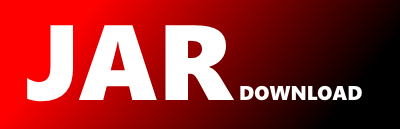
com.vaadin.polymer.platinum.PlatinumBluetoothCharacteristicElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from platinum-bluetooth project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.platinum;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* The <platinum-bluetooth-characteristic>
element allows you to read
and write characteristics on nearby bluetooth devices thanks to the
young Web Bluetooth API. It is currently partially implemented
behind the experimental flag chrome://flags/#enable-web-bluetooth
. It
is also now available in Chrome 53 as an origin trial for Chrome
OS, Android M, and Mac.
* <platinum-bluetooth-characteristic>
needs to be a child of a
<platinum-bluetooth-service>
element.
* For instance, here’s how to read battery level from a nearby bluetooth
device advertising Battery service:
* <platinum-bluetooth-device services-filter='["battery_service"]'>
* <platinum-bluetooth-service service='battery_service'>
* <platinum-bluetooth-characteristic characteristic='battery_level'>
* </platinum-bluetooth-characteristic>
* </platinum-bluetooth-service>
* </platinum-bluetooth-device>
*
* var bluetoothDevice = document.querySelector('platinum-bluetooth-device');
* var batteryLevel = document.querySelector('platinum-bluetooth-characteristic');
*
* button.addEventListener('click', function() {
* bluetoothDevice.request().then(function() {
* return batteryLevel.read().then(function(value) {
* console.log('Battery Level is ' + value.getUint8(0) + '%');
* });
* })
* .catch(function(error) { });
* });
*
* Here’s another example on how to reset energy expended on nearby
bluetooth device advertising Heart Rate service:
* <platinum-bluetooth-device services-filter='["heart_rate"]'>
* <platinum-bluetooth-service service='heart_rate'>
* <platinum-bluetooth-characteristic characteristic='heart_rate_control_point'>
* </platinum-bluetooth-characteristic>
* </platinum-bluetooth-service>
* </platinum-bluetooth-device>
*
* var bluetoothDevice = document.querySelector('platinum-bluetooth-device');
* var heartRateCtrlPoint = document.querySelector('platinum-bluetooth-characteristic');
*
* button.addEventListener('click', function() {
* bluetoothDevice.request().then(function() {
* // Writing 1 is the signal to reset energy expended.
* var resetEnergyExpended = new Uint8Array([1]);
* return heartRateCtrlPoint.write(resetEnergyExpended).then(function() {
* console.log('Energy expended has been reset');
* });
* })
* .catch(function(error) { });
* });
*
* It is also possible for <platinum-bluetooth-characteristic>
to fill in
a data-bound field in response to a read.
* <platinum-bluetooth-device services-filter='["heart_rate"]'>
* <platinum-bluetooth-service service='heart_rate'>
* <platinum-bluetooth-characteristic characteristic='body_sensor_location'
* value={{bodySensorLocation}}>
* </platinum-bluetooth-characteristic>
* </platinum-bluetooth-service>
* </platinum-bluetooth-device>
* ...
* <span>{{bodySensorLocation}}</span>
*
* var bluetoothDevice = document.querySelector('platinum-bluetooth-device');
* var bodySensorLocation = document.querySelector('platinum-bluetooth-characteristic');
*
* button.addEventListener('click', function() {
* bluetoothDevice.request().then(function() {
* return bodySensorLocation.read()
* })
* .catch(function(error) { });
* });
*
* Starting and stopping notifications on a <platinum-bluetooth-characteristic>
is pretty straightforward when taking advantage of the Polymer Change notification protocol. Here’s how to get your Heart Rate Measurement for instance:
* <platinum-bluetooth-device services-filter='["heart_rate"]'>
* <platinum-bluetooth-service service='heart_rate'>
* <platinum-bluetooth-characteristic characteristic='heart_rate_measurement'
* on-value-changed='parseHeartRate'>
* </platinum-bluetooth-characteristic>
* </platinum-bluetooth-service>
* </platinum-bluetooth-device>
*
* var bluetoothDevice = document.querySelector('platinum-bluetooth-device');
* var heartRate = document.querySelector('platinum-bluetooth-characteristic');
*
* startButton.addEventListener('click', function() {
* bluetoothDevice.request().then(function() {
* return heartRate.startNotifications().catch(function(error) { });
* });
* });
*
* stopButton.addEventListener('click', function() {
* heartRate.stopNotifications().catch(function(error) { });
* });
*
* function parseHeartRate(event) {
* let value = event.target.value;
* // Do something with the DataView Object value...
* }
*
* You can also use changes in value
to drive characteristic writes when
auto-write
property is set to true.
* <platinum-bluetooth-device services-filter='["heart_rate"]'>
* <platinum-bluetooth-service service='heart_rate'>
* <platinum-bluetooth-characteristic characteristic='heart_rate_control_point'
* auto-write>
* </platinum-bluetooth-characteristic>
* </platinum-bluetooth-service>
* </platinum-bluetooth-device>
*
* var bluetoothDevice = document.querySelector('platinum-bluetooth-device');
* var heartRateCtrlPoint = document.querySelector('platinum-bluetooth-characteristic');
*
* button.addEventListener('click', function() {
* bluetoothDevice.request().then(function() {
* // Writing 1 is the signal to reset energy expended.
* heartRateCtrlPoint.value = new Uint8Array([1]);
* })
* .catch(function(error) { });
* });
*
* heartRateCtrlPoint.addEventListener('platinum-bluetooth-auto-write-error',
* function(event) {
* // Handle error...
* });
*
*/
@JsType(isNative=true)
public interface PlatinumBluetoothCharacteristicElement extends HTMLElement {
@JsOverlay public static final String TAG = "platinum-bluetooth-characteristic";
@JsOverlay public static final String SRC = "platinum-bluetooth/platinum-bluetooth-elements.html";
/**
* If true, automatically perform a write value
on the characteristic
when value
changes.
*
* JavaScript Info:
* @property autoWrite
* @type Boolean
*
*/
@JsProperty boolean getAutoWrite();
/**
* If true, automatically perform a write value
on the characteristic
when value
changes.
*
* JavaScript Info:
* @property autoWrite
* @type Boolean
*
*/
@JsProperty void setAutoWrite(boolean value);
/**
* Value gets populated with the characteristic value when it’s read
and during a notification session.
*
* JavaScript Info:
* @property value
* @type DataView
*
*/
@JsProperty JavaScriptObject getValue();
/**
* Value gets populated with the characteristic value when it’s read
and during a notification session.
*
* JavaScript Info:
* @property value
* @type DataView
*
*/
@JsProperty void setValue(JavaScriptObject value);
/**
* Required Bluetooth GATT characteristic for read and write operations.
You may provide either the full Bluetooth UUID as a string or a
short 16- or 32-bit form as integers like 0x2A19.
*
* JavaScript Info:
* @property characteristic
* @type String
*
*/
@JsProperty String getCharacteristic();
/**
* Required Bluetooth GATT characteristic for read and write operations.
You may provide either the full Bluetooth UUID as a string or a
short 16- or 32-bit form as integers like 0x2A19.
*
* JavaScript Info:
* @property characteristic
* @type String
*
*/
@JsProperty void setCharacteristic(String value);
/**
* Returns a promise that will resolve when Bluetooth GATT Characteristic
is written.
*
* JavaScript Info:
* @method write
* @param {(ArrayBufferView|ArrayBuffer)} value
*
* @return {JavaScriptObject}
*/
JavaScriptObject write(Object value);
/**
* Returns a promise that will resolve when Bluetooth GATT Characteristic
is read.
*
* JavaScript Info:
* @method read
*
* @return {JavaScriptObject}
*/
JavaScriptObject read();
/**
* Returns a promise that will resolve when Bluetooth GATT Characteristic
notification session starts.
*
* JavaScript Info:
* @method startNotifications
*
* @return {JavaScriptObject}
*/
JavaScriptObject startNotifications();
/**
* Returns a promise that will resolve when Bluetooth GATT Characteristic
notification session stops.
*
* JavaScript Info:
* @method stopNotifications
*
* @return {JavaScriptObject}
*/
JavaScriptObject stopNotifications();
}