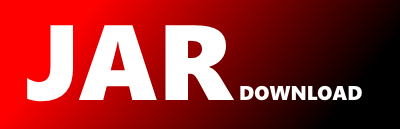
com.vaadin.buildhelpers.FetchReleaseNotesTickets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vaadin-buildhelpers Show documentation
Show all versions of vaadin-buildhelpers Show documentation
Vaadin is a web application framework for Rich Internet Applications (RIA).
Vaadin enables easy development and maintenance of fast and
secure rich web
applications with a stunning look and feel and a wide browser support.
It features a server-side architecture with the majority of the logic
running
on the server. Ajax technology is used at the browser-side to ensure a
rich
and interactive user experience.
The newest version!
/*
* Copyright 2000-2014 Vaadin Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.vaadin.buildhelpers;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.io.IOUtils;
public class FetchReleaseNotesTickets {
private static final String queryURL = "http://dev.vaadin.com/query?status=pending-release&status=released&@milestone@&resolution=fixed&col=id&col=summary&col=owner&col=type&col=priority&col=component&col=version&col=bfptime&col=fv&format=tab&order=id";
private static final String ticketTemplate = ""
+ "@badge@" //
+ "#@ticket@ " //
+ "@description@ " //
+ " "; //
public static void main(String[] args) throws IOException {
String versionsProperty = System.getProperty("vaadin.version");
if (versionsProperty == null || versionsProperty.equals("")) {
usage();
}
String milestone = "";
List versions = new ArrayList();
for (String version : versionsProperty.split(" ")) {
if (version.endsWith(".0") || version.matches(".*\\.rc\\d+")) {
// Find all prerelease versions for final or rc
// Strip potential rc prefix
version = version.replaceAll("\\.rc\\d+$", "");
versions.addAll(findPrereleaseVersions(version));
} else {
versions.add(version);
}
}
for (String version : versions) {
if (!milestone.equals("")) {
milestone += "&";
}
milestone += "milestone=Vaadin+" + version;
}
printMilestone(milestone);
}
private static List findPrereleaseVersions(String baseVersion) {
List versions = new ArrayList();
for (int i = 0; i < 50; i++) {
versions.add(baseVersion + ".alpha" + i);
}
for (int i = 0; i < 10; i++) {
versions.add(baseVersion + ".beta" + i);
}
for (int i = 0; i < 10; i++) {
versions.add(baseVersion + ".rc" + i);
}
return versions;
}
private static void printMilestone(String milestone)
throws MalformedURLException, IOException {
URL url = new URL(queryURL.replace("@milestone@", milestone));
URLConnection connection = url.openConnection();
InputStream urlStream = connection.getInputStream();
List tickets = IOUtils.readLines(urlStream);
for (String ticket : tickets) {
// Omit BOM
if (!ticket.isEmpty() && ticket.charAt(0) == 65279) {
ticket = ticket.substring(1);
}
String[] fields = ticket.split("\t");
if ("id".equals(fields[0])) {
// This is the header
continue;
}
String summary = fields[1];
summary = modifySummaryString(summary);
String badge = " ";
if (fields.length >= 8 && !fields[7].equals("")) {
badge = "Priority ";
} else if (fields.length >= 9 && fields[8].equalsIgnoreCase("true")) {
badge = "Vote ";
}
System.out.println(ticketTemplate.replace("@ticket@", fields[0])
.replace("@description@", summary)
.replace("@badge@", badge));
}
urlStream.close();
}
private static String modifySummaryString(String summary) {
if (summary.startsWith("\"") && summary.endsWith("\"")) {
// If a summary starts with " and ends with " then all quotes in
// the summary are encoded as double quotes
summary = summary.substring(1, summary.length() - 1);
summary = summary.replace("\"\"", "\"");
}
// this is needed for escaping html
summary = escapeHtml(summary);
return summary;
}
/**
* @since 7.4
* @param string
* the string to be html-escaped
* @return string in html-escape format
*/
private static String escapeHtml(String string) {
StringBuffer buf = new StringBuffer(string.length() * 2);
// we check the string character by character and escape only special
// characters
for (int i = 0; i < string.length(); ++i) {
char ch = string.charAt(i);
String charString = ch + "";
if ((charString).matches("[a-zA-Z0-9., ]")) {
// character is letter, digit, dot, comma or whitespace
buf.append(ch);
} else {
int charInt = ch;
buf.append("&");
buf.append("#");
buf.append(charInt);
buf.append(";");
}
}
return buf.toString();
}
private static void usage() {
System.err.println("Usage: "
+ FetchReleaseNotesTickets.class.getSimpleName()
+ " -Dvaadin.version=");
System.exit(1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy