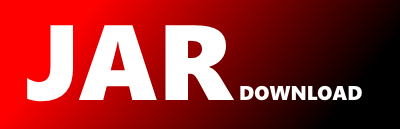
com.vaadin.polymer.platinum.widget.PlatinumBluetoothDevice Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from platinum-bluetooth project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.platinum.widget;
import com.vaadin.polymer.platinum.*;
import com.vaadin.polymer.platinum.widget.event.DeviceChangedEvent;
import com.vaadin.polymer.platinum.widget.event.DeviceChangedEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* The <platinum-bluetooth-device>
element allows you to discover nearby
bluetooth devices thanks to the young Web Bluetooth API. It is
currently partially implemented behind the experimental flag
chrome://flags/#enable-web-bluetooth
. It is also now available in
Chrome 53 as an origin trial for Chrome OS, Android M, and Mac.
* <platinum-bluetooth-device>
is used as a parent element for
<platinum-bluetooth-service>
child elements.
* For instance, here’s how to request a nearby bluetooth device advertising
Battery service:
* <platinum-bluetooth-device services-filter='["battery_service"]'>
* </platinum-bluetooth-device>
*
* button.addEventListener('click', function() {
* document.querySelector('platinum-bluetooth-device').request()
* .then(function(device) { console.log(device.name); })
* .catch(function(error) { console.error(error); });
* });
*
* You can also request a nearby bluetooth device by setting a filter on
a name or name Prefix:
* <platinum-bluetooth-device name-filter='foobar'>
* </platinum-bluetooth-device>
*
* <platinum-bluetooth-device name-prefix-filter='foo'>
* </platinum-bluetooth-device>
*
* And you can combine some of the filters as well. Here’s how to request a
nearby bluetooth device advertising Battery service and whose name is
“foobar”:
* <platinum-bluetooth-device services-filter='["battery_service"]'
* name-filter='foobar'>
* </platinum-bluetooth-device>
*
* If you filter just by name, then you must use optional-services-filter
to get access to any services:
* <platinum-bluetooth-device name-filter='foobar'
* optional-services-filter='["battery_service"]'>
* </platinum-bluetooth-device>
*
* Disconnecting manually from a connected bluetooth device is pretty
straightforward:
* disconnectButton.addEventListener('click', function() {
* document.querySelector('platinum-bluetooth-device').disconnect();
* });
*
*/
public class PlatinumBluetoothDevice extends PolymerWidget {
/**
* Default Constructor.
*/
public PlatinumBluetoothDevice() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PlatinumBluetoothDevice(String html) {
super(PlatinumBluetoothDeviceElement.TAG, PlatinumBluetoothDeviceElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PlatinumBluetoothDeviceElement getPolymerElement() {
return (PlatinumBluetoothDeviceElement) getElement();
}
/**
* Current Bluetooth device picked by user.
*
* JavaScript Info:
* @property device
* @type BluetoothDevice
*
*/
public JavaScriptObject getDevice() {
return getPolymerElement().getDevice();
}
/**
* Current Bluetooth device picked by user.
*
* JavaScript Info:
* @property device
* @type BluetoothDevice
*
*/
public void setDevice(JavaScriptObject value) {
getPolymerElement().setDevice(value);
}
/**
* Optional Bluetooth GATT services filter. This implies that if you
filter just by name, you must use optionalServicesFilter
to get
access to any services. You may provide either the full Bluetooth
UUID as a string or a short 16- or 32-bit form as integers like
0x180d.
*
* JavaScript Info:
* @property optionalServicesFilter
* @type Array
*
*/
public JsArray getOptionalServicesFilter() {
return getPolymerElement().getOptionalServicesFilter();
}
/**
* Optional Bluetooth GATT services filter. This implies that if you
filter just by name, you must use optionalServicesFilter
to get
access to any services. You may provide either the full Bluetooth
UUID as a string or a short 16- or 32-bit form as integers like
0x180d.
*
* JavaScript Info:
* @property optionalServicesFilter
* @type Array
*
*/
public void setOptionalServicesFilter(JsArray value) {
getPolymerElement().setOptionalServicesFilter(value);
}
/**
* Bluetooth GATT services filter. You may provide either the
full Bluetooth UUID as a string or a short 16- or 32-bit form as
integers like 0x180d.
*
* JavaScript Info:
* @property servicesFilter
* @type Array
*
*/
public JsArray getServicesFilter() {
return getPolymerElement().getServicesFilter();
}
/**
* Bluetooth GATT services filter. You may provide either the
full Bluetooth UUID as a string or a short 16- or 32-bit form as
integers like 0x180d.
*
* JavaScript Info:
* @property servicesFilter
* @type Array
*
*/
public void setServicesFilter(JsArray value) {
getPolymerElement().setServicesFilter(value);
}
/**
* Indicates whether the Web Bluetooth API is supported by
this browser.
*
* JavaScript Info:
* @property supported
* @type Boolean
*
*/
public boolean getSupported() {
return getPolymerElement().getSupported();
}
/**
* Indicates whether the Web Bluetooth API is supported by
this browser.
*
* JavaScript Info:
* @property supported
* @type Boolean
*
*/
public void setSupported(boolean value) {
getPolymerElement().setSupported(value);
}
/**
* Device name filter.
*
* JavaScript Info:
* @property nameFilter
* @type String
*
*/
public String getNameFilter() {
return getPolymerElement().getNameFilter();
}
/**
* Device name filter.
*
* JavaScript Info:
* @property nameFilter
* @type String
*
*/
public void setNameFilter(String value) {
getPolymerElement().setNameFilter(value);
}
/**
* Device name prefix filter.
*
* JavaScript Info:
* @property namePrefixFilter
* @type String
*
*/
public String getNamePrefixFilter() {
return getPolymerElement().getNamePrefixFilter();
}
/**
* Device name prefix filter.
*
* JavaScript Info:
* @property namePrefixFilter
* @type String
*
*/
public void setNamePrefixFilter(String value) {
getPolymerElement().setNamePrefixFilter(value);
}
// Needed in UIBinder
/**
* Current Bluetooth device picked by user.
*
* JavaScript Info:
* @attribute device
*
*/
public void setDevice(String value) {
Polymer.property(this.getPolymerElement(), "device", value);
}
// Needed in UIBinder
/**
* Optional Bluetooth GATT services filter. This implies that if you
filter just by name, you must use optionalServicesFilter
to get
access to any services. You may provide either the full Bluetooth
UUID as a string or a short 16- or 32-bit form as integers like
0x180d.
*
* JavaScript Info:
* @attribute optional-services-filter
*
*/
public void setOptionalServicesFilter(String value) {
Polymer.property(this.getPolymerElement(), "optionalServicesFilter", value);
}
// Needed in UIBinder
/**
* Bluetooth GATT services filter. You may provide either the
full Bluetooth UUID as a string or a short 16- or 32-bit form as
integers like 0x180d.
*
* JavaScript Info:
* @attribute services-filter
*
*/
public void setServicesFilter(String value) {
Polymer.property(this.getPolymerElement(), "servicesFilter", value);
}
/**
* Disconnect GATT Server connection from current bluetooth device.
*
* JavaScript Info:
* @method disconnect
*
*
*/
public void disconnect() {
getPolymerElement().disconnect();
}
/**
* Request a nearby bluetooth device and returns a Promise that will
resolve when user picked one Bluetooth device based on filters.
* It must be called on user gesture.
*
* JavaScript Info:
* @method request
*
* @return {JavaScriptObject}
*/
public JavaScriptObject request() {
return getPolymerElement().request();
}
/**
* Reset device to pick a new device.
*
* JavaScript Info:
* @method reset
*
*
*/
public void reset() {
getPolymerElement().reset();
}
/**
* Fired when Bluetooth device picked by user gets updated.
*
* JavaScript Info:
* @event device-changed
*/
public HandlerRegistration addDeviceChangedHandler(DeviceChangedEventHandler handler) {
return addDomHandler(handler, DeviceChangedEvent.TYPE);
}
}