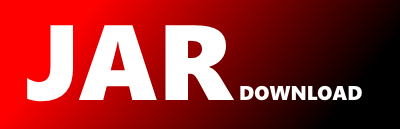
com.vaadin.polymer.platinum.widget.PlatinumSwCache Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from platinum-sw project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.platinum.widget;
import com.vaadin.polymer.platinum.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* The <platinum-sw-cache>
element makes it easy to precache specific resources, perform runtime
caching, and serve your cached resources when a network is unavailable.
Under the hood, the sw-toolbox library is used
for all the caching and request handling logic.
<platinum-sw-cache>
needs to be a child element of <platinum-sw-register>
.
A simple, yet useful configuration is
* <platinum-sw-register auto-register>
* <platinum-sw-cache></platinum-sw-cache>
* </platinum-sw-register>
*
*
*
This is enough to have all of the resources your site uses cached at runtime, both local and
cross-origin.
(It uses the default defaultCacheStrategy
of “networkFirst”.)
When there’s a network available, visits to your site will go against the network copy of the
resources, but if someone visits your site when they’re offline, all the cached resources will
be used.
*/
public class PlatinumSwCache extends PolymerWidget {
/**
* Default Constructor.
*/
public PlatinumSwCache() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PlatinumSwCache(String html) {
super(PlatinumSwCacheElement.TAG, PlatinumSwCacheElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PlatinumSwCacheElement getPolymerElement() {
return (PlatinumSwCacheElement) getElement();
}
/**
* If set to true, this element will not set up service worker caching. This is useful to
conditionally enable or disable caching depending on the build environment.
*
* JavaScript Info:
* @property disabled
* @type Boolean
*
*/
public boolean getDisabled() {
return getPolymerElement().getDisabled();
}
/**
* If set to true, this element will not set up service worker caching. This is useful to
conditionally enable or disable caching depending on the build environment.
*
* JavaScript Info:
* @property disabled
* @type Boolean
*
*/
public void setDisabled(boolean value) {
getPolymerElement().setDisabled(value);
}
/**
* Used to provide a list of URLs that are always precached as soon as the service worker is
installed. Corresponds to sw-toolbox
‘s precache()
method.
* This is useful for URLs that that wouldn’t necessarily be picked up by runtime caching,
i.e. a list of resources that are needed by one of the subpages of your site, or a list of
resources that are only loaded via user interaction.
* precache
can be used in conjunction with cacheConfigFile
, and the two arrays will be
concatenated.
*
* JavaScript Info:
* @property precache
* @type Array
*
*/
public JsArray getPrecache() {
return getPolymerElement().getPrecache();
}
/**
* Used to provide a list of URLs that are always precached as soon as the service worker is
installed. Corresponds to sw-toolbox
‘s precache()
method.
* This is useful for URLs that that wouldn’t necessarily be picked up by runtime caching,
i.e. a list of resources that are needed by one of the subpages of your site, or a list of
resources that are only loaded via user interaction.
* precache
can be used in conjunction with cacheConfigFile
, and the two arrays will be
concatenated.
*
* JavaScript Info:
* @property precache
* @type Array
*
*/
public void setPrecache(JsArray value) {
getPolymerElement().setPrecache(value);
}
/**
* Used to configure <platinum-sw-precache>
behavior via a JSON file instead of via
attributes. This can come in handy when the configuration (e.g. which files to precache)
depends on the results of a build script.
* If configuration for the same properties are provided in both the JSON file and via the
element’s attributes, then in general the JSON file’s values take precedence. The one
exception is the precache
property. Any values in the element’s precache
attribute will
be concatenated with the values in the JSON file’s precache
property and the set of files
that are precached will be the union of the two.
* There’s one additional option, precacheFingerprint
, that can be set in the JSON. If using
a build script that might output a large number of files to precache, its recommended
that your build script generate a unique “fingerprint” of the files. Any changes to the
precacheFingerprint
value will result in the underlying service worker kicking off the
process of caching the files listed in precache
.
While there are a few different strategies for generating an appropriate
precacheFingerprint
value, a process that makes sense is to use a stable hash of the
serialized precache
array. That way, any changes to the list of files in precache
will result in a new precacheFingerprint
value.
If your build script is Node.js based, one way to generate this hash is:
* var md5 = require('crypto').createHash('md5');
* md5.update(JSON.stringify(precache));
* var precacheFingerprint = md5.digest('hex');
*
*
*
Alternatively, you could use something like the
SHA-1 signature
of your latest git
commit for the precacheFingerprint
value.
* An example file may look like:
* {
* "cacheId": "my-cache-id",
* "defaultCacheStrategy": "fastest",
* "disabled": false,
* "precache": ["file1.html", "file2.css"],
* "precacheFingerprint": "FINGERPRINT_OF_FILES_IN_PRECACHE"
* }
*
*
*
* JavaScript Info:
* @property cacheConfigFile
* @type string
*
*/
public String getCacheConfigFile() {
return getPolymerElement().getCacheConfigFile();
}
/**
* Used to configure <platinum-sw-precache>
behavior via a JSON file instead of via
attributes. This can come in handy when the configuration (e.g. which files to precache)
depends on the results of a build script.
* If configuration for the same properties are provided in both the JSON file and via the
element’s attributes, then in general the JSON file’s values take precedence. The one
exception is the precache
property. Any values in the element’s precache
attribute will
be concatenated with the values in the JSON file’s precache
property and the set of files
that are precached will be the union of the two.
* There’s one additional option, precacheFingerprint
, that can be set in the JSON. If using
a build script that might output a large number of files to precache, its recommended
that your build script generate a unique “fingerprint” of the files. Any changes to the
precacheFingerprint
value will result in the underlying service worker kicking off the
process of caching the files listed in precache
.
While there are a few different strategies for generating an appropriate
precacheFingerprint
value, a process that makes sense is to use a stable hash of the
serialized precache
array. That way, any changes to the list of files in precache
will result in a new precacheFingerprint
value.
If your build script is Node.js based, one way to generate this hash is:
* var md5 = require('crypto').createHash('md5');
* md5.update(JSON.stringify(precache));
* var precacheFingerprint = md5.digest('hex');
*
*
*
Alternatively, you could use something like the
SHA-1 signature
of your latest git
commit for the precacheFingerprint
value.
* An example file may look like:
* {
* "cacheId": "my-cache-id",
* "defaultCacheStrategy": "fastest",
* "disabled": false,
* "precache": ["file1.html", "file2.css"],
* "precacheFingerprint": "FINGERPRINT_OF_FILES_IN_PRECACHE"
* }
*
*
*
* JavaScript Info:
* @property cacheConfigFile
* @type string
*
*/
public void setCacheConfigFile(String value) {
getPolymerElement().setCacheConfigFile(value);
}
/**
* An id used to construct the name for the
Cache
in which all the resources will be stored.
* If nothing is provided, the default value set in
toolbox.options.cacheName
will be used.
* The cacheId
is combined with the service worker’s scope to construct the cache name, so
two <platinum-sw-cache>
elements that are associated with different scopes will use
different caches.
*
* JavaScript Info:
* @property cacheId
* @type string
*
*/
public String getCacheId() {
return getPolymerElement().getCacheId();
}
/**
* An id used to construct the name for the
Cache
in which all the resources will be stored.
* If nothing is provided, the default value set in
toolbox.options.cacheName
will be used.
* The cacheId
is combined with the service worker’s scope to construct the cache name, so
two <platinum-sw-cache>
elements that are associated with different scopes will use
different caches.
*
* JavaScript Info:
* @property cacheId
* @type string
*
*/
public void setCacheId(String value) {
getPolymerElement().setCacheId(value);
}
/**
* The caching strategy used for all requests, both for local and cross-origin resources.
* For a list of strategies, see the sw-toolbox
documentation.
Specify a strategy as a string, without the “toolbox” prefix. E.g., for
toolbox.networkFirst
, set defaultCacheStrategy
to “networkFirst”.
* Note that the “cacheFirst” and “cacheOnly” strategies are not recommended, and may be
explicitly prevented in a future release. More information can be found at
https://github.com/PolymerElements/platinum-sw#cacheonly--cachefirst-defaultcachestrategy-considered-harmful
*
* JavaScript Info:
* @property defaultCacheStrategy
* @type String
*
*/
public String getDefaultCacheStrategy() {
return getPolymerElement().getDefaultCacheStrategy();
}
/**
* The caching strategy used for all requests, both for local and cross-origin resources.
* For a list of strategies, see the sw-toolbox
documentation.
Specify a strategy as a string, without the “toolbox” prefix. E.g., for
toolbox.networkFirst
, set defaultCacheStrategy
to “networkFirst”.
* Note that the “cacheFirst” and “cacheOnly” strategies are not recommended, and may be
explicitly prevented in a future release. More information can be found at
https://github.com/PolymerElements/platinum-sw#cacheonly--cachefirst-defaultcachestrategy-considered-harmful
*
* JavaScript Info:
* @property defaultCacheStrategy
* @type String
*
*/
public void setDefaultCacheStrategy(String value) {
getPolymerElement().setDefaultCacheStrategy(value);
}
// Needed in UIBinder
/**
* Used to provide a list of URLs that are always precached as soon as the service worker is
installed. Corresponds to sw-toolbox
‘s precache()
method.
* This is useful for URLs that that wouldn’t necessarily be picked up by runtime caching,
i.e. a list of resources that are needed by one of the subpages of your site, or a list of
resources that are only loaded via user interaction.
* precache
can be used in conjunction with cacheConfigFile
, and the two arrays will be
concatenated.
*
* JavaScript Info:
* @attribute precache
*
*/
public void setPrecache(String value) {
Polymer.property(this.getPolymerElement(), "precache", value);
}
}