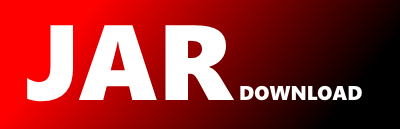
com.vaadin.polymer.vaadin.VaadinElementsComboboxComboBoxBehavior Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from vaadin-combo-box project by Vaadin Ltd
* that is licensed with Apache-2.0 license.
*/
package com.vaadin.polymer.vaadin;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
*
*/
@JsType(isNative=true)
public interface VaadinElementsComboboxComboBoxBehavior {
@JsOverlay public static final String NAME = "vaadin.elements.combobox.ComboBoxBehavior";
@JsOverlay public static final String SRC = "vaadin-combo-box/vaadin-combo-box.html";
/**
* If true
, the user can input a value that is not present in the items list.
value
property will be set to the input value in this case.
Also, when value
is set programmatically, the input value will be set
to reflect that value.
*
* JavaScript Info:
* @property allowCustomValue
* @type Boolean
*
*/
@JsProperty boolean getAllowCustomValue();
/**
* If true
, the user can input a value that is not present in the items list.
value
property will be set to the input value in this case.
Also, when value
is set programmatically, the input value will be set
to reflect that value.
*
* JavaScript Info:
* @property allowCustomValue
* @type Boolean
*
*/
@JsProperty void setAllowCustomValue(boolean value);
/**
* A subset of items, filtered based on the user input. Filtered items
can be assigned directly to omit the internal filtering functionality.
The items can be of either String
or Object
type.
*
* JavaScript Info:
* @property filteredItems
* @type Array
*
*/
@JsProperty JsArray getFilteredItems();
/**
* A subset of items, filtered based on the user input. Filtered items
can be assigned directly to omit the internal filtering functionality.
The items can be of either String
or Object
type.
*
* JavaScript Info:
* @property filteredItems
* @type Array
*
*/
@JsProperty void setFilteredItems(JsArray value);
/**
* A full set of items to filter the visible options from.
The items can be of either String
or Object
type.
*
* JavaScript Info:
* @property items
* @type Array
*
*/
@JsProperty JsArray getItems();
/**
* A full set of items to filter the visible options from.
The items can be of either String
or Object
type.
*
* JavaScript Info:
* @property items
* @type Array
*
*/
@JsProperty void setItems(JsArray value);
/**
* When present, it specifies that the element field is read-only.
*
* JavaScript Info:
* @property readonly
* @type Boolean
*
*/
@JsProperty boolean getReadonly();
/**
* When present, it specifies that the element field is read-only.
*
* JavaScript Info:
* @property readonly
* @type Boolean
*
*/
@JsProperty void setReadonly(boolean value);
/**
* Set to true to disable this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
*
*/
@JsProperty boolean getDisabled();
/**
* Set to true to disable this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
*
*/
@JsProperty void setDisabled(boolean value);
/**
* When set to true
, a loading spinner is displayed on top of the list of options.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
@JsProperty boolean getLoading();
/**
* When set to true
, a loading spinner is displayed on top of the list of options.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
@JsProperty void setLoading(boolean value);
/**
* A read-only property indicating whether this combo box has a value
selected or not. It can be used for example in styling of the component.
*
* JavaScript Info:
* @property hasValue
* @type Boolean
*
*/
@JsProperty boolean getHasValue();
/**
* A read-only property indicating whether this combo box has a value
selected or not. It can be used for example in styling of the component.
*
* JavaScript Info:
* @property hasValue
* @type Boolean
*
*/
@JsProperty void setHasValue(boolean value);
/**
* True if the dropdown is open, false otherwise.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty boolean getOpened();
/**
* True if the dropdown is open, false otherwise.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty void setOpened(boolean value);
/**
* Returns a reference to the input element.
*
* JavaScript Info:
* @property inputElement
* @type HTMLElement
*
*/
@JsProperty JavaScriptObject getInputElement();
/**
* Returns a reference to the input element.
*
* JavaScript Info:
* @property inputElement
* @type HTMLElement
*
*/
@JsProperty void setInputElement(JavaScriptObject value);
/**
* The selected item from the items
array.
*
* JavaScript Info:
* @property selectedItem
* @type Object
*
*/
@JsProperty JavaScriptObject getSelectedItem();
/**
* The selected item from the items
array.
*
* JavaScript Info:
* @property selectedItem
* @type Object
*
*/
@JsProperty void setSelectedItem(JavaScriptObject value);
/**
* Path for label of the item. If items
is an array of objects, the
itemLabelPath
is used to fetch the displayed string label for each
item.
* The item label is also used for matching items when processing user
input, i.e., for filtering and selecting items.
* When using item templates, the property is still needed because it is used
for filtering, and for displaying the selected item value in the input box.
*
* JavaScript Info:
* @property itemLabelPath
* @type String
*
*/
@JsProperty String getItemLabelPath();
/**
* Path for label of the item. If items
is an array of objects, the
itemLabelPath
is used to fetch the displayed string label for each
item.
* The item label is also used for matching items when processing user
input, i.e., for filtering and selecting items.
* When using item templates, the property is still needed because it is used
for filtering, and for displaying the selected item value in the input box.
*
* JavaScript Info:
* @property itemLabelPath
* @type String
*
*/
@JsProperty void setItemLabelPath(String value);
/**
* The String
value for the selected item of the combo box. Provides
the value for iron-form
.
* When there’s no item selected, the value is an empty string.
* Use selectedItem
property to get the raw selected item from
the items
array.
*
* JavaScript Info:
* @property value
* @type String
*
*/
@JsProperty String getValue();
/**
* The String
value for the selected item of the combo box. Provides
the value for iron-form
.
* When there’s no item selected, the value is an empty string.
* Use selectedItem
property to get the raw selected item from
the items
array.
*
* JavaScript Info:
* @property value
* @type String
*
*/
@JsProperty void setValue(String value);
/**
* Filtering string the user has typed into the input field.
*
* JavaScript Info:
* @property filter
* @type String
*
*/
@JsProperty String getFilter();
/**
* Filtering string the user has typed into the input field.
*
* JavaScript Info:
* @property filter
* @type String
*
*/
@JsProperty void setFilter(String value);
/**
* Path for the value of the item. If items
is an array of objects, the
itemValuePath:
is used to fetch the string value for the selected
item.
* The item value is used in the value
property of the combo box,
to provide the form value.
*
* JavaScript Info:
* @property itemValuePath
* @type String
*
*/
@JsProperty String getItemValuePath();
/**
* Path for the value of the item. If items
is an array of objects, the
itemValuePath:
is used to fetch the string value for the selected
item.
* The item value is used in the value
property of the combo box,
to provide the form value.
*
* JavaScript Info:
* @property itemValuePath
* @type String
*
*/
@JsProperty void setItemValuePath(String value);
/**
* Closes the dropdown list.
*
* JavaScript Info:
* @method close
*
*
*/
void close();
/**
* Opens the dropdown list.
*
* JavaScript Info:
* @method open
*
*
*/
void open();
/**
* Reverts back to original value.
*
* JavaScript Info:
* @method cancel
*
*
*/
void cancel();
}