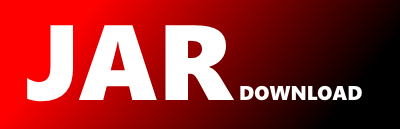
com.vaadin.polymer.paper.widget.PaperTooltip Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-tooltip project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Material design: Tooltips
* <paper-tooltip>
is a label that appears on hover and focus when the user
hovers over an element with the cursor or with the keyboard. It will be centered
to an anchor element specified in the for
attribute, or, if that doesn’t exist,
centered to the parent node containing it.
* Example:
* <div style="display:inline-block">
* <button>Click me!</button>
* <paper-tooltip>Tooltip text</paper-tooltip>
* </div>
*
* <div>
* <button id="btn">Click me!</button>
* <paper-tooltip for="btn">Tooltip text</paper-tooltip>
* </div>
*
*
*
The tooltip can be positioned on the top|bottom|left|right of the anchor using
the position
attribute. The default position is bottom.
* <paper-tooltip for="btn" position="left">Tooltip text</paper-tooltip>
* <paper-tooltip for="btn" position="top">Tooltip text</paper-tooltip>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-tooltip-background
* The background color of the tooltip
* #616161
*
*
* --paper-tooltip-opacity
* The opacity of the tooltip
* 0.9
*
*
* --paper-tooltip-text-color
* The text color of the tooltip
* white
*
*
* --paper-tooltip
* Mixin applied to the tooltip
* {}
*
*
*
*/
public class PaperTooltip extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperTooltip() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperTooltip(String html) {
super(PaperTooltipElement.TAG, PaperTooltipElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperTooltipElement getPolymerElement() {
return (PaperTooltipElement) getElement();
}
/**
* The entry and exit animations that will be played when showing and
hiding the tooltip. If you want to override this, you must ensure
that your animationConfig has the exact format below.
*
* JavaScript Info:
* @property animationConfig
* @type Object
*
*/
public JavaScriptObject getAnimationConfig() {
return getPolymerElement().getAnimationConfig();
}
/**
* The entry and exit animations that will be played when showing and
hiding the tooltip. If you want to override this, you must ensure
that your animationConfig has the exact format below.
*
* JavaScript Info:
* @property animationConfig
* @type Object
*
*/
public void setAnimationConfig(JavaScriptObject value) {
getPolymerElement().setAnimationConfig(value);
}
/**
* If true, no parts of the tooltip will ever be shown offscreen.
*
* JavaScript Info:
* @property fitToVisibleBounds
* @type Boolean
*
*/
public boolean getFitToVisibleBounds() {
return getPolymerElement().getFitToVisibleBounds();
}
/**
* If true, no parts of the tooltip will ever be shown offscreen.
*
* JavaScript Info:
* @property fitToVisibleBounds
* @type Boolean
*
*/
public void setFitToVisibleBounds(boolean value) {
getPolymerElement().setFitToVisibleBounds(value);
}
/**
* Set this to true if you want to manually control when the tooltip
is shown or hidden.
*
* JavaScript Info:
* @property manualMode
* @type Boolean
*
*/
public boolean getManualMode() {
return getPolymerElement().getManualMode();
}
/**
* Set this to true if you want to manually control when the tooltip
is shown or hidden.
*
* JavaScript Info:
* @property manualMode
* @type Boolean
*
*/
public void setManualMode(boolean value) {
getPolymerElement().setManualMode(value);
}
/**
* This property is deprecated, but left over so that it doesn’t
break exiting code. Please use offset
instead. If both offset
and
marginTop
are provided, marginTop
will be ignored.
*
* JavaScript Info:
* @property marginTop
* @type Number
*
*/
public double getMarginTop() {
return getPolymerElement().getMarginTop();
}
/**
* This property is deprecated, but left over so that it doesn’t
break exiting code. Please use offset
instead. If both offset
and
marginTop
are provided, marginTop
will be ignored.
*
* JavaScript Info:
* @property marginTop
* @type Number
*
*/
public void setMarginTop(double value) {
getPolymerElement().setMarginTop(value);
}
/**
* The spacing between the top of the tooltip and the element it is
anchored to.
*
* JavaScript Info:
* @property offset
* @type Number
*
*/
public double getOffset() {
return getPolymerElement().getOffset();
}
/**
* The spacing between the top of the tooltip and the element it is
anchored to.
*
* JavaScript Info:
* @property offset
* @type Number
*
*/
public void setOffset(double value) {
getPolymerElement().setOffset(value);
}
/**
* The delay that will be applied before the entry
animation is
played when showing the tooltip.
*
* JavaScript Info:
* @property animationDelay
* @type Number
*
*/
public double getAnimationDelay() {
return getPolymerElement().getAnimationDelay();
}
/**
* The delay that will be applied before the entry
animation is
played when showing the tooltip.
*
* JavaScript Info:
* @property animationDelay
* @type Number
*
*/
public void setAnimationDelay(double value) {
getPolymerElement().setAnimationDelay(value);
}
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior PaperTooltip
*/
public String getEntryAnimation() {
return getPolymerElement().getEntryAnimation();
}
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior PaperTooltip
*/
public void setEntryAnimation(String value) {
getPolymerElement().setEntryAnimation(value);
}
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior PaperTooltip
*/
public String getExitAnimation() {
return getPolymerElement().getExitAnimation();
}
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior PaperTooltip
*/
public void setExitAnimation(String value) {
getPolymerElement().setExitAnimation(value);
}
/**
* Positions the tooltip to the top, right, bottom, left of its content.
*
* JavaScript Info:
* @property position
* @type String
*
*/
public String getPosition() {
return getPolymerElement().getPosition();
}
/**
* Positions the tooltip to the top, right, bottom, left of its content.
*
* JavaScript Info:
* @property position
* @type String
*
*/
public void setPosition(String value) {
getPolymerElement().setPosition(value);
}
/**
* The id of the element that the tooltip is anchored to. This element
must be a sibling of the tooltip.
*
* JavaScript Info:
* @property for
* @type String
*
*/
public String getFor() {
return getPolymerElement().getFor();
}
/**
* The id of the element that the tooltip is anchored to. This element
must be a sibling of the tooltip.
*
* JavaScript Info:
* @property for
* @type String
*
*/
public void setFor(String value) {
getPolymerElement().setFor(value);
}
// Needed in UIBinder
/**
* The entry and exit animations that will be played when showing and
hiding the tooltip. If you want to override this, you must ensure
that your animationConfig has the exact format below.
*
* JavaScript Info:
* @attribute animation-config
*
*/
public void setAnimationConfig(String value) {
Polymer.property(this.getPolymerElement(), "animationConfig", value);
}
// Needed in UIBinder
/**
* This property is deprecated, but left over so that it doesn’t
break exiting code. Please use offset
instead. If both offset
and
marginTop
are provided, marginTop
will be ignored.
*
* JavaScript Info:
* @attribute margin-top
*
*/
public void setMarginTop(String value) {
Polymer.property(this.getPolymerElement(), "marginTop", value);
}
// Needed in UIBinder
/**
* The spacing between the top of the tooltip and the element it is
anchored to.
*
* JavaScript Info:
* @attribute offset
*
*/
public void setOffset(String value) {
Polymer.property(this.getPolymerElement(), "offset", value);
}
// Needed in UIBinder
/**
* The delay that will be applied before the entry
animation is
played when showing the tooltip.
*
* JavaScript Info:
* @attribute animation-delay
*
*/
public void setAnimationDelay(String value) {
Polymer.property(this.getPolymerElement(), "animationDelay", value);
}
/**
*
*
* JavaScript Info:
* @method hide
*
*
*/
public void hide() {
getPolymerElement().hide();
}
/**
*
*
* JavaScript Info:
* @method updatePosition
*
*
*/
public void updatePosition() {
getPolymerElement().updatePosition();
}
/**
*
*
* JavaScript Info:
* @method show
*
*
*/
public void show() {
getPolymerElement().show();
}
/**
* Cancels the currently running animations.
*
* JavaScript Info:
* @method cancelAnimation
* @behavior PaperTooltip
*
*/
public void cancelAnimation() {
getPolymerElement().cancelAnimation();
}
/**
* Plays an animation with an optional type
.
*
* JavaScript Info:
* @method playAnimation
* @param {string=} type
* @param {!Object=} cookie
* @behavior PaperTooltip
*
*/
public void playAnimation(String type, JavaScriptObject cookie) {
getPolymerElement().playAnimation(type, cookie);
}
}