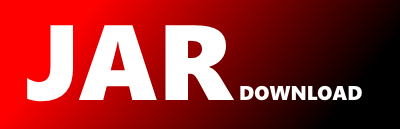
com.vaadin.spring.roo.addon.EntityProviderUtil-template Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.vaadin.spring.roo.addon Show documentation
Show all versions of com.vaadin.spring.roo.addon Show documentation
Spring Roo addon for creating rich internet applications with Vaadin.
The newest version!
package __ABSTRACT_ENTITY_VIEW_PACKAGE__;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.HashMap;
import java.util.Map;
import javax.persistence.EntityManager;
import com.vaadin.addon.jpacontainer.MutableEntityProvider;
import com.vaadin.addon.jpacontainer.provider.MutableLocalEntityProvider;
/**
* Utility class for constructing/obtaining a shared entity provider instance for an entity class.
*/
public class EntityProviderUtil {
private Map, MutableEntityProvider> providerMap = new HashMap, MutableEntityProvider>();
private static EntityProviderUtil instance;
private EntityProviderUtil() {
// singleton pattern
}
public static EntityProviderUtil get() {
if (null == instance) {
instance = new EntityProviderUtil();
}
return instance;
}
@SuppressWarnings("unchecked")
public MutableEntityProvider getEntityProvider(Class cls) {
synchronized (providerMap) {
if (!providerMap.containsKey(cls)) {
MutableLocalEntityProvider provider = buildEntityProvider(cls);
if (null != provider) {
providerMap.put(cls, provider);
}
}
return (MutableEntityProvider) providerMap.get(cls);
}
}
private MutableLocalEntityProvider buildEntityProvider(Class cls) {
EntityManager entityManager = getEntityManager(cls);
if (null != entityManager) {
MutableLocalEntityProvider provider = new MutableLocalEntityProvider(cls, entityManager);
// Spring should manage transactions - writable
provider.setTransactionsHandledByProvider(false);
return provider;
} else {
return null;
}
}
private EntityManager getEntityManager(Class cls) {
try {
Method method = cls.getMethod("entityManager");
if (null != method && (method.getModifiers() & Modifier.STATIC) != 0) {
return (EntityManager) method.invoke(null);
}
} catch (Exception e) {
throw new IllegalArgumentException("Could not obtain entity manager for the entity class "+cls.getCanonicalName(), e);
}
throw new IllegalArgumentException("Could not obtain entity manager for the entity class "+cls.getCanonicalName() + ": missing static method entityManager()");
}
}