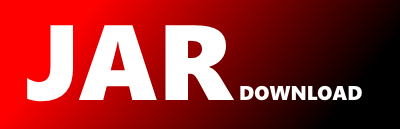
com.vaadin.polymer.platinum.PlatinumPushMessagingElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from platinum-push-messaging project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.platinum;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* <platinum-push-messaging>
sets up a push messaging subscription
and allows you to define what happens when a push message is received.
* The element can be placed anywhere, but should only be used once in a
page. If there are multiple occurrences, only one will be active.
* Sample
* For a complete sample that uses the element, see the Cat Push
Notifications project.
* Requirements
* Push messaging is currently only available in Google Chrome, which
requires you to configure Google Cloud Messaging. Chrome will check that
your page links to a manifest file that contains a gcm_sender_id
field.
You can find full details of how to set all of this up in the HTML5
Rocks guide to push notifications.
* Notification details
* The data for how a notification should be displayed can come from one of
three places.
* Firstly, you can specify a URL from which to fetch the message data.
* <platinum-push-messaging
* message-url="notification-data.json">
* </platinum-push-messaging>
*
The second way is to send the message data in the body of
the push message from your server. In this case you do not need to
configure anything in your page:
* <platinum-push-messaging></platinum-push-messaging>
*
Note that this method is not currently supported by any browser. It
is, however, defined in the
draft W3C specification
and this element should use that data when it is implemented in the
future.
* If a message-url is provided then the message body will be ignored in
favor of the first method.
* Thirdly, you can manually define the attributes on the element:
* <platinum-push-messaging
* title="Application updated"
* message="The application was updated in the background"
* icon-url="icon.png"
* click-url="notification.html">
* </platinum-push-messaging>
*
These values will also be used as defaults if one of the other methods
does not provide a value for that property.
* Testing
* If you have set up Google Cloud Messaging then you can send push messages
to your browser by following the guide in the GCM documentation.
* However, for quick client testing there are two options. You can use the
testPush
method, which allows you to simulate a push message that
includes a payload.
* Or, at a lower level, you can open up chrome://serviceworker-internals in
Chrome and use the ‘Push’ button for the service worker corresponding to
your app.
*/
@JsType(isNative=true)
public interface PlatinumPushMessagingElement extends HTMLElement {
@JsOverlay public static final String TAG = "platinum-push-messaging";
@JsOverlay public static final String SRC = "platinum-push-messaging/platinum-push-messaging.html";
/**
* If true then displaying the notification should not cause any
vibration or sound to be played.
*
* JavaScript Info:
* @property silent
* @type Boolean
*
*/
@JsProperty boolean getSilent();
/**
* If true then displaying the notification should not cause any
vibration or sound to be played.
*
* JavaScript Info:
* @property silent
* @type Boolean
*
*/
@JsProperty void setSilent(boolean value);
/**
* The details of the current push subscription, if any.
*
* JavaScript Info:
* @property subscription
* @type PushSubscription
*
*/
@JsProperty JavaScriptObject getSubscription();
/**
* The details of the current push subscription, if any.
*
* JavaScript Info:
* @property subscription
* @type PushSubscription
*
*/
@JsProperty void setSubscription(JavaScriptObject value);
/**
* When a notification is displayed that has the same tag
as an
existing notification, the existing one will be replaced. If this
flag is true then such a replacement will cause the user to be
alerted as though it were a new notification, by vibration or sound
as appropriate.
*
* JavaScript Info:
* @property renotify
* @type Boolean
*
*/
@JsProperty boolean getRenotify();
/**
* When a notification is displayed that has the same tag
as an
existing notification, the existing one will be replaced. If this
flag is true then such a replacement will cause the user to be
alerted as though it were a new notification, by vibration or sound
as appropriate.
*
* JavaScript Info:
* @property renotify
* @type Boolean
*
*/
@JsProperty void setRenotify(boolean value);
/**
* If true then the notification should be sticky, meaning that it is
not directly dismissable.
*
* JavaScript Info:
* @property sticky
* @type Boolean
*
*/
@JsProperty boolean getSticky();
/**
* If true then the notification should be sticky, meaning that it is
not directly dismissable.
*
* JavaScript Info:
* @property sticky
* @type Boolean
*
*/
@JsProperty void setSticky(boolean value);
/**
* Indicates the status of the element. If true, push messages will be
received.
*
* JavaScript Info:
* @property enabled
* @type Boolean
*
*/
@JsProperty boolean getEnabled();
/**
* Indicates the status of the element. If true, push messages will be
received.
*
* JavaScript Info:
* @property enabled
* @type Boolean
*
*/
@JsProperty void setEnabled(boolean value);
/**
* Indicates whether the Push and Notification APIs are supported by
this browser.
*
* JavaScript Info:
* @property supported
* @type Boolean
*
*/
@JsProperty boolean getSupported();
/**
* Indicates whether the Push and Notification APIs are supported by
this browser.
*
* JavaScript Info:
* @property supported
* @type Boolean
*
*/
@JsProperty void setSupported(boolean value);
/**
* If true then displaying the notification should not turn the device’s
screen on.
*
* JavaScript Info:
* @property noscreen
* @type Boolean
*
*/
@JsProperty boolean getNoscreen();
/**
* If true then displaying the notification should not turn the device’s
screen on.
*
* JavaScript Info:
* @property noscreen
* @type Boolean
*
*/
@JsProperty void setNoscreen(boolean value);
/**
* The pattern of vibration that should be used by default when a
notification is displayed. See
*
* JavaScript Info:
* @property vibrate
* @type Array
*
*/
@JsProperty JsArray getVibrate();
/**
* The pattern of vibration that should be used by default when a
notification is displayed. See
*
* JavaScript Info:
* @property vibrate
* @type Array
*
*/
@JsProperty void setVibrate(JsArray value);
/**
* The URL of a default icon for notifications.
*
* JavaScript Info:
* @property iconUrl
* @type string
*
*/
@JsProperty String getIconUrl();
/**
* The URL of a default icon for notifications.
*
* JavaScript Info:
* @property iconUrl
* @type string
*
*/
@JsProperty void setIconUrl(String value);
/**
* The URL of a default sound file to play when a notification is shown.
*
* JavaScript Info:
* @property sound
* @type string
*
*/
@JsProperty String getSound();
/**
* The URL of a default sound file to play when a notification is shown.
*
* JavaScript Info:
* @property sound
* @type string
*
*/
@JsProperty void setSound(String value);
/**
* The default language to assume for the title and body of the
notification. If set this must be a valid
BCP 47 language tag.
*
* JavaScript Info:
* @property lang
* @type string
*
*/
@JsProperty String getLang();
/**
* The default language to assume for the title and body of the
notification. If set this must be a valid
BCP 47 language tag.
*
* JavaScript Info:
* @property lang
* @type string
*
*/
@JsProperty void setLang(String value);
/**
* The default notification message.
*
* JavaScript Info:
* @property message
* @type string
*
*/
@JsProperty String getMessage();
/**
* The default notification message.
*
* JavaScript Info:
* @property message
* @type string
*
*/
@JsProperty void setMessage(String value);
/**
* A URL from which message information can be retrieved.
* When a push event happens that does not contain a message body this
URL will be fetched. The document will be parsed as JSON, and should
result in an object.
* The valid keys for the object are title
, message
, url
, icon
,
tag
, dir
, lang
, noscreen
, renotify
, silent
, sound
,
sticky
and vibrate
. For documentation of these values see the
attributes of the same names, except that these values override the
element attributes.
*
* JavaScript Info:
* @property messageUrl
* @type string
*
*/
@JsProperty String getMessageUrl();
/**
* A URL from which message information can be retrieved.
* When a push event happens that does not contain a message body this
URL will be fetched. The document will be parsed as JSON, and should
result in an object.
* The valid keys for the object are title
, message
, url
, icon
,
tag
, dir
, lang
, noscreen
, renotify
, silent
, sound
,
sticky
and vibrate
. For documentation of these values see the
attributes of the same names, except that these values override the
element attributes.
*
* JavaScript Info:
* @property messageUrl
* @type string
*
*/
@JsProperty void setMessageUrl(String value);
/**
* A default tag for the notifications that will be generated by
this element. Notifications with the same tag will overwrite one
another, so that only one will be shown at once.
*
* JavaScript Info:
* @property tag
* @type string
*
*/
@JsProperty String getTag();
/**
* A default tag for the notifications that will be generated by
this element. Notifications with the same tag will overwrite one
another, so that only one will be shown at once.
*
* JavaScript Info:
* @property tag
* @type string
*
*/
@JsProperty void setTag(String value);
/**
* The default URL to display when a notification is clicked.
*
* JavaScript Info:
* @property clickUrl
* @type String
*
*/
@JsProperty String getClickUrl();
/**
* The default URL to display when a notification is clicked.
*
* JavaScript Info:
* @property clickUrl
* @type String
*
*/
@JsProperty void setClickUrl(String value);
/**
* The default notification title.
*
* JavaScript Info:
* @property title
* @type string
*
*/
@JsProperty String getTitle();
/**
* The default notification title.
*
* JavaScript Info:
* @property title
* @type string
*
*/
@JsProperty void setTitle(String value);
/**
* The default text direction for the title and body of the
notification. Can be auto
, ltr
or rtl
.
*
* JavaScript Info:
* @property dir
* @type String
*
*/
@JsProperty String getDir();
/**
* The default text direction for the title and body of the
notification. Can be auto
, ltr
or rtl
.
*
* JavaScript Info:
* @property dir
* @type String
*
*/
@JsProperty void setDir(String value);
/**
* The location of the service worker script required by the element.
The script is distributed alongside the main HTML import file for the
element, so the location can normally be determined automatically.
However, if you vulcanize your project you will need to include the
script in your built project manually and use this property to let
the element know how to load it.
*
* JavaScript Info:
* @property workerUrl
* @type String
*
*/
@JsProperty String getWorkerUrl();
/**
* The location of the service worker script required by the element.
The script is distributed alongside the main HTML import file for the
element, so the location can normally be determined automatically.
However, if you vulcanize your project you will need to include the
script in your built project manually and use this property to let
the element know how to load it.
*
* JavaScript Info:
* @property workerUrl
* @type String
*
*/
@JsProperty void setWorkerUrl(String value);
/**
* Programmatically trigger a push message
*
* JavaScript Info:
* @method testPush
* @param {} message
*
*
*/
void testPush(Object message);
/**
* Request push messaging to be disabled.
*
* JavaScript Info:
* @method disable
*
* @return {JavaScriptObject}
*/
JavaScriptObject disable();
/**
* Request push messaging to be enabled.
*
* JavaScript Info:
* @method enable
*
* @return {JavaScriptObject}
*/
JavaScriptObject enable();
}