Material design: Scrolling techniques
* paper-scroll-header-panel
contains a header section and a content section. The
header is initially on the top part of the view but it scrolls away with the
rest of the scrollable content. Upon scrolling slightly up at any point, the
header scrolls back into view. This saves screen space and allows users to
access important controls by easily moving them back to the view.
* Important: The paper-scroll-header-panel
will not display if its parent does not have a height.
* Using layout classes or custom properties, you can easily make the paper-scroll-header-panel
fill the screen
* <body class="fullbleed layout vertical">
* <paper-scroll-header-panel class="flex">
* <paper-toolbar>
* <div>Hello World!</div>
* </paper-toolbar>
* </paper-scroll-header-panel>
* </body>
*
* or, if you would prefer to do it in CSS, just give html
, body
, and paper-scroll-header-panel
a height of 100%:
* html, body {
* height: 100%;
* margin: 0;
* }
* paper-scroll-header-panel {
* height: 100%;
* }
*
* paper-scroll-header-panel
works well with paper-toolbar
but can use any element
that represents a header by adding a paper-header
class to it.
* Note: If the class paper-header
is used, the header must be positioned relative or absolute. e.g.
* .paper-header {
* position: relative;
* }
*
* <paper-scroll-header-panel>
* <div class="paper-header">Header</div>
* <div>Content goes here...</div>
* </paper-scroll-header-panel>
*
* Styling
* =======
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* –paper-scroll-header-panel-full-header
* To change background for toolbar when it is at its full size
* {}
*
*
* –paper-scroll-header-panel-condensed-header
* To change the background for toolbar when it is condensed
* {}
*
*
* –paper-scroll-header-panel-container
* To override or add container styles
* {}
*
*
* –paper-scroll-header-panel-header-container
* To override or add header styles
* {}
*
*
*
*/
public class PaperScrollHeaderPanel extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperScrollHeaderPanel() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperScrollHeaderPanel(String html) {
super(PaperScrollHeaderPanelElement.TAG, PaperScrollHeaderPanelElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperScrollHeaderPanelElement getPolymerElement() {
return (PaperScrollHeaderPanelElement) getElement();
}
/**
* If true, the header is fixed to the top and never moves away.
*
* JavaScript Info:
* @property fixed
* @type Boolean
*
*/
public boolean getFixed() {
return getPolymerElement().getFixed();
}
/**
* If true, the header is fixed to the top and never moves away.
*
* JavaScript Info:
* @property fixed
* @type Boolean
*
*/
public void setFixed(boolean value) {
getPolymerElement().setFixed(value);
}
/**
* The height of the header when it is at its full size.
* By default, the height will be measured when it is ready. If the height
changes later the user needs to either set this value to reflect the
new height or invoke measureHeaderHeight()
.
*
* JavaScript Info:
* @property headerHeight
* @type Number
*
*/
public double getHeaderHeight() {
return getPolymerElement().getHeaderHeight();
}
/**
* The height of the header when it is at its full size.
* By default, the height will be measured when it is ready. If the height
changes later the user needs to either set this value to reflect the
new height or invoke measureHeaderHeight()
.
*
* JavaScript Info:
* @property headerHeight
* @type Number
*
*/
public void setHeaderHeight(double value) {
getPolymerElement().setHeaderHeight(value);
}
/**
* The state of the header. Depending on the configuration and the scrollTop
value,
the header state could change to
Polymer.PaperScrollHeaderPanel.HEADER_STATE_EXPANDED
Polymer.PaperScrollHeaderPanel.HEADER_STATE_HIDDEN
Polymer.PaperScrollHeaderPanel.HEADER_STATE_CONDENSED
Polymer.PaperScrollHeaderPanel.HEADER_STATE_INTERPOLATED
*
* JavaScript Info:
* @property headerState
* @type Number
*
*/
public double getHeaderState() {
return getPolymerElement().getHeaderState();
}
/**
* The state of the header. Depending on the configuration and the scrollTop
value,
the header state could change to
Polymer.PaperScrollHeaderPanel.HEADER_STATE_EXPANDED
Polymer.PaperScrollHeaderPanel.HEADER_STATE_HIDDEN
Polymer.PaperScrollHeaderPanel.HEADER_STATE_CONDENSED
Polymer.PaperScrollHeaderPanel.HEADER_STATE_INTERPOLATED
*
* JavaScript Info:
* @property headerState
* @type Number
*
*/
public void setHeaderState(double value) {
getPolymerElement().setHeaderState(value);
}
/**
* If true, the header’s height will condense to condensedHeaderHeight
as the user scrolls down from the top of the content area.
*
* JavaScript Info:
* @property condenses
* @type Boolean
*
*/
public boolean getCondenses() {
return getPolymerElement().getCondenses();
}
/**
* If true, the header’s height will condense to condensedHeaderHeight
as the user scrolls down from the top of the content area.
*
* JavaScript Info:
* @property condenses
* @type Boolean
*
*/
public void setCondenses(boolean value) {
getPolymerElement().setCondenses(value);
}
/**
* If true, no cross-fade transition from one background to another.
*
* JavaScript Info:
* @property noDissolve
* @type Boolean
*
*/
public boolean getNoDissolve() {
return getPolymerElement().getNoDissolve();
}
/**
* If true, no cross-fade transition from one background to another.
*
* JavaScript Info:
* @property noDissolve
* @type Boolean
*
*/
public void setNoDissolve(boolean value) {
getPolymerElement().setNoDissolve(value);
}
/**
* If true, the header doesn’t slide back in when scrolling back up.
*
* JavaScript Info:
* @property noReveal
* @type Boolean
*
*/
public boolean getNoReveal() {
return getPolymerElement().getNoReveal();
}
/**
* If true, the header doesn’t slide back in when scrolling back up.
*
* JavaScript Info:
* @property noReveal
* @type Boolean
*
*/
public void setNoReveal(boolean value) {
getPolymerElement().setNoReveal(value);
}
/**
* The height of the header when it is condensed.
* By default, condensedHeaderHeight
is 1/3 of headerHeight
unless
this is specified.
*
* JavaScript Info:
* @property condensedHeaderHeight
* @type Number
*
*/
public double getCondensedHeaderHeight() {
return getPolymerElement().getCondensedHeaderHeight();
}
/**
* The height of the header when it is condensed.
* By default, condensedHeaderHeight
is 1/3 of headerHeight
unless
this is specified.
*
* JavaScript Info:
* @property condensedHeaderHeight
* @type Number
*
*/
public void setCondensedHeaderHeight(double value) {
getPolymerElement().setCondensedHeaderHeight(value);
}
/**
* By default, the top part of the header stays when the header is being
condensed. Set this to true if you want the top part of the header
to be scrolled away.
*
* JavaScript Info:
* @property scrollAwayTopbar
* @type Boolean
*
*/
public boolean getScrollAwayTopbar() {
return getPolymerElement().getScrollAwayTopbar();
}
/**
* By default, the top part of the header stays when the header is being
condensed. Set this to true if you want the top part of the header
to be scrolled away.
*
* JavaScript Info:
* @property scrollAwayTopbar
* @type Boolean
*
*/
public void setScrollAwayTopbar(boolean value) {
getPolymerElement().setScrollAwayTopbar(value);
}
/**
* If true, the condensed header is always shown and does not move away.
*
* JavaScript Info:
* @property keepCondensedHeader
* @type Boolean
*
*/
public boolean getKeepCondensedHeader() {
return getPolymerElement().getKeepCondensedHeader();
}
/**
* If true, the condensed header is always shown and does not move away.
*
* JavaScript Info:
* @property keepCondensedHeader
* @type Boolean
*
*/
public void setKeepCondensedHeader(boolean value) {
getPolymerElement().setKeepCondensedHeader(value);
}
// Needed in UIBinder
/**
* The state of the header. Depending on the configuration and the scrollTop
value,
the header state could change to
Polymer.PaperScrollHeaderPanel.HEADER_STATE_EXPANDED
Polymer.PaperScrollHeaderPanel.HEADER_STATE_HIDDEN
Polymer.PaperScrollHeaderPanel.HEADER_STATE_CONDENSED
Polymer.PaperScrollHeaderPanel.HEADER_STATE_INTERPOLATED
*
* JavaScript Info:
* @attribute header-state
*
*/
public void setHeaderState(String value) {
Polymer.property(this.getPolymerElement(), "headerState", value);
}
// Needed in UIBinder
/**
* The height of the header when it is at its full size.
* By default, the height will be measured when it is ready. If the height
changes later the user needs to either set this value to reflect the
new height or invoke measureHeaderHeight()
.
*
* JavaScript Info:
* @attribute header-height
*
*/
public void setHeaderHeight(String value) {
Polymer.property(this.getPolymerElement(), "headerHeight", value);
}
// Needed in UIBinder
/**
* The height of the header when it is condensed.
* By default, condensedHeaderHeight
is 1/3 of headerHeight
unless
this is specified.
*
* JavaScript Info:
* @attribute condensed-header-height
*
*/
public void setCondensedHeaderHeight(String value) {
Polymer.property(this.getPolymerElement(), "condensedHeaderHeight", value);
}
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
public void stopResizeNotificationsFor(Object target) {
getPolymerElement().stopResizeNotificationsFor(target);
}
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
public void assignParentResizable(Object parentResizable) {
getPolymerElement().assignParentResizable(parentResizable);
}
/**
* Scroll to a specific y coordinate.
*
* JavaScript Info:
* @method scroll
* @param {number} top
* @param {boolean} smooth
*
*
*/
public void scroll(double top, boolean smooth) {
getPolymerElement().scroll(top, smooth);
}
/**
* Invoke this to tell paper-scroll-header-panel
to re-measure the header’s
height.
*
* JavaScript Info:
* @method measureHeaderHeight
*
*
*/
public void measureHeaderHeight() {
getPolymerElement().measureHeaderHeight();
}
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
public void notifyResize() {
getPolymerElement().notifyResize();
}
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
public boolean resizerShouldNotify(JavaScriptObject element) {
return getPolymerElement().resizerShouldNotify(element);
}
/**
* Condense the header.
*
* JavaScript Info:
* @method condense
* @param {boolean} smooth
*
*
*/
public void condense(boolean smooth) {
getPolymerElement().condense(smooth);
}
/**
* Scroll to the top of the content.
*
* JavaScript Info:
* @method scrollToTop
* @param {boolean} smooth
*
*
*/
public void scrollToTop(boolean smooth) {
getPolymerElement().scrollToTop(smooth);
}
/**
* Fired when the content has been scrolled.
*
* JavaScript Info:
* @event content-scroll
*/
public HandlerRegistration addContentScrollHandler(ContentScrollEventHandler handler) {
return addDomHandler(handler, ContentScrollEvent.TYPE);
}
/**
* Fired when the header is transformed.
*
* JavaScript Info:
* @event paper-header-transform
*/
public HandlerRegistration addPaperHeaderTransformHandler(PaperHeaderTransformEventHandler handler) {
return addDomHandler(handler, PaperHeaderTransformEvent.TYPE);
}
}