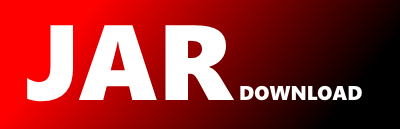
com.vaadin.polymer.paper.widget.PaperToast Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-toast project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.*;
import com.vaadin.polymer.paper.widget.event.IronAnnounceEvent;
import com.vaadin.polymer.paper.widget.event.IronAnnounceEventHandler;
import com.vaadin.polymer.iron.widget.event.IronOverlayCanceledEvent;
import com.vaadin.polymer.iron.widget.event.IronOverlayCanceledEventHandler;
import com.vaadin.polymer.iron.widget.event.IronOverlayClosedEvent;
import com.vaadin.polymer.iron.widget.event.IronOverlayClosedEventHandler;
import com.vaadin.polymer.iron.widget.event.IronOverlayOpenedEvent;
import com.vaadin.polymer.iron.widget.event.IronOverlayOpenedEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Material design: Snackbars & toasts
* paper-toast
provides a subtle notification toast. Only one paper-toast
will
be visible on screen.
* Use opened
to show the toast:
* Example:
* <paper-toast text="Hello world!" opened></paper-toast>
*
*
*
Also open()
or show()
can be used to show the toast:
* Example:
* <paper-button on-click="openToast">Open Toast</paper-button>
* <paper-toast id="toast" text="Hello world!"></paper-toast>
*
* ...
*
* openToast: function() {
* this.$.toast.open();
* }
*
*
*
Set duration
to 0, a negative number or Infinity to persist the toast on screen:
* Example:
* <paper-toast text="Terms and conditions" opened duration="0">
* <a href="#">Show more</a>
* </paper-toast>
*
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-toast-background-color
* The paper-toast background-color
* #323232
*
*
* --paper-toast-color
* The paper-toast color
* #f1f1f1
*
*
*
* This element applies the mixin --paper-font-common-base
but does not import paper-styles/typography.html
.
In order to apply the Roboto
font to this element, make sure you’ve imported paper-styles/typography.html
.
*/
public class PaperToast extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperToast() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperToast(String html) {
super(PaperToastElement.TAG, PaperToastElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperToastElement getPolymerElement() {
return (PaperToastElement) getElement();
}
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by horizontalAlign
.
* If horizontalAlign
is “left”, this offset will increase or decrease
the distance to the left side of the screen: a negative offset will
move the dropdown to the left; a positive one, to the right.
* Conversely if horizontalAlign
is “right”, this offset will increase
or decrease the distance to the right side of the screen: a negative
offset will move the dropdown to the right; a positive one, to the left.
*
* JavaScript Info:
* @property horizontalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
public double getHorizontalOffset() {
return getPolymerElement().getHorizontalOffset();
}
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by horizontalAlign
.
* If horizontalAlign
is “left”, this offset will increase or decrease
the distance to the left side of the screen: a negative offset will
move the dropdown to the left; a positive one, to the right.
* Conversely if horizontalAlign
is “right”, this offset will increase
or decrease the distance to the right side of the screen: a negative
offset will move the dropdown to the right; a positive one, to the left.
*
* JavaScript Info:
* @property horizontalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
public void setHorizontalOffset(double value) {
getPolymerElement().setHorizontalOffset(value);
}
/**
* The backdrop element.
*
* JavaScript Info:
* @property backdropElement
* @type Element
* @behavior VaadinContextMenuOverlay
*/
public Element getBackdropElement() {
return getPolymerElement().getBackdropElement();
}
/**
* The backdrop element.
*
* JavaScript Info:
* @property backdropElement
* @type Element
* @behavior VaadinContextMenuOverlay
*/
public void setBackdropElement(Element value) {
getPolymerElement().setBackdropElement(value);
}
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by verticalAlign
.
* If verticalAlign
is “top”, this offset will increase or decrease
the distance to the top side of the screen: a negative offset will
move the dropdown upwards; a positive one, downwards.
* Conversely if verticalAlign
is “bottom”, this offset will increase
or decrease the distance to the bottom side of the screen: a negative
offset will move the dropdown downwards; a positive one, upwards.
*
* JavaScript Info:
* @property verticalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
public double getVerticalOffset() {
return getPolymerElement().getVerticalOffset();
}
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by verticalAlign
.
* If verticalAlign
is “top”, this offset will increase or decrease
the distance to the top side of the screen: a negative offset will
move the dropdown upwards; a positive one, downwards.
* Conversely if verticalAlign
is “bottom”, this offset will increase
or decrease the distance to the bottom side of the screen: a negative
offset will move the dropdown downwards; a positive one, upwards.
*
* JavaScript Info:
* @property verticalOffset
* @type Number
* @behavior VaadinContextMenuOverlay
*/
public void setVerticalOffset(double value) {
getPolymerElement().setVerticalOffset(value);
}
/**
* The duration in milliseconds to show the toast.
Set to 0
, a negative number, or Infinity
, to disable the
toast auto-closing.
*
* JavaScript Info:
* @property duration
* @type Number
*
*/
public double getDuration() {
return getPolymerElement().getDuration();
}
/**
* The duration in milliseconds to show the toast.
Set to 0
, a negative number, or Infinity
, to disable the
toast auto-closing.
*
* JavaScript Info:
* @property duration
* @type Number
*
*/
public void setDuration(double value) {
getPolymerElement().setDuration(value);
}
/**
* Set to true to keep overlay always on top.
*
* JavaScript Info:
* @property alwaysOnTop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getAlwaysOnTop() {
return getPolymerElement().getAlwaysOnTop();
}
/**
* Set to true to keep overlay always on top.
*
* JavaScript Info:
* @property alwaysOnTop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setAlwaysOnTop(boolean value) {
getPolymerElement().setAlwaysOnTop(value);
}
/**
* True if the overlay is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getOpened() {
return getPolymerElement().getOpened();
}
/**
* True if the overlay is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setOpened(boolean value) {
getPolymerElement().setOpened(value);
}
/**
* Set to true to enable restoring of focus when overlay is closed.
*
* JavaScript Info:
* @property restoreFocusOnClose
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getRestoreFocusOnClose() {
return getPolymerElement().getRestoreFocusOnClose();
}
/**
* Set to true to enable restoring of focus when overlay is closed.
*
* JavaScript Info:
* @property restoreFocusOnClose
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setRestoreFocusOnClose(boolean value) {
getPolymerElement().setRestoreFocusOnClose(value);
}
/**
* Set to true to display a backdrop behind the overlay. It traps the focus
within the light DOM of the overlay.
*
* JavaScript Info:
* @property withBackdrop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getWithBackdrop() {
return getPolymerElement().getWithBackdrop();
}
/**
* Set to true to display a backdrop behind the overlay. It traps the focus
within the light DOM of the overlay.
*
* JavaScript Info:
* @property withBackdrop
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setWithBackdrop(boolean value) {
getPolymerElement().setWithBackdrop(value);
}
/**
* Overridden from IronOverlayBehavior
.
Set to true to disable auto-focusing the toast or child nodes with
the autofocus
attribute` when the overlay is opened.
*
* JavaScript Info:
* @property noAutoFocus
* @type Boolean
*
*/
public boolean getNoAutoFocus() {
return getPolymerElement().getNoAutoFocus();
}
/**
* Overridden from IronOverlayBehavior
.
Set to true to disable auto-focusing the toast or child nodes with
the autofocus
attribute` when the overlay is opened.
*
* JavaScript Info:
* @property noAutoFocus
* @type Boolean
*
*/
public void setNoAutoFocus(boolean value) {
getPolymerElement().setNoAutoFocus(value);
}
/**
* True if the overlay was canceled when it was last closed.
*
* JavaScript Info:
* @property canceled
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getCanceled() {
return getPolymerElement().getCanceled();
}
/**
* True if the overlay was canceled when it was last closed.
*
* JavaScript Info:
* @property canceled
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setCanceled(boolean value) {
getPolymerElement().setCanceled(value);
}
/**
* Set to true to auto-fit on attach.
*
* JavaScript Info:
* @property autoFitOnAttach
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getAutoFitOnAttach() {
return getPolymerElement().getAutoFitOnAttach();
}
/**
* Set to true to auto-fit on attach.
*
* JavaScript Info:
* @property autoFitOnAttach
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setAutoFitOnAttach(boolean value) {
getPolymerElement().setAutoFitOnAttach(value);
}
/**
* Overridden from IronOverlayBehavior
.
Set to false to enable closing of the toast by clicking outside it.
*
* JavaScript Info:
* @property noCancelOnOutsideClick
* @type Boolean
*
*/
public boolean getNoCancelOnOutsideClick() {
return getPolymerElement().getNoCancelOnOutsideClick();
}
/**
* Overridden from IronOverlayBehavior
.
Set to false to enable closing of the toast by clicking outside it.
*
* JavaScript Info:
* @property noCancelOnOutsideClick
* @type Boolean
*
*/
public void setNoCancelOnOutsideClick(boolean value) {
getPolymerElement().setNoCancelOnOutsideClick(value);
}
/**
* If true, it will use horizontalAlign
and verticalAlign
values as preferred alignment
and if there’s not enough space, it will pick the values which minimize the cropping.
*
* JavaScript Info:
* @property dynamicAlign
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getDynamicAlign() {
return getPolymerElement().getDynamicAlign();
}
/**
* If true, it will use horizontalAlign
and verticalAlign
values as preferred alignment
and if there’s not enough space, it will pick the values which minimize the cropping.
*
* JavaScript Info:
* @property dynamicAlign
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setDynamicAlign(boolean value) {
getPolymerElement().setDynamicAlign(value);
}
/**
* Set to true to disable canceling the overlay with the ESC key.
*
* JavaScript Info:
* @property noCancelOnEscKey
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getNoCancelOnEscKey() {
return getPolymerElement().getNoCancelOnEscKey();
}
/**
* Set to true to disable canceling the overlay with the ESC key.
*
* JavaScript Info:
* @property noCancelOnEscKey
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setNoCancelOnEscKey(boolean value) {
getPolymerElement().setNoCancelOnEscKey(value);
}
/**
* Will position the element around the positionTarget without overlapping it.
*
* JavaScript Info:
* @property noOverlap
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public boolean getNoOverlap() {
return getPolymerElement().getNoOverlap();
}
/**
* Will position the element around the positionTarget without overlapping it.
*
* JavaScript Info:
* @property noOverlap
* @type Boolean
* @behavior VaadinContextMenuOverlay
*/
public void setNoOverlap(boolean value) {
getPolymerElement().setNoOverlap(value);
}
/**
* The element that should be used to position the element. If not set, it will
default to the parent node.
*
* JavaScript Info:
* @property positionTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
public JavaScriptObject getPositionTarget() {
return getPolymerElement().getPositionTarget();
}
/**
* The element that should be used to position the element. If not set, it will
default to the parent node.
*
* JavaScript Info:
* @property positionTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
public void setPositionTarget(JavaScriptObject value) {
getPolymerElement().setPositionTarget(value);
}
/**
* Contains the reason(s) this overlay was last closed (see iron-overlay-closed
).
IronOverlayBehavior
provides the canceled
reason; implementers of the
behavior can provide other reasons in addition to canceled
.
*
* JavaScript Info:
* @property closingReason
* @type Object
* @behavior VaadinContextMenuOverlay
*/
public JavaScriptObject getClosingReason() {
return getPolymerElement().getClosingReason();
}
/**
* Contains the reason(s) this overlay was last closed (see iron-overlay-closed
).
IronOverlayBehavior
provides the canceled
reason; implementers of the
behavior can provide other reasons in addition to canceled
.
*
* JavaScript Info:
* @property closingReason
* @type Object
* @behavior VaadinContextMenuOverlay
*/
public void setClosingReason(JavaScriptObject value) {
getPolymerElement().setClosingReason(value);
}
/**
* The element to fit this
into.
*
* JavaScript Info:
* @property fitInto
* @type Object
* @behavior VaadinContextMenuOverlay
*/
public JavaScriptObject getFitInto() {
return getPolymerElement().getFitInto();
}
/**
* The element to fit this
into.
*
* JavaScript Info:
* @property fitInto
* @type Object
* @behavior VaadinContextMenuOverlay
*/
public void setFitInto(JavaScriptObject value) {
getPolymerElement().setFitInto(value);
}
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @property sizingTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
public JavaScriptObject getSizingTarget() {
return getPolymerElement().getSizingTarget();
}
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @property sizingTarget
* @type !Element
* @behavior VaadinContextMenuOverlay
*/
public void setSizingTarget(JavaScriptObject value) {
getPolymerElement().setSizingTarget(value);
}
/**
* The orientation against which to align the element vertically
relative to the positionTarget
. Possible values are “top”, “bottom”, “auto”.
*
* JavaScript Info:
* @property verticalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
public String getVerticalAlign() {
return getPolymerElement().getVerticalAlign();
}
/**
* The orientation against which to align the element vertically
relative to the positionTarget
. Possible values are “top”, “bottom”, “auto”.
*
* JavaScript Info:
* @property verticalAlign
* @type String
* @behavior VaadinContextMenuOverlay
*/
public void setVerticalAlign(String value) {
getPolymerElement().setVerticalAlign(value);
}
/**
* The text to display in the toast.
*
* JavaScript Info:
* @property text
* @type String
*
*/
public String getText() {
return getPolymerElement().getText();
}
/**
* The text to display in the toast.
*
* JavaScript Info:
* @property text
* @type String
*
*/
public void setText(String value) {
getPolymerElement().setText(value);
}
/**
* The orientation against which to align the dropdown content
horizontally relative to positionTarget
.
Overridden from Polymer.IronFitBehavior
.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
*
*/
public String getHorizontalAlign() {
return getPolymerElement().getHorizontalAlign();
}
/**
* The orientation against which to align the dropdown content
horizontally relative to positionTarget
.
Overridden from Polymer.IronFitBehavior
.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
*
*/
public void setHorizontalAlign(String value) {
getPolymerElement().setHorizontalAlign(value);
}
// Needed in UIBinder
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by horizontalAlign
.
* If horizontalAlign
is “left”, this offset will increase or decrease
the distance to the left side of the screen: a negative offset will
move the dropdown to the left; a positive one, to the right.
* Conversely if horizontalAlign
is “right”, this offset will increase
or decrease the distance to the right side of the screen: a negative
offset will move the dropdown to the right; a positive one, to the left.
*
* JavaScript Info:
* @attribute horizontal-offset
* @behavior VaadinContextMenuOverlay
*/
public void setHorizontalOffset(String value) {
Polymer.property(this.getPolymerElement(), "horizontalOffset", value);
}
// Needed in UIBinder
/**
* The backdrop element.
*
* JavaScript Info:
* @attribute backdrop-element
* @behavior VaadinContextMenuOverlay
*/
public void setBackdropElement(String value) {
Polymer.property(this.getPolymerElement(), "backdropElement", value);
}
// Needed in UIBinder
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
, in the direction of alignment. You can think
of it as increasing or decreasing the distance to the side of the
screen given by verticalAlign
.
* If verticalAlign
is “top”, this offset will increase or decrease
the distance to the top side of the screen: a negative offset will
move the dropdown upwards; a positive one, downwards.
* Conversely if verticalAlign
is “bottom”, this offset will increase
or decrease the distance to the bottom side of the screen: a negative
offset will move the dropdown downwards; a positive one, upwards.
*
* JavaScript Info:
* @attribute vertical-offset
* @behavior VaadinContextMenuOverlay
*/
public void setVerticalOffset(String value) {
Polymer.property(this.getPolymerElement(), "verticalOffset", value);
}
// Needed in UIBinder
/**
* The duration in milliseconds to show the toast.
Set to 0
, a negative number, or Infinity
, to disable the
toast auto-closing.
*
* JavaScript Info:
* @attribute duration
*
*/
public void setDuration(String value) {
Polymer.property(this.getPolymerElement(), "duration", value);
}
// Needed in UIBinder
/**
* The element that should be used to position the element. If not set, it will
default to the parent node.
*
* JavaScript Info:
* @attribute position-target
* @behavior VaadinContextMenuOverlay
*/
public void setPositionTarget(String value) {
Polymer.property(this.getPolymerElement(), "positionTarget", value);
}
// Needed in UIBinder
/**
* Contains the reason(s) this overlay was last closed (see iron-overlay-closed
).
IronOverlayBehavior
provides the canceled
reason; implementers of the
behavior can provide other reasons in addition to canceled
.
*
* JavaScript Info:
* @attribute closing-reason
* @behavior VaadinContextMenuOverlay
*/
public void setClosingReason(String value) {
Polymer.property(this.getPolymerElement(), "closingReason", value);
}
// Needed in UIBinder
/**
* The element to fit this
into.
*
* JavaScript Info:
* @attribute fit-into
* @behavior VaadinContextMenuOverlay
*/
public void setFitInto(String value) {
Polymer.property(this.getPolymerElement(), "fitInto", value);
}
// Needed in UIBinder
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @attribute sizing-target
* @behavior VaadinContextMenuOverlay
*/
public void setSizingTarget(String value) {
Polymer.property(this.getPolymerElement(), "sizingTarget", value);
}
/**
* Show the toast. Without arguments, this is the same as open()
from IronOverlayBehavior
.
*
* JavaScript Info:
* @method show
* @param {(Object|string)=} properties
*
*
*/
public void show(Object properties) {
getPolymerElement().show(properties);
}
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
public void assignParentResizable(Object parentResizable) {
getPolymerElement().assignParentResizable(parentResizable);
}
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
public void stopResizeNotificationsFor(Object target) {
getPolymerElement().stopResizeNotificationsFor(target);
}
/**
* Invalidates the cached tabbable nodes. To be called when any of the focusable
content changes (e.g. a button is disabled).
*
* JavaScript Info:
* @method invalidateTabbables
* @behavior VaadinContextMenuOverlay
*
*/
public void invalidateTabbables() {
getPolymerElement().invalidateTabbables();
}
/**
* Open the overlay.
*
* JavaScript Info:
* @method open
* @behavior VaadinContextMenuOverlay
*
*/
public void open() {
getPolymerElement().open();
}
/**
* Toggle the opened state of the overlay.
*
* JavaScript Info:
* @method toggle
* @behavior VaadinContextMenuOverlay
*
*/
public void toggle() {
getPolymerElement().toggle();
}
/**
* Centers horizontally and vertically if not already positioned. This also sets
position:fixed
.
*
* JavaScript Info:
* @method center
* @behavior VaadinContextMenuOverlay
*
*/
public void center() {
getPolymerElement().center();
}
/**
* Hide the toast. Same as close()
from IronOverlayBehavior
.
*
* JavaScript Info:
* @method hide
*
*
*/
public void hide() {
getPolymerElement().hide();
}
/**
* Positions and fits the element into the fitInto
element.
*
* JavaScript Info:
* @method fit
* @behavior VaadinContextMenuOverlay
*
*/
public void fit() {
getPolymerElement().fit();
}
/**
* Positions the element according to horizontalAlign, verticalAlign
.
*
* JavaScript Info:
* @method position
* @behavior VaadinContextMenuOverlay
*
*/
public void position() {
getPolymerElement().position();
}
/**
* Equivalent to calling resetFit()
and fit()
. Useful to call this after
the element or the fitInto
element has been resized, or if any of the
positioning properties (e.g. horizontalAlign, verticalAlign
) is updated.
It preserves the scroll position of the sizingTarget.
*
* JavaScript Info:
* @method refit
* @behavior VaadinContextMenuOverlay
*
*/
public void refit() {
getPolymerElement().refit();
}
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
public void notifyResize() {
getPolymerElement().notifyResize();
}
/**
* Constrains the size of the element to fitInto
by setting max-height
and/or max-width
.
*
* JavaScript Info:
* @method constrain
* @behavior VaadinContextMenuOverlay
*
*/
public void constrain() {
getPolymerElement().constrain();
}
/**
* Close the overlay.
*
* JavaScript Info:
* @method close
* @behavior VaadinContextMenuOverlay
*
*/
public void close() {
getPolymerElement().close();
}
/**
* Resets the target element’s position and size constraints, and clear
the memoized data.
*
* JavaScript Info:
* @method resetFit
* @behavior VaadinContextMenuOverlay
*
*/
public void resetFit() {
getPolymerElement().resetFit();
}
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
public boolean resizerShouldNotify(JavaScriptObject element) {
return getPolymerElement().resizerShouldNotify(element);
}
/**
* Cancels the overlay.
*
* JavaScript Info:
* @method cancel
* @param {Event=} event
* @behavior VaadinContextMenuOverlay
*
*/
public void cancel(JavaScriptObject event) {
getPolymerElement().cancel(event);
}
/**
* Fired when paper-toast
is opened.
*
* JavaScript Info:
* @event 'iron-announce'
*/
public HandlerRegistration addIronAnnounceHandler(IronAnnounceEventHandler handler) {
return addDomHandler(handler, IronAnnounceEvent.TYPE);
}
/**
* Fired when the overlay is canceled, but before it is closed.
*
* JavaScript Info:
* @event iron-overlay-canceled
*/
public HandlerRegistration addIronOverlayCanceledHandler(IronOverlayCanceledEventHandler handler) {
return addDomHandler(handler, IronOverlayCanceledEvent.TYPE);
}
/**
* Fired after the overlay closes.
*
* JavaScript Info:
* @event iron-overlay-closed
*/
public HandlerRegistration addIronOverlayClosedHandler(IronOverlayClosedEventHandler handler) {
return addDomHandler(handler, IronOverlayClosedEvent.TYPE);
}
/**
* Fired after the overlay opens.
*
* JavaScript Info:
* @event iron-overlay-opened
*/
public HandlerRegistration addIronOverlayOpenedHandler(IronOverlayOpenedEventHandler handler) {
return addDomHandler(handler, IronOverlayOpenedEvent.TYPE);
}
}