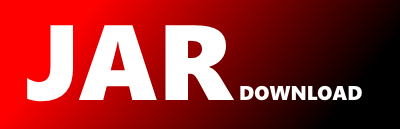
com.vaadin.polymer.paper.widget.PaperTab Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-tabs project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* paper-tab
is styled to look like a tab. It should be used in conjunction with
paper-tabs
.
* Example:
* <paper-tabs selected="0">
* <paper-tab>TAB 1</paper-tab>
* <paper-tab>TAB 2</paper-tab>
* <paper-tab>TAB 3</paper-tab>
* </paper-tabs>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-tab-ink
* Ink color
* --paper-yellow-a100
*
*
* --paper-tab
* Mixin applied to the tab
* {}
*
*
* --paper-tab-content
* Mixin applied to the tab content
* {}
*
*
* --paper-tab-content-unselected
* Mixin applied to the tab content when the tab is not selected
* {}
*
*
*
* This element applies the mixin --paper-font-common-base
but does not import paper-styles/typography.html
.
In order to apply the Roboto
font to this element, make sure you’ve imported paper-styles/typography.html
.
*/
public class PaperTab extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperTab() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperTab(String html) {
super(PaperTabElement.TAG, PaperTabElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperTabElement getPolymerElement() {
return (PaperTabElement) getElement();
}
/**
* If true, the tab will forward keyboard clicks (enter/space) to
the first anchor element found in its descendants
*
* JavaScript Info:
* @property link
* @type Boolean
*
*/
public boolean getLink() {
return getPolymerElement().getLink();
}
/**
* If true, the tab will forward keyboard clicks (enter/space) to
the first anchor element found in its descendants
*
* JavaScript Info:
* @property link
* @type Boolean
*
*/
public void setLink(boolean value) {
getPolymerElement().setLink(value);
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTab
*/
public JavaScriptObject getKeyBindings() {
return getPolymerElement().getKeyBindings();
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTab
*/
public void setKeyBindings(JavaScriptObject value) {
getPolymerElement().setKeyBindings(value);
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public JavaScriptObject getKeyEventTarget() {
return getPolymerElement().getKeyEventTarget();
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(JavaScriptObject value) {
getPolymerElement().setKeyEventTarget(value);
}
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
public boolean getDisabled() {
return getPolymerElement().getDisabled();
}
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
public void setDisabled(boolean value) {
getPolymerElement().setDisabled(value);
}
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
public boolean getPointerDown() {
return getPolymerElement().getPointerDown();
}
/**
* True if the element is currently being pressed by a “pointer,” which
is loosely defined as mouse or touch input (but specifically excluding
keyboard input).
*
* JavaScript Info:
* @property pointerDown
* @type Boolean
* @behavior PaperTab
*/
public void setPointerDown(boolean value) {
getPolymerElement().setPointerDown(value);
}
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
public boolean getPressed() {
return getPolymerElement().getPressed();
}
/**
* If true, the user is currently holding down the button.
*
* JavaScript Info:
* @property pressed
* @type Boolean
* @behavior PaperTab
*/
public void setPressed(boolean value) {
getPolymerElement().setPressed(value);
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public boolean getStopKeyboardEventPropagation() {
return getPolymerElement().getStopKeyboardEventPropagation();
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public void setStopKeyboardEventPropagation(boolean value) {
getPolymerElement().setStopKeyboardEventPropagation(value);
}
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
public boolean getReceivedFocusFromKeyboard() {
return getPolymerElement().getReceivedFocusFromKeyboard();
}
/**
* True if the input device that caused the element to receive focus
was a keyboard.
*
* JavaScript Info:
* @property receivedFocusFromKeyboard
* @type Boolean
* @behavior PaperTab
*/
public void setReceivedFocusFromKeyboard(boolean value) {
getPolymerElement().setReceivedFocusFromKeyboard(value);
}
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
public boolean getToggles() {
return getPolymerElement().getToggles();
}
/**
* If true, the button toggles the active state with each tap or press
of the spacebar.
*
* JavaScript Info:
* @property toggles
* @type Boolean
* @behavior PaperTab
*/
public void setToggles(boolean value) {
getPolymerElement().setToggles(value);
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
public boolean getFocused() {
return getPolymerElement().getFocused();
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
public void setFocused(boolean value) {
getPolymerElement().setFocused(value);
}
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
public boolean getActive() {
return getPolymerElement().getActive();
}
/**
* If true, the button is a toggle and is currently in the active state.
*
* JavaScript Info:
* @property active
* @type Boolean
* @behavior PaperTab
*/
public void setActive(boolean value) {
getPolymerElement().setActive(value);
}
/**
* If true, the element will not produce a ripple effect when interacted
with via the pointer.
*
* JavaScript Info:
* @property noink
* @type Boolean
* @behavior PaperTab
*/
public boolean getNoink() {
return getPolymerElement().getNoink();
}
/**
* If true, the element will not produce a ripple effect when interacted
with via the pointer.
*
* JavaScript Info:
* @property noink
* @type Boolean
* @behavior PaperTab
*/
public void setNoink(boolean value) {
getPolymerElement().setNoink(value);
}
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
public String getAriaActiveAttribute() {
return getPolymerElement().getAriaActiveAttribute();
}
/**
* The aria attribute to be set if the button is a toggle and in the
active state.
*
* JavaScript Info:
* @property ariaActiveAttribute
* @type String
* @behavior PaperTab
*/
public void setAriaActiveAttribute(String value) {
getPolymerElement().setAriaActiveAttribute(value);
}
// Needed in UIBinder
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @attribute key-bindings
* @behavior VaadinDatePicker
*/
public void setKeyBindings(String value) {
Polymer.property(this.getPolymerElement(), "keyBindings", value);
}
// Needed in UIBinder
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @attribute key-event-target
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(String value) {
Polymer.property(this.getPolymerElement(), "keyEventTarget", value);
}
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
public void addOwnKeyBinding(Object eventString, Object handlerName) {
getPolymerElement().addOwnKeyBinding(eventString, handlerName);
}
/**
* Returns true if this element currently contains a ripple effect.
*
* JavaScript Info:
* @method hasRipple
* @behavior PaperTab
* @return {boolean}
*/
public boolean hasRipple() {
return getPolymerElement().hasRipple();
}
/**
* Returns the <paper-ripple>
element used by this element to create
ripple effects. The element’s ripple is created on demand, when
necessary, and calling this method will force the
ripple to be created.
*
* JavaScript Info:
* @method getRipple
* @behavior PaperTab
*
*/
public void getRipple() {
getPolymerElement().getRipple();
}
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
public void removeOwnKeyBindings() {
getPolymerElement().removeOwnKeyBindings();
}
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
public boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString) {
return getPolymerElement().keyboardEventMatchesKeys(event, eventString);
}
/**
* Ensures this element contains a ripple effect. For startup efficiency
the ripple effect is dynamically on demand when needed.
*
* JavaScript Info:
* @method ensureRipple
* @param {!Event=} optTriggeringEvent
* @behavior PaperTab
*
*/
public void ensureRipple(JavaScriptObject optTriggeringEvent) {
getPolymerElement().ensureRipple(optTriggeringEvent);
}
}