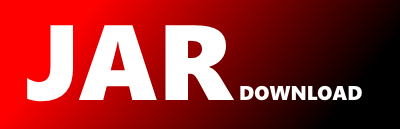
com.vaadin.polymer.platinum.widget.PlatinumSwFetch Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from platinum-sw project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.platinum.widget;
import com.vaadin.polymer.platinum.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* The <platinum-sw-fetch>
element creates custom fetch
event
handlers for given URL patterns. Possible use cases include:
*
* - Using a special caching strategy for specific URLs.
* - Returning static “fallback” responses instead of network errors when a remote API
is unavailable.
*
* In short, any scenario in which you’d like a service worker to intercept network
requests and provide custom response handling.
* If you’d like a single caching policy applied to all same-origin requests, then an alternative
to using <platinum-sw-fetch>
is to use <platinum-sw-cache>
with the defaultCacheStategy
property set.
* Under the hood, the sw-toolbox library is used
for all the request handling logic.
* <platinum-sw-fetch>
needs to be a child element of <platinum-sw-register>
.
* An example configuration is:
* <platinum-sw-register auto-register>
* <platinum-sw-import-script href="custom-fetch-handler.js"></platinum-sw-import-script>
* <platinum-sw-fetch handler="customFetchHandler"
* path="/(.*)/customFetch"></platinum-sw-fetch>
* </platinum-sw-register>
*
*
*
This implies that there’s a custom-fetch-handler.js
file in the same directory as the current
page, which defines a sw-toolbox
compliant
request handler named
customFetchHandler
. This definition is imported using <platinum-sw-import-script>
. The
<platinum-sw-fetch>
element takes care of mapping which request paths are handled by
customFetchHandler
.
* Anything not matching the path
pattern is ignored by <platinum-sw-fetch>
,
and it’s possible to have multiple <platinum-sw-fetch>
elements that each define different
paths and different handlers. The path matching is performed top-down, starting with the first
<platinum-sw-fetch>
element.
* The path
will, by default, only match same-origin requests. If you’d like to define a custom
handler for requests on a specific cross-origin domain, you must use the origin
parameter
in conjunction with path
to match the domains you’d like to handle.
*/
public class PlatinumSwFetch extends PolymerWidget {
/**
* Default Constructor.
*/
public PlatinumSwFetch() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PlatinumSwFetch(String html) {
super(PlatinumSwFetchElement.TAG, PlatinumSwFetchElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PlatinumSwFetchElement getPolymerElement() {
return (PlatinumSwFetchElement) getElement();
}
/**
* The name of the request handler to use. This should be a sw-toolbox
-style
request handler.
* handler
is a String
, not a function
, so you’re providing the name of a function, not
the function itself. It can be a function defined in the
toolbox
scope
(e.g. ‘networkFirst’, ‘fastest’, ‘networkOnly’, etc.) or a function defined in the
ServiceWorkerGlobalScope
,
like a function that is defined in a file that’s imported via platinum-sw-import-script
.
*
*
* JavaScript Info:
* @property handler
* @type string
*
*/
public String getHandler() {
return getPolymerElement().getHandler();
}
/**
* The name of the request handler to use. This should be a sw-toolbox
-style
request handler.
* handler
is a String
, not a function
, so you’re providing the name of a function, not
the function itself. It can be a function defined in the
toolbox
scope
(e.g. ‘networkFirst’, ‘fastest’, ‘networkOnly’, etc.) or a function defined in the
ServiceWorkerGlobalScope
,
like a function that is defined in a file that’s imported via platinum-sw-import-script
.
*
*
* JavaScript Info:
* @property handler
* @type string
*
*/
public void setHandler(String value) {
getPolymerElement().setHandler(value);
}
/**
* By default, path
will only match URLs under the current host (i.e. same-origin requests).
If you’d like to apply handler
to cross-origin requests, then use origin
to specify
which hosts will match. Setting origin
is optional.
* origin
is a String
, but it is used internally to construct a
RegExp
object,
which is used for the matching.
* Note that the origin
value will be matched against the full domain name and the protocol.
If you want to match ‘http’ and ‘https’, then use ‘https?://‘ at the start of your string.
* Some examples:
*
* origin="https?://.+\.google\.com"
→ a RegExp that matches http
or https
requests
made to any domain that ends in .google.com
.
* origin="https://www\.example\.com" → a RegExp that will only match
httpsrequests to
* one domain,
www.example.com`.
*
*
* JavaScript Info:
* @property origin
* @type string
*
*/
public String getOrigin() {
return getPolymerElement().getOrigin();
}
/**
* By default, path
will only match URLs under the current host (i.e. same-origin requests).
If you’d like to apply handler
to cross-origin requests, then use origin
to specify
which hosts will match. Setting origin
is optional.
* origin
is a String
, but it is used internally to construct a
RegExp
object,
which is used for the matching.
* Note that the origin
value will be matched against the full domain name and the protocol.
If you want to match ‘http’ and ‘https’, then use ‘https?://‘ at the start of your string.
* Some examples:
*
* origin="https?://.+\.google\.com"
→ a RegExp that matches http
or https
requests
made to any domain that ends in .google.com
.
* origin="https://www\.example\.com" → a RegExp that will only match
httpsrequests to
* one domain,
www.example.com`.
*
*
* JavaScript Info:
* @property origin
* @type string
*
*/
public void setOrigin(String value) {
getPolymerElement().setOrigin(value);
}
/**
* URLs with paths matching path
will have handler
applied to them.
* By default, path
will only match same-origin URLs. If you’d like it to match
cross-origin URLs, use path
in conjunction with origin
.
* As explained in the
sw-toolbox
docs,
the URL path matching is done using the path-to-regexp
module, which is the same logic used in Express-style routing.
* In practice, you need to always use ‘/‘ as the first character of your path
, and then
can use ‘(.*)’ as a wildcard.
* Some examples:
*
* path="/(.*)/customFetch"
→ matches any path that ends with ‘/customFetch’.
* path="/customFetch(.*)"
→ matches any path that starts with ‘/customFetch’, optionally
followed by other characters.
*
*
* JavaScript Info:
* @property path
* @type string
*
*/
public String getPath() {
return getPolymerElement().getPath();
}
/**
* URLs with paths matching path
will have handler
applied to them.
* By default, path
will only match same-origin URLs. If you’d like it to match
cross-origin URLs, use path
in conjunction with origin
.
* As explained in the
sw-toolbox
docs,
the URL path matching is done using the path-to-regexp
module, which is the same logic used in Express-style routing.
* In practice, you need to always use ‘/‘ as the first character of your path
, and then
can use ‘(.*)’ as a wildcard.
* Some examples:
*
* path="/(.*)/customFetch"
→ matches any path that ends with ‘/customFetch’.
* path="/customFetch(.*)"
→ matches any path that starts with ‘/customFetch’, optionally
followed by other characters.
*
*
* JavaScript Info:
* @property path
* @type string
*
*/
public void setPath(String value) {
getPolymerElement().setPath(value);
}
}