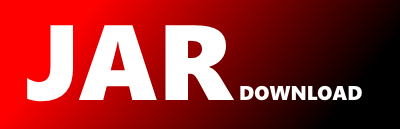
com.vaadin.polymer.paper.widget.PaperSlider Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-slider project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.*;
import com.vaadin.polymer.paper.widget.event.ChangeEvent;
import com.vaadin.polymer.paper.widget.event.ChangeEventHandler;
import com.vaadin.polymer.paper.widget.event.ImmediateValueChangeEvent;
import com.vaadin.polymer.paper.widget.event.ImmediateValueChangeEventHandler;
import com.vaadin.polymer.paper.widget.event.ValueChangeEvent;
import com.vaadin.polymer.paper.widget.event.ValueChangeEventHandler;
import com.vaadin.polymer.iron.widget.event.IronFormElementRegisterEvent;
import com.vaadin.polymer.iron.widget.event.IronFormElementRegisterEventHandler;
import com.vaadin.polymer.iron.widget.event.IronFormElementUnregisterEvent;
import com.vaadin.polymer.iron.widget.event.IronFormElementUnregisterEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Material design: Sliders
* paper-slider
allows user to select a value from a range of values by
moving the slider thumb. The interactive nature of the slider makes it a
great choice for settings that reflect intensity levels, such as volume,
brightness, or color saturation.
* Example:
* <paper-slider></paper-slider>
*
*
*
Use min
and max
to specify the slider range. Default is 0 to 100.
* Example:
* <paper-slider min="10" max="200" value="110"></paper-slider>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-slider-container-color
* The background color of the bar
* --paper-grey-400
*
*
* --paper-slider-bar-color
* The background color of the slider
* transparent
*
*
* --paper-slider-active-color
* The progress bar color
* --google-blue-700
*
*
* --paper-slider-secondary-color
* The secondary progress bar color
* --google-blue-300
*
*
* --paper-slider-knob-color
* The knob color
* --google-blue-700
*
*
* --paper-slider-disabled-knob-color
* The disabled knob color
* --paper-grey-400
*
*
* --paper-slider-pin-color
* The pin color
* --google-blue-700
*
*
* --paper-slider-font-color
* The pin’s text color
* #fff
*
*
* --paper-slider-markers-color
* The snaps markers color
* #000
*
*
* --paper-slider-disabled-active-color
* The disabled progress bar color
* --paper-grey-400
*
*
* --paper-slider-disabled-secondary-color
* The disabled secondary progress bar color
* --paper-grey-400
*
*
* --paper-slider-knob-start-color
* The fill color of the knob at the far left
* transparent
*
*
* --paper-slider-knob-start-border-color
* The border color of the knob at the far left
* --paper-grey-400
*
*
* --paper-slider-pin-start-color
* The color of the pin at the far left
* --paper-grey-400
*
*
* --paper-slider-height
* Height of the progress bar
* 2px
*
*
* --paper-slider-input
* Mixin applied to the input in editable mode
* {}
*
*
*
*/
public class PaperSlider extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperSlider() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperSlider(String html) {
super(PaperSliderElement.TAG, PaperSliderElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperSliderElement getPolymerElement() {
return (PaperSliderElement) getElement();
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
public JavaScriptObject getKeyBindings() {
return getPolymerElement().getKeyBindings();
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
*
*/
public void setKeyBindings(JavaScriptObject value) {
getPolymerElement().setKeyBindings(value);
}
/**
*
*
* JavaScript Info:
* @property markers
* @type Array
*
*/
public JsArray getMarkers() {
return getPolymerElement().getMarkers();
}
/**
*
*
* JavaScript Info:
* @property markers
* @type Array
*
*/
public void setMarkers(JsArray value) {
getPolymerElement().setMarkers(value);
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public JavaScriptObject getKeyEventTarget() {
return getPolymerElement().getKeyEventTarget();
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(JavaScriptObject value) {
getPolymerElement().setKeyEventTarget(value);
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public boolean getStopKeyboardEventPropagation() {
return getPolymerElement().getStopKeyboardEventPropagation();
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public void setStopKeyboardEventPropagation(boolean value) {
getPolymerElement().setStopKeyboardEventPropagation(value);
}
/**
* If true, the element will not produce a ripple effect when interacted
with via the pointer.
*
* JavaScript Info:
* @property noink
* @type Boolean
* @behavior PaperTab
*/
public boolean getNoink() {
return getPolymerElement().getNoink();
}
/**
* If true, the element will not produce a ripple effect when interacted
with via the pointer.
*
* JavaScript Info:
* @property noink
* @type Boolean
* @behavior PaperTab
*/
public void setNoink(boolean value) {
getPolymerElement().setNoink(value);
}
/**
* If true, a pin with numeric value label is shown when the slider thumb
is pressed. Use for settings for which users need to know the exact
value of the setting.
*
* JavaScript Info:
* @property pin
* @type Boolean
*
*/
public boolean getPin() {
return getPolymerElement().getPin();
}
/**
* If true, a pin with numeric value label is shown when the slider thumb
is pressed. Use for settings for which users need to know the exact
value of the setting.
*
* JavaScript Info:
* @property pin
* @type Boolean
*
*/
public void setPin(boolean value) {
getPolymerElement().setPin(value);
}
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
public boolean getActive() {
return getPolymerElement().getActive();
}
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
public void setActive(boolean value) {
getPolymerElement().setActive(value);
}
/**
* If true, the slider thumb snaps to tick marks evenly spaced based
on the step
property value.
*
* JavaScript Info:
* @property snaps
* @type Boolean
*
*/
public boolean getSnaps() {
return getPolymerElement().getSnaps();
}
/**
* If true, the slider thumb snaps to tick marks evenly spaced based
on the step
property value.
*
* JavaScript Info:
* @property snaps
* @type Boolean
*
*/
public void setSnaps(boolean value) {
getPolymerElement().setSnaps(value);
}
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
public boolean getPointerDown() {
return getPolymerElement().getPointerDown();
}
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
public void setPointerDown(boolean value) {
getPolymerElement().setPointerDown(value);
}
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
public boolean getPressed() {
return getPolymerElement().getPressed();
}
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
public void setPressed(boolean value) {
getPolymerElement().setPressed(value);
}
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
public boolean getReceivedFocusFromKeyboard() {
return getPolymerElement().getReceivedFocusFromKeyboard();
}
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
public void setReceivedFocusFromKeyboard(boolean value) {
getPolymerElement().setReceivedFocusFromKeyboard(value);
}
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
public boolean getToggles() {
return getPolymerElement().getToggles();
}
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
public void setToggles(boolean value) {
getPolymerElement().setToggles(value);
}
/**
* Set to true to mark the input as required. If used in a form, a
custom element that uses this behavior should also use
Polymer.IronValidatableBehavior and define a custom validation method.
Otherwise, a required
element will always be considered valid.
It’s also strongly recommended to provide a visual style for the element
when its value is invalid.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior VaadinDatePicker
*/
public boolean getRequired() {
return getPolymerElement().getRequired();
}
/**
* Set to true to mark the input as required. If used in a form, a
custom element that uses this behavior should also use
Polymer.IronValidatableBehavior and define a custom validation method.
Otherwise, a required
element will always be considered valid.
It’s also strongly recommended to provide a visual style for the element
when its value is invalid.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior VaadinDatePicker
*/
public void setRequired(boolean value) {
getPolymerElement().setRequired(value);
}
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
public boolean getDisabled() {
return getPolymerElement().getDisabled();
}
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
public void setDisabled(boolean value) {
getPolymerElement().setDisabled(value);
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
public boolean getFocused() {
return getPolymerElement().getFocused();
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
public void setFocused(boolean value) {
getPolymerElement().setFocused(value);
}
/**
* If true, an input is shown and user can use it to set the slider value.
*
* JavaScript Info:
* @property editable
* @type Boolean
*
*/
public boolean getEditable() {
return getPolymerElement().getEditable();
}
/**
* If true, an input is shown and user can use it to set the slider value.
*
* JavaScript Info:
* @property editable
* @type Boolean
*
*/
public void setEditable(boolean value) {
getPolymerElement().setEditable(value);
}
/**
*
*
* JavaScript Info:
* @property transiting
* @type Boolean
*
*/
public boolean getTransiting() {
return getPolymerElement().getTransiting();
}
/**
*
*
* JavaScript Info:
* @property transiting
* @type Boolean
*
*/
public void setTransiting(boolean value) {
getPolymerElement().setTransiting(value);
}
/**
* If true, the knob is expanded
*
* JavaScript Info:
* @property expand
* @type Boolean
*
*/
public boolean getExpand() {
return getPolymerElement().getExpand();
}
/**
* If true, the knob is expanded
*
* JavaScript Info:
* @property expand
* @type Boolean
*
*/
public void setExpand(boolean value) {
getPolymerElement().setExpand(value);
}
/**
* True when the user is dragging the slider.
*
* JavaScript Info:
* @property dragging
* @type Boolean
*
*/
public boolean getDragging() {
return getPolymerElement().getDragging();
}
/**
* True when the user is dragging the slider.
*
* JavaScript Info:
* @property dragging
* @type Boolean
*
*/
public void setDragging(boolean value) {
getPolymerElement().setDragging(value);
}
/**
* Specifies the value granularity of the range’s value.
*
* JavaScript Info:
* @property step
* @type Number
* @behavior PaperSlider
*/
public double getStep() {
return getPolymerElement().getStep();
}
/**
* Specifies the value granularity of the range’s value.
*
* JavaScript Info:
* @property step
* @type Number
* @behavior PaperSlider
*/
public void setStep(double value) {
getPolymerElement().setStep(value);
}
/**
* The number that represents the current value.
*
* JavaScript Info:
* @property value
* @type Number
* @behavior PaperSlider
*/
public double getValue() {
return getPolymerElement().getValue();
}
/**
* The number that represents the current value.
*
* JavaScript Info:
* @property value
* @type Number
* @behavior PaperSlider
*/
public void setValue(double value) {
getPolymerElement().setValue(value);
}
/**
* The maximum number of markers
*
* JavaScript Info:
* @property maxMarkers
* @type Number
*
*/
public double getMaxMarkers() {
return getPolymerElement().getMaxMarkers();
}
/**
* The maximum number of markers
*
* JavaScript Info:
* @property maxMarkers
* @type Number
*
*/
public void setMaxMarkers(double value) {
getPolymerElement().setMaxMarkers(value);
}
/**
* The immediate value of the slider. This value is updated while the user
is dragging the slider.
*
* JavaScript Info:
* @property immediateValue
* @type Number
*
*/
public double getImmediateValue() {
return getPolymerElement().getImmediateValue();
}
/**
* The immediate value of the slider. This value is updated while the user
is dragging the slider.
*
* JavaScript Info:
* @property immediateValue
* @type Number
*
*/
public void setImmediateValue(double value) {
getPolymerElement().setImmediateValue(value);
}
/**
* The number that represents the current secondary progress.
*
* JavaScript Info:
* @property secondaryProgress
* @type Number
*
*/
public double getSecondaryProgress() {
return getPolymerElement().getSecondaryProgress();
}
/**
* The number that represents the current secondary progress.
*
* JavaScript Info:
* @property secondaryProgress
* @type Number
*
*/
public void setSecondaryProgress(double value) {
getPolymerElement().setSecondaryProgress(value);
}
/**
* Returns the ratio of the value.
*
* JavaScript Info:
* @property ratio
* @type Number
* @behavior PaperSlider
*/
public double getRatio() {
return getPolymerElement().getRatio();
}
/**
* Returns the ratio of the value.
*
* JavaScript Info:
* @property ratio
* @type Number
* @behavior PaperSlider
*/
public void setRatio(double value) {
getPolymerElement().setRatio(value);
}
/**
* The number that indicates the maximum value of the range.
*
* JavaScript Info:
* @property max
* @type Number
* @behavior PaperSlider
*/
public double getMax() {
return getPolymerElement().getMax();
}
/**
* The number that indicates the maximum value of the range.
*
* JavaScript Info:
* @property max
* @type Number
* @behavior PaperSlider
*/
public void setMax(double value) {
getPolymerElement().setMax(value);
}
/**
* The number that indicates the minimum value of the range.
*
* JavaScript Info:
* @property min
* @type Number
* @behavior PaperSlider
*/
public double getMin() {
return getPolymerElement().getMin();
}
/**
* The number that indicates the minimum value of the range.
*
* JavaScript Info:
* @property min
* @type Number
* @behavior PaperSlider
*/
public void setMin(double value) {
getPolymerElement().setMin(value);
}
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
public String getAriaActiveAttribute() {
return getPolymerElement().getAriaActiveAttribute();
}
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
public void setAriaActiveAttribute(String value) {
getPolymerElement().setAriaActiveAttribute(value);
}
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
public String getName() {
return getPolymerElement().getName();
}
/**
* The name of this element.
*
* JavaScript Info:
* @property name
* @type String
* @behavior VaadinDatePicker
*/
public void setName(String value) {
getPolymerElement().setName(value);
}
// Needed in UIBinder
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @attribute key-bindings
* @behavior VaadinDatePicker
*/
public void setKeyBindings(String value) {
Polymer.property(this.getPolymerElement(), "keyBindings", value);
}
// Needed in UIBinder
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @attribute key-event-target
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(String value) {
Polymer.property(this.getPolymerElement(), "keyEventTarget", value);
}
// Needed in UIBinder
/**
*
*
* JavaScript Info:
* @attribute markers
*
*/
public void setMarkers(String value) {
Polymer.property(this.getPolymerElement(), "markers", value);
}
// Needed in UIBinder
/**
* The number that indicates the maximum value of the range.
*
* JavaScript Info:
* @attribute max
* @behavior PaperSlider
*/
public void setMax(String value) {
Polymer.property(this.getPolymerElement(), "max", value);
}
// Needed in UIBinder
/**
* Specifies the value granularity of the range’s value.
*
* JavaScript Info:
* @attribute step
* @behavior PaperSlider
*/
public void setStep(String value) {
Polymer.property(this.getPolymerElement(), "step", value);
}
// Needed in UIBinder
/**
* The number that represents the current value.
*
* JavaScript Info:
* @attribute value
* @behavior PaperSlider
*/
public void setValue(String value) {
Polymer.property(this.getPolymerElement(), "value", value);
}
// Needed in UIBinder
/**
* The maximum number of markers
*
* JavaScript Info:
* @attribute max-markers
*
*/
public void setMaxMarkers(String value) {
Polymer.property(this.getPolymerElement(), "maxMarkers", value);
}
// Needed in UIBinder
/**
* The immediate value of the slider. This value is updated while the user
is dragging the slider.
*
* JavaScript Info:
* @attribute immediate-value
*
*/
public void setImmediateValue(String value) {
Polymer.property(this.getPolymerElement(), "immediateValue", value);
}
// Needed in UIBinder
/**
* The number that represents the current secondary progress.
*
* JavaScript Info:
* @attribute secondary-progress
*
*/
public void setSecondaryProgress(String value) {
Polymer.property(this.getPolymerElement(), "secondaryProgress", value);
}
// Needed in UIBinder
/**
* Returns the ratio of the value.
*
* JavaScript Info:
* @attribute ratio
* @behavior PaperSlider
*/
public void setRatio(String value) {
Polymer.property(this.getPolymerElement(), "ratio", value);
}
// Needed in UIBinder
/**
* The number that indicates the minimum value of the range.
*
* JavaScript Info:
* @attribute min
* @behavior PaperSlider
*/
public void setMin(String value) {
Polymer.property(this.getPolymerElement(), "min", value);
}
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
public void addOwnKeyBinding(Object eventString, Object handlerName) {
getPolymerElement().addOwnKeyBinding(eventString, handlerName);
}
/**
* Increases value by step
but not above max
.
*
* JavaScript Info:
* @method increment
*
*
*/
public void increment() {
getPolymerElement().increment();
}
/**
* Returns true if this element currently contains a ripple effect.
*
* JavaScript Info:
* @method hasRipple
* @behavior PaperToggleButton
* @return {boolean}
*/
public boolean hasRipple() {
return getPolymerElement().hasRipple();
}
/**
* Decreases value by step
but not below min
.
*
* JavaScript Info:
* @method decrement
*
*
*/
public void decrement() {
getPolymerElement().decrement();
}
/**
* Returns the <paper-ripple>
element used by this element to create
ripple effects. The element’s ripple is created on demand, when
necessary, and calling this method will force the
ripple to be created.
*
* JavaScript Info:
* @method getRipple
* @behavior PaperToggleButton
*
*/
public void getRipple() {
getPolymerElement().getRipple();
}
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
public void removeOwnKeyBindings() {
getPolymerElement().removeOwnKeyBindings();
}
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
public boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString) {
return getPolymerElement().keyboardEventMatchesKeys(event, eventString);
}
/**
* Ensures this element contains a ripple effect. For startup efficiency
the ripple effect is dynamically on demand when needed.
*
* JavaScript Info:
* @method ensureRipple
* @param {!Event=} optTriggeringEvent
* @behavior PaperToggleButton
*
*/
public void ensureRipple(JavaScriptObject optTriggeringEvent) {
getPolymerElement().ensureRipple(optTriggeringEvent);
}
/**
* Fired when the slider’s value changes due to user interaction.
* Changes to the slider’s value due to changes in an underlying
bound variable will not trigger this event.
*
* JavaScript Info:
* @event change
*/
public HandlerRegistration addChangeHandler(ChangeEventHandler handler) {
return addDomHandler(handler, ChangeEvent.TYPE);
}
/**
* Fired when the slider’s immediateValue changes. Only occurs while the
user is dragging.
* To detect changes to immediateValue that happen for any input (i.e.
dragging, tapping, clicking, etc.) listen for immediate-value-changed
instead.
*
* JavaScript Info:
* @event immediate-value-change
*/
public HandlerRegistration addImmediateValueChangeHandler(ImmediateValueChangeEventHandler handler) {
return addDomHandler(handler, ImmediateValueChangeEvent.TYPE);
}
/**
* Fired when the slider’s value changes.
*
* JavaScript Info:
* @event value-change
*/
public HandlerRegistration addValueChangeHandler(ValueChangeEventHandler handler) {
return addDomHandler(handler, ValueChangeEvent.TYPE);
}
/**
* Fired when the element is added to an iron-form
.
*
* JavaScript Info:
* @event iron-form-element-register
*/
public HandlerRegistration addIronFormElementRegisterHandler(IronFormElementRegisterEventHandler handler) {
return addDomHandler(handler, IronFormElementRegisterEvent.TYPE);
}
/**
* Fired when the element is removed from an iron-form
.
*
* JavaScript Info:
* @event iron-form-element-unregister
*/
public HandlerRegistration addIronFormElementUnregisterHandler(IronFormElementUnregisterEventHandler handler) {
return addDomHandler(handler, IronFormElementUnregisterEvent.TYPE);
}
}