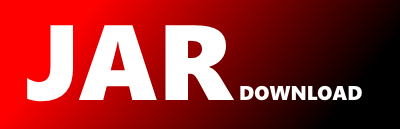
com.vaadin.polymer.paper.widget.PaperTextarea Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-input project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.*;
import com.vaadin.polymer.paper.widget.event.ChangeEvent;
import com.vaadin.polymer.paper.widget.event.ChangeEventHandler;
import com.vaadin.polymer.iron.widget.event.IronFormElementRegisterEvent;
import com.vaadin.polymer.iron.widget.event.IronFormElementRegisterEventHandler;
import com.vaadin.polymer.iron.widget.event.IronFormElementUnregisterEvent;
import com.vaadin.polymer.iron.widget.event.IronFormElementUnregisterEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* <paper-textarea>
is a multi-line text field with Material Design styling.
* <paper-textarea label="Textarea label"></paper-textarea>
*
*
*
See Polymer.PaperInputBehavior
for more API docs.
* Validation
* Currently only required
and maxlength
validation is supported.
* Styling
* See Polymer.PaperInputContainer
for a list of custom properties used to
style this element.
*/
public class PaperTextarea extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperTextarea() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperTextarea(String html) {
super(PaperTextareaElement.TAG, PaperTextareaElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperTextareaElement getPolymerElement() {
return (PaperTextareaElement) getElement();
}
/**
* The maximum number of rows this element can grow to until it
scrolls. 0 means no maximum.
*
* JavaScript Info:
* @property maxRows
* @type Number
*
*/
public double getMaxRows() {
return getPolymerElement().getMaxRows();
}
/**
* The maximum number of rows this element can grow to until it
scrolls. 0 means no maximum.
*
* JavaScript Info:
* @property maxRows
* @type Number
*
*/
public void setMaxRows(double value) {
getPolymerElement().setMaxRows(value);
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public JavaScriptObject getKeyEventTarget() {
return getPolymerElement().getKeyEventTarget();
}
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @property keyEventTarget
* @type ?EventTarget
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(JavaScriptObject value) {
getPolymerElement().setKeyEventTarget(value);
}
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @property keyBindings
* @type !Object
* @behavior VaadinDatePicker
*/
public JavaScriptObject getKeyBindings() {
return getPolymerElement().getKeyBindings();
}
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @property keyBindings
* @type !Object
* @behavior VaadinDatePicker
*/
public void setKeyBindings(JavaScriptObject value) {
getPolymerElement().setKeyBindings(value);
}
/**
* Set to true to show a character counter.
*
* JavaScript Info:
* @property charCounter
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getCharCounter() {
return getPolymerElement().getCharCounter();
}
/**
* Set to true to show a character counter.
*
* JavaScript Info:
* @property charCounter
* @type Boolean
* @behavior PaperTextarea
*/
public void setCharCounter(boolean value) {
getPolymerElement().setCharCounter(value);
}
/**
* Set to true to disable this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
both the <paper-input-container>
‘s and the input’s disabled
property.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getDisabled() {
return getPolymerElement().getDisabled();
}
/**
* Set to true to disable this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
both the <paper-input-container>
‘s and the input’s disabled
property.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTextarea
*/
public void setDisabled(boolean value) {
getPolymerElement().setDisabled(value);
}
/**
* Set to true to mark the input as required. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s required
property.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getRequired() {
return getPolymerElement().getRequired();
}
/**
* Set to true to mark the input as required. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s required
property.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior PaperTextarea
*/
public void setRequired(boolean value) {
getPolymerElement().setRequired(value);
}
/**
* Returns true if the value is invalid. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to both the
<paper-input-container>
‘s and the input’s invalid
property.
* If autoValidate
is true, the invalid
attribute is managed automatically,
which can clobber attempts to manage it manually.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getInvalid() {
return getPolymerElement().getInvalid();
}
/**
* Returns true if the value is invalid. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to both the
<paper-input-container>
‘s and the input’s invalid
property.
* If autoValidate
is true, the invalid
attribute is managed automatically,
which can clobber attempts to manage it manually.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior PaperTextarea
*/
public void setInvalid(boolean value) {
getPolymerElement().setInvalid(value);
}
/**
* Set to true to always float the label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getAlwaysFloatLabel() {
return getPolymerElement().getAlwaysFloatLabel();
}
/**
* Set to true to always float the label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
* @behavior PaperTextarea
*/
public void setAlwaysFloatLabel(boolean value) {
getPolymerElement().setAlwaysFloatLabel(value);
}
/**
* Set to true to auto-validate the input value. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s autoValidate
property.
*
* JavaScript Info:
* @property autoValidate
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getAutoValidate() {
return getPolymerElement().getAutoValidate();
}
/**
* Set to true to auto-validate the input value. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s autoValidate
property.
*
* JavaScript Info:
* @property autoValidate
* @type Boolean
* @behavior PaperTextarea
*/
public void setAutoValidate(boolean value) {
getPolymerElement().setAutoValidate(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the<input is="iron-input">
‘s multiple
property,
used with type=file.
*
* JavaScript Info:
* @property multiple
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getMultiple() {
return getPolymerElement().getMultiple();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the<input is="iron-input">
‘s multiple
property,
used with type=file.
*
* JavaScript Info:
* @property multiple
* @type Boolean
* @behavior PaperTextarea
*/
public void setMultiple(boolean value) {
getPolymerElement().setMultiple(value);
}
/**
* Set to true to disable the floating label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getNoLabelFloat() {
return getPolymerElement().getNoLabelFloat();
}
/**
* Set to true to disable the floating label. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <paper-input-container>
‘s noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
* @behavior PaperTextarea
*/
public void setNoLabelFloat(boolean value) {
getPolymerElement().setNoLabelFloat(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autofocus
property.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getAutofocus() {
return getPolymerElement().getAutofocus();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autofocus
property.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
* @behavior PaperTextarea
*/
public void setAutofocus(boolean value) {
getPolymerElement().setAutofocus(value);
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public boolean getStopKeyboardEventPropagation() {
return getPolymerElement().getStopKeyboardEventPropagation();
}
/**
* If true, this property will cause the implementing element to
automatically stop propagation on any handled KeyboardEvents.
*
* JavaScript Info:
* @property stopKeyboardEventPropagation
* @type Boolean
* @behavior VaadinDatePicker
*/
public void setStopKeyboardEventPropagation(boolean value) {
getPolymerElement().setStopKeyboardEventPropagation(value);
}
/**
* Set to true to prevent the user from entering invalid input. If you’re
using PaperInputBehavior to implement your own paper-input-like element,
bind this to <input is="iron-input">
‘s preventInvalidInput
property.
*
* JavaScript Info:
* @property preventInvalidInput
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getPreventInvalidInput() {
return getPolymerElement().getPreventInvalidInput();
}
/**
* Set to true to prevent the user from entering invalid input. If you’re
using PaperInputBehavior to implement your own paper-input-like element,
bind this to <input is="iron-input">
‘s preventInvalidInput
property.
*
* JavaScript Info:
* @property preventInvalidInput
* @type Boolean
* @behavior PaperTextarea
*/
public void setPreventInvalidInput(boolean value) {
getPolymerElement().setPreventInvalidInput(value);
}
/**
* The maximum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s maxlength
property.
*
* JavaScript Info:
* @property maxlength
* @type Number
* @behavior PaperTextarea
*/
public double getMaxlength() {
return getPolymerElement().getMaxlength();
}
/**
* The maximum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s maxlength
property.
*
* JavaScript Info:
* @property maxlength
* @type Number
* @behavior PaperTextarea
*/
public void setMaxlength(double value) {
getPolymerElement().setMaxlength(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @property size
* @type Number
* @behavior PaperTextarea
*/
public double getSize() {
return getPolymerElement().getSize();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @property size
* @type Number
* @behavior PaperTextarea
*/
public void setSize(double value) {
getPolymerElement().setSize(value);
}
/**
* The initial number of rows.
*
* JavaScript Info:
* @property rows
* @type Number
*
*/
public double getRows() {
return getPolymerElement().getRows();
}
/**
* The initial number of rows.
*
* JavaScript Info:
* @property rows
* @type Number
*
*/
public void setRows(double value) {
getPolymerElement().setRows(value);
}
/**
* The minimum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @property minlength
* @type Number
* @behavior PaperTextarea
*/
public double getMinlength() {
return getPolymerElement().getMinlength();
}
/**
* The minimum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @property minlength
* @type Number
* @behavior PaperTextarea
*/
public void setMinlength(double value) {
getPolymerElement().setMinlength(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s results
property,
used with type=search.
*
* JavaScript Info:
* @property results
* @type Number
* @behavior PaperTextarea
*/
public double getResults() {
return getPolymerElement().getResults();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s results
property,
used with type=search.
*
* JavaScript Info:
* @property results
* @type Number
* @behavior PaperTextarea
*/
public void setResults(double value) {
getPolymerElement().setResults(value);
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
public boolean getFocused() {
return getPolymerElement().getFocused();
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
public void setFocused(boolean value) {
getPolymerElement().setFocused(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s readonly
property.
*
* JavaScript Info:
* @property readonly
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getReadonly() {
return getPolymerElement().getReadonly();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s readonly
property.
*
* JavaScript Info:
* @property readonly
* @type Boolean
* @behavior PaperTextarea
*/
public void setReadonly(boolean value) {
getPolymerElement().setReadonly(value);
}
/**
* The type of the input. The supported types are text
, number
and password
.
If you’re using PaperInputBehavior to implement your own paper-input-like element,
bind this to the <input is="iron-input">
‘s type
property.
*
* JavaScript Info:
* @property type
* @type String
* @behavior PaperTextarea
*/
public String getType() {
return getPolymerElement().getType();
}
/**
* The type of the input. The supported types are text
, number
and password
.
If you’re using PaperInputBehavior to implement your own paper-input-like element,
bind this to the <input is="iron-input">
‘s type
property.
*
* JavaScript Info:
* @property type
* @type String
* @behavior PaperTextarea
*/
public void setType(String value) {
getPolymerElement().setType(value);
}
/**
* The value for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s bindValue
property, or the value property of your input that is notify:true
.
*
* JavaScript Info:
* @property value
* @type String
* @behavior PaperTextarea
*/
public String getValue() {
return getPolymerElement().getValue();
}
/**
* The value for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s bindValue
property, or the value property of your input that is notify:true
.
*
* JavaScript Info:
* @property value
* @type String
* @behavior PaperTextarea
*/
public void setValue(String value) {
getPolymerElement().setValue(value);
}
/**
* Limits the numeric or date-time increments.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s step
property.
*
* JavaScript Info:
* @property step
* @type String
* @behavior PaperTextarea
*/
public String getStep() {
return getPolymerElement().getStep();
}
/**
* Limits the numeric or date-time increments.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s step
property.
*
* JavaScript Info:
* @property step
* @type String
* @behavior PaperTextarea
*/
public void setStep(String value) {
getPolymerElement().setStep(value);
}
/**
* A placeholder string in addition to the label. If this is set, the label will always float.
*
* JavaScript Info:
* @property placeholder
* @type String
* @behavior PaperTextarea
*/
public String getPlaceholder() {
return getPolymerElement().getPlaceholder();
}
/**
* A placeholder string in addition to the label. If this is set, the label will always float.
*
* JavaScript Info:
* @property placeholder
* @type String
* @behavior PaperTextarea
*/
public void setPlaceholder(String value) {
getPolymerElement().setPlaceholder(value);
}
/**
* A pattern to validate the input
with. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s pattern
property.
*
* JavaScript Info:
* @property pattern
* @type String
* @behavior PaperTextarea
*/
public String getPattern() {
return getPolymerElement().getPattern();
}
/**
* A pattern to validate the input
with. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s pattern
property.
*
* JavaScript Info:
* @property pattern
* @type String
* @behavior PaperTextarea
*/
public void setPattern(String value) {
getPolymerElement().setPattern(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s name
property.
*
* JavaScript Info:
* @property name
* @type String
* @behavior PaperTextarea
*/
public String getName() {
return getPolymerElement().getName();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s name
property.
*
* JavaScript Info:
* @property name
* @type String
* @behavior PaperTextarea
*/
public void setName(String value) {
getPolymerElement().setName(value);
}
/**
* The minimum (numeric or date-time) input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s min
property.
*
* JavaScript Info:
* @property min
* @type String
* @behavior PaperTextarea
*/
public String getMin() {
return getPolymerElement().getMin();
}
/**
* The minimum (numeric or date-time) input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s min
property.
*
* JavaScript Info:
* @property min
* @type String
* @behavior PaperTextarea
*/
public void setMin(String value) {
getPolymerElement().setMin(value);
}
/**
* The maximum (numeric or date-time) input value.
Can be a String (e.g. "2000-01-01"
) or a Number (e.g. 2
).
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s max
property.
*
* JavaScript Info:
* @property max
* @type String
* @behavior PaperTextarea
*/
public String getMax() {
return getPolymerElement().getMax();
}
/**
* The maximum (numeric or date-time) input value.
Can be a String (e.g. "2000-01-01"
) or a Number (e.g. 2
).
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s max
property.
*
* JavaScript Info:
* @property max
* @type String
* @behavior PaperTextarea
*/
public void setMax(String value) {
getPolymerElement().setMax(value);
}
/**
* The datalist of the input (if any). This should match the id of an existing <datalist>
.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s list
property.
*
* JavaScript Info:
* @property list
* @type String
* @behavior PaperTextarea
*/
public String getList() {
return getPolymerElement().getList();
}
/**
* The datalist of the input (if any). This should match the id of an existing <datalist>
.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s list
property.
*
* JavaScript Info:
* @property list
* @type String
* @behavior PaperTextarea
*/
public void setList(String value) {
getPolymerElement().setList(value);
}
/**
* The label for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
<label>
‘s content and hidden
property, e.g.
<label hidden$="[[!label]]">[[label]]</label>
in your template
*
* JavaScript Info:
* @property label
* @type String
* @behavior PaperTextarea
*/
public String getLabel() {
return getPolymerElement().getLabel();
}
/**
* The label for this input. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
<label>
‘s content and hidden
property, e.g.
<label hidden$="[[!label]]">[[label]]</label>
in your template
*
* JavaScript Info:
* @property label
* @type String
* @behavior PaperTextarea
*/
public void setLabel(String value) {
getPolymerElement().setLabel(value);
}
/**
* Name of the validator to use. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s validator
property.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior PaperTextarea
*/
public String getValidator() {
return getPolymerElement().getValidator();
}
/**
* Name of the validator to use. If you’re using PaperInputBehavior to
implement your own paper-input-like element, bind this to
the <input is="iron-input">
‘s validator
property.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior PaperTextarea
*/
public void setValidator(String value) {
getPolymerElement().setValidator(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s accept
property,
used with type=file.
*
* JavaScript Info:
* @property accept
* @type String
* @behavior PaperTextarea
*/
public String getAccept() {
return getPolymerElement().getAccept();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s accept
property,
used with type=file.
*
* JavaScript Info:
* @property accept
* @type String
* @behavior PaperTextarea
*/
public void setAccept(String value) {
getPolymerElement().setAccept(value);
}
/**
* The error message to display when the input is invalid. If you’re using
PaperInputBehavior to implement your own paper-input-like element,
bind this to the <paper-input-error>
‘s content, if using.
*
* JavaScript Info:
* @property errorMessage
* @type String
* @behavior PaperTextarea
*/
public String getErrorMessage() {
return getPolymerElement().getErrorMessage();
}
/**
* The error message to display when the input is invalid. If you’re using
PaperInputBehavior to implement your own paper-input-like element,
bind this to the <paper-input-error>
‘s content, if using.
*
* JavaScript Info:
* @property errorMessage
* @type String
* @behavior PaperTextarea
*/
public void setErrorMessage(String value) {
getPolymerElement().setErrorMessage(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autosave
property,
used with type=search.
*
* JavaScript Info:
* @property autosave
* @type String
* @behavior PaperTextarea
*/
public String getAutosave() {
return getPolymerElement().getAutosave();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autosave
property,
used with type=search.
*
* JavaScript Info:
* @property autosave
* @type String
* @behavior PaperTextarea
*/
public void setAutosave(String value) {
getPolymerElement().setAutosave(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocorrect
property.
*
* JavaScript Info:
* @property autocorrect
* @type String
* @behavior PaperTextarea
*/
public String getAutocorrect() {
return getPolymerElement().getAutocorrect();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocorrect
property.
*
* JavaScript Info:
* @property autocorrect
* @type String
* @behavior PaperTextarea
*/
public void setAutocorrect(String value) {
getPolymerElement().setAutocorrect(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocomplete
property.
*
* JavaScript Info:
* @property autocomplete
* @type String
* @behavior PaperTextarea
*/
public String getAutocomplete() {
return getPolymerElement().getAutocomplete();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocomplete
property.
*
* JavaScript Info:
* @property autocomplete
* @type String
* @behavior PaperTextarea
*/
public void setAutocomplete(String value) {
getPolymerElement().setAutocomplete(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocapitalize
property.
*
* JavaScript Info:
* @property autocapitalize
* @type String
* @behavior PaperTextarea
*/
public String getAutocapitalize() {
return getPolymerElement().getAutocapitalize();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s autocapitalize
property.
*
* JavaScript Info:
* @property autocapitalize
* @type String
* @behavior PaperTextarea
*/
public void setAutocapitalize(String value) {
getPolymerElement().setAutocapitalize(value);
}
/**
* Set this to specify the pattern allowed by preventInvalidInput
. If
you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s allowedPattern
property.
*
* JavaScript Info:
* @property allowedPattern
* @type String
* @behavior PaperTextarea
*/
public String getAllowedPattern() {
return getPolymerElement().getAllowedPattern();
}
/**
* Set this to specify the pattern allowed by preventInvalidInput
. If
you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s allowedPattern
property.
*
* JavaScript Info:
* @property allowedPattern
* @type String
* @behavior PaperTextarea
*/
public void setAllowedPattern(String value) {
getPolymerElement().setAllowedPattern(value);
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s inputmode
property.
*
* JavaScript Info:
* @property inputmode
* @type String
* @behavior PaperTextarea
*/
public String getInputmode() {
return getPolymerElement().getInputmode();
}
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s inputmode
property.
*
* JavaScript Info:
* @property inputmode
* @type String
* @behavior PaperTextarea
*/
public void setInputmode(String value) {
getPolymerElement().setInputmode(value);
}
// Needed in UIBinder
/**
* The maximum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s maxlength
property.
*
* JavaScript Info:
* @attribute maxlength
* @behavior PaperTextarea
*/
public void setMaxlength(String value) {
Polymer.property(this.getPolymerElement(), "maxlength", value);
}
// Needed in UIBinder
/**
* To be used to express what combination of keys will trigger the relative
callback. e.g. keyBindings: { 'esc': '_onEscPressed'}
*
* JavaScript Info:
* @attribute key-bindings
* @behavior VaadinDatePicker
*/
public void setKeyBindings(String value) {
Polymer.property(this.getPolymerElement(), "keyBindings", value);
}
// Needed in UIBinder
/**
* The maximum number of rows this element can grow to until it
scrolls. 0 means no maximum.
*
* JavaScript Info:
* @attribute max-rows
*
*/
public void setMaxRows(String value) {
Polymer.property(this.getPolymerElement(), "maxRows", value);
}
// Needed in UIBinder
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @attribute size
* @behavior PaperTextarea
*/
public void setSize(String value) {
Polymer.property(this.getPolymerElement(), "size", value);
}
// Needed in UIBinder
/**
* The initial number of rows.
*
* JavaScript Info:
* @attribute rows
*
*/
public void setRows(String value) {
Polymer.property(this.getPolymerElement(), "rows", value);
}
// Needed in UIBinder
/**
* The minimum length of the input value.
If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @attribute minlength
* @behavior PaperTextarea
*/
public void setMinlength(String value) {
Polymer.property(this.getPolymerElement(), "minlength", value);
}
// Needed in UIBinder
/**
* If you’re using PaperInputBehavior to implement your own paper-input-like
element, bind this to the <input is="iron-input">
‘s results
property,
used with type=search.
*
* JavaScript Info:
* @attribute results
* @behavior PaperTextarea
*/
public void setResults(String value) {
Polymer.property(this.getPolymerElement(), "results", value);
}
// Needed in UIBinder
/**
* The EventTarget that will be firing relevant KeyboardEvents. Set it to
null
to disable the listeners.
*
* JavaScript Info:
* @attribute key-event-target
* @behavior VaadinDatePicker
*/
public void setKeyEventTarget(String value) {
Polymer.property(this.getPolymerElement(), "keyEventTarget", value);
}
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior VaadinDatePicker
*
*/
public void addOwnKeyBinding(Object eventString, Object handlerName) {
getPolymerElement().addOwnKeyBinding(eventString, handlerName);
}
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior VaadinDatePicker
*
*/
public void removeOwnKeyBindings() {
getPolymerElement().removeOwnKeyBindings();
}
/**
* Validates the input element and sets an error style if needed.
*
* JavaScript Info:
* @method validate
* @behavior PaperTextarea
* @return {boolean}
*/
public boolean validate() {
return getPolymerElement().validate();
}
/**
* Returns true if a keyboard event matches eventString
.
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {KeyboardEvent} event
* @param {string} eventString
* @behavior VaadinDatePicker
* @return {boolean}
*/
public boolean keyboardEventMatchesKeys(JavaScriptObject event, String eventString) {
return getPolymerElement().keyboardEventMatchesKeys(event, eventString);
}
/**
* Restores the cursor to its original position after updating the value.
*
* JavaScript Info:
* @method updateValueAndPreserveCaret
* @param {string} newValue
* @behavior PaperTextarea
*
*/
public void updateValueAndPreserveCaret(String newValue) {
getPolymerElement().updateValueAndPreserveCaret(newValue);
}
/**
* Fired when the input changes due to user interaction.
*
* JavaScript Info:
* @event change
*/
public HandlerRegistration addChangeHandler(ChangeEventHandler handler) {
return addDomHandler(handler, ChangeEvent.TYPE);
}
/**
* Fired when the element is added to an iron-form
.
*
* JavaScript Info:
* @event iron-form-element-register
*/
public HandlerRegistration addIronFormElementRegisterHandler(IronFormElementRegisterEventHandler handler) {
return addDomHandler(handler, IronFormElementRegisterEvent.TYPE);
}
/**
* Fired when the element is removed from an iron-form
.
*
* JavaScript Info:
* @event iron-form-element-unregister
*/
public HandlerRegistration addIronFormElementUnregisterHandler(IronFormElementUnregisterEventHandler handler) {
return addDomHandler(handler, IronFormElementUnregisterEvent.TYPE);
}
}