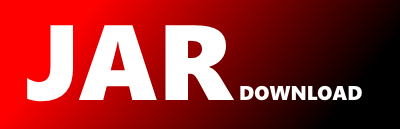
com.vaadin.collaborationengine.MapChangeEvent Maven / Gradle / Ivy
/*
* Copyright 2020-2022 Vaadin Ltd.
*
* This program is available under Commercial Vaadin Runtime License 1.0
* (CVRLv1).
*
* For the full License, see http://vaadin.com/license/cvrl-1
*/
package com.vaadin.collaborationengine;
import java.util.EventObject;
import java.util.Objects;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.JsonNode;
/**
* Event that is fired when the value in a collaboration map changes.
*
* @author Vaadin Ltd
* @since 1.0
*/
public class MapChangeEvent extends EventObject {
private final String key;
private final JsonNode oldValue;
private final JsonNode value;
/**
* Creates a new map change event.
*
* @param source
* the collaboration map for which the event is fired, not
* null
* @param change
* detail of the change, not null
*
* @since 1.0
*/
public MapChangeEvent(CollaborationMap source, MapChange change) {
super(source);
Objects.requireNonNull(change, "Entry change must not be null");
this.key = change.getKey();
this.oldValue = change.getOldValue();
this.value = change.getValue();
}
@Override
public CollaborationMap getSource() {
return (CollaborationMap) super.getSource();
}
/**
* Gets the updated map key.
*
* @return the updated map key, not null
*
* @since 1.0
*/
public String getKey() {
return key;
}
/**
* Gets the old value as an instance of the given class.
*
* @param type
* the expected type of the returned instance
* @param
* the type of the value from type
parameter, e.g.
* String
* @return the old map value, or null
if no value was present
* previously
*
* @since 1.0
*/
public T getOldValue(Class type) {
return JsonUtil.toInstance(oldValue, type);
}
/**
* Gets the old value as an instance corresponding to the given type
* reference.
*
* @param typeRef
* the expected type reference of the returned instance
* @param
* the type reference of the value from typeRef
* parameter, e.g. List>
* @return the old map value, or null
if no value was present
* previously
*
* @since 1.0
*/
public T getOldValue(TypeReference typeRef) {
return JsonUtil.toInstance(oldValue, typeRef);
}
/**
* Gets the new value as an instance of the given class.
*
* @param type
* the expected type of the returned instance
* @param
* the type of the value from type
parameter, e.g.
* String
* @return the new map value, or null
if the association was
* removed
*
* @since 1.0
*/
public T getValue(Class type) {
return JsonUtil.toInstance(value, type);
}
/**
* Gets the new value as an instance corresponding to the given type
* reference.
*
* @param typeRef
* the expected type reference of the returned instance
* @param
* the type reference of the value from `typeRef` parameter, e.g.
* List>
* @return the new map value, or null
if the association was
* removed
*
* @since 1.0
*/
public T getValue(TypeReference typeRef) {
return JsonUtil.toInstance(value, typeRef);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy